Common Python Problems - [4] Scripts Without __main__
By JoeVu, at: Jan. 13, 2023, 8:40 p.m.
Estimated Reading Time: __READING_TIME__ minutes
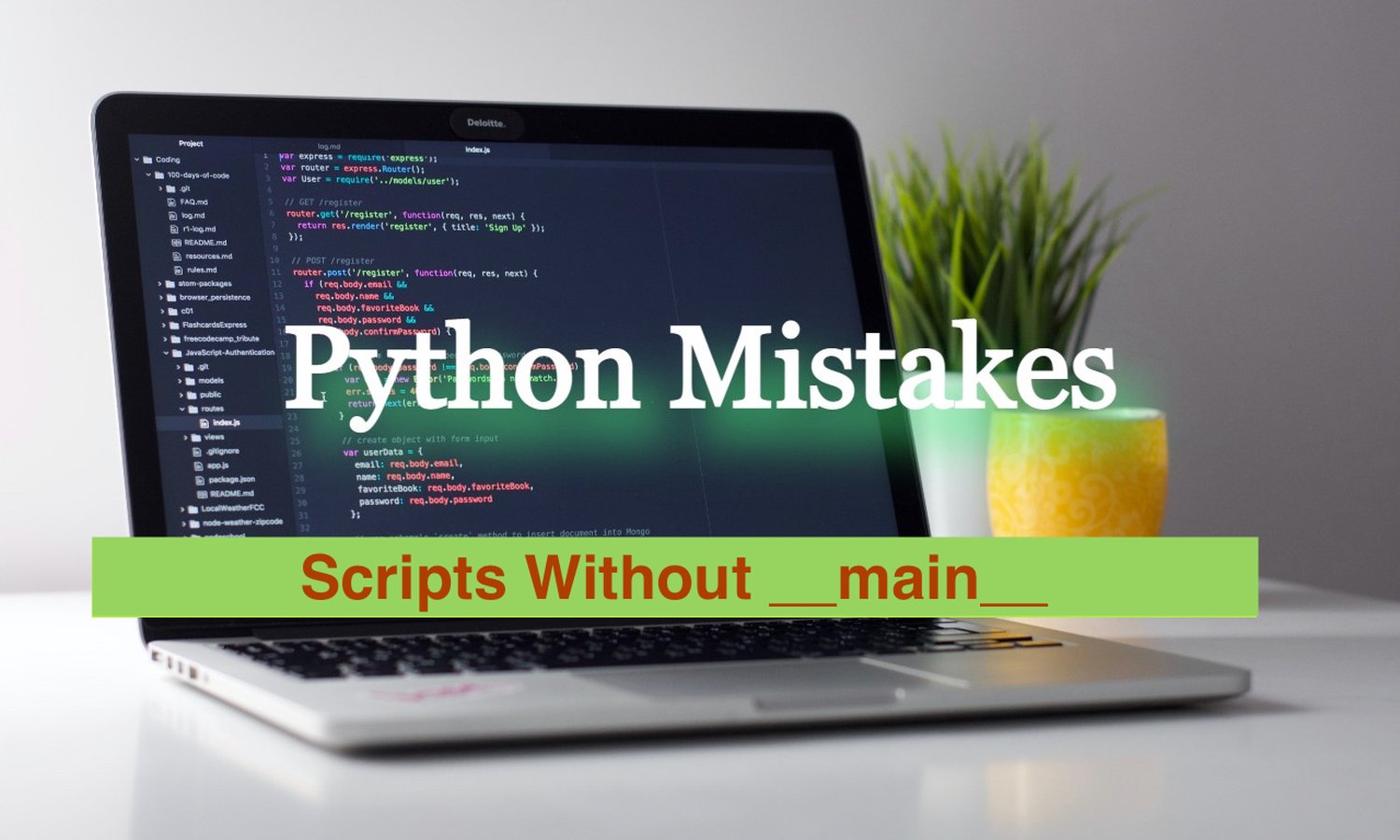
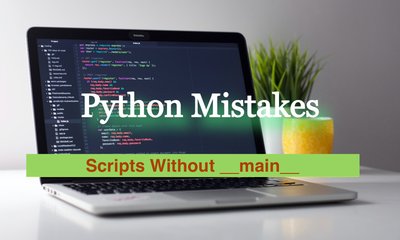
Python scripts often need to be run in order to perform specific tasks or to produce certain results. However, if the script is not written correctly, or if it doesn't include the __main__
statement, it will not be able to run properly. In this article, we'll discuss the common Python problems related to scripts without __main__
, and how to fix them.
What is __main__?
The __main__
statement is a special statement that is run when a script is executed. The line if __name__ == "__main__"
allows you to execute code when the file runs as a script, but not when it’s imported as a module
What is the potential problem with __main__?
Let consider the examples below with/out if __name__ == "__main__"
script_1.py
def greeting(name):
print(f"Hello {name}")
greeting("Joe")
script_2.py
import script_1
script_1.greeting("Snow")
Run
python script_1.py
python script_2.py
The expected output is
Hello Joe
Hello Snow
However, the actual output is
Hello Joe
Hello Joe
Hello Snow
Reason: When the script_1 is imported in the script_2, the script_1 source code is executed immediately. That's why the line "Hello Joe" is show 2 times in the result.
To fix this, we need to add the if __name__ == "__main__"
idiom to the script_1.py
script_1.py
def greeting(name):
print(f"Hello {name}")
if __name__ == "__main__":
greeting("Joe")
script_2.py
import script_1
if __name__ == "__main__":
script_1.greeting("Snow")
Reason: When you execute the script_2.py, it imports the script_1.py, however, in the script_1.py, the __name__ does not equal to __main__, __name__ now is "script_1". That's why the line greeting("Joe")
is not executed.
Conclusion
The __main__ statement is an important piece of code that is necessary for a Python script to be executed correctly. Without the __main__ statement, the script will not be able to run. If you have written a script without the __main__ statement, you should add it in order to ensure that the script is executable.