Common Python Problems - [5] Module Name Clash in Python
By JoeVu, at: Jan. 14, 2023, 2:40 p.m.
Estimated Reading Time: __READING_TIME__ minutes

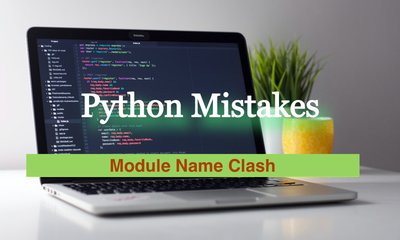
A module name clash occurs when two different modules have the same name. This can cause issues when trying to import one of the modules, as the interpreter won’t know which module to use. For example, if you have two modules called client.py”, the interpreter would not be able to tell which one to import.
1. In order to avoid name clashes, it’s important to give your modules unique names.
For example
libs/
google/
client.py
facebook/
client.py
and there is a script import both client.py
. Then you can simply name them differently, and the interpreter would have no trouble identifying them.
libs/
google/
google_client.py
facebook/
facebook_client.py
in the script.py, you can import them as
import libs.google.google_client
import libs.facebook.facebook_client
2. Another way to avoid module name clashes is to use the “as” keyword when importing modules. This allows you to assign an alias to the imported module, so that the interpreter can easily identify it. This is the preferred solution
Regarding to the above example, we can import both client module as
import libs.google.client as google_client
import libs.facebook.client as facebook_client
The interpreter would then be able to distinguish between the two modules, as they would each have a different alias.
3. Finally, you can also use the “importlib” module to dynamically load modules. This allows you to load modules at runtime, meaning that you can avoid any potential problems with module name clashes.
Same problem above, we can do
import importlib
google_client = importlib.import_module('libs.google.client')
facebook_client = importlib.import_module('libs.facebook.client')
The interpreter would then be able to distinguish between the two modules, as they would each have a unique name.
In conclusion, it is important to be aware of the potential for module name clashes when programming in Python. By giving your modules unique names, using the “as” keyword when importing, and using the “importlib” module to dynamically load modules, you can avoid any potential problems.