Common Python Problems - [7] List Mutation Inside Iteration
By JoeVu, at: Jan. 16, 2023, 3:42 p.m.
Estimated Reading Time: __READING_TIME__ minutes

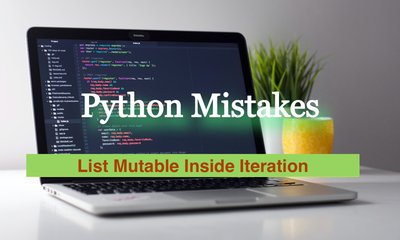
Python is a powerful programming language with many built-in functions and data structures. One of the most commonly used data structures is the list. Lists are mutable, which means they can be changed in place, and can be easily iterated over using loops. However, a common mistake that developers make when working with lists is performing list mutation inside a loop that iterates over the same list. This mistake can lead to unexpected behavior and bugs in your code. In this article, we will explore this mistake in more detail, and discuss how to avoid it in your Python code.
1. Understanding List Mutation
List mutation refers to the process of changing the contents of a list. This can be done using various methods, such as appending new elements, deleting existing elements, or modifying existing elements. In Python, lists are mutable, which means that they can be modified in place. This is different from immutable data structures, such as tuples and strings, which cannot be changed once they are created.
2. The Problem with List Mutation Inside Iteration
The problem with performing list mutation inside a loop that iterates over the same list is that it can lead to unexpected behavior.
Consider the following example:
fruits = ['apple', 'banana', 'orange', 'kiwi']
for fruit in fruits:
if fruit == 'banana':
fruits.remove(fruit)
In this code, we are iterating over the fruits list using a for loop. Inside the loop, we check if the current fruit is equal to 'banana', and if it is, we remove it from the list using the remove() method. However, this code will not behave as expected. Instead of removing just the 'banana' element from the list, it will also skip the 'orange' element. This is because when we remove an element from the list, the indices of the remaining elements are shifted down, and the loop continues with the next index, which is now the 'orange' element.
3. How to Avoid the List Mutation Inside Iteration Mistake
To avoid this mistake, you should never perform list mutation inside a loop that iterates over the same list. Instead, you should create a new list or use a list comprehension to generate a new list with the desired modifications. Here's an example of how to do this:
fruits = ['apple', 'banana', 'orange', 'kiwi']
# Create a new list with the 'banana' element removed
new_fruits = [fruit for fruit in fruits if fruit != 'banana']
In this code, we are using a list comprehension to create a new list called new_fruits. The comprehension iterates over the fruits list, and includes only the elements that are not equal to 'banana'. This creates a new list without modifying the original fruits list, and avoids the list mutation inside iteration mistake.
4. Conclusion
In conclusion, performing list mutation inside a loop that iterates over the same list is a common mistake that can lead to unexpected behavior in your Python code. To avoid this mistake, you should create a new list or use a list comprehension to generate a new list with the desired modifications. By following this best practice, you can avoid bugs and ensure that your code behaves as expected.