Common Python Problems - [8] Function Arguments By Reference And Value
By JoeVu, at: Jan. 17, 2023, 9:49 a.m.
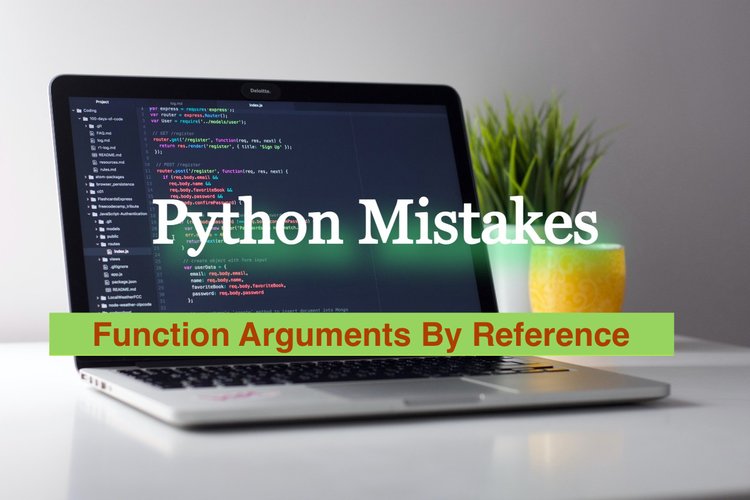
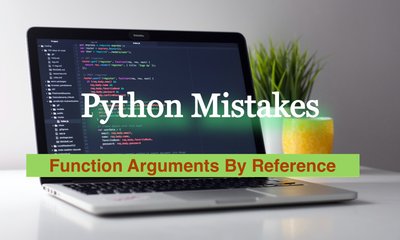
Python is a powerful programming language that supports a variety of programming paradigms. One of the most common paradigms in Python is object-oriented programming, which makes use of functions and methods to manipulate objects. However, a common mistake that many Python developers make is not understanding the difference between function arguments passed by reference and by value. In this article, we will discuss this common mistake in more detail, and provide guidance on how to avoid it.
1. Understanding Function Arguments in Python
Before we dive into the mistake, let's first understand how function arguments work in Python. In Python, when you pass an argument to a function, it can be passed by reference or by value. When an argument is passed by reference, the function can modify the original object. When an argument is passed by value, the function receives a copy of the original object and cannot modify the original object.
2. The Problem with Function Arguments Passed by Reference
The problem with function arguments passed by reference is that it can lead to unexpected behavior.
Consider the following example:
def add_element(my_list, element):
my_list.append(element)
fruits = ['apple', 'banana', 'orange']
add_element(fruits, 'kiwi')
print(fruits)
In this code, we define a function called add_element that takes a list and an element as arguments. The function appends the element to the list using the append() method. We then define a list called fruits, and call the add_element() function with fruits and the string 'kiwi' as arguments. Finally, we print the fruits list.
The output of this code will be ['apple', 'banana', 'orange', 'kiwi']. This is because the add_element() function modifies the original fruits list passed to it as an argument. This can be a problem if you intended to preserve the original list.
3. How to Avoid the Function Arguments by Reference Mistake
To avoid the mistake of modifying function arguments by reference, you can use the copy() method to create a copy of the original object before passing it to the function.
Here's an example:
def add_element(my_list, element):
my_list.append(element)
fruits = ['apple', 'banana', 'orange']
add_element(fruits.copy(), 'kiwi')
print(fruits)
In this code, we are calling the copy() method on the fruits list before passing it to the add_element() function. This creates a copy of the fruits list, which is passed to the function instead of the original fruits list. As a result, the original fruits list remains unchanged, and the output of this code will be ['apple', 'banana', 'orange'].
4. Conclusion
In conclusion, understanding the difference between function arguments passed by reference and by value is essential in Python programming. Modifying function arguments by reference can lead to unexpected behavior and bugs in your code. To avoid this mistake, you can use the copy() method to create a copy of the original object before passing it to the function. By following this best practice, you can ensure that your code behaves as expected and avoid common mistakes in Python programming.