Common Python Problems - [9] References, Copies, And Deep Copies
By JoeVu, at: Jan. 18, 2023, 10:24 a.m.
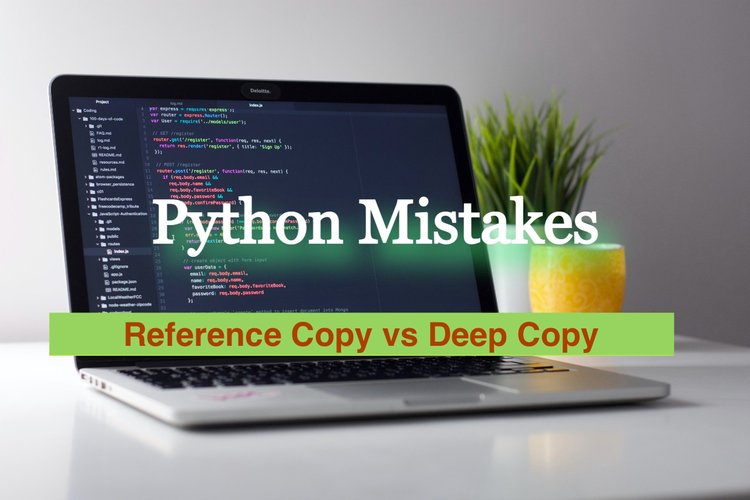
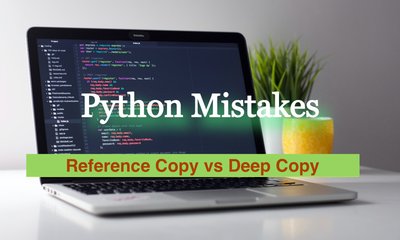
One of the key features of Python is the way it handles variables, which are often used to store data and other important information. However, when working with variables in Python, it is important to understand the differences between references, copies, and deep copies. In this article, we will explore these concepts and provide examples of how they work.
1. References in Python
In Python, a reference is a way to access an object in memory. When you create a variable in Python, you are essentially creating a reference to an object. For example, consider the following code:
original_list = [1, 2, 3]
new_list = original_list
In this code, we have created a list called "original_list" with three elements. We then create a new variable called "new_list" and assign it to "original_list". However, rather than creating a new copy of the list, "new_list" is simply a reference to the same list in memory. This means that if we modify the list using "original_list" or "new_list", the changes will be reflected in both variables.
original_list.append(4)
print(new_list) # Output: [1, 2, 3, 4]
In this code, we append the value "4" to the list using "original_list". When we print "new_list", we see that it has been updated to include the new element.
2. Copy in Python
A copy in Python is a new object that contains the same values as the original object. When you create a copy of an object, you are creating a new instance in memory with the same data as the original. In Python, there are two types of copies: shallow copies and deep copies.
2.1 Shallow Copy
A shallow copy in Python is a copy that contains references to the same object as the original. When you create a shallow copy of an object, you are creating a new object with references to the same data as the original. For example, consider the following code:
original_list = [1, 2, 3]
new_list = original_list.copy()
In this code, we create a new list called "new_list" using the copy() method of the original list "original_list". However, because this is a shallow copy, "new_list" still contains references to the same object as "original_list".
original_list.append(4)
print(new_list) # Output: [1, 2, 3]
In this code, we append the value "4" to the list using "original_list". When we print "new_list", we see that it has not been updated to include the new element. This is because "new_list" is a copy of "original_list" and contains references to the same object.
Advantages
- Avoid creating unnecessary copies of objects.
- Create aliases for variables, which can make code easier to read and write.
Disadvantages
- Can be tricky to use because changes made to the original object will also affect all of the references to that object
2.2 Deep Copy
A deep copy in Python is a copy that contains new object with the same values as the original. When you create a deep copy of an object, you are creating a new instance in memory with new object that contain the same data as the original. For example, consider the following code:
import copy
original_list = [1, 2, 3]
new_list = copy.deepcopy(original_list)
In this code, we create a new list called "new_list" using the deepcopy() method from the copy module. This creates a new list in memory with new objects that contain the same data as the original.
original_list.append(4)
print(new_list) # Output: [1, 2, 3]
In this code, we append the value "4" to the list using "original_list". When we print "new_list", we see that it has not been updated to include the new element. This is because new_list is a deep copy of "original_list" and contains new objects with the same data.
Advantages
- Can be useful when you want to create a new object with some shared data, but with other data that is unique to the new object.
- Can be useful when you want to create a completely new object with no shared data.
Disadvantages
- Can be memory-intensive and can slow down your code if you are creating many copies of large objects.
- Can slow down your code if you are creating many copies of large objects.
3. Conclusion
In conclusion, understanding references, copy, and deep copy is important when working with variables in Python.
Shallow Copy can be useful when you want to create a new object that is identical to the original object, but can be memory-intensive and slow down your code if you are creating many copies of large objects.
Meanwhile, Deep Copy are useful when you want to create a completely new object with no shared data, but can also be memory-intensive and slow down your code if you are creating many copies of large objects.