Common Python Problems - [6] Unstoppable Scripts
By JoeVu, at: Jan. 15, 2023, 3:02 p.m.
Estimated Reading Time: __READING_TIME__ minutes

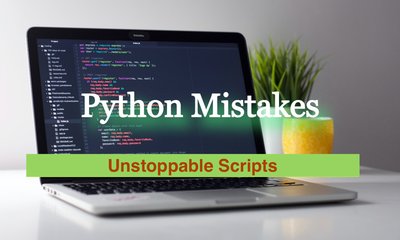
1. Introduction
1.1 Explanation of Python scripting
Python scripting is the process of writing programs in the Python programming language that can be executed without the need for compiling the code. Python scripting is widely used in various fields such as web development, scientific computing, data analysis, and automation.
1.2 Advantages of Python scripting
Python scripting is an efficient way of automating repetitive tasks, reducing manual intervention, and increasing productivity. Python scripting is easy to learn, and its syntax is simple and straightforward. Python scripting also has a large number of libraries and modules that can be used for various purposes, making it a versatile language.
2. Unstoppable Scripts
2.1 Definition of Unstoppable Scripts
Unstoppable scripts are scripts that can continue to run and perform their designated tasks despite encountering errors or exceptions. Unstoppable scripts are useful when dealing with tasks that are time-sensitive or require continuous monitoring and execution.
2.2 Advantages of Unstoppable Scripts
Unstoppable scripts can ensure that important tasks are completed regardless of errors or exceptions. This can be particularly useful in scenarios where manual intervention may not be possible, such as during system maintenance or when performing critical operations.
3. Using try-except block
3.1 Explanation of try-except block
The try-except block is a feature in Python that allows you to handle exceptions in code. When an error occurs, the code in the try block stops executing, and the code in the except block is executed instead. This allows you to gracefully handle errors and continue executing the script.
3.2 Code snippet for try-except block
try:
# code that might raise an exception
except Exception as e:
# handle the exception here
4. Example of an unstoppable script
4.1 Code snippet for an unstoppable script
import time
while True:
try:
# code that might raise an exception
time.sleep(10)
except Exception as e:
# handle the exception here
print("An error occurred: ", e)
4.2 Explanation of the code snippet
In this code snippet, we have a while loop that will continue to run indefinitely. Inside the loop, we have a try-except block that contains the code we want to run. In this example, we are using the time.sleep() function to pause the execution of the script for 10 seconds. This code will continue to run even if an exception is raised, and the except block will print the error message to the console.
5. Dangers of Unstoppable Scripts
5.1 Explanation of potential dangers
Unstoppable scripts can be dangerous if they are not designed and implemented properly. If a script continues to run despite encountering errors or exceptions, it can cause further problems or damage to the system. Unstoppable scripts can also consume system resources, leading to system instability and crashes.
5.2 How to use Unstoppable Scripts safely
To use unstoppable scripts safely, it is important to ensure that the code is designed to handle exceptions gracefully and that the script has a way to stop execution if necessary. It is also important to monitor the script and the system it is running on to ensure that it is not causing any issues or consuming too many resources.
6. Conclusion
6.1 Recap of main points
Python scripting is an efficient way of automating tasks, and unstoppable scripts can ensure that critical tasks are completed even if errors or exceptions are encountered. The try-except block is a feature in Python that allows you to handle exceptions gracefully, and it can be used to create unstoppable scripts safely.
6.2 Final thoughts
Unstoppable scripts can be a powerful tool when used correctly, but they should be used with caution. It is important to design and implement the script properly, monitor its execution, and be prepared to stop it if necessary.