Advance Python Unit Testing - Optimising Performance
By JoeVu, at: Nov. 27, 2022, 7:41 p.m.
Estimated Reading Time: __READING_TIME__ minutes

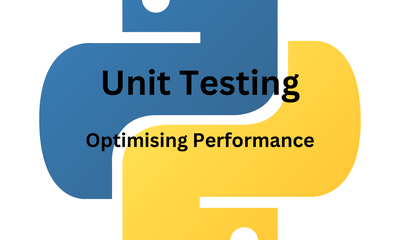
Advanced Unit Testing in Python - Optimizing Performance
Unit testing is an essential part of software development, but as the size of the test suite grows, the time it takes to run the tests can become a bottleneck in the development process. In this post, we will explore some advanced techniques for optimizing the performance of unit tests in Python.
Test Selection
One way to optimize the performance of unit tests is to run only the tests that are relevant to the changes made. This is known as test selection. Python's unittest
module includes a test runner called unittest discover
that supports test selection.
To run only the relevant tests, you can use a tool like pytest-watch
. This tool watches the code for changes and automatically runs the relevant tests. This saves time by avoiding the need to run the entire test suite every time a change is made.
For example, let's say you have a test suite with 1000 tests, and you make a change to a single test. Instead of running all 1000 tests, you can use pytest-watch
to run only the changed test, saving time and resources.
# Install pytest-watch
pip install pytest-watch
# Run pytest-watch
ptw
Test Parallelization
Another way to optimize the performance of unit tests is to run them in parallel. This can be especially effective if the test suite is large and takes a long time to run. Python's unittest
module includes a test runner called unittest runner
that supports test parallelization.
To run tests in parallel, you can use a tool like pytest-xdist
. This tool splits the test suite into multiple processes and runs them in parallel. This saves time by utilizing the available processing power and running the tests faster.
For example, let's say you have a test suite with 1000 tests, and it takes 10 minutes to run the entire suite. By using pytest-xdist
with 4 workers, you can run the tests in 2.5 minutes.
# Install pytest-xdist
pip install pytest-xdist
# Run tests in parallel with 4 workers
pytest -n 4
Test Isolation
Test isolation is the practice of running each test in its own environment to prevent interference between tests. This can be especially important if the tests rely on external dependencies like databases or web services. Python's unittest
module includes a test runner called unittest runner
that supports test isolation.
To run tests in isolation, you can use a tool like pytest-mock
. This tool creates a new mock object for each test, ensuring that each test runs in its environment. This saves time by avoiding the need to set up and tear down the environment for each test.
For example, let's say you have a test suite that relies on a database connection. Without test isolation, each test would need to set up and tear down the database connection, which can be time-consuming. By using pytest-mock
, each test can run in its environment, and the database connection can be mocked, saving time and resources.
# Install pytest-mock
pip install pytest-mock
# Use pytest-mock in a testimport pytest
from unittest.mock import Mock
def test_database_connection(mocker):
# Mock the database connection
mock_connection = Mock()
mocker.patch('database.connect', return_value=mock_connection)
# Run the test
assert database.connect() == mock_connection
Code Coverage
Code coverage is a measure of how much of the code is covered by the tests. By measuring code coverage, you can identify areas of the code that are not being tested and prioritize the testing efforts accordingly. Python's unittest
module includes a code coverage tool called coverage.py
.
To measure code coverage, you can run the tests with the coverage
command:
# Install coverage.py
pip install coverage
# Run tests with coverage
coverage run -m unittest discover
You can then generate a report of the code coverage with the coverage report
command:
# Generate a report of code coverage
coverage report
This report shows the percentage of code covered by the tests and identifies the areas of the code that need more testing.
For example, let's say you have a codebase with 1000 lines of code, and the code coverage report shows that only 80% of the code is covered by the tests. You can use this information to prioritize testing efforts and write more tests to increase code coverage.
Conclusion
Optimizing the performance of unit tests is an important part of software development. By using advanced techniques like test selection, test parallelization, test isolation, and code coverage, you can speed up the test suite and catch errors early in the development cycle. These techniques can save time and resources, making the development process more efficient and effective.