Arguments Passing In Python
By JoeVu, at: March 6, 2023, 9:29 p.m.
Estimated Reading Time: __READING_TIME__ minutes

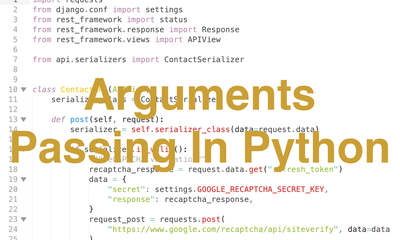
Arguments Passing In Python
When developing with Python, developers often need to pass parameters when calling functions. It is important to understand how parameters are passed in Python, as this can have a significant impact on the code you write. This can result in a hidden bug in your code and hard to find.
1. All parameters (arguments) in the Python language are passed by reference.
It means if you change what a parameter refers to within a function, the change also reflects back in the calling function.
Let’s look at this example below
def double_price(number):
number *= 2
return number
original_number = 5
new_number = double_price(original_number)
print(original_number) # 10
print(new_number) # 10
In this example, the parameter number is a reference to the argument original_number, which is set to 5.
When the double_price function is called, the value of number is set to 5.
Then, the number is multiplied by 2 to 10 and returned.
Because number is a reference to original_number, it is also set to 10. As you can see, original_number and new_number both equal 10, because number was a reference to original_number.
The main advantage of passing parameters by reference is that it allows you to modify the argument outside the function, without having to return a value. This can be useful in certain situations, such as when you want to modify a list in-place.
2. However, passing parameters by reference can also be dangerous.
Because the parameter is a reference to the argument, any changes to the parameter inside the function will be reflected in the argument outside the function. This can lead to unexpected behavior and can be difficult to debug.
Let’s look at an example. Say we have a list of numbers, and we want to pass it to a function that squares each number and returns the result. We might write function like this:
def square_list(nums):
for i in range(len(nums)):
nums[i] = nums[i] * nums[i]
return nums
If we pass our list of numbers to this function by reference, calling it like this:
my_list = [1, 2, 3]
square_list(my_list)
After the function is finished running, the value of my_list will be [1, 4, 9], not [1, 2, 3] as we might expect. This is because the square_list function changed the original list object, rather than creating a new one.
If we wanted the original list to remain unchanged, we could pass it by value instead of by reference. To do this, we could write the square_list function like this:
def square_list(nums):
new_list = []
for i in range(len(nums)):
new_list.append(nums[i] * nums[i])
return new_list
Now, if we call the square_list function like before, the value of my_list will remain unchanged, and the function will return a new list with the squared values.
When passing arguments to functions, it’s important to keep in mind that passing by reference can be dangerous. If you’re not sure whether a function will modify the original object, it’s best to pass it by value instead.