Basics of Vue.js: Lifecycle, Data Binding and Event Handling
By khoanc, at: May 12, 2024, 6:02 p.m.
Estimated Reading Time: __READING_TIME__ minutes

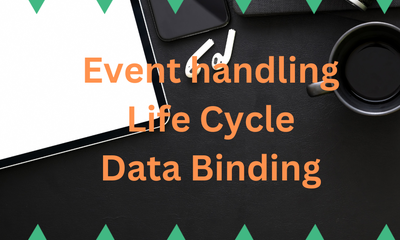
Basics of Vue.js: Lifecycle, Data Binding and Event Handling
Introduction
Vue.js stands as a popular JavaScript framework for building interactive and dynamic user interfaces. Its simplicity paired with powerful features makes it a go-to choice for developers. In this guide, we delve into the core concepts of Vue.js: the Vue instance and its lifecycle, data binding and event handling, as well as how to employ conditionals and loops to create dynamic content. Whether you’re starting your journey in web development or looking to enhance your Vue.js skills, this post is tailored for you.
Understanding the Vue Instance and Lifecycle Hooks
First, we establish the base Vue instance that will be referenced throughout this section:
var app = new Vue({
el: '#app',
data: {
message: 'Glinteco is an IT outsourcing company!',
updatedMessage: 'Glinteco is professional and responsible!'
},
methods: {
updateMessage() {
this.message = this.updatedMessage;
}
}
})
Lifecycle Hooks
Created
The created
hook is called right after the instance has been initialized. This hook is used for code that needs to run as soon as the data is set up but before the instance is mounted to the DOM. It's ideal for initializing data or making API calls.
created: function() {
console.log('Component is created. Message is: ' + this.message);
}
Explanation: At this stage, data observability is set up, and properties such as data
, computed
, methods
, and watchers
are available and can be used or modified.
Mounted
The mounted
hook is called after the instance has been mounted, meaning the template has been rendered into the DOM. It's used for DOM manipulation or when you need to access the rendered DOM (e.g., to use third-party JavaScript libraries).
mounted: function() { console.log('Component is mounted to the DOM.'); }
Explanation: At this point, your component has been fully initialized and inserted into the DOM. It's the right place to access or manipulate your component’s DOM directly.
Updated
The updated
hook is called after data changes on your component cause the DOM to re-render. Note that this hook does not guarantee that all child components have also been re-rendered. It's typically used to perform actions after the component has updated.
updated: function() { console.log('Component has been updated with new data.'); }
Explanation: This hook is particularly useful for performing post-patch DOM operations or updates after Vue has completed the DOM updates triggered by data changes.
Destroyed
The destroyed
hook is called after a Vue instance has been destroyed. This includes the teardown of watchers, child components, and event listeners. It's useful for cleanup activities.
destroyed: function() { console.log('Component has been destroyed.'); }
Explanation: Use this hook to perform any cleanup tasks or to release resources that were set up in the earlier lifecycle stages (like removing event listeners or cancelling timers).
Putting It All Together
Integrating these hooks into our Vue instance, we get:
var app = new Vue({
el: '#app',
data: {
message: 'Vue is amazing!',
updatedMessage: 'Vue is fantastic!'
},
methods: {
updateMessage() {
this.message = this.updatedMessage;
}
},
created: function() {
console.log('Component is created. Message is: ' + this.message);
},
mounted: function() {
console.log('Component is mounted to the DOM.');
},
updated: function() {
console.log('Component has been updated with new data.');
},
destroyed: function() {
console.log('Component has been destroyed.');
}
});
These detailed explanations and coding snippets provide a deeper insight into how the Vue instance and lifecycle hooks work, enabling you to control your components effectively throughout their lifecycle.
Data Binding in Vue.js
Data binding is a powerful feature of Vue.js that automatically synchronizes the data in your Vue components with the user interface. Vue.js offers several ways to bind data to your templates, but the most common are:
- Interpolation: Using the "Mustache" syntax (
{{ }}
) for text. - Directives: Special tokens in the markup that tell the library to do something to a DOM element.
Interpolation
Text interpolation allows you to embed expressions within your HTML:
< div id="app" > < p >{{ message }}< / p > < / div >
JS Code
var app = new Vue({ el: '#app', data: { message: 'Hello Vue!' } });
Explanation: This snippet will render "Hello Vue!" inside the paragraph. If message
changes, the content of the paragraph will automatically update to reflect the new value.
v-bind
For binding element attributes, use v-bind
(or simply :
):
var app = new Vue({ el: '#app', data: { url: 'https://vuejs.org/' } });
Explanation: This binds the href
attribute of the anchor tag to the url
data property. If url
changes, the href
attribute will automatically update.
Event Handling
Vue.js allows you to listen to DOM events and execute JavaScript when they're triggered using the v-on
directive (or @
for shorthand).
Listening to Events
To react to an event, use the v-on
directive:
var app = new Vue({
el: '#app',
methods: {
greet: function(event) {
alert('Hello Vue!');
if (event) alert(event.target.tagName);
}
}
})
Explanation: This binds a click event to the greet
method. When the button is clicked, it alerts "Hello Vue!" and then alerts the tag name of the event target.
Method Event Handlers
Methods in the methods
object can be used as event handlers. Arguments can be passed to these methods, including the native event object:
# Pass the native event object
< button v-on:click="greet($event)" >Greet with Event< / button >
Two-Way Data Binding with v-model
Vue.js also provides the v-model
directive, which creates a two-way binding on form input, textarea, and select elements. It automatically picks the correct way to update the element based on the input type.
< div id="app">
< input v-model="message">
< p >The message is: {{ message }}< / p >
< / div >
JS code
var app = new Vue({
el: '#app',
data: {
message: ''
}
});
Explanation: This example binds the value of the input to the message
data property. Any changes to the input will immediately update message
, and any changes to message
will update the input's value.
Conditionals in Vue.js
Vue.js provides powerful directives to conditionally render elements in the DOM: v-if
, v-else-if
, v-else
, and v-show
. These directives offer flexibility in displaying content based on the state of your data.
v-if, v-else-if, v-else
The v-if
directive allows you to conditionally render a block. Blocks with v-else-if
and v-else
are only allowed immediately following a v-if
or another v-else-if
block.
< p v-if="seen" >Now you see me
Explanation: Depending on the value of type, a different paragraph will be displayed. This approach is ideal for exclusive conditions within your application's UI.
v-show
The v-show directive toggles the display CSS property of the element based on the truthiness of the expression provided. Unlike v-if, elements with v-show are always rendered and remain in the DOM; they are simply hidden if the condition is false.
< div id="app"> < p v-show="isVisible">Hello!
JS Code
var app = new Vue({ el: '#app', data: { isVisible: true } });
Explanation: This snippet uses v-show to control the visibility of the paragraph. It's efficient for toggling visibility on/off without inserting/removing elements from the DOM.
Loops in Vue.js
Vue.js uses the v-for directive to render a list of items based on an array. It’s powerful for generating dynamic lists in your application.
v-for
Use v-for to bind an array of items to elements:
< div id="app"> < ul> < li v-for="item in items" :key="item.id"> {{ item.message }}
JS Code
var app = new Vue({ el: '#app', data: { items: [ { id: 1, message: 'Foo' }, { id: 2, message: 'Bar' } ] } });
Explanation: This code renders a list of items as an unordered list in HTML. Each item in the items
array is mapped to an element. The :key
is used to maintain the identity of each component in the list, which is essential for efficient updates.
Conclusion
These expanded explanations and code snippets provide a deeper understanding of data binding and event handling, application life cycle in Vue.js, illustrating how these mechanisms work together to create interactive and responsive web applications. By mastering these concepts, developers can efficiently develop dynamic user interfaces that react seamlessly to user inputs and changes in application data.