Best Practices for Setting up a Flask Web Application: A Comprehensive Guide
By JoeVu, at: May 8, 2023, 9:19 a.m.
Estimated Reading Time: __READING_TIME__ minutes

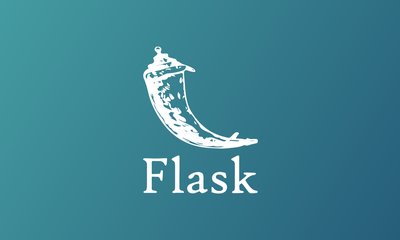
1. Introduction
Flask, that is a popular micro Python web framework for building web applications with Python, is known for its simplicity, flexibility, and ease of use. This framework provides a lightweight and minimalist approach to web development, allowing developers to quickly build web applications without being constrained by a complex architecture.
However, setting up a Flask application can be challenging for both beginners and experienced developers. In this article, we will provide a comprehensive guide to sett up a Flask application with best practices (recommended by the community and blog posts). We will cover everything such as caching, logging, REST API design, background tasks, and unit testing with Pytest. By the end of this article, you will have a solid understanding of how to build a scalable, maintainable Flask application that adheres to best practices.
Before you go further, please check our sample code for a simple Flask application
1.1 Brief Explanation of Flask
As a lightweight and flexible Python web framework that provides developers with the tools they need to build web applications faster. It is popular among developers due to its simplicity and ease of use, making it an excellent choice for small to medium-sized projects.
One of Flask's primary strengths is its flexibility
. Flask provides developers with a simple and intuitive API
, allowing them to customize and extend
their application as needed. Flask also provides several built-in features
, such as integrated support for testing and debugging, that can help speed up the development process.
1.2 Importance of Following Best Practices when Setting up a Flask Application
While Flask is easy to use and flexible, it is essential to follow best practices when setting up a Flask application to ensure its scalability, maintainability, and security. By following best practices, you can build a Flask application that is reliable, efficient, and easy to maintain.
Some of the best practices to follow when setting up a Flask application include using blueprints to modularize your application, implementing caching to improve performance, using environment variables to store sensitive information, and writing clean and readable code.
In addition to following best practices, there are several helpful packages and extensions available for Flask that can help simplify the development process. Some popular packages and extensions include Flask-SQLAlchemy for database integration, Flask-RESTful for building RESTful APIs, Flask-Login for authentication and authorization, and Flask-Caching for caching.
In the next sections, we will dive deeper into these best practices and packages to help you build a robust and scalable Flask application.
2. Virtual Environment Setup
2.1 Explanation of Virtual Environment Setup
Virtual environments are an essential tool for Python development. They allow you to create an isolated environment where you can install Python packages and dependencies without affecting the system-wide installation of Python. This ensures that your application will work consistently, regardless of the Python version or installed packages on the host machine.
2.2 Creating a Virtual Environment with pyenv, pip, and requirements.txt
To create a virtual environment, we will use `pyenv`, `pip`, and `requirements.txt` file.
Install pyenv
using the following command
curl https://pyenv.run | bash
Once pyenv
is installed, we can create a new virtual environment for our Flask application using the following command
pyenv virtualenv <python_version> <environment_name></environment_name></python_version>
Replace <python_version> with the version of Python you want to use, and <environment_name> with a name for your virtual environment.</environment_name></python_version>
Activate the virtual environment using the following command
pyenv activate <environment_name></environment_name>
Create a requirements.txt file in your project directory with the following contents
Flask==2.3.0
requests==2.2.0
Add any other required packages and dependencies to this file.
Install the required packages using pip
pip install -r requirements.txt
2.3 Installing Flask and Necessary Dependencies
Once you have created and activated your virtual environment, you can install Flask and any other necessary dependencies using pip. To install Flask, simply run the following command:
pip install Flask
This will install the latest version of Flask, along with any required dependencies. If you need a specific version of Flask, you can specify it using the == operator, like this:
pip install Flask==<version></version>
By setting up a virtual environment and installing Flask and its dependencies, you can ensure that your application will work consistently, regardless of the Python version or installed packages on the host machine.
3. Environment Variables Setup
3.1 Explanation of Environment Variables
Environment variables are variables that are set in the operating system and can be accessed by programs running on the system. In Flask, environment variables are often used to store sensitive information such as database passwords, API keys, and other configuration values.
3.2 Setting up Environment Variables
To set up environment variables for your Flask application, you can use a variety of methods depending on your operating system and deployment environment. Some common ways to set up environment variables include:
1. .env
file
A .env
file is a simple text file that contains key-value pairs of environment variables. You can use the python-dotenv package to load these variables into your Flask application.
Example .env
file
SECRET_KEY=your_secret_key
DB_HOST=your_database_host
DB_NAME=your_database_name
DB_USER=your_database_user
DB_PASS=your_database_password
To load the variables from this file, you can add the following code to your Flask application:
from dotenv import load_dotenv
import os
# Load environment variables from .env file
load_dotenv()
# Access environment variables
secret_key = os.getenv('SECRET_KEY')
db_host = os.getenv('DB_HOST')
db_name = os.getenv('DB_NAME')
db_user = os.getenv('DB_USER')
db_pass = os.getenv('DB_PASS')
Recommended packages: python-dotenv
2. JSON file
You can also use a JSON file to store environment variables for your Flask application. To load variables from a JSON file, you can use the json package that comes with Python.
Example JSON file
{
"SECRET_KEY": "your_secret_key",
"DB_HOST": "your_database_host",
"DB_NAME": "your_database_name",
"DB_USER": "your_database_user",
"DB_PASS": "your_database_password"
}
To load the variables from this file, you can add the following code to your Flask application
import json
import os
# Load environment variables from JSON file
with open('config.json') as f:
config = json.load(f)
# Access environment variables
secret_key = config['SECRET_KEY']
db_host = config['DB_HOST']
db_name = config['DB_NAME']
db_user = config['DB_USER']
db_pass = config['DB_PASS']
3. TOML file
You can also use a TOML file to store environment variables for your Flask application. To load variables from a TOML file, you can use the toml package.
Example TOML file
SECRET_KEY = "your_secret_key"
DB_HOST = "your_database_host"
DB_NAME = "your_database_name"
DB_USER = "your_database_user"
DB_PASS = "your_database_password"
To load the variables from this file, you can add the following code to your Flask application
import toml
import os
# Load environment variables from TOML file
config = toml.load('config.toml')
# Access environment variables
secret_key = config['SECRET_KEY']
db_host = config['DB_HOST']
db_name = config['DB_NAME']
db_user = config['DB_USER']
db_pass = config['DB_PASS']
Regardless of the method you choose, it's important to make sure that sensitive information is kept secure and not exposed in any publicly accessible code or files.
4. Database Setup
4.1 Explanation of Flask database
A database is an essential component of most web applications as it stores the data that the application works with. Flask supports several databases including PostgreSQL, MySQL, SQLite, and others. Flask uses SQLAlchemy, a popular Object-Relational Mapping (ORM) library that provides a high-level interface for interacting with databases. SQLAlchemy makes it easy to work with databases, and it abstracts away much of the complexity of working with raw SQL.
4.2 Choosing a database engine - SQLAlchemy
The choice of a database engine depends on the requirements of the application. PostgreSQL and MySQL are popular choices for Flask applications due to their features, performance, and reliability. After choosing a database engine, the next step is to create a database and connect to it using Flask-SQLAlchemy. SQLAlchemy provides a consistent interface to connect to different databases, and it supports many database engines.
Sample json config file
{
"SECRET_KEY": "randon-secret-123",
"CELERY": {
"broker_url": "redis://localhost:6379/0",
"result_backend": "redis://localhost:6379/0",
"task_ignore_result": false,
"imports": ["tasks"],
"worker_redirect_stdouts_level": "CRITICAL"
},
"REDIS_URL": "redis://localhost:6379/1",
"SQLALCHEMY_DATABASE_URI": "postgresql://samples:password@localhost:5432/samples"
}
4.3 Creating database models
After connecting to the database, the next step is to create database models. A database model defines the structure of a database table, including its fields and relationships with other tables. In Flask, you can define database models using Flask-SQLAlchemy, which is a powerful SQL toolkit and ORM.
Here is an example of a simple database model
from app import db
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=False)
email = db.Column(db.String(120), unique=True, nullable=False)
password = db.Column(db.String(80), nullable=False)
def __repr__(self):
return '
<user>' % self.username
</user>
In the above example, we define a User model with four fields: id, username, email, and password. The id field is the primary key for the table, while username and email fields are unique and cannot be null.
4.4 What is Database Migration? Why do we need that?
Database migration is the process of updating the structure of a database schema without losing any existing data. Database migration is important because:
- As the application evolves, the database schema may need to change to accommodate new features or changes in business requirements.
- Migrations help to keep track of changes to the database schema over time and make it easier to roll back changes if necessary.
- Migrations enable a team of developers to collaborate on the database schema and ensure consistency across different environments (development, testing, production).
4.5 Recommended packages for Flask database migration: Flask-migrate (pros and cons)
Flask-Migrate is a Flask extension that provides a simple way to handle database migrations using SQLAlchemy. It offers a simple way to upgrade and downgrade your database schema.
To install Flask-Migrate, use the following command
To use Flask-Migrate in your Flask application, you need to initialize it with the following command
After that, you can create migration scripts by running the following command
flask db migrate -m "migration message"
To apply the migration to the database, use the following command
flask db upgrade
Here are some useful command lines to work with Flask-Migrate
flask db init
: Initializes the migration environment.
flask db migrate
: Generates a new migration script based on changes to the database model.
flask db upgrade
: Applies the migration to the database.
flask db downgrade
: Rolls back the last migration.
flask db history [--rev-range REV_RANGE] [--verbose]
: Shows the list of migrations. If a range isn’t given then the entire history is shown.
flask db stamp [--sql] [--tag TAG]
<revision>: Sets the revision in the database to the one given as an argument, without performing any migrations. This is EXTREMELY USEFUL when you have troubles with inconsistent database migration states.</revision>
Some common problems that developers may face when using Flask-Migrate include
- Conflicts between migration scripts: When two developers work on different features, they may both generate a migration script that conflicts with the other. To resolve this, developers should communicate frequently and merge their migration scripts before applying them to the database.
- Data loss: If a migration script is not written properly, it may cause data loss during the upgrade process. It is important to test migration scripts thoroughly before applying them to the production database.
5. Cache Setup and Test
5.1 Explanation of Flask Cache and When to Use Cache
Flask-Cache is a simple and lightweight caching library that helps you cache the results of expensive operations in memory or on disk. By caching data, you can improve the performance of your Flask application and reduce the response time for your users.
Cache is particularly useful for frequently accessed data that doesn't change often. This includes data that comes from expensive queries, data that is computationally expensive to generate, and data that is static and doesn't change often.
5.2 Cache Pros and Cons
Pros
- Improved performance and reduced response time for users
- Caching frequently accessed data can help reduce load on the database
- Caching can help speed up computationally expensive operations
Cons
- Cached data can become stale if it's not updated frequently
- Caching can take up memory or disk space, depending on the cache configuration
- Caching can add complexity to your code and make it harder to reason about
5.3 Examples of Using Cache
Here are a few examples of how you can use Flask Cache
- Caching function results
from flask import Flask
from flask_caching import Cache
app = Flask(__name__)
cache = Cache(app, config={'CACHE_TYPE': 'simple'})
@app.route('/hello')
@cache.cached(timeout=60)
def hello():
return 'Hello, World!'
In this example, the @cache.cached
decorator is used to cache the result of the hello function for 60 seconds.
- Caching expensive database queries
from flask import Flask, request
from flask_caching import Cache
from sqlalchemy import create_engine
app = Flask(__name__)
cache = Cache(app, config={'CACHE_TYPE': 'simple'})
db = create_engine('postgresql://user:password@localhost/mydatabase')
@app.route('/users')
def get_users():
user_id = request.args.get('id')
cache_key = f'user_{user_id}'
cached_data = cache.get(cache_key)
if cached_data:
return cached_data
else:
result = db.execute(f"SELECT * FROM users WHERE id = {user_id}").fetchall()
cache.set(cache_key, result, timeout=60)
return result
In this example, the cache.get
method is used to check if the result of the query is already cached. If it is, the cached data is returned immediately. Otherwise, the query is executed and the result is cached for 60 seconds using the cache.set
method.
5.4 Common mistakes with Flask cache
When working with Flask cache, there are some common mistakes that developers should avoid.
Here are a few examples
- Caching too much data: It can be tempting to cache all data, but this can lead to performance issues and potentially even a crash. It's important to be selective about what data is cached and how long it should be cached.
- Not setting a timeout: Without a timeout, the cache will keep data indefinitely, which can lead to stale data being returned. It's important to set an appropriate timeout to ensure the cache is refreshed regularly.
- Not handling cache failures: Caching can fail for various reasons, such as when the cache server goes down or runs out of memory. It's important to handle these failures gracefully, such as by falling back to another cache or by recomputing the data if necessary.
- Not considering cache invalidation: When data is updated, the cache needs to be invalidated to ensure that the latest data is returned. It's important to have a mechanism in place for invalidating the cache when data changes.
By avoiding these common mistakes, developers can make the most of Flask cache and improve the performance of their applications.
6. Logging Setup
6.1 Explanation of Flask Logging
Flask logging is used to record events that occur during the execution of a Flask application. It is an essential part of application development and helps in debugging, error tracking, and performance monitoring. Flask logging can be configured to write logs to files, system logs, or third-party services.
6.2 Setting up Flask Logging
To set up Flask logging, you need to import the Python logging module and initialize a logger instance in your Flask application. You can then configure the logger to write logs to various targets such as console, files, or third-party services like Elasticsearch or Loggly.
Here's an example of how to set up Flask logging
import logging
from flask import Flask
app = Flask(__name__)
# Set up logger
app.logger.setLevel(logging.INFO)
handler = logging.StreamHandler()
handler.setLevel(logging.INFO)
formatter = logging.Formatter("%(asctime)s - %(name)s - %(levelname)s - %(message)s")
handler.setFormatter(formatter)
app.logger.addHandler(handler)
# Log some messages
app.logger.info("Info message")
app.logger.warning("Warning message")
app.logger.error("Error message")
In the example above, we initialize a logger instance using app.logger
and set its log level to INFO
. We then create a StreamHandler
instance and set its log level to INFO. We also set a log message format using formatter. Finally, we add the StreamHandler instance to the logger instance using app.logger.addHandler(handler)
.
This logger will log messages to the console because we're using StreamHandler()
. However, you can also configure the logger to write logs to files or third-party services.
6.3 Verify logging setup
To verify the correct logging setup, you can add some log messages to your Flask application and check if they are being written to the configured target. Here's an example of how to log a message in a Flask view function
@app.route("/")
def hello():
app.logger.info("Hello, World!")
return "Hello, World!"
You can then start your Flask application and check the console or log file to see if the log message was written successfully.
$ export FLASK_APP=app.py
$ export FLASK_ENV=development
$ flask run
* Running on http://127.0.0.1:5000/
Once you visit http://127.0.0.1:5000/, you should see the log message in the console or log file.
7. Blueprints Setup
7.1 Explanation of Flask Blueprints
A Flask Blueprint is a way to organize related views and other code into a module that can be registered with an application. It allows you to create a modular application that can be extended easily.
7.2 Setting up Blueprints for modular application design
Here is an example of setting up a blueprint in a Flask application
from flask import Blueprint, render_template
user_blueprints = Blueprint('user', __name__, url_prefix='/')
@user_blueprints.route('/')
def index():
return render_template('index.html')
In this example, we are creating a blueprint named 'main' and defining a view function for the '/' URL.
To register the blueprint with the Flask application, we can do the following
from flask import Flask
app = Flask(__name__)
app.register_blueprint(bp)
7.3 Best practices for organizing and structuring blueprints
- Create a separate directory for each blueprint.
- Put all views, templates, and static files for a blueprint in its directory.
- Name the blueprint after the feature or area of the application it represents.
- Use the url_prefix argument to specify a prefix for all the URLs in the blueprint.
- Use the url_for function with the blueprint's name to generate URLs for its views.
Here is an example of how to organize a Flask application using blueprints:
/app
/main
/templates
index.html
/static
main.css
main.js
__init__.py
views.py
/auth
/templates
login.html
register.html
/static
auth.css
auth.js
__init__.py
views.py
__init__.py
config.py
models.py
In this example, we have two blueprints
, main and auth. Each blueprint has its own directory with templates and static files. The views and other code for each blueprint are in separate modules named views.py
and __init__.py.
The main application code is in __init__.py
, and the configuration and models are in separate files.
8. View - Template - Form
8.1 Explanation of Flask's view-template-form architecture
Flask uses the view-template-form architecture, which is a design pattern commonly used in web applications. In this pattern, views handle user requests, retrieve and manipulate data as needed, and pass it on to templates for rendering. Forms handle user input and validation, and ensure that data is correctly formatted and processed.
8.2 Sample code for setting up views, templates, and forms
Here's some sample code for setting up views, templates, and forms in Flask
Views
from flask import Flask, render_template, request
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
@app.route('/form', methods=['GET', 'POST'])
def form():
if request.method == 'POST':
name = request.form['name']
email = request.form['email']
return f'Thank you, {name}! Your email address is {email}.'
else:
return render_template('form.html')
Forms
from flask_wtf import FlaskForm
from wtforms import StringField, SubmitField
from wtforms.validators import DataRequired, Email
class ContactForm(FlaskForm):
name = StringField('Name', validators=[DataRequired()])
email = StringField('Email', validators=[DataRequired(), Email()])
submit = SubmitField('Submit')
In this example, we have a simple Flask app that has two views, one for the index page and one for the form page. The form view accepts both GET and POST requests, and uses a template to render the form itself. When the form is submitted, the data is retrieved from the request object and a response is returned with the user's name and email address.
The templates for the views are written in HTML and use Jinja2 syntax to inject dynamic content into the page. The forms are defined using the Flask-WTF extension and WTForms library, which provides a simple and flexible way to create and validate web forms.
8.3 Best practices for view-template-form architecture
- Keep the views simple and focused on handling requests and responses.
- Use separate template files for each view or group of related views.
- Use template inheritance to reduce duplication and enforce consistency across pages.
- Use forms for all user input and validation to ensure data integrity and security.
- Use Flask's built-in support for CSRF protection to prevent cross-site request forgery attacks.
- Use a consistent naming convention for views, templates, and forms to improve readability and maintainability.
- Use Flask's support for custom error handling to handle common error scenarios gracefully and provide a better user experience.
8.4 Example code for views, templates, and forms
Example view
from flask import render_template, request
from . import app
from .forms import ContactForm
@app.route('/contact', methods=['GET', 'POST'])
def contact():
form = ContactForm()
if request.method == 'POST' and form.validate():
# handle form submission
return 'Thank you for contacting us!'
return render_template('contact.html', form=form)
Example template (contact.html)
{% extends "base.html" %}
{% block content %}
Contact Us
{% endblock %}
Example form
from flask_wtf import FlaskForm
from wtforms import StringField, TextAreaField
from wtforms.validators import DataRequired, Email
class ContactForm(FlaskForm):
name = StringField('Name', validators=[DataRequired()])
email = StringField('Email', validators=[DataRequired(), Email()])
message = TextAreaField('Message', validators=[DataRequired()])
This is just an example, and the actual implementation may vary depending on the specific requirements of the project.
8.5 Most common mistakes with Flask View Template Form
- Not properly handling form validation errors: When using Flask-WTF to handle forms, it's important to properly handle form validation errors to provide feedback to users if there are any issues with their input.
- Not properly using Flask's templating engine: Flask uses Jinja2 as its templating engine, which allows for easy and flexible rendering of dynamic content. However, it's important to properly use Jinja2 syntax to avoid errors.
- Not properly structuring code: As with any code, it's important to properly structure and organize Flask View-Template-Form code for readability and maintainability. This includes properly separating concerns and avoiding mixing business logic with presentation logic.
- Not properly using Flask's built-in view functions: Flask provides built-in view functions for handling common HTTP methods like GET and POST. It's important to properly use these functions to avoid errors and to ensure that the application behaves correctly.
- Not properly handling CSRF protection: When using Flask-WTF to handle forms, it's important to properly handle Cross-Site Request Forgery (CSRF) protection to prevent malicious attacks. This involves generating and validating CSRF tokens for each form submission.
9. REST API Setup
9.1 Explanation of REST APIs
A REST (Representational State Transfer) API is a web-based architectural style that allows for data to be exchanged between clients and servers. It operates by using HTTP verbs (GET, POST, PUT, DELETE) to indicate the type of action being performed on the resource. REST APIs use the stateless nature of HTTP to provide a simple, consistent, and scalable way to access and manipulate resources.
9.2 Setting up a Flask app to serve REST APIs
Flask can be used to set up a RESTful API by defining endpoints that handle HTTP requests and responses. These endpoints are implemented as routes in a Flask app and can return data in a variety of formats such as JSON, XML, or HTML.
9.3 Best practices for designing RESTful APIs
Some best practices for designing RESTful APIs include using HTTP verbs appropriately, following a consistent URL structure, providing error messages in a clear and consistent way, and properly handling authentication and authorization.
9.4 Useful packages: Flask Restful, Flask Marshmallow
Flask Restful is an extension for Flask that provides a lightweight framework for building RESTful APIs. It simplifies the process of creating endpoints by allowing developers to define resources and methods that map to HTTP verbs. Flask Marshmallow is another extension that provides a simple and lightweight way to serialize and deserialize data to and from JSON. It can be used in conjunction with Flask Restful to make it easier to parse and validate incoming requests and format outgoing responses.
9.5 Examples
1. Simple example
from flask import Flask
from flask_restful import Resource, Api
app = Flask(__name__)
api = Api(app)
class HelloWorld(Resource):
def get(self):
return {'hello': 'world'}
api.add_resource(HelloWorld, '/')
if __name__ == '__main__':
app.run(debug=True)
In this example, we define a Flask app and create an instance of the Flask-RESTful API. Then, we define a class HelloWorld that inherits from the Resource class provided by Flask-RESTful. This class defines a single method, get(), which returns a dictionary containing the message "hello: world".
We then add an instance of the HelloWorld resource to our API, mapping it to the root URL '/'. Finally, we start the Flask app with debugging enabled.
With this code in place, we can start our Flask app and visit http://localhost:5000 in a web browser. We should see the message {'hello': 'world'}
displayed on the page. This is the response returned by our HelloWorld resource when we make a GET request to the root URL of our API.
2. Complex example
Sure, here's an example of setting up a Flask app to serve a RESTful API with resource and detail/list endpoints
from flask import Flask, request, jsonify
from flask_sqlalchemy import SQLAlchemy
from flask_marshmallow import Marshmallow
from flask_restful import Api, Resource, abort
# Initialize Flask app and database connection
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///db.sqlite'
db = SQLAlchemy(app)
ma = Marshmallow(app)
api = Api(app)
# Define database model
class Book(db.Model):
id = db.Column(db.Integer, primary_key=True)
title = db.Column(db.String(100))
author = db.Column(db.String(100))
def __repr__(self):
r
eturn f'book {self.id}'
# Define serialization schema
class BookSchema(ma.Schema):
class Meta:
fields = ('id', 'title', 'author')
book_schema = BookSchema()
books_schema = BookSchema(many=True)
# Define resource endpoint for individual books
class BookResource(Resource):
def get(self, book_id):
book = Book.query.get_or_404(book_id)
return book_schema.dump(book)
def put(self, book_id):
book = Book.query.get_or_404(book_id)
data = request.json
book.title = data['title']
book.author = data['author']
db.session.commit()
return book_schema.dump(book)
def delete(self, book_id):
book = Book.query.get_or_404(book_id)
db.session.delete(book)
db.session.commit()
return '', 204
# Define list endpoint for all books
class BookList(Resource):
def get(self):
books = Book.query.all()
return books_schema.dump(books)
def post(self):
data = request.json
book = Book(title=data['title'], author=data['author'])
db.session.add(book)
db.session.commit()
return book_schema.dump(book), 201
# Register resources with API
api.add_resource(BookResource, '/books/<int:book_id>')
api.add_resource(BookList, '/books')</int:book_id>
# Run Flask app
if __name__ == '__main__':
app.run(debug=True)
This example defines a Book model with title and author attributes, along with corresponding serialization schema using Marshmallow. It then defines a BookResource class for handling individual book resources, as well as a BookList class for handling a list of all books. These are registered as endpoints with the Api object, and the app is run in debug mode.
With this setup, a client can make requests to the /books
endpoint to retrieve a list of all books or create a new book, and to the /books/
<book_id> endpoint to retrieve, update, or delete a specific book resource.</book_id>
10. Background tasks Setup
10.1 Why we need background tasks, cron jobs
- To run long-running tasks that can't be done as part of a web request/response cycle.
- To schedule tasks at specific times or intervals, such as sending a reminder email every day at a specific time.
- To offload work from the main application to prevent it from slowing down or becoming unresponsive.
- To perform tasks asynchronously, allowing the main application to continue processing other requests.
- To reduce the latency of user requests by processing non-essential tasks in the background.
10.2 Explanation of Celery and Redis
- Celery is a distributed task queue that allows you to run asynchronous tasks in your application.
- Redis is an open-source in-memory data structure store that is often used as a message broker or database for Celery.
10.3 Setting up Celery + Redis with Flask
To set up Celery + Redis with Flask, you will need to install the following packages
- Flask
- Celery
- Redis
Here is an example code snippet to set up Celery + Redis with Flask:
from celery import Celery, shared_task
from flask import Flask
app = Flask(__name__)
app.config['CELERY_BROKER_URL'] = 'redis://localhost:6379/0'
app.config['CELERY_RESULT_BACKEND'] = 'redis://localhost:6379/0'
celery = Celery(app.name, broker=app.config['CELERY_BROKER_URL'])
celery.conf.update(app.config)
@shared_task
def my_background_task(arg1, arg2):
# do some work here
pass
10.4 Most common mistakes with Celery + Redis
- Failing to set up the Celery broker and result backend correctly.
- Not properly configuring the Celery worker or beat processes.
- Not handling errors or exceptions in the background tasks.
- Overloading the worker with too many tasks.
- Failing to monitor the worker and beat processes for errors or crashes.
10.5 Redis can be used for cache too, show code snippet for Redis cache configuration using JSON file
Here is an example code snippet for configuring Redis cache using a JSON file
import json
from redis import Redis
with open('config.json', 'r') as f:
config = json.load(f)
redis = Redis(host=config['redis']['host'], port=config['redis']['port'], db=config['redis']['db'])
@app.route('/')
@cache.cached(timeout=60)
def index():
# do some work here
pass
In this example, the Redis cache is configured using a JSON file named config.json
. The Redis connection parameters are read from the file and used to create a Redis object. The cache.cached()
decorator is used to cache the index()
view for 60 seconds.
11. Unit Test Setup with Pytest
11.1 Explanation of unit test with web application, pytest overview
Unit testing is the process of testing individual components or units of code in isolation to ensure that they function correctly. In the context of a web application, unit tests are used to test the various components of the application, such as views, models, and forms, to ensure that they are working as expected.
Pytest is a testing framework for Python that makes writing tests easy and straightforward. It provides a simple and intuitive syntax for writing tests, and it can automatically discover and run tests in your application.
11.2 Setting up Pytest for a Flask application
To set up Pytest for a Flask application, you need to install the pytest package:
Once installed, you can run tests by creating test files with names that start with test_, and then running the pytest command in the terminal from the root directory of your Flask application.
11.3 Writing unit tests with pytest
To write unit tests for a Flask application, you can use Pytest's built-in testing functions, such as assert. You can also use the pytest-flask extension, which provides additional functionality for testing Flask applications.
Here's an example of a simple unit test using Pytest:
def test_hello():
assert 'hello' == 'hello'
This test checks that the string 'hello' is equal to the string 'hello'. If the test fails, Pytest will raise an error.
11.4 Some pytest examples for a Flask application
API tests: Testing the API endpoints using pytest-flask
def test_get_tasks(client):
response = client.get('/tasks')
assert response.status_code == 200
Form tests: Testing Flask-WTF forms
from myapp.forms import LoginForm
def test_valid_login_form(client):
form = LoginForm(username='user', password='password')
assert form.validate() is True
def test_invalid_login_form(client):
form = LoginForm(username='', password='')
assert form.validate() is False
Function tests: Testing individual functions within the Flask app
from myapp import app
def test_addition():
assert app.add_numbers(1, 2) == 3
def test_subtraction():
assert app.subtract_numbers(5, 2) == 3
Note that these examples are not exhaustive and are meant to serve as a starting point. The specific tests you need to write will depend on the requirements of your application.
Overall, Pytest is a powerful testing framework that makes it easy to write unit tests for Flask applications. With Pytest, you can ensure that your application is functioning correctly and catch bugs before they make it into production.
12. Conclusion
In conclusion, setting up a Flask application requires careful planning and implementation of best practices. Some key takeaways from this guide include:
- Use a virtual environment to manage dependencies
- Structure your code into modular components using blueprints
- Follow the View-Template-Form architecture for building user interfaces
- Set up logging to effectively monitor your application
- Use caching and background tasks to improve performance
- Test your code using Pytest to ensure functionality and prevent regressions
By following these best practices, you can create a robust and scalable Flask application that is easy to maintain and extend.
As with any technology, Flask and its associated tools and packages are constantly evolving. It's important to stay up-to-date with new developments and best practices in order to keep your application secure and performant. Consider joining the Flask community, attending meetups or conferences, or reading relevant books and blog posts to stay informed.
Finally, I hope this guide has been helpful in providing a solid foundation for building Flask applications. Remember to always write clean, readable, and maintainable code, and to prioritize user experience and security in all aspects of your application development.
13. References
- Flask framework https://flask.palletsprojects.com/en/2.3.x/installation/
- Flask Form https://flask-wtf.readthedocs.io/en/1.0.x/
- Flask Marshmallow https://flask-marshmallow.readthedocs.io/en/latest/
- Flask RESTFUL https://flask-restful.readthedocs.io/en/latest/
- Flask test https://pytest-flask.readthedocs.io/en/latest/
- Flask Celery https://flask.palletsprojects.com/en/2.3.x/patterns/celery/
- Flask migrate https://flask-migrate.readthedocs.io/en/latest/
- Python dotenv https://github.com/theskumar/python-dotenv
- Flask SQLAlchemy https://flask-sqlalchemy.palletsprojects.com/en/3.0.x/
- Flask Migrate https://flask-migrate.readthedocs.io/en/latest/