Common Python Problems - [1] None and Boolean Comparison
By JoeVu, at: Jan. 10, 2023, 2:09 p.m.
Estimated Reading Time: __READING_TIME__ minutes
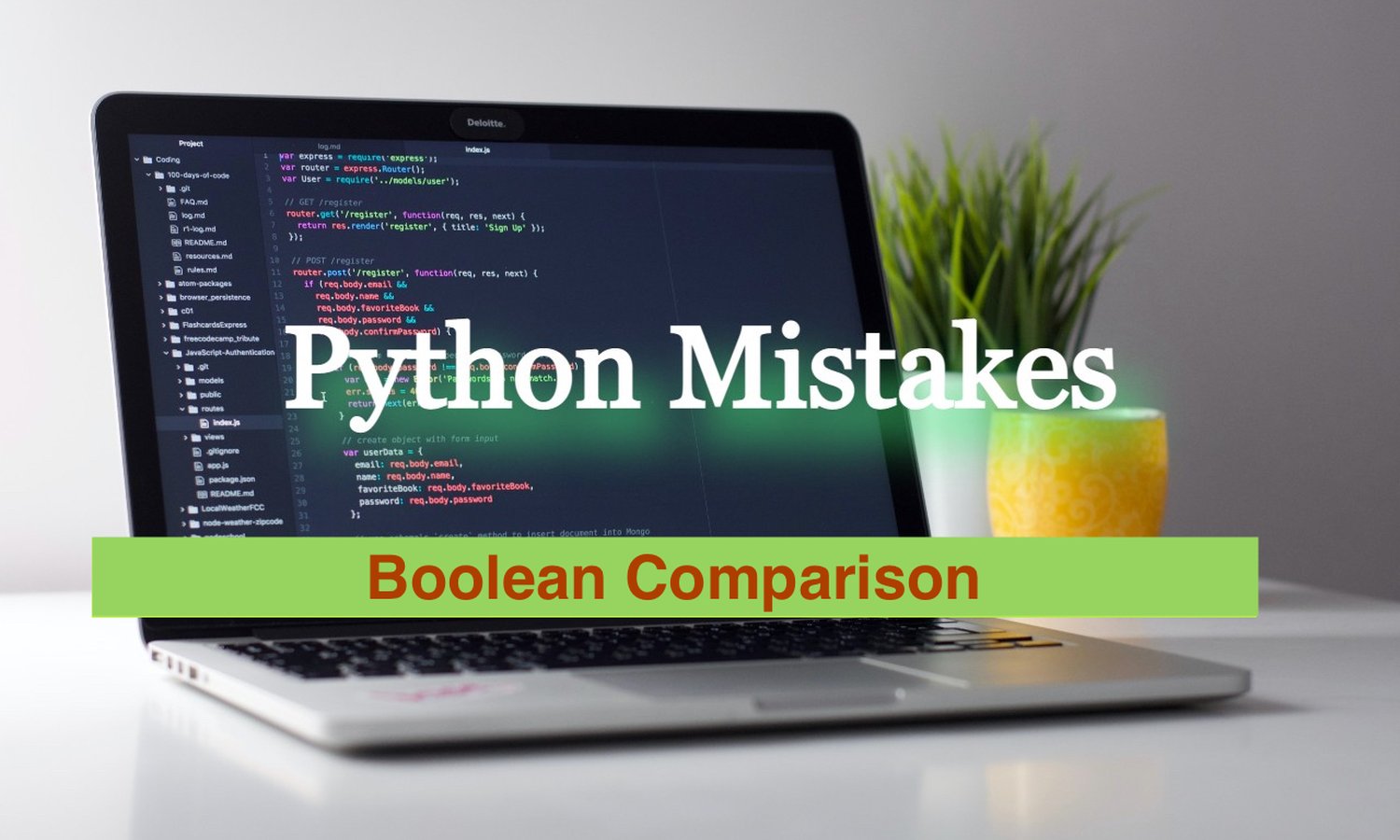
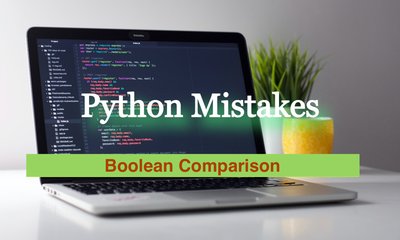
The Boolean Comparison Confusion in Python is a common issue for those learning to program in Python. It is caused by differences in the way Python evaluates comparison operators and the values they return. This can lead to unexpected results and confusion when comparing values, such as when using the == operator. This guide will provide an overview of the issue and explain how to avoid it.
1. The Boolean Confusion
Look at this code snippet, and think abour your guess result for each statement. How many correct answers do you get?
'' == False
False
In [2]: if '':
...: print('an empty string is true')
...: else:
...: print('an empty string is false')
...:
an empty string is false
bool('') == False
True
0 == False
True
0.0 == False
True
1 == True
True
[] == False
False
{} == False
False
set() == False
False
bool(None) == False
True
None == False
False
None == True
False
a = None
a == None
True
a is None
True
As can be seen from above, it is safe to check if a variable is NOT an empty string, an empty list/dict/set, a None value by using
if not variable: # equivalent to: if bool(variable) is not False
print(variable)
While None
isn't True, and isn't False. None is a special value.
Let look closely in another issue
result = None
for i in range(50):
if i % 99 == 0:
result = i
break
if result:
print(result)
print(result)
Output
0
What is the problem here?
It is the problem with boolean comparison in if result.
As we initialized the result as None from the beginning, we expect the result must be not None if we have found the number divisible by 99. The answer is 0.
So the correct solution is
result = None
for i in range(50):
if i % 99 == 0:
result = i
break
if result is not None:
print(result)
Output
0