Comparing Flask, Flask-RESTful, and Flask-RESTPlus: A Comprehensive Guide
By hientd, at: Nov. 12, 2023, 6:23 p.m.
Estimated Reading Time: __READING_TIME__ minutes

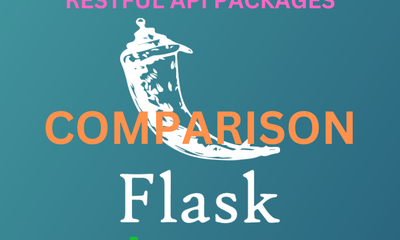
Comparing Flask, Flask-RESTful, and Flask-RESTPlus: A Comprehensive Guide
In the realm of web development, building robust and efficient APIs is a common task. Flask, a micro web framework for Python, provides a solid foundation for API development. However, when it comes to building RESTFUL APIs, developers have multiple options. In this article, we will delve into a comprehensive comparison of three popular Flask-based packages for building RESTFUL APIs:
- Flask
- Flask-RESTful - you can find one of our article about this integration here
- Flask-RESTPlus
Why Choose Flask for API Development
Before we dive into the comparison, it's essential to recognize why Flask is a favored choice for API development. Flask is renowned for its flexibility and ease of use. With a small core and a philosophy that emphasizes simplicity and extensibility, Flask empowers developers to build APIs quickly and efficiently.
Package Overviews
Flask
Flask REST is a minimalistic package that extends Flask's capabilities for building RESTful APIs. It provides the essential tools for routing and request handling, making it an excellent choice for developers who prefer a lightweight, hands-on approach to API development.
Flask-RESTful
Flask-RESTful, on the other hand, is a full-fledged extension of Flask designed to facilitate the creation of clean, object-oriented code for rapid API development. It offers abstractions to work with Object Relational Mapping (ORM) systems and supports various useful libraries, allowing developers to build highly reusable and maintainable APIs.
Flask-RESTPlus
Flask-RESTPlus is another powerful extension for Flask, which takes API development to the next level. Like Flask-RESTful, it encourages best practices with minimal setup. However, Flask-RESTPlus goes a step further by providing a cohesive collection of decorators and tools to describe your API. Notably, Flask-RESTPlus offers comprehensive Swagger documentation, a feature that can greatly benefit API developers.
Code Comparison
Let's take a closer look at how these packages handle the minimal code required for creating a simple Flask app with GET and POST endpoints.
Flask
# Minimal code for GET and POST requests
from flask import Flask
app = Flask(__name)
@app.route('/tasks', methods=['GET', 'POST'])
def tasks():
if request.method == 'GET':
# Handle GET request
elif request.method == 'POST':
# Handle POST request
Flask-RESTful
# Minimal code for GET and POST requests
from flask import Flask
from flask_restful import Api, Resource
app = Flask(__name)
api = Api(app)
class TaskResource(Resource):
def get(self):
# Handle GET request
def post(self):
# Handle POST request
api.add_resource(TaskResource, '/tasks')
Flask-RESTPlus
# Minimal code for GET and POST requests
from flask import Flask
from flask_restx import Api, Resource
app = Flask(__name)
api = Api(app)
@api.route('/tasks')
class TaskResource(Resource):
def get(self):
# Handle GET request
def post(self):
# Handle POST request
Ease of Use
- Flask is the most minimalistic and hands-on package. It provides flexibility but requires more manual setup for common API features.
- Flask-RESTful and Flask-RESTPlus, on the other hand, offer more structured and opinionated solutions, which can streamline the development process.
- Flask-RESTPlus, with its enhanced documentation support, is often considered the most developer-friendly option.
Flexibility and Extensibility
All three packages are extensible, allowing developers to customize their APIs to suit specific needs.
Flask-RESTful and Flask-RESTPlus offer abstractions for common API tasks, such as routing, parameter handling, and error management. This can lead to more organized and maintainable code. However, Flask provides the highest level of flexibility, enabling developers to design their API architecture from the ground up.
Documentation and Testing
Flask-RESTPlus stands out in terms of documentation, with built-in support for Swagger. This feature makes it incredibly easy to generate and browse API documentation, simplifying testing and understanding of the API's capabilities.
Flask-RESTful also supports documentation but requires additional configuration. - ex: https://flask-restful-swagger.readthedocs.io/en/latest/
Flask provides the least built-in documentation support.
Community and Ecosystem
Flask-RESTful and Flask-RESTPlus have established and active communities. A larger community often results in more resources, plugins, and support. Flask, being more minimal, may have a smaller community but can still be a suitable choice for projects with specific requirements.
Performance and Scaling
All three packages are designed for building scalable and production-ready APIs. Performance differences are typically negligible and depend more on the overall design of the API and application architecture.
Recommendations
Choosing the right package depends on your specific project requirements and your preference for code structure:
- Use Flask if you prefer minimalism and need complete control over your API structure. It's an excellent choice for small, custom projects.
- Opt for Flask-RESTful if you want a structured, object-oriented approach and need abstractions for common API tasks. It's ideal for projects that require a balance between flexibility and structure.
- Choose Flask-RESTPlus if you want the best of both worlds. It offers structure and abstractions while providing comprehensive Swagger documentation. This package is particularly suitable for larger projects with a focus on API documentation and testing.
- Additional resources:
- Pydantic: This is well-known for the data validation
- Swagger: This is for API documentation
- MarshMallow: This is great for data serialization
- Pydantic: This is well-known for the data validation
Conclusion
In the Flask-based API development world, the choice between Flask, Flask-RESTful, and Flask-RESTPlus depends on your development style and project requirements. Each package has its strengths and weaknesses, so it's essential to consider your specific needs when making a decision.