Comprehensive Look at Vue.js: Benefits, Challenges, and Common Issues
By khoanc, at: June 24, 2024, 10:37 a.m.

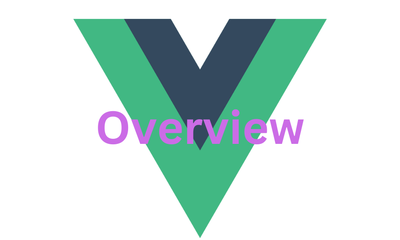
Comprehensive Look at Vue.js: Benefits, Challenges, and Common Issues
Introduction
Vue.js is a progressive JavaScript framework that has gained popularity for its simplicity and flexibility. However, like any technology, it comes with its own set of benefits and challenges. This article combines insights from various sources to provide a holistic view of Vue.js.
Benefits of Vue.js
-
Adaptability: Vue.js framework is designed to be incrementally adoptable, meaning developers can use as much or as little of the framework as needed. This makes transitioning from other frameworks relatively straightforward.
-
Enhanced HTML Capabilities: Vue.js extends standard HTML with directives and attributes that offer enhanced functionality, making it easier to develop dynamic applications.
-
Ease of Integration: Vue.js can be easily integrated into existing projects, whether it’s a single component or a full-fledged application, which is particularly beneficial for incremental migration.
-
Scalability: The simple and consistent structure of Vue.js makes it easy to scale applications, whether you're adding new features or expanding the project size.
-
Excellent Documentation: Vue.js is known for its thorough and well-organized documentation, which provides clear guidance and support for developers at all levels.
-
Lightweight Nature: Vue.js is a lightweight framework, with a size of around 20kB. This ensures fast load times and a smooth user experience.
Challenges of Vue.js
-
Smaller Market Share and Community: Compared to React and Angular, Vue.js has a smaller community and market presence. This can result in fewer resources and third-party libraries.
-
Integration in Large Projects: While Vue.js excels in smaller applications, integrating it into larger projects can be more challenging, particularly when dealing with legacy systems.
-
Incomplete English Documentation: Some areas of Vue.js documentation may lack depth, particularly in translations, which can be a barrier for non-native speakers.
Common Issues and Solutions in Vue.js
Data Mutation: Vue.js often mutates data, which can make debugging and testing more difficult. To mitigate this, developers should adopt immutable state management practices.
const state = Object.freeze({
user: {
name: 'John Doe',
age: 30
}
});
// Attempting to modify state will now throw an error
state.user.age = 31; // Error: Cannot assign to read only property 'age'
=> Solution: Use immutable state management. For instance, use Object.freeze()
to create immutable objects.
Custom Syntax: Vue.js introduces custom syntax (e.g., directives like v-if
and v-for
), which can increase the learning curve. Leveraging the framework’s extensive documentation can help bridge this gap.
< ul >
< li v-for="item in items" :key="item.id">{{ item.name }}
< /ul >
< script >
new Vue({
el: '#app',
data: {
items: [
{ id: 1, name: 'Item 1' },
{ id: 2, name: 'Item 2' }
]
}
});
=> Solution: Familiarize yourself with Vue.js directives through the documentation. Here's an example using v-for
:
Poor IDE Support: Although improving, IDE support for Vue.js, especially with TypeScript, has lagged behind. Utilizing IDEs and plugins that are specifically designed for Vue.js can enhance the development experience.
=> Solution: Visual Studio Code with Vetur extension.
Implicit Behavior: Many Vue.js features operate implicitly, which can lead to unexpected behaviors. Understanding these implicit behaviors through comprehensive testing and documentation review is crucial.
Vue.component('child', {
props: ['initialCounter'],
data() {
return { counter: this.initialCounter };
},
watch: {
initialCounter(newVal) {
this.counter = newVal;
}
}
});
=> Solution: Understand and control these behaviors. For example, watch for prop changes explicitly:
Function Overloading: Vue.js’s approach to function overloading can add complexity. Clear documentation and consistent coding practices can help manage this complexity.
< input v-model="message" placeholder="edit me" >
< input v-model.number="age" placeholder="enter age" type="number" >
< script >
new Vue({
el: '#app',
data: {
message: '',
age: null
}
});
=> Solution: Use clear and consistent coding practices. Here’s an example of v-model
handling different input types:
Performance Pitfalls: Tracking down performance issues in Vue.js applications often involves identifying and optimizing slow components. Tools like Vue Devtools can be invaluable for performance debugging.
// Lazy load component
const LazyComponent = () => import('./components/LazyComponent.vue');
new Vue({
el: '#app',
components: {
LazyComponent
}
});
=> Solution: Use Vue Devtools to profile and optimize components. Here's a basic example of lazy loading a component:
Conclusion
Vue.js is a powerful framework that offers significant benefits for web development, including adaptability, ease of integration, and excellent documentation. However, developers should be aware of its challenges, such as a smaller community, integration difficulties in large projects, and certain performance pitfalls. By understanding these issues and leveraging the extensive resources available, developers can effectively utilize Vue.js to build dynamic and scalable applications.
For further details, you can refer to the original articles: