Django Application Cleanup
By khoanc, at: July 17, 2023, 11:26 a.m.
Estimated Reading Time: __READING_TIME__ minutes

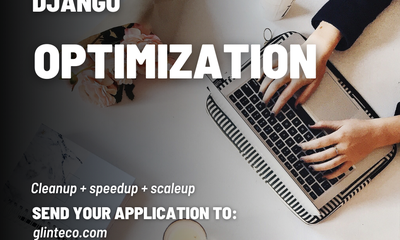
How to Clean Up a Django Application for Peak Performance
Introduction
Are you struggling with a sluggish Django application?
Is your codebase becoming increasingly cluttered and difficult to maintain?
It might be time for a cleanup! A well-organized and optimized Django application not only enhances performance but also simplifies future development. In this article, we'll delve into the process of cleaning up a Django application to achieve peak performance. We'll cover essential points, tackle edge cases, provide handy tips and tricks, share best practices, and even illustrate with code snippet examples.
Table of Contents
- Why Clean Up Your Django Application?
- Assessing Your Application
- Identifying Bottlenecks
- Analyzing Code Complexity
- Writing performance tests
- Prioritizing Tasks for Cleanup
- Unused Code and Dependencies
- Duplicated Code and Logic
- Inefficient Database Queries
- Code Refactoring and Optimization
- Streamlining Views and Models
- Utilizing Django's Built-in Features
- Database Cleanup
- Removing Redundant Data
- Indexing for Performance
- Managing Static and Media Files
- Deleting Unused Files
- Optimizing File Storage
- Automating Cleanup Processes
- Continuous Integration
- Task Runners and Scripts
- Best Practices for Sustaining Cleanliness
- Regular Code Reviews
- Documentation Maintenance
- Edge Cases and Complexities
- Handling Legacy Code
- Data Migration Strategies
- Tips and Tricks for Efficient Cleanup
- Version Control and Branching
- Logging and Monitoring
- Conclusion
- FAQs
1. Why Clean Up Your Django Application?
There is an undenying fact that the codebase is getting worse overtime due to the human resource change, a poor management and a tight budget. This results in a high maintenance cost and bad application performance.
Maintaining a clean codebase is crucial for long-term success. Accumulated technical debt and cluttered code can slow down development, hinder debugging, and compromise performance. Cleaning up your Django application provides several benefits:
-
Improved Performance: Unnecessary code and redundant data can impact your application's speed. By removing them, you can significantly enhance response times. Some pieces of code are smell and buggy, this would slowdown the application speed.
-
Enhanced Maintainability: A tidy codebase is easier to understand and work with. Developers can quickly find what they need and make changes without fear of unintended consequences.
-
Reduced Bugs and Errors: Duplicated logic and unnecessary dependencies increase the likelihood of bugs. Cleaning up minimizes these risks and improves the overall reliability of your application.
2. Assessing Your Application
Identifying Bottlenecks
To begin the cleanup process, identify performance bottlenecks using tools like Django Debug Toolbar or profiling tools. Pinpoint slow views, database queries, and resource-intensive operations.
Another option would be purchasing some application monitoring services (Sentry/NewRelic) that would help us to acknowledge the application weakness with ease.
Analyzing Code Complexity
Evaluate the complexity of your code using tools such as Radon. Identifying overly complex functions or classes helps you focus your cleanup efforts where they are most needed.
Django-Query-Inspect is also an useful tool that logs all database queries.
Writing performance tests
We never know the application performance if we don't use the application daily. Writing performance tests would be the best option for all developers (who do not play with application like real users) to acknowledge the code quality before publishing.
3. Prioritizing Tasks for Cleanup
Unused Code and Dependencies
Scan your codebase for unused code, templates, and dependencies. Removing them not only reduces bloat but also eliminates potential security vulnerabilities. This is not an easy task which can only be done by senior developers who have been working on many projects with a lot of experience.
One useful package would be Vulture
Duplicated Code and Logic
Search for duplicated code and consolidate it into reusable functions or classes. This enhances code clarity and simplifies future updates. Pylint definitely is a good helper here.
Inefficient Database Queries
Optimize database queries using Django's query optimization techniques. Use the select_related
and prefetch_related
methods to minimize the number of queries executed.
As mentioned earlier, Django-Query-Inspect list all queries with their execution time, this is exactly what we should look for when perform database profiling.
4. Code Refactoring and Optimization
Streamlining Views and Models
Review your views and models to ensure they follow the Single Responsibility Principle. Split large views into smaller ones and refactor models for clarity and efficiency.
The best practice is to have one class in one file, View must be THIN and Model should be FAT.
Utilizing Django's Built-in Features
Leverage Django's built-in features like caching, middleware, and signals. These tools can significantly enhance performance with minimal effort.
This is a mistake when developers do not care much about those tools when it comes to optimization. Those are the core features of the Django application, some can help us to speed up a lot or secure the application (ex: Django-ratelimit)
5. Database Cleanup
Removing Redundant Data
Identify and remove unnecessary data from your database. This could include expired sessions, unused user accounts, or orphaned records.
Many database tables, views, fields are redundant, not used for a while when the project is getting bigger. That is the result of moving fast and breaking things later, this is a trade-off and easy to deal with.
Indexing for Performance
Optimize your database by adding appropriate indexes to frequently queried fields. This speeds up data retrieval and improves overall response times.
Thanks to the help of Django-query-inspect and Analyze statement, developers are able to see how indexes work on all queries and how to optimize them using indexing mechanism. Which can be done by Django-model-index
6. Managing Static and Media Files
Deleting Unused Files
Clean up static and media directories by removing unused files and assets. This reduces storage requirements and speeds up file handling.
One possible solution would be using the package Django-distill and Cloudflare
Optimizing File Storage
Consider using Content Delivery Networks (CDNs) to offload file serving. CDNs distribute files to geographically distributed servers, reducing server load.
7. Automating Cleanup Processes
Continuous Integration
Integrate code quality checks and automated tests into your development workflow. This prevents new clutter from entering the codebase and enforces cleanup best practices.
A full combo for cleaning up, re-styling and security checking is Pre-commit + Fourmat
Task Runners and Scripts
Create scripts or use task runners like Celery to automate routine cleanup tasks, such as removing temporary files or old database records.
8. Best Practices for Sustaining Cleanliness
Regular Code Reviews
Implement regular code reviews to catch clutter and deviations from coding standards early. Peer feedback helps maintain a clean codebase.
Documentation Maintenance
Keep your documentation up to date. Documenting your codebase helps new developers understand the architecture and make informed contributions.
9. Edge Cases and Complexities
Handling Legacy Code
Dealing with legacy code requires careful planning. Gradually refactor and clean up legacy sections while maintaining functionality.
Data Migration Strategies
When cleaning up data, devise comprehensive migration strategies. Back up data before making any changes and ensure a smooth transition.
10. Tips and Tricks for Efficient Cleanup
Version Control and Branching
Use version control to experiment with cleanup changes without affecting the main codebase. Create feature branches for cleanup tasks.
Logging and Monitoring
Implement thorough logging to track changes and errors during cleanup. Monitor your application's performance post-cleanup to ensure improvements.
Django has a powerful logging system which can be easily integrated with many 3rd party services including Loggly
Conclusion
A well-maintained Django application is a joy to work with. By investing time in cleaning up your codebase, optimizing queries, and managing resources, you can achieve a high-performing application that's easy to maintain. Remember, cleanup is not a one-time task; it's an ongoing process that pays off in the long run.
FAQs
-
Is cleaning up a Django application time-consuming? Cleaning up may take some time initially, but the long-term benefits in terms of performance and maintainability are well worth it.
-
Can I automate the cleanup process? Absolutely! Automating cleanup tasks through scripts and continuous integration helps ensure consistent cleanliness.
-
What's the best approach to dealing with legacy code during cleanup? Gradual refactoring and strategic changes are key. Prioritize critical areas and ensure the application remains functional throughout the process.