Django Manager Queryset Advanced Topics
By hientd, at: June 28, 2023, 2:52 p.m.
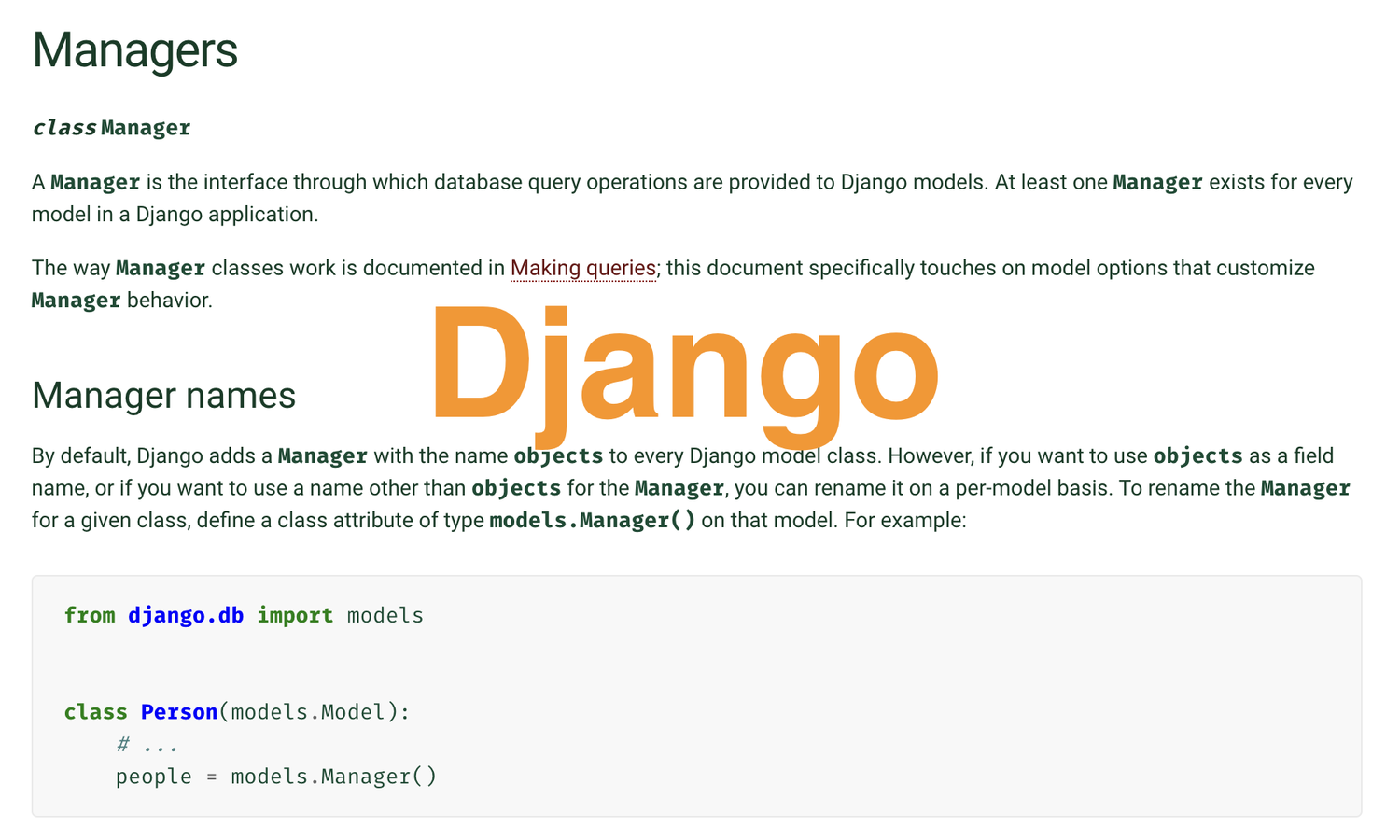
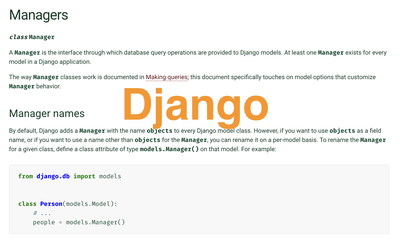
1. Introduction
1.1 Overview of Django Managers and Querysets
When developing web applications using Django, you'll often work with two essential components: Managers and Querysets.
-
Managers: Django Managers act as intermediaries between your models and the database. They provide a high-level interface for managing database operations related to your models, such as creating, updating, deleting, and querying objects. Managers are defined within model classes and enable you to perform common database tasks in a more organized and reusable manner.
-
Querysets: Querysets, on the other hand, represent a collection of objects from the database that match certain criteria. They allow you to retrieve and manipulate data from the database using a chainable API provided by Django's ORM (Object-Relational Mapping) system. Querysets provide a powerful and expressive way to construct database queries without directly writing SQL.
1.2 Importance of Manager Queryset Customization in Django
While Django's built-in Managers and Querysets offer a wide range of functionalities, there may be situations where you need to customize and extend their capabilities to meet specific requirements. Manager Queryset customization allows you to tailor the behavior of Managers and fine-tune the queries performed on the database.
One common scenario is when you encounter complex queries or advanced filtering logic that cannot be achieved using the standard query methods provided by Django. By customizing Managers and Querysets, you gain the flexibility to construct complex queries, apply advanced filtering conditions, and perform more intricate data manipulations.
To illustrate, let's consider an example where you have a model called Product
representing products in an e-commerce application. You want to retrieve all products with a price greater than a certain threshold and sort them by their popularity rating. This requires a customized query that combines filtering and ordering. Here's an example code snippet demonstrating how Manager Queryset customization can be used to achieve this:
class ProductManager(models.Manager):
def get_popular_products_with_price_above(self, threshold):
return self.filter(price__gt=threshold).order_by('-rating')
class Product(models.Model):
name = models.CharField(max_length=100)
price = models.DecimalField(max_digits=8, decimal_places=2)
rating = models.FloatField()
objects = ProductManager()
In the above example, we define a custom Manager called ProductManager
that extends the default Manager for the Product
model. The get_popular_products_with_price_above
method performs a customized query using the filter
and order_by
methods provided by the Queryset API. This allows us to retrieve the desired products based on the given conditions.
By utilizing Manager Queryset customization, you can extend the functionality of Managers and Querysets to cater to your specific data retrieval and manipulation needs in Django applications.
2. When to Use Queryset Customization
2.1 Complex queries and advanced filtering
There are situations where the standard query methods provided by Django may not be sufficient to express complex database queries or advanced filtering conditions. Queryset customization allows you to handle such scenarios effectively.
Example: Suppose you have a model called Article
that represents blog articles in your application. You want to retrieve all articles written by authors from a specific country and published in the last month. Here's an example of how you can customize the queryset to achieve this:
from django.db import models
from django.utils import timezone
class ArticleQuerySet(models.QuerySet):
def from_country(self, country):
return self.filter(author__country=country)
def published_last_month(self):
start_date = timezone.localtime() - timezone.timedelta(days=30)
return self.filter(published_date__gte=start_date)
class Article(models.Model):
title = models.CharField(max_length=100)
author = models.ForeignKey(Author, on_delete=models.CASCADE)
published_date = models.DateField()
objects = ArticleQuerySet.as_manager()
Here, we define a custom QuerySet called ArticleQuerySet
and add two methods from_country
and published_last_month
.
from_country
: filters articles based on the author's countrypublished_last_month
: filters articles published within the last month.
By customizing the QuerySet, we can easily chain these methods to construct the desired query. Ex: Article.objects.from_country('USA').published_last_month()
2.2 Performance optimization and query tuning
Queryset customization can be beneficial for optimizing query performance and fine-tuning database queries to improve efficiency.
Example: Consider a scenario where you have a model called Order
that represents customer orders in an e-commerce application. You want to retrieve all orders with a total amount greater than a certain threshold and order them by the most recent ones. Here's an example of how you can customize the queryset to optimize the query:
from django.db import models
class OrderQuerySet(models.QuerySet):
def filter_by_total_amount(self, threshold):
return self.filter(total_amount__gt=threshold)
def order_by_most_recent(self):
return self.order_by('-created_at')
class Order(models.Model):
total_amount = models.DecimalField(max_digits=8, decimal_places=2)
created_at = models.DateTimeField(auto_now_add=True)
objects = OrderQuerySet.as_manager()
Again, we define a custom QuerySet called OrderQuerySet
and add two methods filter_by_total_amount
and order_by_most_recent
.
filter_by_total_amount
method filters orders based on the total amountorder_by_most_recent
method orders the orders in descending order of their creation timestamps.
Therefore, we can isolate different methods/queries to see which cause the slowndown and optimise one by one at a time
2.3 Data transformation and aggregation
Queryset customization enables you to perform data transformation and aggregation directly within the query, reducing the need for additional post-processing or data manipulation in application code.
Example: Suppose you have a model called Transaction
that represents financial transactions in your application. You want to retrieve the total amount spent by each customer. Here's an example of how you can customize the queryset to perform data aggregation:
from django.db import models
class TransactionQuerySet(models.QuerySet):
def total_amount_by_customer(self):
return self.values('customer').annotate(total_amount=models.Sum('amount'))
class Transaction(models.Model):
customer = models.ForeignKey(Customer, on_delete=models.CASCADE)
amount = models.DecimalField(max_digits=8, decimal_places=2)
objects = TransactionQuerySet.as_manager()
The method total_amount_by_customer
performs data aggregation using the values
and annotate
methods provided by the QuerySet API.
2.4 Integration with external systems or libraries
Queryset customization allows you to seamlessly integrate with external systems or libraries by extending the functionality of Managers and Querysets.
Example: Suppose you're using a search engine integration like Elasticsearch in your Django application. You want to perform a full-text search on a specific model. Here's an example of how you can customize the queryset for integration with Elasticsearch:
from django.db import models
from elasticsearch import Elasticsearch
class SearchQuerySet(models.QuerySet):
def search(self, query):
es = Elasticsearch()
# Perform Elasticsearch search using the query parameter
results = es.search(index='your_index', body={'query': {'match': {'content': query}}})
# Extract relevant document IDs from the search results
document_ids = [hit['_id'] for hit in results['hits']['hits']]
# Return filtered queryset based on the retrieved document IDs
return self.filter(id__in=document_ids)
class Article(models.Model):
title = models.CharField(max_length=100)
content = models.TextField()
objects = SearchQuerySet.as_manager()
By customizing Managers and Querysets in Django, you can extend their functionality to handle complex queries, optimize performance, perform data transformations, and integrate with external systems or libraries, making your application more powerful and flexible
3. Pros of Manager/Queryset Customization
3.1 Flexibility in constructing tailored queries
One of the major advantages of Queryset customization is the flexibility it provides in constructing tailored queries. By customizing Managers and Querysets, you gain fine-grained control over the database queries and can express complex conditions and filtering logic that go beyond the standard query methods offered by Django. This allows you to retrieve precisely the data you need, improving the overall efficiency and accuracy of your application's data retrieval process.
This also means code re-usable and clean which also results in a better management and less maintenance cost.
3.2 Performance optimization and efficiency gains
Queryset customization plays a crucial role in optimizing query performance and achieving efficiency gains. By customizing the Queryset, you can
- optimize the queries to fetch only the necessary data
- reduce the number of database hits
- leverage advanced database features such as indexes and query optimization techniques.
This can lead to significant improvements in the response time and overall performance of your application, especially when dealing with large datasets or complex queries.
There are many application monitoring services, such as Sentry, NewRelic, DataDog. They are all easily integrated with Django.
3.3 Simplified data manipulation and aggregation
Customizing Managers and Querysets simplifies data manipulation and aggregation tasks. You can define custom methods that encapsulate complex data transformations or aggregations directly within the query. This eliminates the need for post-processing the retrieved data in application code and enables you to perform calculations, aggregations, and other data manipulations efficiently within the database itself. It leads to cleaner and more concise code, making it easier to understand and maintain.
3.4 Seamless integration with external systems
Queryset customization allows for seamless integration with external systems or libraries. By extending Managers and Querysets, you can incorporate functionality from third-party libraries or external systems directly into your database queries. This enables you to leverage the features and capabilities of external components while maintaining a unified and consistent interface within your Django application.
4. Cons of Manager/Queryset Customization
4.1 Increased complexity in query construction
One of the challenges of Queryset customization is the increased complexity in query construction. As you customize Managers and Querysets to handle specific requirements, the codebase can become more intricate and harder to understand, especially for developers who are not familiar with the customization. It's crucial to maintain proper documentation and follow best practices to mitigate this complexity and ensure the maintainability of the code.
However, in the long term, it will gain more benefits
4.2 Portability concerns with database-specific features
When customizing Querysets, there's a risk of relying on database-specific features that may not be available or behave differently in other database backends supported by Django (ex: Django PostgreSQL). This can lead to portability concerns, as the customized queries may not work seamlessly when switching between different databases. It's important to carefully consider the portability implications and use Django's abstraction layer effectively to maintain compatibility across multiple database engines if required.
This seems to be rare but worth consideration
4.3 Maintenance challenges and documentation requirements
Queryset customization introduces maintenance challenges and documentation requirements. As the codebase evolves (changes to models, database schemas, or requirements) may necessitate updates to the customized queries. It's essential to have a comprehensive understanding of the customized code and its dependencies to ensure proper maintenance. Additionally, documenting the purpose and usage of customized Managers and Querysets is crucial for the future maintenance and collaboration of the codebase.
In general, if you write simple query functions, then they would be easy to understand and more human friendly readable. This would minimize the difficulty of understanding big functions.
4.4 Learning curve for developers new to query customization
Queryset customization has a learning curve, especially for developers who are new to this aspect of Django development. Customizing Managers and Querysets requires understanding the intricacies of Django's ORM and the Queryset API. Developers need to familiarize themselves with the available methods, query construction techniques, and best practices to leverage the full potential of Queryset customization effectively. Providing adequate training and resources can help developers overcome this learning curve and utilize Queryset customization to its fullest potential.
Despite these challenges, the benefits of Queryset customization, including flexibility, performance optimization, simplified data manipulation, and seamless integration with external systems, make it a valuable tool for developing robust and efficient Django applications
With careful consideration and adherence to best practices, Queryset customization can greatly enhance the power and efficiency of your Django project.
5. Best Practices for Manager/Queryset Customization
5.1 Balancing readability and query complexity
When customizing Querysets, it's important to strike a balance between query complexity and code readability. While it's tempting to include all the logic within a single query method, it can make the code harder to understand and maintain. Instead, consider breaking down complex queries into smaller, reusable methods. This improves code readability and allows for easier comprehension and debugging.
class ArticleQuerySet(models.QuerySet):
def published_in_year(self, year):
return self.filter(published_date__year=year)
def authored_by_user(self, user):
return self.filter(author=user)
def get_featured_articles(self):
return self.filter(is_featured=True)
The ArticleQuerySet
customizes three query methods: published_in_year
, authored_by_user
, and get_featured_articles
. Each method handles a specific filtering condition, making the code more readable and maintainable.
5.2 Effective use of Django's Query API
Django's Query API provides a rich set of methods and operators to construct queries. Familiarize yourself with the available methods and choose the most appropriate ones for your use case. Using the appropriate methods not only improves code readability but also ensures that Django optimizes the resulting query execution.
class ProductQuerySet(models.QuerySet):
def in_stock(self):
return self.filter(quantity__gt=0)
def price_range(self, min_price, max_price):
return self.filter(price__range=(min_price, max_price))
Here, the ProductQuerySet
customizes two query methods: in_stock
and price_range
. By utilizing the filter
method with appropriate operators, such as gt
(greater than) and range
, the code becomes more expressive and aligns with Django's Query API conventions.
5.3 Optimizing queries for performance and efficiency
Efficient query design plays a vital role in the performance of your Django application. When customizing Querysets, consider optimizing queries by reducing database hits, utilizing indexes, and leveraging efficient query techniques like prefetching related data or using select_related and prefetch_related methods.
class OrderQuerySet(models.QuerySet):
def with_items(self):
return self.prefetch_related('items')
def total_amount_gte(self, amount):
return self.filter(total_amount__gte=amount).select_related('customer')
The OrderQuerySet
customizes two query methods: with_items
and total_amount_gte
. The with_items
method utilizes prefetch_related
to optimize fetching related items in bulk, reducing the number of database hits. The total_amount_gte
method combines filtering with select_related
to fetch the associated customer data in a single database query, improving query performance.
5.4 Testing and documentation guidelines
When customizing Querysets, it's essential to write comprehensive tests and maintain proper documentation. Unit tests should cover various scenarios to ensure the customized Querysets function as expected. Additionally, documenting the purpose, usage, and any limitations of the customized Querysets helps other developers understand and utilize them correctly.
class ProductQuerySet(models.QuerySet):
def discount_price(self, percentage):
"""
Returns a queryset of products with a discounted price.
Args:
percentage (float): Discount percentage to apply.
Returns:
QuerySet: A filtered queryset of products with the discounted price.
"""
return self.annotate(discounted_price=F('price') * (1 - percentage / 100))
# Unit test example
def test_discount_price(self):
queryset = Product.objects.all().discount_price(10)
product = queryset.first()
assert product.discounted_price == product.price * 0.9
In the above example, the ProductQuerySet
customizes the discount_price
method and provides detailed documentation about its purpose and behavior. Additionally, an example unit test is included to validate the functionality of the customized method.
By following these best practices, you can ensure that your customized Querysets are maintainable, performant, and well-documented, leading to more efficient development and a robust Django application.
6. FAQs (Frequently Asked Questions)
6.1. What is the difference between a Manager and a Queryset in Django?
In Django, a Manager is responsible for handling database queries and retrieving objects from the database. It provides high-level methods for common query operations. On the other hand, a Queryset represents a collection of objects retrieved from the database based on a query. It allows further filtering, slicing, and manipulation of the retrieved data. Managers provide access to Querysets and allow customization of query behavior.
6.2 Can Queryset customization lead to SQL injection vulnerabilities?
No, Queryset customization in Django is designed to prevent SQL injection vulnerabilities. Django's ORM uses parameterized queries by default, which ensure that user-supplied values are properly escaped and treated as data rather than executable code. As long as you use the provided methods and follow the Django documentation, you can avoid SQL injection vulnerabilities when customizing Querysets.
6.3 How can I ensure portability when using database-specific features in Queryset customization?
To ensure portability when using database-specific features in Queryset customization, it's recommended to leverage Django's abstraction layer. Django provides an ORM that abstracts away the underlying database details. You can use database-agnostic methods and operators provided by Django's Query API and avoid relying on database-specific features directly. Additionally, you can use database routing and configuration settings in Django to handle different database backends if needed.
6.4 Are there any performance implications of using complex query customizations?
Complex query customizations can have performance implications depending on the nature of the queries and the size of the data. While Queryset customization offers flexibility, it's important to optimize queries for performance. Consider factors such as database indexing, efficient data retrieval techniques like prefetching, and leveraging appropriate query optimization methods provided by Django. Properly designed and optimized query customizations can improve performance, but it's crucial to benchmark and test the queries to ensure they meet performance requirements.
6.5 Can I combine multiple Queryset customizations together?
Yes, you can combine multiple Queryset customizations together. Querysets are chainable, allowing you to apply multiple customizations in a single query. You can call custom query methods sequentially to build upon the previous customizations and create more complex queries. This provides a powerful way to create tailored queries that meet specific requirements by combining different filtering, ordering, and aggregation operations.
7. Conclusion
In conclusion, Queryset customization in Django offers developers the flexibility to tailor database queries to their specific needs. By customizing Querysets, you can construct complex queries, optimize performance, transform data, and seamlessly integrate with external systems. However, it's important to strike a balance between query complexity and code readability, follow best practices for efficient query design, and thoroughly test and document your customizations. With the right approach, Queryset customization empowers you to harness the full potential of Django's ORM and build powerful, efficient, and maintainable applications. Happy customizing!
8. Reference
- https://sayari3.com/articles/32-custom-managers-and-queryset-methods-in-django/
- https://subscription.packtpub.com/book/web-development/9781838981952/1/ch01lvl1sec06/working-with-querysets-and-managers
- https://blog.devjunction.in/how-to-customize-the-queryset-in-the-django-admin-change-list-view
- https://ilovedjango.com/django/orm/django-custom-managers/
- https://riptutorial.com/django/example/22038/define-custom-managers
- https://micropyramid.com/blog/how-to-add-a-custom-managers-in-django