Enhanced Django IP Address Detection: Efficient Logging in High Traffic Applications
By JoeVu, at: May 18, 2024, 3:58 p.m.
Estimated Reading Time: __READING_TIME__ minutes

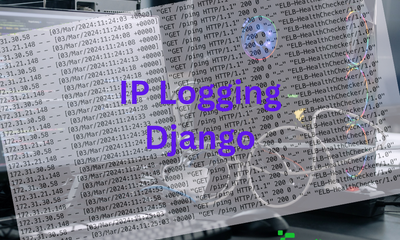
Introduction
Logging user requests in Django applications, particularly for authenticated users, is crucial for security and analytics. However, the challenge of handling massive volumes of data efficiently can be daunting. This post explores practical/commercial solutions to this challenge, emphasizing Django middleware customizations and NGINX logging.
The Task: Logging User Requests
Logging every request made by authenticated users is important for monitoring and security purposes, especially for finance applications. Capturing the source IP address of these requests allows for a detailed analysis of user interactions and potential security breaches. This is a basic step for every secure mechanism.
The Challenge: High Volume Data Management
With hundreds of thousands of requests each day, the high volume of data poses significant challenges in storage, performance, and cost. Efficiently managing this data is paramount to maintaining system integrity and responsiveness.
The Solutions
Solution 1: Custom Middleware with Django-ipware
Solution Overview:
Utilizing django-ipware, we can create custom middleware to extract and log the IP address of each request. This approach minimizes database load while ensuring that essential data is captured.
Code Snippet:
# Install django-ipware
# pip install django-ipware
# Middleware Example
from ipware import get_client_ip
class LogUserIPMiddleware:
def __init__(self, get_response):
self.get_response = get_response
def __call__(self, request):
client_ip, is_routable = get_client_ip(request)
if client_ip is not None:
# Store the IP on the request object
request.user_ip = client_ip
# Here, you can also implement logging logic or any other action with the IP
response = self.get_response(request)
return response
Pros:
- Lightweight and easy to integrate.
- Minimizes direct database interactions.
Cons:
- Still requires custom logic to efficiently log and manage IP data without straining the database.
- Make sure you have
try/catch
to avoid unwanted exceptions blocking your application
Improvements:
- Implement asynchronous logging to a separate data store optimized for write-heavy operations, reducing the load on the primary database. Celery task is great tool.
Solution 2: Utilizing Django-request for Comprehensive Request Logging
Solution Overview:
The Django-request package offers a robust framework for tracking and logging web requests in Django applications. It captures detailed request data, including IP addresses, without the need for custom middleware, making it an attractive option for developers looking to implement comprehensive logging with minimal setup.
Code Snippet:
First, install django-request
using pip:
pip install django-request
Then, add django_request
to your INSTALLED_APPS
in settings.py
and include its middleware in the MIDDLEWARE
settings:
INSTALLED_APPS = [
...
'django_request',
...
]
MIDDLEWARE = [
...
'request.middleware.RequestMiddleware',
...
]
django-request
automatically logs every incoming request, including its IP address, view used, response code, and more. You can review and analyze these logs through the Django admin interface, provided by the package.
Pros:
- Easy to install and integrate into existing Django projects.
- Automatically logs a wide range of request details, including IP addresses, without additional code.
- Provides a Django admin interface for viewing and analyzing request logs.
Cons:
- Storing extensive request data for every request can significantly increase database size and potentially impact performance.
- Less flexibility compared to custom solutions for filtering which requests to log or summarizing data before storage.
Improvements:
- Implement data retention policies to periodically clean up old logs, helping manage database size and performance.
- Customize the logging settings to exclude less critical requests or to log only specific details, reducing the storage requirement.
Solution 3: Leveraging NGINX Logging
Solution Overview:
Configuring NGINX to log IP addresses offloads the data collection workload, allowing for efficient handling of high-volume traffic.
Code Snippet:
To enable IP logging in NGINX, you can add the following to your NGINX configuration:
log_format main '$remote_addr - $remote_user [$time_local] "$request" '
'$status $body_bytes_sent "$http_referer" '
'"$http_user_agent" "$http_x_forwarded_for"';
access_log /var/log/nginx/access.log main;
This configuration captures the client IP address ($remote_addr
) along with other request details.
Pros:
- Offloads logging from the Django application, enhancing performance.
- Provides a robust and scalable solution for logging high volumes of data.
Cons:
- Requires additional setup and configuration outside of Django.
- Accessing and analyzing the logged data might require extra tools or processes.
Improvements:
- Implement a log rotation and archiving strategy to manage log size and retention effectively.
- Use a log management tool to index and analyze the log data, providing insights without direct database queries.
Conclusion
In high-traffic Django applications, efficient request logging is crucial but challenging. This post has introduced three effective solutions: custom middleware with django-ipware for targeted logging, NGINX for scalable system-level logging, and django-request for an easy, comprehensive logging setup. Each approach offers unique benefits, from precise control to ease of implementation, catering to diverse application needs and performance considerations. The key to successful logging lies in choosing the solution that best aligns with your specific requirements, ensuring detailed insights without sacrificing system performance.