FastAPI and SQLAlchemy: A Powerful Combination for Building High-Performance Web Applications
By khoanc, at: May 27, 2023, 7:42 p.m.
Estimated Reading Time: __READING_TIME__ minutes

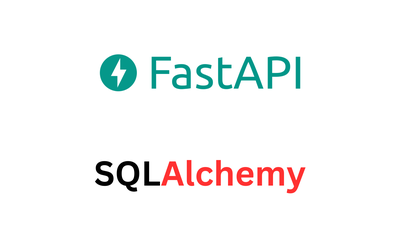
FastAPI and SQLAlchemy: A Powerful Combination for Building High-Performance Web Applications
1. Introduction
Two powerful packages (FastAPI and SQLAlchemy), that play a significant role in modern web development, are easy to integrate together. In which, FastAPI is a high-performance web framework for building APIs with Python, while SQLAlchemy is a widely used Object-Relational Mapping (ORM) tool that simplifies database interactions.
FastAPI has gained immense popularity in recent years due to its unique combination of speed, simplicity, and robustness. It is built on top of the Starlette framework and leverages Python type annotations for automatic data validation and documentation generation. In addition, FastAPI's asynchronous capabilities allow it to handle high loads efficiently, making it an excellent choice for building scalable web applications. (Facts: Django also support async since version 3.1 - 2020)
Beside, SQLAlchemy includes a comprehensive set of tools for working with databases in Python. It offers a powerful and flexible ORM, allowing developers to interact with databases using Python objects, rather than writing raw SQL queries. SQLAlchemy supports various database systems, making it a versatile choice for projects with different data storage requirements.
Both FastAPI and SQLAlchemy have gained recognition and widespread adoption within the Python community. Their features all come together at once that allows simplifying complex tasks, enhancing developer productivity, and delivering high-performance solutions. By leveraging the strengths of both packages, developers can create robust and scalable web applications with ease.
In the upcoming sections of this article, we will explore the those packages in depth in different angles.
2. Is FastAPI worth learning?
2.1 Discuss the benefits of learning FastAPI
FastAPI offers several compelling benefits that make it worth learning for web developers.
- Intuitive and easy-to-use API design allows developers to quickly build robust and scalable APIs
- Leverage Python's type annotations to automatically validate and serialize request and response data
- Reduce the likelihood of errors
- Enhance code maintainability
2.2 Highlight its efficiency, high performance, and scalability
One of FastAPI's standout features is its exceptional performance. By utilizing asynchronous programming and leveraging the high-performance capabilities of the underlying Starlette framework, FastAPI can handle a large number of concurrent requests with ease. This makes it an ideal choice for applications that require high throughput and low latency.
Furthermore, FastAPI's efficient request routing and automatic serialization/deserialization of data help reduce overhead, resulting in faster response time. Its ability to handle heavy workload efficiently makes it well-suited for highly-scalable applications.
2.3 Mention its growing adoption in the industry
FastAPI has witnessed a significant surge in adoption within the industry. Its powerful features and excellent performance have attracted the attention of developers and organizations worldwide. Many prominent companies (ex: Uber and Netflix) have chosen FastAPI for their projects, contributing to its growing ecosystem and community support. This increasing adoption ensures that learning FastAPI opens up new opportunities to work on a wide range of projects and collaborate with like-minded developers.
2.4 Drawbacks of FastAPI, how to resolve/minimise them
While FastAPI offers numerous advantages, it is essential to consider its potential drawbacks.
As a relatively new framework, FastAPI might have a steeper learning curve for developers who are accustomed to other frameworks like Flask or Django. However, the well-structured documentation, vibrant community, and numerous online resources available can help address any learning challenges.
Additionally, FastAPI's reliance on Python type annotations may require developers to have a good understanding of type hints. However, this feature also enhances code readability, reduces bugs, and facilitates API documentation generation.
By being aware of these potential challenges and utilizing the available resources, developers can effectively overcome them and fully leverage the benefits and capabilities of FastAPI.
3. Which ORM to use with FastAPI? How to connect SQLAlchemy with FastAPI?
3.1 Compare and contrast different ORMs compatible with FastAPI?
When it comes to choosing an ORM (Object-Relational Mapping) tool to work with FastAPI, developers have several options to consider. Some popular ORMs compatible with FastAPI include SQLAlchemy, Tortoise ORM, and GINO.
While Tortoise ORM and GINO offer their own unique features and advantages, SQLAlchemy stands out as a versatile and powerful ORM that has been widely adopted in the Python community. It provides a comprehensive set of tools and features for working with databases, making it a preferred choice for many developers.
3.2 Discuss the advantages of SQLAlchemy as a versatile and powerful ORM
The advantages of SQLAlchemy are numerous.
- Support a wide range of database systems, including PostgreSQL, MySQL, SQLite, and more. This versatility allows developers to seamlessly switch between different database engines without having to rewrite their code.
- Provide a flexible and expressive syntax for constructing database queries. It offers both an Object-Relational API, where database tables are represented as Python classes, and a SQL Expression Language, which allows developers to write raw SQL queries using Pythonic syntax.
This flexibility empowers developers to choose the level of abstraction that best suits their needs.
3.3 Explain the role of SQLAlchemy as an Object-Relational Mapping (ORM) tool
As an ORM tool, SQLAlchemy acts as a bridge between the application's object-oriented code and the relational database. It enables developers to interact with the database using Python objects and methods, abstracting away the complexity of writing raw SQL queries. SQLAlchemy handles the translation of Python objects into database tables, allowing seamless data manipulation and retrieval.
By utilizing SQLAlchemy's ORM capabilities, developers can focus on writing Python code that represents their application's domain logic, while SQLAlchemy takes care of translating those operations into the appropriate database queries.
3.4 Provide step-by-step instructions on integrating SQLAlchemy with FastAPI
To integrate SQLAlchemy with FastAPI, follow these steps:
- Install the required packages: Use pip to install
sqlalchemy
and the appropriate database driver for your chosen database system (e.g., "psycopg2" for PostgreSQL). - Import the necessary modules: In your FastAPI application,
import
the required SQLAlchemy modules, such ascreate_engine
anddeclarative_base
- Create a SQLAlchemy engine: Set up a SQLAlchemy engine by providing the database URL, which includes the necessary connection details.
- Define database models: Create Python classes that represent your database tables by subclassing SQLAlchemy's
declarative_base
class and defining the necessary columns and relationships. - Create a session: Use the SQLAlchemy's session to interact with the database. You can create a session by using the
sessionmaker
function and binding it to the SQLAlchemy engine. - Use SQLAlchemy within FastAPI: Now, you can utilize the SQLAlchemy session within your FastAPI application's routes or functions to perform database operations.
3.6 Offer an example of using SQLAlchemy with FastAPI, with detail description
Here's an example that demonstrates the integration of SQLAlchemy with FastAPI:
from fastapi import FastAPI
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.orm import declarative_base, sessionmaker
# Step 1: Install the required packages
# Step 2: Import the necessary modules
Base = declarative_base()
app = FastAPI()
# Step 3: Create a SQLAlchemy engine
engine = create_engine("sqlite:///example.db", echo=True)
# Step 4: Define database models
class User(Base):
__tablename__ = "users"
id = Column(Integer, primary_key=True)
name = Column(String)
email = Column(String)
# Step 5: Create a session
Session = sessionmaker(bind=engine)
session = Session()
# Step 6: Use SQLAlchemy within FastAPI
@app.get("/users/{user_id}")
def get_user(user_id: int):
user = session.query(User).get(user_id)
return user
@app.post("/users")
def create_user(user: User):
session.add(user)
session.commit()
return user
In this example, we create a FastAPI application that interacts with a SQLite database using SQLAlchemy. We define a User
model as a subclass of SQLAlchemy's declarative_base
class and use it in the application's routes to perform database operations.
By following these steps and incorporating SQLAlchemy into your FastAPI application, you can efficiently work with databases and leverage the power and flexibility provided by SQLAlchemy
4. Is FastAPI slower than Flask?
4.1 Provide a comparison between FastAPI and Flask in terms of performance
When comparing the performance of FastAPI and Flask, it is important to note that both frameworks have their strengths and trade-offs. However, FastAPI's design and features give it a performance advantage over Flask in certain scenarios.
FastAPI's performance stems from its use of asynchronous programming. By leveraging the asynchronous capabilities of Python and the underlying Starlette framework, FastAPI can handle concurrent requests more efficiently. As a result, FastAPI has the potential to deliver faster response times and handle higher loads compared to Flask.
4.2 Discuss how FastAPI's asynchronous capabilities contribute to its speed
Asynchronous capabilities play a crucial role in FastAPI's speed and efficiency. Asynchronous programming allows FastAPI to handle multiple requests concurrently, without blocking the execution of other tasks. This non-blocking nature enables FastAPI to make more efficient use of system resources, resulting in high performance.
By utilizing asynchronous features such as async
and await
, FastAPI can perform I/O-bound operations more efficiently. This includes tasks such as accessing databases, making API calls, or performing computationally intensive operations. The ability to handle multiple requests concurrently and efficiently manage I/O operations contributes to FastAPI's speed and responsiveness.
4.3 Benchmarks and real-world use cases
Various benchmarks and real-world use cases demonstrate FastAPI's superior performance compared to Flask. Benchmarks have shown that FastAPI can handle a significantly higher number of requests per second (RPS) than Flask, especially when dealing with I/O-bound operations or high-concurrency scenarios.
Real-world use cases that require handling a large number of concurrent requests, such as real-time applications, data-intensive APIs, or microservices, have witnessed significant performance improvements when using FastAPI. Its ability to efficiently handle high load and I/O-bound operations makes it a preferred choice for applications where speed and scalability are crucial.
However, it is important to note that Flask remains a reliable and widely used framework that excels in simpler applications or cases where asynchronous capabilities are not a requirement. Flask's simplicity and ease of use make it a popular choice for smaller projects or applications with less demanding performance requirements.
5. Can FastAPI replace Flask?
5.1 Analyze the suitability of FastAPI as a replacement for Flask
While FastAPI offers significant performance advantages and a more modern feature set compared to Flask, it may not always be a direct replacement for Flask in every scenario. The suitability of FastAPI as a replacement for Flask depends on various factors, including the specific requirements of the project, the development team's familiarity with the frameworks, and the design philosophy of the application.
5.2 Highlight the difference in design philosophy and use cases
Flask follows a minimalist design philosophy, focusing on simplicity and flexibility. It provides a lightweight framework to quickly build small to medium-sized applications. Flask's simplicity and minimalistic approach make it an excellent choice for rapid prototyping, smaller projects, and applications that require customizability and fine-grained control.
On the other hand, FastAPI embraces modern Python features, such as type hints and asynchronous programming, to provide high performance and productivity. It enforces a stricter structure and encourages the use of standardized patterns, making it suitable for larger applications and projects that prioritize scalability, performance, and maintainability.
5.3 Scenarios where FastAPI may be a better fit
FastAPI shines in scenarios where high performance, scalability, and efficiency are critical. It excels in applications that handle a significant amount of concurrent requests, perform heavy I/O-bound operations, or require real-time capabilities. FastAPI's asynchronous capabilities make it well-suited for building APIs that interact with databases, integrate with external services, or involve real-time data processing.
Furthermore, if your development team is already familiar with modern Python features and async programming, adopting FastAPI can leverage their existing skills and enhance productivity.
FastAPI's automatic generation of API documentation based on type annotations and its support for data validation and serialization also make it an attractive choice for building robust and well-documented APIs.
However, it's important to note that Flask still has its advantages in certain use cases. If simplicity, flexibility, and a lightweight framework are more suitable for your project, or if you require extensive customization and prefer a more hands-on approach, Flask may be the better choice.
6. Should I learn FastAPI or Flask?
6.1 Guidance on choosing between FastAPI and Flask
Choosing between FastAPI and Flask depends on several factors that are specific to your project requirements, development experience, and the ecosystem surrounding each framework. Consider the following factors when making your decision:
- Project Requirements: Evaluate the specific needs of your project. FastAPI is well-suited for high-performance applications that require scalability, asynchronous capabilities, and real-time functionalities. Flask, on the other hand, is a great choice for smaller projects, prototypes, or applications that require flexibility and customization.
- Learning Curve: Consider your team's familiarity with Python frameworks and their learning preferences. FastAPI, with its emphasis on modern Python features and async programming, may require a steeper learning curve, especially for developers who are new to these concepts. Flask, with its minimalist design and simplicity, has a shallower learning curve and is easier to get started with.
- Ecosystem and Community Support: Assess the availability of libraries, extensions, and community support for each framework. Flask has a mature and extensive ecosystem with a wide range of third-party libraries and extensions, making it easier to find solutions and leverage existing tools. FastAPI, although relatively newer, has a growing ecosystem with increasing community support and a focus on modern Python development practices.
6.2 Highlight the strengths and weaknesses of each framework
FastAPI strengths:
- High performance and scalability due to its asynchronous capabilities
- Automatic API documentation generation based on type annotations
- Built-in data validation and serialization
- Modern Python features and support for async programming
- Growing community support and adoption
FastAPI weaknesses:
- Steeper learning curve, especially for developers new to async programming and modern Python features
- Relatively newer framework with a smaller ecosystem compared to Flask
Flask strengths:
- Simplicity and flexibility, making it easy to get started
- Extensive ecosystem with a wide range of third-party libraries and extensions
- Customizability and fine-grained control over application structure
- Large community with a wealth of resources and support
Flask weaknesses:
- Not as performant or scalable as FastAPI, particularly in high-concurrency scenarios
- Lack of built-in support for certain modern Python features, such as async programming
7. Is FastAPI better than Django?
7.1 Compare FastAPI and Django in terms of features and performance
FastAPI and Django are both popular Python web frameworks, but they differ in their design philosophies and target use cases. Comparing the two frameworks in terms of features and performance can help you determine which one is better suited for your specific needs.
FastAPI stands out for its high performance and scalability. It leverages asynchronous programming and modern Python features to handle concurrent requests efficiently. FastAPI's asynchronous capabilities make it particularly well-suited for building APIs that require real-time functionalities, handle heavy load, or involve extensive I/O operations.
On the other hand, Django offers a full-featured and batteries-included framework for web development. It follows a monolithic design philosophy and provides a robust set of tools and features for building complex applications. Django excels in areas such as content management systems, e-commerce platforms, and applications that require a comprehensive administration interface.
When it comes to performance, FastAPI has an advantage due to its asynchronous nature and focus on high-concurrency scenarios. However, Django's performance can still be optimized by employing caching mechanisms, employing efficient database queries, and using appropriate scaling techniques.
7.2 The differences in design philosophy and target use cases
FastAPI and Django have different design philosophies and cater to different target use cases:
FastAPI's design philosophy revolves around speed, performance, and modern Python development practices. It embraces asynchronous programming, type hints, and automatic API documentation generation based on type annotations. FastAPI is well-suited for building APIs that require high performance, scalability, and real-time capabilities.
Django, on the other hand, emphasizes the principle of "batteries included" and follows a monolithic design philosophy. It provides a comprehensive set of tools and features out-of-the-box, such as an ORM, a templating engine, and an administration interface. Django shines in building complex applications that require extensive functionality, content management systems, and applications with a strong focus on database interactions.
7.3 Advantages and disadvantages
Advantages of FastAPI:
- High performance and scalability, especially in high-concurrency scenarios
- Efficient handling of asynchronous operations and real-time functionalities
- Modern Python features and support for type annotations
- Automatic API documentation generation based on type hints
Disadvantages of FastAPI:
- Steeper learning curve, especially for developers new to async programming and modern Python features
- Smaller ecosystem compared to Django
- Less suited for complex applications that require a comprehensive set of features out-of-the-box
Advantages of Django:
- Comprehensive set of features and tools for building complex applications
- Rich ecosystem with a wide range of third-party libraries and extensions
- Strong community support and extensive documentation
- Suitable for content management systems, e-commerce platforms, and applications with a strong focus on database interactions
Disadvantages of Django:
- Less performant in high-concurrency scenarios compared to FastAPI
- Heavier footprint and longer development time for simpler projects
- Less flexible and customizable compared to FastAPI
8. Why is FastAPI so popular?
8.1 Explore the reasons behind FastAPI's popularity
FastAPI has gained significant popularity in the Python web development community for several reasons:
- Intuitive API Design: FastAPI provides a clean and intuitive API design that is easy to understand and use. It leverages modern Python features such as type hints and decorators to create self-documenting and expressive APIs. This simplicity and developer-friendly approach contribute to its popularity.
- Performance and Modern Features: FastAPI's asynchronous capabilities and focus on performance make it appealing to developers working on high-concurrency applications or real-time functionalities. It leverages modern Python features such as async and await, allowing for efficient handling of concurrent requests and I/O operations.
- Growing Ecosystem and Community Support: FastAPI benefits from a growing ecosystem of libraries, extensions, and community support. While the ecosystem may not be as mature as Django's, it is expanding rapidly, offering more resources and solutions for developers. The active community also contributes to the framework's popularity by sharing knowledge and providing assistance.
8.2 API design, performance, and modern features
FastAPI's intuitive API design is one of its standout features. It leverages Python's type hints and decorators to create self-documenting APIs, reducing the need for additional documentation efforts (ex: Sphinx). This design approach allows developers to build APIs quickly and efficiently while maintaining code readability.
In terms of performance, FastAPI's asynchronous capabilities enable it to handle a large number of concurrent requests efficiently. By utilizing async and await syntax, it can effectively manage I/O-bound operations and improve response times. This performance advantage makes FastAPI well-suited for applications that require real-time updates or handle heavy workloads.
FastAPI also incorporates modern features such as dependency injection, data validation, and serialization. These features streamline development and help in building robust and secure APIs. Additionally, FastAPI's support for type hints and automatic API documentation generation based on those type hints greatly improves code maintainability and reduces human errors.
8.3 Highlight the support for async programming and type annotations
Async programming is a significant factor contributing to its popularity. By leveraging Python's async and await syntax, FastAPI can handle concurrent requests efficiently, resulting in improved performance and scalability. This makes it ideal for applications that require real-time functionalities, such as chat applications, social media platforms, or IoT systems.
Type annotations, which allow developers to define and enforce data types throughout their codebase, are embraced. This not only enhances code readability but also enables automatic data validation and serialization. The automatic API documentation generation based on type hints further simplifies the development process and improves the overall developer experience.
9. What companies use FastAPI?
9.1 Provide examples of companies or projects that utilize FastAPI
FastAPI has gained significant adoption and is used by a variety of companies and projects. Here are some notable examples:
- Uber: Uber, the renowned ride-sharing platform, utilizes FastAPI for building high-performance APIs. FastAPI's scalability and efficiency align well with Uber's requirements for handling a large volume of real-time requests.
- Microsoft: Microsoft, one of the leading technology companies, has incorporated FastAPI into its projects. FastAPI's asynchronous capabilities and modern features make it an attractive choice for building efficient and responsive APIs within the Microsoft ecosystem.
- J.P. Morgan: J.P. Morgan, a global financial services firm, leverages FastAPI for developing robust and scalable financial applications. FastAPI's high-performance nature and support for asynchronous programming align well with the demands of the finance industry.
9.2 Real-world use cases and success stories
FastAPI has been successfully employed in various real-world use cases across different industries:
- Real-time Analytics: FastAPI's asynchronous capabilities make it suitable for real-time analytics applications that require processing large volumes of data with low latency. Companies in industries such as e-commerce, finance, and IoT leverage FastAPI to build real-time analytics platforms that provide valuable insights to their customers.
- Microservices Architecture: FastAPI's lightweight and high-performance nature make it an excellent choice for building microservices-based architectures. By utilizing FastAPI to create independent microservices, companies can develop scalable and modular systems that are easier to maintain and deploy.
- API Backend for Mobile Applications: FastAPI's efficiency and support for real-time functionalities make it an ideal choice for developing API backends for mobile applications. Companies in the mobile app development space utilize FastAPI to build robust and responsive APIs that power their mobile applications.
9.3 Highlight the versatility and adoption of FastAPI
FastAPI's versatility and adoption can be attributed to its numerous advantages, including its high performance, scalability, and support for modern Python features. Its ease of use, intuitive API design, and extensive documentation have also contributed to its growing popularity.
FastAPI's adoption is not limited to large corporations. It is also widely used by startups, independent developers, and small to medium-sized enterprises (SMEs) due to its efficiency and developer-friendly approach. The framework's versatility allows it to be utilized in a wide range of applications, from small projects to large-scale enterprise systems.
Furthermore, FastAPI's active community and growing ecosystem provide additional resources, libraries, and extensions that further enhance its adoption and make it easier for developers to leverage its capabilities.
10. Is FastAPI a REST API?
10.1 The nature of FastAPI as a framework for building RESTful APIs
Yes, FastAPI is a framework specifically designed for building RESTful APIs. It adheres to the principles and conventions of REST (Representational State Transfer), which is an architectural style for designing networked applications.
10.2 How FastAPI adheres to REST principles and conventions
FastAPI follows the core principles of REST, including:
- Stateless Communication: FastAPI treats each request as an independent transaction, without storing any client state on the server. This allows for scalability and ease of scaling the API to handle a high volume of requests.
- Uniform Interface: FastAPI provides a consistent and uniform interface for interacting with the API. It supports standard HTTP methods (GET, POST, PUT, DELETE, etc.) and adheres to RESTful conventions for resource naming and representation.
- Resource-Based Architecture: FastAPI organizes APIs around resources, where each resource has a unique identifier (URL) and can be operated upon using the supported HTTP methods. This approach promotes a clean and structured API design.
10.3 Highlight thesupport for HTTP methods and status codes
FastAPI fully supports the standard HTTP methods, including GET, POST, PUT, DELETE, and more. Developers can define API routes and map them to specific functions or methods, allowing for handling different HTTP methods and processing requests accordingly.
FastAPI also provides comprehensive support for handling HTTP status codes. Developers can return appropriate status codes in their API responses to indicate the success or failure of a request. This ensures proper communication between the API and clients, adhering to RESTful conventions.
In summary, FastAPI is a framework specifically designed for building RESTful APIs. It adheres to REST principles and conventions, supporting standard HTTP methods and providing flexibility in handling different HTTP status codes. Its design and features make it a powerful tool for developing robust and scalable API backends.
11. Conclusion
In conclusion, learning FastAPI and using it in combination with SQLAlchemy offers numerous benefits, including efficient API development, high performance, and scalability. We encourage readers to explore both frameworks and evaluate their suitability for their projects based on specific requirements. FastAPI and SQLAlchemy provide a robust and versatile toolset for building powerful and modern web applications.
Reference: