How to Build RESTFUL APIs in Flask using flask-restful Package
By khoanc, at: March 9, 2023, 3:30 p.m.
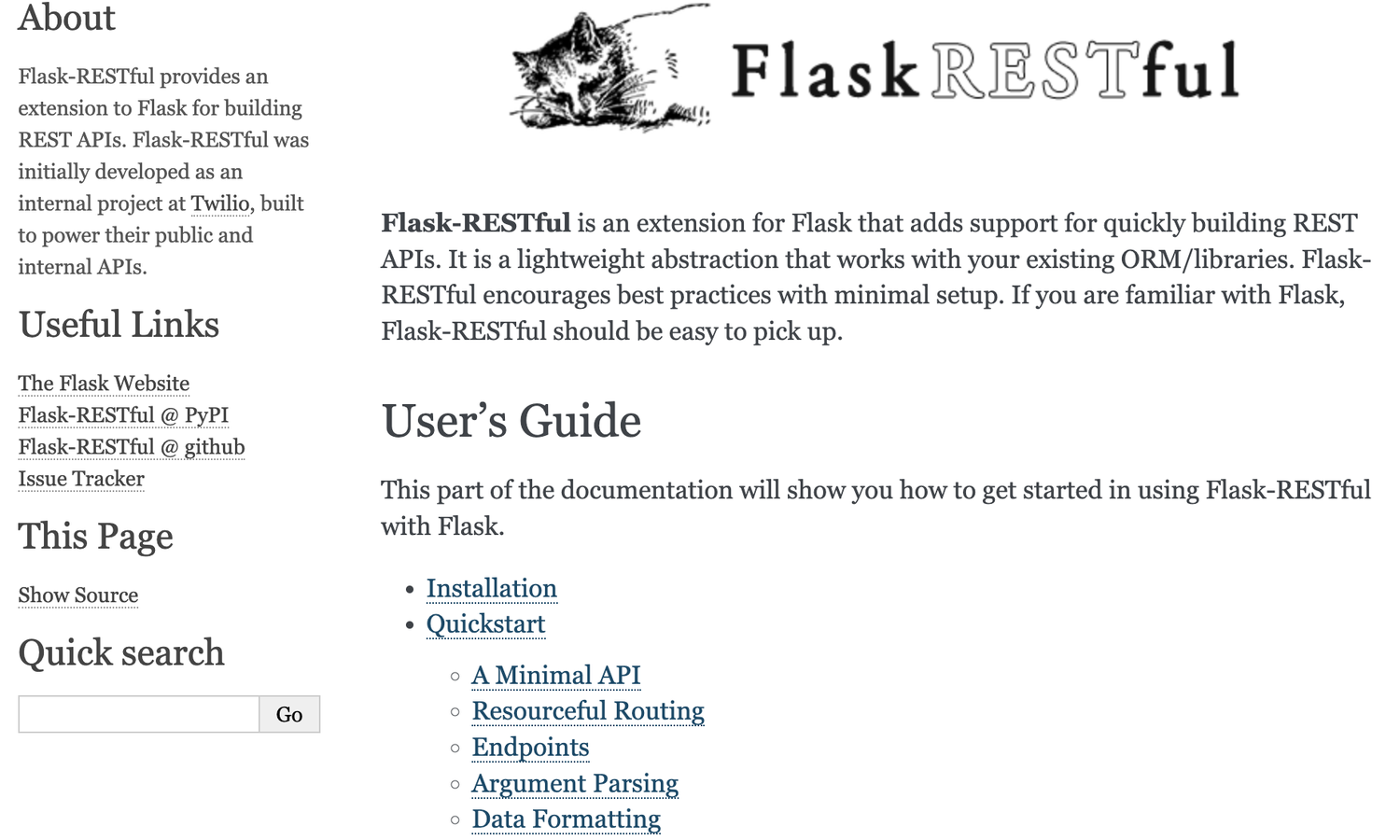
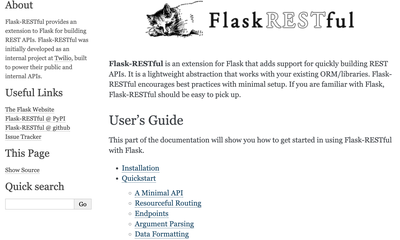
How to Build RESTFUL APIs in Flask using flask-restful Package
1. Introduction
RESTFUL API (Representational State Transfer Application Programming Interfaces) play a crucial role in enabling communication and data exchange between different systems in today's web development landscape. This provides a standardized architecture that allows developers to design scalable, flexible, and interoperable web services. Thanks to REST principles, developers can create APIs that are well-structured, maintainable, and easily consumable by client applications.
Flask, a popular microframework for Python, offers a fast, robust and flexible platform for building web applications, including RESTFUL APIs. Its well-known characteristics are minimalistic, powerful and easy. To further enhance Flask's capabilities for building RESTFUL APIs, the flask-restful package comes into play. This package simplifies the process of creating RESTFUL APIs in Flask by providing a set of intuitive features and decorators that streamline API development.
In this blog post, we will discuss the process of building RESTFUL APIs in Flask using the flask-restful package. We will also dive into the fundamental concepts of RESTFUL architecture and its benefits in modern web development. A complete example is listed at the end of this artcle.
2. FAQs
2.1 How to create an API in Python?
Creating an API in Python using flask-restful is a straightforward process that involves a few simple steps.
1. Install Flask and flask-restful
Open Terminal and type the following commands
pip install Flask
pip install flask-restful
2. Import necessary modules
from flask import Flask
from flask_restful import Api, Resource
3. Create an instance of the Flask application and the flask-restful API
app = Flask(__name__)
api = Api(app) # init the api with the current application
4. Define resources, endpoints, and methods
Define your resources as classes that inherit from the flask-restful Resource class. Within these classes, define the HTTP methods you want to support, such as GET, POST, PUT, DELETE. Ex:
class HelloWorld(Resource):
def get(self):
return {'message': 'Hello, World!'}
def post(self):
pass
api.add_resource(HelloWorld, '/hello')
5. Run the Flask application
At the end of your script, add the following lines to run the Flask application:
if __name__ == '__main__':
app.run(debug=True)
2.2 Is Flask good for API?
Yes, Flask, which is an excellent choice for API developmentm, is a lightweight microframework that allows developers to quickly build web applications and RESTFUL APIs. Here are some reasons why Flask is a good choice for building APIs:
1. Flexibility: Flask offers a high level of flexibility, allowing developers to customize and fine-tune their APIs according to their specific needs. Flask's minimalistic design enables you to choose the components and libraries you want to use, giving you full control over your API development.
2. Simplicity: A straightforward and intuitive approach to web development is the guideline of Flask. The framework has a simple and elegant API that is easy to learn and understand, making it accessible for both beginners and experienced developers.
3. Extensive Ecosystem: Thanks to its easy to use and integrate, many packages/extensions are developed by thousands of developers around the world. The flask-restful extension, which we will explore in this blog post, is a prime example of the rich ecosystem surrounding Flask. These extensions provide additional features and tools that streamline API development and make it even more efficient.
2.3 How to create a REST API?
RESTFUL API implementation involves adhering to certain principles and best practices. REST (Representational State Transfer) is an architectural style that emphasizes a stateless client-server communication model. Here are some guidelines to follow when creating a RESTFUL API using flask-restful:
1. Resource-Oriented Design: Identify the resources your API will expose and design your endpoints around them. Each resource should have a unique URI (Uniform Resource Identifier) that represents its location within the API.
2. Use HTTP Verbs: Leverage the appropriate HTTP verbs (GET, POST, PUT, DELETE, etc.) to perform specific actions on your resources. For example, use GET for retrieving data, POST for creating new resources, PUT for updating existing resources, and DELETE for removing resources.
3. URI Structure: Design your URI structure to be hierarchical and meaningful. Use subpaths to represent relationships between resources and ensure consistency in your naming conventions.
4. Status Codes: Use HTTP status codes to communicate the outcome of API requests. Properly handle and return the relevant status codes such as 200 (OK), 201 (Created), 400 (Bad Request), 404 (Not Found), etc.
5. Request and Response Formats: Determine the formats for sending and receiving data in your API. JSON (JavaScript Object Notation) is a common and widely supported format for RESTFUL APIs due to its simplicity and ease of use.
flask-restful simplifies the implementation of these RESTFUL conventions by providing decorators and methods that align with the principles mentioned above. It handles routing, request parsing, and response formatting, allowing you to focus on the logic of your API.
2.4 How to create an API in Python JSON?
When building a flask-restful API in Python, handling JSON data is a common requirement. flask-restful provides built-in functionality to parse JSON requests and generate JSON responses. Here's how you can handle JSON data in flask-restful APIs:
1. Parse JSON Requests
Use the `request.get_json()` method provided by Flask to extract JSON data from incoming requests. This method automatically parses the request body and returns a Python dictionary containing the JSON data. For example:
from flask import request
class MyResource(Resource):
def post(self):
data = request.get_json()
return {'message': 'JSON data received'}
2. Validate JSON Requests
After parsing the JSON data, you can perform validation to ensure that the received data meets the required criteria. flask-restful allows you to use additional libraries, such as Flask-Inputs
or Cerberus, for validating JSON requests. These libraries provide mechanisms for defining validation rules and handling validation errors.
3. Generate JSON Responses
flask-restful automatically converts Python dictionaries to JSON format when returning responses. Simply return a dictionary from your resource methods, and flask-restful will handle the conversion. For example:
class MyResource(Resource):
def get(self):
data = {'name': 'John Doe', 'age': 30}
return data
By leveraging flask-restful's JSON handling capabilities, you can easily handle JSON data in your Python APIs and ensure seamless communication with clients.
3. Understanding flask-restful and its Benefits
flask-restful is an extension for Flask that simplifies the development of RESTFUL APIs. It provides a set of tools and decorators that streamline the creation of APIs, making the process more efficient and manageable. Let's explore the features and benefits of using flask-restful for API development
3.1 Simplified Routing
Tthe routing of API endpoints by using resource-based routing is easy to setup. Instead of manually defining routes for each endpoint, you define resources that correspond to different entities in your API. flask-restful automatically maps HTTP methods (GET, POST, PUT, DELETE) to the appropriate resource methods, reducing the amount of repetitive code needed. This is similar to the Django-Rest-Framework package (for Django framework)
3.2 Request Parsing
A built-in request parsing capabilities allow an easily access and a request data validation. We can define expected request parameters, enforce data types, and handle validation errors using flask-restful decorators, ensuring that your API receives the correct data format.
3.3 Error Handling
A built-in error handling mechanisms is simplified. It automatically generates appropriate HTTP status codes and error messages for common scenarios, such as invalid requests or resource not found. Additionally, you can customize error handling to meet your specific requirements.
3.4 Content Negotiation
APIs often need to support multiple data formats. flask-restful handles content negotiation, allowing you to easily respond with different data formats based on the client's request. By specifying the expected response format, flask-restful takes care of converting the data into the requested format, such as JSON or XML.
3.5 Input and Output Marshaling
flask-restful offers input and output marshaling capabilities, which help transform complex data structures into simpler representations. This feature allows you to define schemas for input and output data, making it easier to work with complex objects and ensuring consistency in the API responses.
3.6 Authentication and Authorization
Securing your API is crucial, and flask-restful provides mechanisms for handling authentication and authorization. You can integrate authentication schemes, such as JSON Web Tokens (JWT) or OAuth, to protect your API endpoints and control access to specific resources.
3.7 Flask Ecosystem Integration
flask-restful seamlessly integrates with the wider Flask ecosystem, enabling you to leverage other Flask extensions and libraries. You can combine flask-restful with Flask-SQLAlchemy for database integration, Flask-JWT for token-based authentication, or Flask-Cache for caching responses, among many other possibilities. This integration expands the capabilities of your API and allows you to build more sophisticated applications.
4. How to use flask-restful - a complete example
You can find more details in here, and the code snippet below is a complete simple todo app with RESTFUL APIs using Flask-RESTFUL
from flask import Flask, request
from flask_restful import Api, Resource, fields, marshal_with
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__) # init the app
api = Api(app) # init the api
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///todos.db' # setup the database url
db = SQLAlchemy(app) # init the database connection and database instance
# Todo Model class
class TodoModel(db.Model):
id = db.Column(db.Integer, primary_key=True)
title = db.Column(db.String(100), nullable=False)
completed = db.Column(db.Boolean, default=False)
# Resource fields definition
resource_fields = {
'id': fields.Integer,
'title': fields.String,
'completed': fields.Boolean
}
# Todo Detail Resource Class to represent a Todo object in the response
class TodoResource(Resource):
# Get resouroce detail
@marshal_with(resource_fields)
def get(self, todo_id):
todo = TodoModel.query.get(todo_id)
if not todo:
return {'message': 'Todo not found'}, 404
return todo
# Delete resource
def delete(self, todo_id):
todo = TodoModel.query.get(todo_id)
if not todo:
return {'message': 'Todo not found'}, 404
db.session.delete(todo)
db.session.commit()
return {'message': 'Todo deleted'}
# Update resource
@marshal_with(resource_fields)
def put(self, todo_id):
todo = TodoModel.query.get(todo_id)
if not todo:
return {'message': 'Todo not found'}, 404
data = request.get_json()
todo.title = data['title']
todo.completed = data['completed']
db.session.commit()
return todo
# Todo List Resource Class to represent a list of Todo objects in the response
class TodoListResource(Resource):
# Get all todo resources
@marshal_with(resource_fields)
def get(self):
todos = TodoModel.query.all()
return todos
# Create a todo resource
@marshal_with(resource_fields)
def post(self):
data = request.get_json()
todo = TodoModel(title=data['title'], completed=data['completed'])
db.session.add(todo)
db.session.commit()
return todo, 201
api.add_resource(TodoListResource, '/todos') # Register path for LIST API (GET LIST, CREATE)
api.add_resource(TodoResource, '/todos/
<int:todo_id>') # Register path for DETAIL API (GET DETAIL, DELETE, UPDATE)
</int:todo_id>if __name__ == '__main__':
db.create_all()
app.run(debug=True)
Conclusion
Fask-restful, with its rich set of features and an easy integration with Flask, empowers developers to implement RESTFUL APIs efficiently, easily and scalably. Whether you are a beginner or an experienced developer, flask-restful is a powerful choice. So, don't hesitate to dive into flask-restful and unlock the full potential of RESTFUL API development in Flask.
Reference
- https://code.tutsplus.com/tutorials/building-restful-apis-with-flask-diy--cms-26625
- https://auth0.com/blog/developing-restful-apis-with-python-and-flask/
- https://www.analyticsvidhya.com/blog/2022/01/rest-api-with-python-and-flask/
- https://towardsdatascience.com/creating-restful-apis-using-flask-and-python-655bad51b24
- https://realpython.com/flask-connexion-rest-api/