How to Read PDF Files with Python
By JoeVu, at: Dec. 1, 2023, 5:53 p.m.
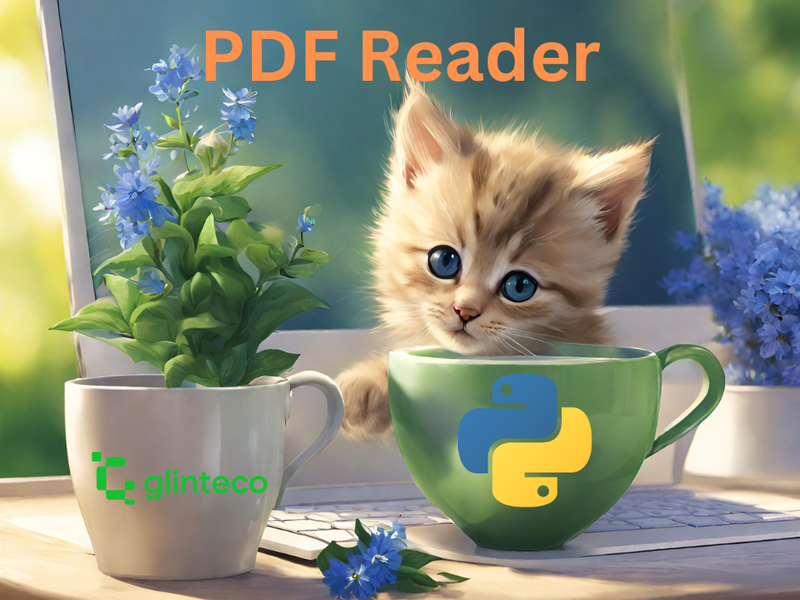

Introduction
Python, with its diverse set of libraries, empowers developers to efficiently extract information from PDF files. In this comprehensive guide, we'll explore various Python libraries (its supported features, pros and cons), including the often-overlooked gem, pdfplumber, to enhance your PDF reading capabilities.
Understanding PDF Files
PDF Structure
To navigate the intricacies of PDF manipulation, understanding the file structure is crucial. PDFs encapsulate text, images, metadata, and interactive elements, forming a complex hierarchy.
Ex: PyPDF2 is a great tool for unstructural PDF files
Text Extraction
The foundation of PDF manipulation lies in text extraction. We'll discuss methods for efficiently extracting text, considering the nuances of different PDF structures. Almost all libraries support the text extraction feature, some might keep the text format.
Libraries for PDF Manipulation in Python
1. PyPDF2
Code Snippet:
import PyPDF2
with open('example.pdf', 'rb') as file:
pdf_reader = PyPDF2.PdfFileReader(file)
text = ""
for page_num in range(pdf_reader.numPages):
page = pdf_reader.getPage(page_num)
text += page.extractText()
Pros:
- Simple and easy to use for basic tasks.
- Good for merging and splitting PDFs.
Cons:
- Limited support for advanced features.
2. pdfminer.six
Code Snippet:
from pdfminer.high_level import extract_text
text = extract_text('example.pdf')
Pros:
- Handles complex PDF structures effectively.
- Provides detailed information extraction.
Cons:
- Steeper learning curve for beginners.
3. PyMuPDF
Code Snippet:
import fitz # PyMuPDF
doc = fitz.open('example.pdf')
text = ""
for page_num in range(doc.page_count):
page = doc[page_num]
text += page.get_text()
Pros:
- Excellent for handling both text and images.
- Efficient and lightweight.
Cons:
- Limited support for interactive features.
4. pdfplumber
Code Snippet:
import pdfplumber
with pdfplumber.open('example.pdf') as pdf:
text = ""
for page in pdf.pages:
text += page.extract_text()
Pros:
- User-friendly and easy to use.
- Provides functionalities for tables and images.
Cons:
- May not be suitable for highly complex PDFs.
Reading PDF Text Content
Basic Text Extraction
We'll kick off with a simple example using PyPDF2 to extract text from a PDF. Understanding these basic methods sets the stage for more advanced techniques.
import PyPDF2
# Open the PDF file in binary mode
with open('example.pdf', 'rb') as file:
# Create a PDF reader object
pdf_reader = PyPDF2.PdfFileReader(file)
# Initialize an empty string to store the extracted text
text = ""
# Iterate through each page in the PDF
for page_num in range(pdf_reader.numPages):
# Get the page
page = pdf_reader.getPage(page_num)
# Extract text from the page and append to the 'text' string
text += page.extractText()
# Print the extracted text
print(text)
Handling Encoded Text
We'll explore techniques to gracefully handle encoding issues, focusing on pdfminer.six for its ability to tackle complex text structures.
from pdfminer.high_level import extract_text
# Specify the path to the PDF file with encoded text
pdf_path = 'encoded_text_example.pdf'
# Extract text using pdfminer.six
text = extract_text(pdf_path, codec='utf-8')
# Print the extracted text
print(text)
Extracting Images from PDF
Using PyMuPDF for Image Extraction
Beyond text, PDFs often contain valuable images. PyMuPDF provides a robust solution for image extraction, and we'll demonstrate its implementation.
import fitz # PyMuPDF
# Specify the path to the PDF file with images
pdf_path = 'pdf_with_images.pdf'
# Open the PDF file
doc = fitz.open(pdf_path)
# Iterate through each page in the PDF
for page_num in range(doc.page_count):
# Get the page
page = doc[page_num]
# Get the images on the page
images = page.get_images(full=True)
# Iterate through each image on the page
for img_index, img_info in enumerate(images):
# Get the image data
img_index = img_info[0]
base_image = doc.extract_image(img_index)
image_bytes = base_image["image"]
# Specify the image file name (you can customize the naming)
image_filename = f"page{page_num + 1}_image{img_index}.{base_image['ext']}"
# Save the image to a file
with open(image_filename, "wb") as image_file:
image_file.write(image_bytes)
# Close the PDF file
doc.close()
pdfplumber for Image Extraction
Let's not forget pdfplumber. We'll showcase how pdfplumber simplifies image extraction and discuss its advantages.
import pdfplumber
# Specify the path to the PDF file with images
pdf_path = 'pdf_with_images.pdf'
# Open the PDF file using pdfplumber
with pdfplumber.open(pdf_path) as pdf:
# Iterate through each page in the PDF
for page_number in range(len(pdf.pages)):
# Get the page
page = pdf.pages[page_number]
# Get the images on the page
images = page.images
# Iterate through each image on the page
for image_index, image in enumerate(images):
# Get the image data
image_data = image['data']
# Specify the image file name (you can customize the naming)
image_filename = f"page{page_number + 1}_image{image_index + 1}.{image['ext']}"
# Save the image to a file
with open(image_filename, "wb") as image_file:
image_file.write(image_data)
Dealing with Image Formats
Extracted images come in various formats. Understanding how to handle different image formats ensures seamless integration into your workflow.
Advanced Techniques
Working with PDF Metadata
Unlock the hidden information within PDFs by exploring metadata. Extracting details like author, creation date, and keywords adds valuable context to your data.
import fitz # PyMuPDF
# Specify the path to the PDF file
pdf_path = 'example.pdf'
# Open the PDF file
doc = fitz.open(pdf_path)
# Get document metadata
metadata = doc.metadata
# Print document metadata
print("Title:", metadata.get('title', 'N/A'))
print("Author:", metadata.get('author', 'N/A'))
print("Subject:", metadata.get('subject', 'N/A'))
print("Creator:", metadata.get('creator', 'N/A'))
print("Producer:", metadata.get('producer', 'N/A'))
print("Creation Date:", metadata.get('created', 'N/A'))
print("Modification Date:", metadata.get('modified', 'N/A'))
# Close the PDF file
doc.close()
Interactive Features and Forms
Navigate the world of interactive PDFs and learn how to programmatically handle form data.
import fitz # PyMuPDF
# Specify the path to the PDF file with forms
pdf_path = 'interactive_pdf_with_forms.pdf'
# Open the PDF file
doc = fitz.open(pdf_path)
# Iterate through each page in the PDF
for page_num in range(doc.page_count):
# Get the page
page = doc[page_num]
# Check if the page has form fields
if page.formWidgetAnnots():
print(f"Page {page_num + 1} has interactive features:")
# Iterate through each form field on the page
for form_field in page.formWidgetAnnots():
field_name = form_field.field_name
field_value = form_field.get_text("")
print(f"Field Name: {field_name}, Field Value: {field_value}")
print("\n")
# Close the PDF file
doc.close()
Best Practices for Efficient PDF Reading in Python
Memory Management
Efficient memory usage is critical, especially when dealing with large PDF files. We'll share best practices to optimize memory management.
-
age-wise Processing: Rather than loading the entire PDF into memory at once, consider processing pages one at a time. This approach minimizes the memory footprint, making it more feasible to handle large documents.
-
Resource Release: Explicitly release resources and close PDF files once they are no longer needed. Forgetting to close files can lead to memory leaks, causing unnecessary consumption of system resources.
-
Streaming Techniques: Implement streaming techniques for large PDFs, allowing the application to read and process data in smaller, manageable chunks. This approach reduces the demand on system memory.
-
Caching Mechanisms: Employ caching mechanisms selectively to store frequently accessed or essential data, avoiding the need to repeatedly load the same information from the PDF file.
-
Optimized Libraries: Choose PDF processing libraries that prioritize memory efficiency. Some libraries are specifically designed to handle large documents with minimal memory impact.
Error Handling
PDF reading can be unpredictable. Implement robust error handling to make your code resilient to unexpected scenarios.
Optimizing Code for Large PDFs
Discover strategies to optimize your code for processing large PDFs swiftly, avoiding common pitfalls.
Optimizing code for large PDFs is crucial to ensure efficient processing and responsiveness in your Python application. Dealing with extensive documents requires thoughtful strategies to minimize resource consumption and improve overall performance. Here are key considerations when optimizing code for large PDFs:
-
Page-Level Processing: Instead of loading the entire PDF into memory, adopt a page-by-page processing approach. This method allows you to selectively extract information, reducing the overall memory footprint.
-
Lazy Loading: Implement lazy loading techniques, loading only the necessary components when required. This approach defers resource allocation until specific elements, such as pages or images, are actively accessed.
-
Chunked Reading: Break down large PDFs into smaller chunks or sections for more manageable processing. This can be particularly effective for tasks like text extraction, where handling portions of the document sequentially is feasible.
-
Asynchronous Operations: Leverage asynchronous programming to parallelize operations, enabling the concurrent processing of different parts of the PDF. This can significantly enhance performance, especially when dealing with multi-core systems.
-
Streaming Content: Utilize streaming techniques to process content incrementally without fully loading it into memory. This is particularly beneficial for tasks like text extraction and can prevent memory overflow.
-
Resource Recycling: Explicitly release resources as soon as they are no longer needed. This practice helps prevent memory leaks and ensures efficient resource utilization throughout the PDF processing workflow.
-
Optimized Libraries: Choose or develop libraries optimized for handling large PDFs. Some libraries are specifically designed to manage memory efficiently and handle documents of varying sizes without compromising performance.
-
Pagination Control: If applicable, consider paginating the PDF content dynamically based on user interactions. This ensures that only the relevant sections are processed, reducing the overall workload.
Enhancing Text Extraction Accuracy
Fine-tune your text extraction techniques to ensure accuracy, especially when dealing with intricate layouts.
Conclusion
Armed with the knowledge from this journey, you are now equipped to navigate the complexities of PDFs using Python. From basic text extraction to handling intricate features, you can extract the information you need with confidence.
FAQs
-
Can I use pdfplumber exclusively for PDF manipulation?
- While pdfplumber is a robust tool, the choice of library depends on your specific requirements. Consider the features offered by each discussed library for a tailored solution.
-
How does pdfplumber simplify image extraction compared to other libraries?
- Pdfplumber provides a user-friendly interface specifically designed for extracting images and tables from PDFs, streamlining the process compared to other libraries.
-
Is pdfplumber suitable for beginners?
- Yes, pdfplumber's simplicity makes it accessible for beginners while offering advanced capabilities for more experienced developers.
-
Can pdfplumber handle complex PDF structures?
- Pdfplumber is adept at handling various PDF structures, making it suitable for a wide range of PDF manipulation tasks.
-
Are there any limitations to using pdfplumber?
- While pdfplumber is a powerful tool, like any library, it may have limitations. Always refer to the documentation and consider your specific use case.