How to setup a Python working environment - Mac
By khoanc, at: April 30, 2023, 11:27 a.m.
Estimated Reading Time: __READING_TIME__ minutes

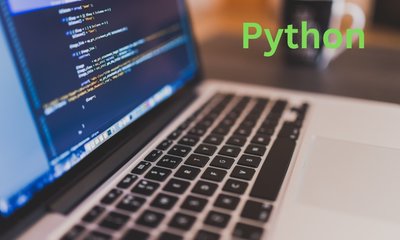
Setting up your Python working environment is crucial to ensure that you can work efficiently and effectively on your Python projects. In this article, we'll provide detailed instructions on how to set up your Python working environment in Mac.
1. Installing Python
Mac comes with Python pre-installed, but it's usually an older version. To install the latest version of Python, we recommend using pyenv, which is a Python version management tool.
To install pyenv, open your Terminal/iTerm and type the following command:
brew install pyenv
This will install pyenv on your Mac.
If you haven't installed brew, please run this command
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
brew install pyenv
After installing pyenv, you can install the latest version of Python by typing the following command
pyenv install 3.11.0 # 3.11.0 is the python version.
This will install Python version 3.11.0. You can replace "3.11.0" with any version of Python you prefer.
2. Setting Up a Virtual Environment
There are few ways that we can use to create virtualenv: pyenv-virtualenv vs venv
2.1 The built-in virtual environment
A virtual environment is a tool that allows you to create an isolated Python environment for each project you work on. This helps to keep your project dependencies separate and prevents version conflicts between different projects.
To create a virtual environment, navigate to your project directory in your Terminal and type the following command
python3 -m venv venv
This will create a virtual environment named venv
in your project directory. You can replace venv
with any name you prefer.
To activate the virtual environment, type the following command
source venv/bin/activate
To deactivate the virtual environment
deactivate
2.2 The pyenv-virtualenv
pyenv-virtualenv is a pyenv plugin that provides features to manage virtualenvs and conda environments for Python on UNIX-like systems.
Install pyenv-virtualenv
brew install pyenv-virtualenv
To create a virtual environment, navigate to your project directory in your Terminal and type the following command
pyenv virtualenv 3.11.0 myenv
This will create a virtual environment named "env" in your project directory. You can replace "env" with any name you prefer.
To activate the virtual environment, type the following command
pyenv activate myenv
pyenv deactivate myenv
REMEMBER: always use virtual environment for your development by running the commands
pyenv activate myenv # if you use pyenv
source venv/bin/activate # if you use venv
3. Choosing an Editor
Choosing an editor is a matter of personal preference. There are several editors available for Mac, including Visual Studio Code, PyCharm, and Sublime Text.
Visual Studio Code is a popular and lightweight editor that has excellent support for Python. PyCharm is a powerful and full-featured Python IDE that's specifically designed for Python development. Sublime Text is a lightweight and customizable text editor that has support for many programming languages.
Check our detail blog for more information
4. Using IPython Shell
IPython is an interactive shell for Python that provides enhanced features over the default Python shell. To install IPython, type the following command in your Terminal
pip install ipython
After installing IPython, you can launch it by typing the following command in your Terminal:
ipython
This will launch the IPython shell, where you can test your Python code interactively.
You can try copy and paste your code here and execute it
def sum_up(x, y, z):
return x + y + z
print(sum_up(10, 20, 30))
5. Using a Debugging Tool
Debugging is an essential part of Python development, and there are several debugging tools available for Mac. One popular debugging tool is PyCharm's integrated debugger, which allows you to step through your code and inspect variables.
Or you can simply put breakpoint()
where you want to debug
For example
Assuming I have a example.py
file with content as below
def find_even_numbers(a_list):
even_numbers = []
for element in a_list:
if element % 2 == 1:
even_numbers.append(element)
return even_numbers
if __name__ == "__main__":
print(find_even_numbers([1, 3, 4, 7, 8]))
The function find_even_numbers
does not work correctly so we can debug it like
def find_even_numbers(a_list):
breakpoint()
even_numbers = []
for element in a_list:
if element % 2 == 1:
even_numbers.append(element)
return even_numbers
Run the example.py
as
python example.py
You will see
❯ python example.py
> /Users/joe/Downloads/example.py(3)find_even_numbers()
-> even_numbers = []
(Pdb) h
Documented commands (type help <topic>):
========================================
EOF c d h list q rv undisplay
a cl debug help ll quit s unt
alias clear disable ignore longlist r source until
args commands display interact n restart step up
b condition down j next return tbreak w
break cont enable jump p retval u whatis
bt continue exit l pp run unalias where
Miscellaneous help topics:
==========================
exec pdb</topic>
the execution will stop at the line even_numbers = []
for debugging.
6. Conclusion
Setting up your Python working environment is essential to ensure that you can work efficiently and effectively on your Python projects. By following the steps outlined in this article, you should be able to set up your Python working environment in Mac without any issues. Happy coding!