How To Write Unit Test in Python - Beginner
By JoeVu, at: Jan. 2, 2023, 11 a.m.
Estimated Reading Time: __READING_TIME__ minutes
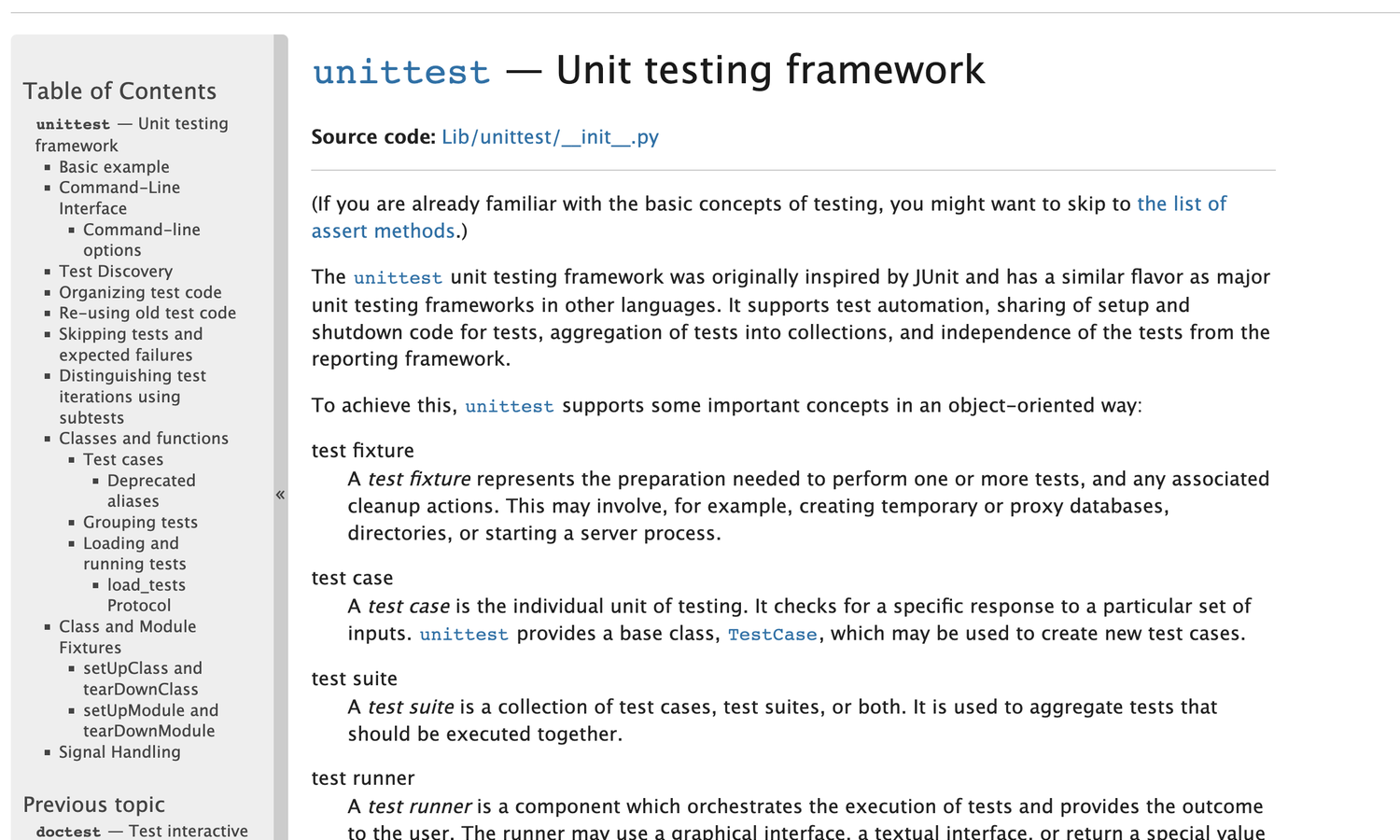
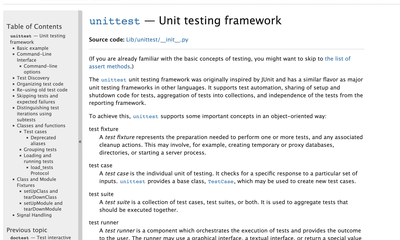
How To Write Unit Test in Python - Beginner
Writing unit tests plays a vital role in software development. Unit tests help protecting harmful changes to the current functions/logic of the application and improving the application accuracy. A project with higher number of code test coverage usually has a higher quality and performance.
In this article, we’ll go over the basics of how to write unit tests (particularly in Python - unittest), including examples and best practices.
What is a Unit Test?
Before we get into how to write unit tests in Python, let’s start by defining what a unit test is. A unit test is a type of automated test that checks an individual piece of code or a "unit” to make sure that it’s functioning as expected. In other word, unittest is a piece of code that is written to test another piece of code.
Unit tests identify bugs earlier, save time/money, and resources because it’s easier to fix a bug when it first appears rather than trying to track down the issue later in the development cycle.
On the other hand, writing unit tests also means more development time at the beginning state but less maintenance cost in a long term.
How to Write Unit Tests in Python
Now that we understand what a unit test is, let’s look at how to write unit tests in Python. Writing unit tests in Python is relatively straightforward and can be done with the help of a few libraries and frameworks.
The most popular library for writing unit tests in Python is the unittest library, which has a set of classes and functions that make it easy to achieve our goals.
The unittest library is part of the Python standard library, so it can be used without the need for any additional libraries or frameworks. The unittest library is well-documented and provides a number of features for writing unit tests in Python.
Examples of Unit Tests in Python
Now that we’ve covered the basics of writing unit tests in Python, let’s look at some examples of unit tests written in Python.
Example 1: Write a test for a function that multiple 2 numbers
1. Write a helpful function in a file libs.py
def multiply(a, b):
return a * b
2. Write a test file named test_libs.py
import unittest
from libs import multiply
class TestMultiply(unittest.TestCase):
def test_multiply(self):
result = multiply(2, 3)
expected_result = 6
self.assertEqual(expected_result, result)
3. Run the test
python -m unittest test_libs.py
Example 2: Write unit tests for a Class
1. Create a new file named subject.py
class Subject:
def __init__(self, name, total):
self.name = name
self.total = total
def is_full(self):
return self.total >= 30
2. Create a new test file test_subject.py
import unittest
from subject import Subject
class TestSubject(unittest.TestCase):
def test_init(self):
subject = Subject('Physics', 25)
self.assertEqual(subject.name, 'Physics')
self.assertEqual(subject.total, 25)
def test_is_full_return_true(self):
subject = Subject('Math', 35)
self.assertTrue(subject.is_full())
def test_is_full_return_false(self):
subject = Subject('English', 17)
self.assertFalse(subject.is_full())
3. Run the test
python -m unittest test_subject.py
There are some pros and cons while working with UnitTest
Pros:
1. Easy to use with minimal setup
2. Python Standard Library
3. Better code design/architecture
4. Powerful assertion methods
Cons:
1. Difficult to debug test failures
2. Difficult to read test results
3. Difficult to maintain large test suites
4. Less ability to run tests in parallel
Best Practices
-
Test Naming: Clear and descriptive
-
Test Independence: Tests are independent and isolated, no test is affected by other tests
-
Test Coverage: Cover all test cases, logic and conditions
-
Single Responsibility: Each test should test ONLY one thing
-
Readability: Clear and readable test code with meaningful variable names
-
Test Setup and Teardown: Use the
setUp()
andtearDown()
to setup proper test data and release memory/redundant data after tests.
-
Test Organization: Group tests by class or functions for better management.
Conclusion
We’ve gone over the basics of how to write unit tests in Python. We’ve also looked at some examples of unit tests written in Python. There will be more to learn and dig further in this blog. Check our blog content for more info.
Reference: