Mastering File Handling in Python: A Comprehensive Guide
By khoanc, at: Jan. 4, 2024, 11:15 a.m.
Estimated Reading Time: __READING_TIME__ minutes

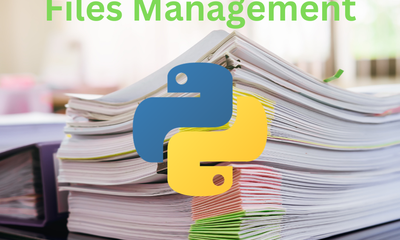
Mastering File Handling in Python: A Comprehensive Guide
Welcome to our in-depth exploration of Python file handling! Efficiently working with files is a fundamental skill for every Python developer, and in this comprehensive guide, we will dive into the intricacies of file I/O, best practices, and advanced techniques. Whether you are just starting your Python journey or are a seasoned developer looking to sharpen your skills, this guide has something for everyone.
1. Opening and Closing Files
Explanation: Opening and closing files is the foundation of file handling in Python. The open()
function is used to open files in various modes (read, write, append, binary).
Sample Code:
try:
with open('example.txt', 'r') as file:
# File operations go here
except FileNotFoundError as e:
print(f"Error: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
Potential Exceptions:
FileNotFoundError
: Raised when the specified file does not exist.- Other general exceptions might occur for various reasons.
2. Reading from Files
Explanation: Reading from files involves methods like read()
, readline()
, and readlines()
to access file content.
Sample Code:
try:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
except FileNotFoundError as e:
print(f"Error: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
Potential Exceptions:
FileNotFoundError
: Raised when the specified file does not exist.- Other exceptions might occur based on file access or content issues.
3. Writing to Files
Explanation: Writing to files involves methods like write()
and writelines()
to add content to a file.
Sample Code:
try:
with open('example.txt', 'w') as file:
file.write("Hello, World!\n")
file.writelines(["Line 2\n", "Line 3\n"])
except Exception as e:
print(f"An unexpected error occurred: {e}")
Potential Exceptions:
- File permission issues might occur.
- Other exceptions might occur based on file access or disk space issues.
4. Context Managers and the with
Statement
Explanation: Using the with
statement ensures proper file closure and exception handling by creating a context manager.
Sample Code:
try:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
except FileNotFoundError as e:
print(f"Error: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
Potential Exceptions:
FileNotFoundError
: Raised when the specified file does not exist.- Other general exceptions might occur for various reasons.
5. File Position and Seek
Explanation: The file cursor position can be adjusted using the seek()
method.
Sample Code:
try:
with open('example.txt', 'r') as file:
content = file.read(10)
print(content)
file.seek(0) # Move the cursor to the beginning
content_again = file.read(10)
print(content_again)
except FileNotFoundError as e:
print(f"Error: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
6. File Iteration
Explanation: You can iterate through lines in a file using a for
loop.
Sample Code:
try:
with open('example.txt', 'r') as file:
for line in file:
print(line.strip())
except FileNotFoundError as e:
print(f"Error: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
7. Working with Text Files
Explanation: Handling text files involves considering encoding issues. The encoding
parameter in the open()
function helps address these concerns.
Sample Code:
try:
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
print(content)
except FileNotFoundError as e:
print(f"Error: {e}")
except UnicodeDecodeError as e:
print(f"Error decoding file content: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
Potential Exceptions:
FileNotFoundError
: Raised when the specified file does not exist.UnicodeDecodeError
: Raised when there is an issue decoding file content.
8. Working with CSV Files
Explanation: The csv
module facilitates reading and writing CSV files, offering various methods to handle CSV data.
Sample Code:
import csv
try:
with open('example.csv', 'r') as file:
reader = csv.reader(file) # or we can use reader = csv.DictReader(csvfile)
for row in reader:
print(row)
except FileNotFoundError as e:
print(f"Error: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
9. Working with JSON Files
Explanation: The json
module simplifies working with JSON data, providing methods to read and write JSON files.
Sample Code:
import json
try:
with open('example.json', 'r') as file:
data = json.load(file)
print(data)
except FileNotFoundError as e:
print(f"Error: {e}")
except json.JSONDecodeError as e:
print(f"Error decoding JSON: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
Potential Exceptions:
FileNotFoundError
: Raised when the specified file does not exist.JSONDecodeError
: Raised when there is an issue decoding JSON content.
10. Error Handling and Exceptions
Explanation: Implementing proper error handling with try-except blocks is crucial to address exceptions that may occur during file operations.
Sample Code:
try:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
except FileNotFoundError as e:
print(f"Error: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
11. File Management
Explanation: File management involves checking file existence, properties, deletion, renaming, and moving operations using the os
module.
Sample Code:
import os
try:
if os.path.exists('example.txt'):
print(f"File size: {os.path.getsize('example.txt')} bytes")
os.rename('example.txt', 'renamed_example.txt')
except FileNotFoundError as e:
print(f"Error: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
12. Working with Directories
Explanation: The os
and os.path
modules provide functions for directory-related operations, such as listing files and creating directories.
Sample Code:
import os
try:
for file in os.listdir('.'):
if os.path.isfile(file):
print(f"File: {file}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
Potential Exceptions:
PermissionError
: Raised when there are insufficient permissions to perform directory operations.- Other general exceptions might occur for various reasons.
13. File Permissions
Explanation: Checking and modifying file permissions can be done using the os
module.
Sample Code:
import os
try:
permissions = os.stat('example.txt').st_mode
print(f"File permissions: {permissions}")
except FileNotFoundError as e:
print(f"Error: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
14. Reading and Writing Large Files
Explanation: Efficiently handling large files involves strategies like buffering and careful memory management.
Sample Code:
try:
with open('large_file.txt', 'r') as file:
for line in file:
# Process line without loading the entire file into memory
pass
except FileNotFoundError as e:
print(f"Error: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
Or if the file contains 1 single super long row, ex: big json data
def read_in_chunks(file_object, chunk_size=1024):
"""Lazy function (generator) to read a file piece by piece.
Default chunk size: 1k."""
while True:
data = file_object.read(chunk_size)
if not data:
break
yield data
with open('really_big_file.dat') as f:
for piece in read_in_chunks(f):
do_sth(piece)
15. File Compression
Explanation: The zipfile
module allows working with zip archives, including compressing and decompressing files.
Sample Code:
import zipfile
try:
with zipfile.ZipFile('example.zip', 'r') as zip_file:
zip_file.extractall('extracted_folder')
except FileNotFoundError as e:
print(f"Error: {e}")
except zipfile.BadZipFile as e:
print(f"Error with ZIP file: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
Potential Exceptions:
FileNotFoundError
: Raised when the specified file does not exist.BadZipFile
: Raised for issues with the ZIP file.
16. Working with Pickle
Explanation: The pickle
module is used for serializing and deserializing Python objects.
Sample Code:
import pickle
try:
data = {'name': 'John', 'age': 30}
with open('example.pkl', 'wb') as file:
pickle.dump(data, file)
except Exception as e:
print(f"An unexpected error occurred: {e}")
Potential Exceptions:
PicklingError
: Raised for issues with pickling objects.- Other general exceptions might occur for various reasons.
17. File Locking
Explanation: File locking prevents conflicts in multi-process or multi-threaded applications. The fcntl
or msvcrt
modules can be used for file locking.
Sample Code: (Note: File locking examples can be platform-specific and complex. Below is a simplified illustration.)
import fcntl
try:
with open('example.txt', 'r') as file:
fcntl.flock(file, fcntl.LOCK_EX) # Acquire an exclusive lock
content = file.read()
print(content)
fcntl.flock(file, fcntl.LOCK_UN) # Release the lock
except FileNotFoundError as e:
print(f"Error: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
Potential Exceptions:
FileNotFoundError
: Raised when the specified file does not exist.- Other general exceptions might occur for various reasons.
18. Working with Binary Files
Explanation: Binary files contain non-textual data and require specific handling. Different data types must be considered when reading or writing binary data.
Sample Code:
try:
with open('binary_file.bin', 'rb') as file:
binary_data = file.read(4) # Read 4 bytes
integer_value = int.from_bytes(binary_data, byteorder='big')
print(f"Integer value: {integer_value}")
except FileNotFoundError as e:
print(f"Error: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
Potential Exceptions:
FileNotFoundError
: Raised when the specified file does not exist.- Other general exceptions might occur for various reasons.
19. Temporary Files and Directories
Explanation: The tempfile
module is used for creating temporary files and directories, useful for temporary data storage.
Sample Code:
import tempfile
try:
with tempfile.NamedTemporaryFile(mode='w', delete=False) as temp_file:
temp_file.write("Temporary data")
print(f"Temporary file created at: {temp_file.name}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
Potential Exceptions:
PermissionError
: Raised when there are insufficient permissions to create a temporary file.- Other general exceptions might occur for various reasons.
20. File Handling Best Practices
Explanation: Summarize best practices for file handling in Python, emphasizing error handling, context managers, and efficient file operations.
Summary:
- Always use the
with
statement for proper file closure. - Implement robust error handling to address potential exceptions.
- Consider encoding when working with text files.
- Be mindful of memory usage, especially when working with large files.
- Use specific modules (
csv
,json
,zipfile
,pickle
) for specialized file formats. - Follow platform-specific best practices, especially for file locking.
FAQs
Q1: Why is file handling important in Python?
A1: File handling is crucial as it enables reading from and writing to files, a fundamental aspect of data manipulation and storage in Python. It allows developers to interact with various file formats, manage data persistence, and implement functionalities like logging and configuration.
Q2: What are context managers, and why are they used in file handling?
A2: Context managers, implemented using the with
statement, ensure proper resource management, such as closing files after usage. In file handling, they enhance code readability, simplify error handling, and guarantee that resources are released when they are no longer needed.
Q3: How can I handle errors when working with files in Python?
A3: Error handling is vital for robust file operations. Wrap file-related code in try-except blocks, catching specific exceptions like FileNotFoundError
or PermissionError
. This allows you to handle errors gracefully and provide meaningful feedback to users.
Q4: What are the best practices for file handling in Python?
A4: Best practices include using the with
statement for automatic resource management, considering encoding when working with text files, and employing specific modules (csv
, json
, etc.) for specialized file formats. Additionally, implementing error handling and adhering to platform-specific conventions ensures code reliability.
Q5: How can I efficiently handle large files in Python?
A5: To handle large files efficiently, use techniques such as reading files line by line, employing buffering, and avoiding loading the entire file into memory. These practices ensure optimal memory usage and prevent performance bottlenecks when working with substantial datasets.