Mastering JavaScript Optimization: 16 Hacks and Tips for Developers
By khoanc, at: Dec. 24, 2023, 12:10 p.m.
Estimated Reading Time: __READING_TIME__ minutes

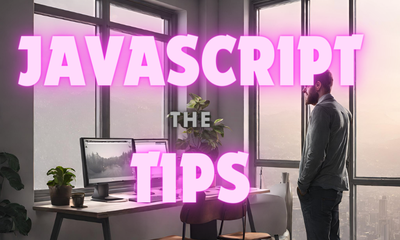
Introduction
JavaScript, or JS, serves as the backbone for implementing complex functionalities on web pages. While many developers understand the importance of minified JavaScript files, only a few are aware of the power of optimized JavaScript code. An optimized codebase combines smartly programmed logic and small hacks to enhance performance, speed, and save development time. In this article, we present 16 sweet JS hacks and tips to help developers optimize JavaScript, improving performance and execution time without compromising server resources.
1. Utilize Array Filter for Efficient Filtering
Optimize your array manipulation by employing the filter
method. This hack efficiently creates a new array filled with elements that pass a specified test, allowing for the creation of a callback function for non-required elements.
let schema = ["hi", "joe", null, null, "goodbye"];
schema = schema.filter(n => n);
// Output: ["hi", "joe", "goodbye"]
This hack streamlines code, saving both time and lines for developers.
2. String Replace Function for Global Replacement
Enhance string manipulation by using the replace
function. To replace all occurrences, employ the replaceAll
function with the /g
flag at the end of a regex.
let string = "hello joe";
console.log(string.replace("e", "o")); // "hollo joe"
console.log(string.replace(/e/g, "o")); // "hollo joo"
3. Debugging with Breakpoints and Consol
Facilitate debugging by strategically placing breakpoints. Use the console and breakpoints to identify and rectify errors at various stages of script execution.
Press F11 for the next function call and F8 to resume script execution.
4. Convert to Floating Number with ~~ Operator
Optimize floating-point number conversion using the ~~
operator. Replace traditional methods like Math.round
for faster micro-optimizations.
let randomNumber = ~~(Math.random() * 100);
// Instead of Math.round(Math.random() * 100)
5. Use Array Length to Delete Empty Elements
Efficiently resize and empty an array using its length property.
let array = [1, 2, 3, 4, 5, 6];
array.length = 3;
console.log(array); // [1, 2, 3]
// For emptying array
array.length = 0;
console.log(array); // []
This technique is preferred for resizing or unsetting array elements.
6. Merge Arrays with Array.concat() and Array.push.apply()
Optimize array merging by using Array.concat()
for small arrays and Array.push.apply()
for larger arrays to reduce memory usage.
let array1 = [1, 2, 3];
let array2 = [4, 5, 6];
console.log(array1.concat(array2)); // [1, 2, 3, 4, 5, 6]
// For larger arrays
Array.push.apply(array1, array2);
console.log(array1); // [1, 2, 3, 4, 5, 6]
7. Use Splice to Delete Array Elements
Optimize array deletion using splice
instead of delete
. This practice saves space and improves JS code speed.
let myArray = ["a", "b", "c", "d"];
myArray.splice(0, 2);
// Result: myArray ["c", "d"]
8. Check Values in an Object
Determine if an object is empty by checking its keys' length.
let isEmpty = Object.keys(YOUR_OBJECT).length === 0;
// Returns true if the object is empty
9. Cache Variables for Improved Performance
Significantly boost JavaScript performance by caching frequently accessed elements instead of repeatedly traversing the DOM.
let cached = document.getElementById('passwordText');
cached.classList.add('cached-element');
This simple optimization impacts performance, especially when processing large arrays in loops.
10. Use Switch Case Instead of If/Else
Enhance script execution time by favoring switch
cases over if/else
statements. The expression to test is evaluated only once in a switch
statement.
switch (expression) {
case value1:
// Code block
break;
case value2:
// Code block
break;
// Additional cases
}
11. Short-Circuit Conditionals for Conciseness
Improve code readability and brevity by leveraging short-circuiting. This occurs when a logical operator doesn't evaluate all its arguments.
// Traditional if statement
if (loggedin) {
welcome_message();
}
// Short-circuited version
loggedin && welcome_message();
By using a combination of a verified variable and the &&
(AND operator), you can achieve the same result more succinctly.
12. Retrieve the Last Item in an Array
Employ the slice
method with negative values to effortlessly obtain the last elements from an array.
let array = [1, 2, 3, 4, 5, 6];
console.log(array.slice(-1)); // [6]
console.log(array.slice(-2)); // [5, 6]
console.log(array.slice(-3)); // [4, 5, 6]
This smart hack simplifies the process of extracting the tail end of an array.
13. Set Default Values with the || Operator
Address the issue of undefined values by providing default values using the ||
operator.
let a = a || 'hello';
// Ensures a has a default value of 'hello' if it's initially falsy or undefined
Developers must be cautious of conflicting values passed to functions to prevent potential bugs.
14. Beautify Your JS Code
Enhance code readability and aesthetics by using tools like jsbeautifier to format messy JavaScript code into a well-structured and organized format.
Before Beautifying:
// Clumsy JS code
function messyCode(){console.log('Hello');}
After Beautifying:
// Well-structured code
function tidyCode() {
console.log('Hello');
}
15. Evaluate JS Performance with jsperf
Assess the efficiency of your JavaScript code and share results with the community using jsperf. It provides a simple way to create and share test cases.
16. Explore Online JavaScript Editors
Experiment with your JavaScript code in real-time using online editors like Jsfiddle and jsbin. These tools not only allow you to test your code but also provide a platform for code sharing.
These 16 JavaScript hacks and tips offer valuable insights for optimizing performance. While it's not necessary to use them in every scenario, incorporating them judiciously based on specific cases and conditions can lead to significant improvements. If you have additional tricks or tips, feel free to share them in the comments section.
Master the art of JavaScript optimization and watch your web applications flourish with enhanced speed and efficiency!