Pytest And Unittest Comparison
By JoeVu, at: March 2, 2023, 9:52 p.m.
Estimated Reading Time: __READING_TIME__ minutes

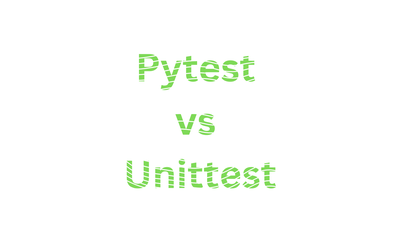
Writing test cases is a crucial part of software development, and two of the most popular and widely used Python testing frameworks are pytest and unittest. They have different approaches to writing tests, and in this article, we’ll look at the differences between them and how they compare. We’ll look at the advantages and disadvantages of each framework, and also provide some tips on when it’s best to use each one.
- Introduction
- What is unittest?
- What is Pytest?
- Benefits of unittest
- Easy to use
- Robust
- Comprehensive
- Flexible
- Scalable
- Benefits of Pytest
- Easy to Use
- Comprehensive Assertions Library
- Flexible Fixtures
- Test Parameterization
- 3.5. Parallel Execution
- Comparison of unittest and Pytest
- Syntax
- Test organization
- Error reporting
- Fixtures
- Performance
- Conclusion
1. Introduction
1.1. What is unittest?
Python unittest is a unit testing framework that is part of the Python Standard Library. Unittest helps developers to automate test cases and make sure that their code is functioning as expected. Unittest is based on the xUnit architecture and is commonly used in Test Driven Development (TDD).
A unit test is a piece of code written by a developer that tests a single unit of functionality in the software. It is an automated process to validate that the code works as expected. Unit tests are written to check for edge cases and make sure the code works in different scenarios.
For example, let's say we have a function that calculates the area of a circle. We can use unittest to validate that the function works correctly. We can create a test class with a setUp() method to create the objects we need and then write our tests.
File test_circle_area.py
import unittest
class TestCircleArea(unittest.TestCase):
def setUp(self):
self.radius = 5
def test_area(self):
result = self.radius**2*3.14
self.assertEqual(result, 78.5)
Run test
python -m unittest test_circle_area.py
In this example, we create a test class called TestCircleArea and a setUp method to create the objects we need. We then define a test_area() method which uses the assertEqual() method to check if the result is equal to 78.5. If it is, then the test passes. If not, then the test fails.
1.2. What is Pytest?
Pytest is a popular testing framework for Python. It is designed to be simple, scalable, and powerful. It is a framework that makes writing and running tests easier by providing tools for organizing and running tests as well as collecting and displaying results. Pytest supports numerous features including test discovery, fixtures, assertions, and more.
An example of using Pytest is to test a function that adds two numbers. The following is a sample code snippet:
File test_add.py
def add(x, y):
return x + y
def test_add():
assert add(2, 3) == 5
Run test
pytest test_add.py
The above code uses the Pytest framework to test the add() function. If the assertion passes, then the test will pass. If the assertion fails, then the test will fail. Pytest will report the results of the test, including any errors encountered.
2. Benefits of unittest
2.1. Easy to use
Python unittest is easy to use and has a simple syntax and structure. Writing tests is simpler and faster than with other frameworks.
2.2. Robust
Python unittest is a robust framework that can handle complex testing scenarios. It is extensible and can be used to test a variety of different types of applications.
2.3. Comprehensive
Python unittest offers a comprehensive set of features for testing. It includes support for test discovery, assertions, test fixtures, and mocking.
2.4. Flexible
Python unittest is highly flexible and can be used to test a wide variety of applications. It can be integrated with other frameworks and libraries to create a powerful testing suite.
2.5. Scalable
Python unittest is highly scalable and can be used to test large applications. It is designed to handle large, complex projects with minimal effort.
3. Benefits of Pytest
3.1. Easy to Use
Python pytest has a simple and intuitive API that makes it easy to write and execute unit tests. It follows a “convention over configuration” approach, meaning that it requires minimal setup and configuration.
3.2. Comprehensive Assertions Library
Python pytest provides a comprehensive set of assertions for testing the behavior of code. It also includes built-in assertions for common situations, such as checking the output of a function or verifying the value of a variable.
3.3. Flexible Fixtures
Python pytest provides a powerful fixture system that allows developers to easily set up and tear down resources for their tests. Fixtures can be used to set up databases, mock objects, or any other resources needed for tests.
3.4. Test Parameterization
Python pytest allows developers to easily parameterize their tests. This makes it easy to run the same test with different inputs or test different scenarios in a single test.
3.5. Parallel Execution
Python pytest supports running tests in parallel, which can significantly reduce the time needed to run a full test suite.
4. Comparison of unittest and Pytest
4.1. Syntax
The main difference between Python unittest and pytest is the syntax and the features they offer. Python unittest uses the traditional xUnit style setup with class-based tests and assert statements, while pytest offers a more concise syntax, which is easier to write and read.
For example, let's say we want to test a function that checks if a number is even or odd. In Python unittest, we would write something like this:
import unittest
def is_even(number):
return number % 2 == 0
class TestIsEven(unittest.TestCase):
def test_is_even_with_even_number(self):
self.assertTrue(is_even(4))
def test_is_even_with_odd_number(self):
self.assertFalse(is_even(3))
if __name__ == '__main__':
unittest.main()
In pytest, this test could be written simpler and in fewer lines of code, like this:
import pytest
def is_even(number):
return number % 2 == 0
@pytest.mark.parametrize("num, expected", [
(4, True),
(3, False)
])
def test_is_even(num, expected):
assert is_even(num) == expected
Overall, Python unittest is a good choice for beginners because it's easy to learn, but experienced developers may prefer pytest for its concise syntax and additional features.
4.2. Test organization
Pytest uses a modular approach to test organization, where tests are organized into test modules. This makes it easier to organize tests into logical groups and allows developers to run individual tests or test modules.
Unittest, on the other hand, uses a class-based approach to test organization. This means that tests are organized into classes, which can be further broken down into test methods. This organization makes it easier to share common setup and teardown code between tests, but can make it harder to run individual tests or test modules.
4.3. Error reporting
Pytest reports errors with more detail and offers more information than unittest. This extra detail can help developers quickly identify the source of errors and address them. Pytest also provides more accurate and concise reporting of errors, which makes it easier for developers to quickly debug and address them.
When it comes to error reporting, unittest takes a more basic approach. Its error messages are much more generic and do not provide as much detail as pytest. This makes it more difficult to identify the source of errors and address them.
4.4. Fixtures
In pytest, fixtures are used to set up test data before tests are run and to clean up after tests have run. Fixtures can also be used to inject dependencies or mock objects into tests. In unittest, fixtures are used to set up and tear down the environment before and after tests are run.
4.5. Performance
Pytest is more flexible and has more features than unittest, such as built-in assert statements, test parametrization, and automatic test discovery. This allows you to write more comprehensive tests with less code, and therefore less time required to run them.
Pytest also has better support for third-party plugins, which can help improve the speed of test runs even further. These plugins can be used to speed up the execution of certain tests, or to add features such as code coverage and profiling.
5. Conclusion
Pytest and unittest are both great tools for testing code. Both are popular, well-supported, and have strong communities. The main difference between them is that pytest is more straightforward, supports more advanced test features, and is easier to use. Unittest is more verbose, requires more setup and maintenance, and is less flexible.
For most developers, I would recommend pytest for its ease of use and advanced features. However, if you need to maintain legacy unittest code, then you should use unittest. Ultimately, it comes down to the preferences of the developers and the needs of the project.
You can learn more about the Python unittest in our blog posts
References