Python Data Structures: A Quick Guide to the Most Commonly Used Types
By hientd, at: Oct. 24, 2024, 3:22 p.m.
Estimated Reading Time: __READING_TIME__ minutes

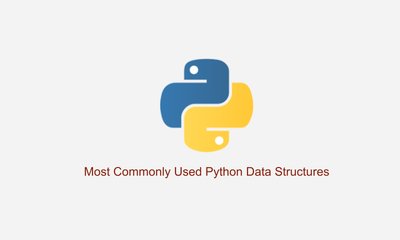
Introduction to Python's Most Commonly Used Data Structures
Python is a versatile programming language that has become increasingly popular due to its simplicity, readability, and flexibility. One of the key aspects that makes Python so powerful is its set of built-in data structures. These data structures allow programmers to organize and manipulate data in a variety of ways, making it easier to write efficient and robust code for a wide range of applications.
In this blog post, we'll introduce you to Python's most commonly used data structures, from basic to advanced. We'll provide examples and reference links to official Python documentation and a Python Data Structures course on Coursera. By mastering these data structures, you'll be able to write Python code more efficiently and effectively.
1. Lists
1.1 What is Python list?
Python Lists are one of the most versatile and commonly used data structures in Python. They are used to store an ordered collection of items, which can be of varying types.
To create a list, you simply enclose a comma-separated sequence of values in square brackets:
my_list = [1, 2, 3, "four", 5.0]
You can access individual elements of a list using their index, which starts at 0:
print(my_list[0]) # output: 1
You can also modify elements of a list by assigning a new value to their index:
my_list[3] = "four!"
print(my_list) # output: [1, 2, 3, 'four!', 5.0]
1.2 Use Cases
Lists can be used in a variety of applications, here are some common use cases
-
Storing and manipulating data: Lists are versatile and essential for both beginners and advanced programmers for storing and manipulating data. For example, you can use a list to store a collection of numbers, strings, or objects.
-
Iteration: Lists provide a simple way to iterate over a collection of elements. You can use a for loop to iterate over a list and perform operations on each element.
-
Sorting and searching: Lists provide built-in methods for sorting and searching elements. You can use the sort() method to sort elements in ascending or descending order. You can also use the index() method to search for the index of a specific element.
1.3 Pros and Cons
-
Pros
-
Versatile: Lists can store any type of data, making them very versatile.
-
Easy to use: Lists are easy to create, access, and modify. They are also intuitive and easy to understand.
-
Built-in methods: Lists provide built-in methods for common operations such as appending, inserting, sorting, and searching.
-
-
Cons
-
Slow for large data sets: Lists can become slow when dealing with large data sets. This is because they are implemented as a dynamic array and need to be resized when new elements are added.
-
Not ideal for certain operations: Lists are not ideal for certain operations such as random access or insertion and deletion from the middle of the list. In such cases, other data structures such as arrays or linked lists may be more appropriate.
-
1.4 Overall
Python List is a versatile and essential data structure for storing and manipulating ordered collections of elements in Python programming. While it has its pros and cons, it remains a fundamental tool for both beginner and advanced programmers.
1.5 More information and examples
2. Tuples
2.1 What is Python Tuples?
Tuples are similar to lists in that they are used to store an ordered collection of items. However, unlike lists, tuples are immutable, meaning that their values cannot be modified after they are created. To create a tuple, you enclose a comma-separated sequence of values in parentheses:
my_tuple = (1, 2, 3, "four", 5.0)
You can access individual elements of a tuple using their index, just like with lists:
print(my_tuple[0]) # output: 1
1.2 Some common use cases
Because tuples are immutable, they can be used in situations where you need to ensure that the values of a collection cannot be modified. For example:
-
Storing a set of values that should not be modified, such as days of the week or months of the year.
-
Returning multiple values from a function.
-
Passing data between functions or modules.
1.3 Pros and Cons
-
Pros
-
Tuples are faster than lists because they are immutable and can be optimized by the interpreter.
-
Tuples can be used as keys in dictionaries because they are immutable.
-
Tuples can be used in situations where you want to ensure that the data is not changed accidentally.
-
-
Cons
-
Tuples cannot be modified, so if you need to add or remove elements, you will need to create a new tuple.
-
Tuples can be confusing to work with because they are similar to lists but have different properties.
-
Tuples do not have as many built-in methods as lists, so you may need to write your own functions to perform certain tasks.
-
1.4 Overall
Python Tuples are a versatile and useful data structure that can be used in a variety of situations, but they may not be the best choice for every situation.
1.5 More information and examples
3. Sets
3.1 What is Python Sets?
Sets are used to store an unordered collection of unique elements. To create a set, you enclose a comma-separated sequence of values in curly braces:
my_set = {1, 2, 3, "four", 5.0}
You can access individual elements of a set, but you cannot modify them:
for element in my_set:
print(element)
3.2 Use Cases
Sets are commonly used in situations where you need to perform operations like union, intersection, or difference on collections of unique elements.
One use case for a set is to remove duplicates from a list or other iterable. Sets can also be used to perform mathematical operations such as union, intersection, and difference. Additionally, they are useful for membership testing and filtering out elements that do not meet certain criteria.
3.3 Pros and Cons
-
Pros: of using a set include its ability to quickly determine membership and to perform mathematical operations. Sets are also mutable and can be modified using methods such as add and remove.
-
Cons: of using a set include its lack of order, which can be problematic in certain situations. Additionally, sets can only store hashable elements, which limits the types of data that can be stored. Finally, sets are not as widely used as other data structures such as lists and dictionaries, which can make them less familiar to some programmers.
3.4 More information and examples
4. Dictionaries
4.1 What is Python Dictionary?
Dictionaries are used to store an unordered collection of key-value pairs. Each key in a dictionary must be unique, and values can be of any type. To create a dictionary, you enclose a comma-separated sequence of key-value pairs in curly braces:
my_dict = {"name": "John", "age": 30, "city": "New York"}
You can access individual values of a dictionary using their key:
print(my_dict["name"]) # output: "John"
You can also modify values of a dictionary by assigning a new value to their key:
my_dict["age"] = 31
print(my_dict) # output: {'name': 'John', 'age': 31, 'city': 'New York'}
4.2 Use Cases
Dictionaries are commonly used in situations where you need to store and access data using a key-value mapping. It is used to represent real-life data like user profiles, product details, and much more. One of the main advantages of dictionaries is that they allow for fast lookup of values based on the key. This makes dictionaries ideal for situations where you need to perform frequent lookups or need to access values based on a specific key.
4.3 Pros and Cons
-
Pros: Despite these limitations, dictionaries are still very useful, and they are widely used in Python programming. They are commonly used for tasks like caching data, storing user preferences, and managing configuration settings.
-
Cons: Some of the cons of dictionaries include the fact that they are not suitable for ordering data and they are mutable, meaning that the values can be changed. Additionally, dictionaries can be memory-intensive if they contain a large number of key-value pairs, which can slow down the performance of your program
4.4 Overall
The pros of using dictionaries outweigh the cons, making them a valuable data structure to have in your programming toolkit.
4.5 More information and examples
5. Queues
5.1 What is Python Queues?
Queues are used to store an ordered collection of elements, similar to lists. However, the key difference between a list and a queue is that items are added to the end of a queue and removed from the front. This makes queues a useful data structure for implementing algorithms like breadth-first search.
To create a queue, you can use the queue.Queue
class in Python's standard library:
import queue
my_queue = queue.Queue()
my_queue.put(1)
my_queue.put(2)
my_queue.put(3)
while not my_queue.empty():
print(my_queue.get())
This will output
1
2
3
5.2 Use Cases
Queues can be used in a variety of applications, such as implementing search algorithms, handling events, and more. Here are some commonly used:
-
Multi-threaded Programming: Queues can be used to pass data between threads in a multi-threaded program. This is useful when multiple threads need to share data, but only one thread can access the data at a time.
-
Networking: Queues can be used to store incoming data packets in a network application. The packets can then be processed in order, ensuring that the application works as expected.
-
Task Processing: Queues can be used to store tasks that need to be processed in order. This is useful in applications such as job schedulers or task queues.
5.3 Pros and Cons
-
Pros:
-
Thread-Safe: Python queues are thread-safe, meaning that they can be used in multi-threaded programs without the risk of data corruption.
-
Synchronized: Queues are synchronized data structures, meaning that they ensure that data is stored and retrieved in the correct order.
-
Easy to Use: Python queues are easy to use and can be implemented quickly in a program.
-
-
Cons:
-
Limited Functionality: Queues are limited in functionality compared to other data structures, such as lists or arrays.
-
Slow: Queues can be slower than other data structures when adding or removing large amounts of data.
-
Fixed Size: Python queues have a fixed size, meaning that they cannot be resized once they are created.
-
5.4 Overall
Python queues are a useful data structure that can be used in a variety of applications. They are thread-safe, synchronized, and easy to use, making them a great choice for multi-threaded programming, networking, and task processing. However, they are limited in functionality and can be slower than other data structures in certain situations.
5.5 More information and examples:
6. Conclusion
In this blog post, we've introduced you to Python's most commonly used data structures, from basic to advanced. We've provided examples and reference links to official Python documentation and a Python Data Structures course on Coursera. By mastering these data structures, you'll be able to write Python code more efficiently and effectively, and tackle a wide range of programming challenges. So start practicing with these data structures today, and see how they can help you build better Python code!