Python Decorators
By JoeVu, at: March 13, 2023, 10:28 p.m.
Estimated Reading Time: __READING_TIME__ minutes

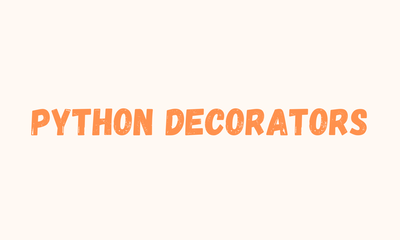
1. Introduction
Python decorators are a powerful and versatile feature of the language that allow you to modify the behavior of functions or classes without changing their source code. Whether you're an experienced Python developer or just starting out, understanding decorators is essential for writing clean, maintainable code. In this article, we'll explore what decorators are, how they work, and some of the most common use cases for decorators in Python. We'll also discuss common pitfalls and how to avoid them, so you can start using decorators effectively in your own code.
1.1 Explanation of what Python decorators are
Decorators are essentially functions that take another function or class as input, and return a modified version of that input. This makes it possible to add functionality to existing code in a clean and modular way, without cluttering up the original code with additional logic. Decorators are widely used in Python libraries and frameworks to implement features such as caching, logging, and authentication, and are a key tool in the toolbox of any serious Python developer.
1.2 Why decorators are useful
- Allow you to add functionality to existing code without modifying that code directly. This is important for keeping your code modular and easy to maintain, as it allows you to separate concerns and keep different aspects of your code in separate modules or files
- Help reduce code duplication by providing a way to apply common functionality to multiple functions or classes. For example, if you have a group of functions that all need to log their inputs and outputs, you can write a logging decorator once and apply it to all of those functions, rather than copying and pasting logging code into each one.
In general, decorators are a great tool for writing clean, concise, and maintainable code in Python.
2. Syntax and basic usage
The basic syntax of a Python decorator involves using the '@' symbol followed by the name of the decorator function, which is then placed above the function that is being decorated. The decorator function takes the function being decorated as its argument and returns a new function, which can then be called instead of the original function.
Here's an example of a basic decorator that simply prints a message before and after the decorated function is called:
def my_decorator(func):
def wrapper():
print("Before the function is called.")
func()
print("After the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
When we call say_hello() in this example, the output will be:
Before the function is called.
Hello!
After the function is called.
As you can see, the decorator function my_decorator() wraps the say_hello() function and adds additional functionality to it. The wrapper() function is returned by my_decorator() and replaces say_hello() in the code. When we call say_hello(), we're actually calling the new wrapper() function, which calls the original say_hello() function and adds the message printing functionality.
Decorators can be used for a wide range of purposes, such as adding logging, timing, authentication, or caching functionality to functions without modifying their code. In fact, decorators are one of the most powerful features of Python, allowing for a great deal of flexibility and customization.
Here's an example of a decorator that logs the name of the decorated function and the arguments it was called with:
def log_func(func):
def wrapper(*args, **kwargs):
print(f"Calling function '{func.__name__}' with arguments: {args}, {kwargs}")
return func(*args, **kwargs)
return wrapper
@log_func
def multiply(x, y):
return x * y
result = multiply(3, 5)
print(result)
When we call multiply(3, 5)
in this example, the output will be:
Calling function 'multiply' with arguments: (3, 5), {}
15
As you can see, the log_func() decorator wraps the multiply() function and adds logging functionality to it. The wrapper() function takes arbitrary arguments using the *args and **kwargs syntax and returns the result of calling the original multiply() function.
3. Decorator types
3.1 Function decorators
Function decorators are the most common type of Python decorators. They are used to modify the behavior of functions or methods by wrapping them with additional code. In Python, functions are first-class objects, which means they can be passed as arguments to other functions and returned as values from functions. This is the key feature that allows for the creation of function decorators.
Ex: timer, log, cache
def log_it(func):
def wrapper(*args, **kwargs):
print(f"Calling function '{func.__name__}' with arguments: {args}, {kwargs}")
return func(*args, **kwargs)
return wrapper
@log_it
def add(x, y):
return x + y
result = add(3, 5)
import time
def time_it(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"Function '{func.__name__}' took {end_time - start_time:.4f} seconds to execute")
return result
return wrapper
@time_it
def multiply(x, y):
return x * y
3.2 Class decorators
We can also define a decorator as a class in order to do that, we have to use a __call__ method of classes. When a user needs to create an object that acts as a function then function decorator needs to return an object that acts like a function, so __call__ can be useful. For Example
from time import time
class TimeIt:
def __init__(self, func):
self.function = func
def __call__(self, *args, **kwargs):
start_time = time()
result = self.function(*args, **kwargs)
end_time = time()
print(f"Function '{self.function.__name__}' took {end_time - start_time:.4f} seconds to execute")
return result
# adding a decorator to the function
@TimeIt
def delay_it(delay_time):
from time import sleep
print(f"delay {delay_time} seconds")
sleep(delay_time)
delay_it(3)
3.3 Nested decorators
Nested decorators are simply decorators that are applied to other decorators. This means that a decorator can itself be decorated with another decorator, creating a nested structure. This is useful for building more complex and customized decorators that can modify multiple aspects of a function or class.
def log_it(func):
def wrapper(*args, **kwargs):
print(f"Calling function: {func.__name__}")
return func(*args, **kwargs)
return wrapper
def time_it(func):
import time
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"Executed in {end_time - start_time} seconds")
return result
return wrapper
@log_it
@time_it
def double_it(x):
return x*2
result = double_it(10)
print(result)
The function double_it will be represented as
double_it(10) = log_it(time_it(double_it))(10)
4. Common pitfalls and how to avoid them
4.1 Not returning a value from the decorator
Decorators must return a function, or else the function being decorated will be replaced with None. This can cause errors or unexpected behavior. Here is an example:
def test_decorator(func):
def wrapper(*args, **kwargs):
# do something
func(*args, **kwargs)
return wrapper
@test_decorator
def test_function():
print("Hello")
test_function() # Output: None
4.2 Applying decorators incorrectly
Decorators are applied from top to bottom, so the order in which they are defined and applied can affect the behavior of the function being decorated. For example, if a decorator modifies the arguments of a function, then decorators that depend on those arguments should be applied afterwards. Here is an example:
def add_two(func):
def wrapper(x):
return func(x + 2)
return wrapper
def multiply_by_four(func):
def wrapper(x):
return func(x * 4)
return wrapper
@multiply_by_four
@add_two
def test_function(x):
return x
print(test_function(3)) # Output: 14
@add_two
@multiply_by_four
def test_function(x):
return x
print(test_function(3)) # Output: 20
4.3 Not preserving the function's metadata
When defining a decorator, it's important to preserve the metadata of the function being decorated, such as its name, docstring, and arguments. One way to do this is by using the functools.wraps decorator, as shown below:
import functools
def log_it(func):
@functools.wraps(func)
def wrapper(*args, **kwargs):
print(f"Calling function '{func.__name__}' with arguments: {args}, {kwargs}")
return func(*args, **kwargs)
return wrapper
@log_it
def test_function():
"""This is a test function"""
return 10
print(test_function.__name__) # Output: "test_function"
print(test_function.__doc__) # Output: "This is a test function"
5. How to avoid overusing or misusing decorators
Decorators are a powerful feature in Python that allow you to modify the behavior of a function or a class without changing its source code. However, if you overuse or misuse decorators, it can make your code harder to read, understand, and maintain. Here are some tips on how to avoid overusing or misusing decorators in Python:
- Use decorators sparingly: Don't use decorators for everything. Use them only when it makes sense and when they make your code more readable and maintainable.
- Keep decorators simple: Don't create complex decorators that do too many things. A decorator should do one thing and do it well.
- Document your decorators: It's important to document your decorators so that other developers can understand what they do and how to use them.
- Don't use too many decorators on one function: Too many decorators can make a function hard to read and understand. Try to limit the number of decorators you use on a single function.
- Don't create circular dependencies: Be careful when using decorators that depend on each other. Circular dependencies can lead to infinite loops or other unexpected behavior.
- Use meaningful names: Choose meaningful names for your decorators so that other developers can easily understand what they do.
- Use functools.wraps: If you're creating a decorator that modifies a function's behavior, be sure to use the functools.wraps decorator to preserve the function's original name and documentation.
By following these tips, you can avoid overusing or misusing decorators in Python and create code that is easy to read, understand, and maintain.