Python Dictionary: Tips, Tricks, and Best Practices
By hientd, at: April 24, 2023, 9:57 a.m.
Estimated Reading Time: __READING_TIME__ minutes

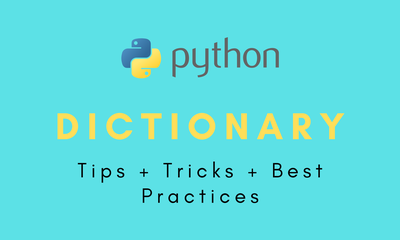
Python Dictionary: Tips, Tricks, and Best Practices
1. Introduction
Python dictionary is a built-in data type that is used to store data in key-value pairs. Each key-value pair is called an item, and dictionaries are created by enclosing key-value pairs in curly braces {} separated by commas. Dictionaries in Python are mutable, unordered, and can have keys of any immutable data type.
Dictionaries are one of the most frequently used data structures in Python programming due to their flexibility and efficiency. They provide a way to access and manipulate data quickly and efficiently, making them an essential tool for developers in various fields.
In this article, we will explore some of the best practices, tips, and tricks for working with Python dictionaries. By the end of this article, you will be equipped with the knowledge to improve your Python dictionary skills and write more efficient and effective code.
2. Python Dictionary Iteration
Python dictionaries are versatile and allow for different ways to iterate over their content.
Here are some tips and tricks on how to iterate over Python dictionaries
2.1 Using for loops to iterate over dictionaries
A for loop can be used to iterate over a dictionary's keys and then access the corresponding values.
For example
my_dict = {'a': 1, 'b': 2, 'c': 3}
for key in my_dict:
print(key, my_dict[key])
# Output
a 1
b 2
c 3
2.2 Using dictionary comprehension to iterate over dictionaries
Dictionary comprehension is a concise way to create a new dictionary based on an existing one. It can also be used to iterate over a dictionary and filter its items.
For example
my_dict = {'a': 1, 'b': 2, 'c': 3}
new_dict = {key: value for key, value in my_dict.items() if value % 2 == 0}
print(new_dict)
#Output
{'b': 2}
2.3 Using the items() method to iterate over keys and values
The items()
method returns a view object that contains tuples of the dictionary's (key, value) pairs. It can be used to iterate over both keys and values.
For example
my_dict = {'a': 1, 'b': 2, 'c': 3}
for key, value in my_dict.items():
print(key, value)
# Output
a 1
b 2
c 3
2.4 Using the keys() method to iterate over keys
The keys()
method returns a view object that contains the dictionary's keys. It can be used to iterate over only the keys.
For example
my_dict = {'a': 1, 'b': 2, 'c': 3}
for key in my_dict.keys():
print(key)
# Output
a
b
c
2.5 Using the values() method to iterate over values
The values()
method returns a view object that contains the dictionary's values. It can be used to iterate over only the values.
For example
my_dict = {'a': 1, 'b': 2, 'c': 3}
for value in my_dict.values():
print(value)
# Output
1
2
3
2.6 Using the enumerate() function to iterate over dictionaries
The enumerate()
function can be used to iterate over a dictionary and return the index and the corresponding (key, value) pair.
For example
my_dict = {'a': 1, 'b': 2, 'c': 3}
for index, (key, value) in enumerate(my_dict.items()):
print(index, key, value)
# Output
0 a 1
1 b 2
2 c 3
3. Python Dictionary Tricks
Python dictionaries are powerful data structures that come with several built-in methods and tricks that can help you work with them more effectively. Here are some of the most useful tricks for working with Python dictionaries
3.1 Merge Dictionaries using the update() Method
If you have two dictionaries with overlapping keys and you want to merge them, you can use the update()
method. This method takes another dictionary as an argument and adds its key-value pairs to the original dictionary. If a key already exists in the original dictionary, its value is updated with the value from the new dictionary.
For example
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
dict1.update(dict2)
print(dict1)
# Output
{'a': 1, 'b': 3, 'c': 4}
3.2 Use the get() Method to Handle Missing Keys
If you try to access a key that doesn't exist in a dictionary, you'll get a KeyError
exception. To avoid this, you can use the get()
method, which returns the value for a given key if it exists in the dictionary, and a default value if it doesn't.
For example
my_dict = {'a': 1, 'b': 2}
print(my_dict.get('c', 0))
# Output
0
In this example, the get()
method returns 0 because the key 'c'
doesn't exist in the dictionary.
3.3 Set Default Values with the setdefault() Method
The setdefault()
method is similar to the get()
method, but it also sets a default value for a key if it doesn't already exist in the dictionary.
For example
my_dict = {'a': 1, 'b': 2}
my_dict.setdefault('c', 0)
print(my_dict)
# Output
{'a': 1, 'b': 2, 'c': 0}
In this example, the setdefault()
method sets the value of the key 'c'
to 0 because it doesn't already exist in the dictionary.
3.4 Remove Duplicates with dict.fromkeys()
If you have a list with duplicate values and you want to remove them, you can use the dict.fromkeys()
method. This method takes a list as an argument and returns a dictionary with the list values as keys and None
as the default value.
For example
my_list = [1, 2, 2, 3, 3, 3]
my_dict = dict.fromkeys(my_list)
print(my_dict)
# Output
{1: None, 2: None, 3: None}
In this example, the dict.fromkeys()
method returns a dictionary with the unique values from my_list
.
3.5 Convert Two Lists into a Dictionary with dict()
If you have two lists of equal length and you want to combine them into a dictionary, you can use the dict()
constructor with the zip()
function. The zip()
function creates an iterator that aggregates elements from each of the lists.
For example
keys = ['a', 'b', 'c']
values = [1, 2, 3]
my_dict = dict(zip(keys, values))
print(my_dict)
# Output
{'a': 1, 'b': 2, 'c': 3}
In this example, the zip()
function creates an iterator that aggregates the elements from keys
The iterator is then passed to the dict() function, which converts it into a dictionary. The resulting dictionary has keys from the keys list and values from the values list. Here's an example code snippet:
keys = ['name', 'age', 'city']
values = ['Joe', '25', 'New York']
my_dict = dict(zip(keys, values))
print(my_dict)
# Output
{'name': 'Joe', 'age': '25', 'city': 'New York'}
This code creates a dictionary with keys 'name', 'age', and 'city', and values 'Joe', '25', and 'New York', respectively.
3.6 Convert Lists into a Dictionary with Zip
In addition to using the dict()
function to convert two lists into a dictionary, you can also use the zip()
function. The zip()
function takes two or more lists and returns an iterator of tuples, where each tuple contains the corresponding elements from each list.
To convert two lists into a dictionary with zip(), you simply pass the two lists to the zip() function, and then pass the resulting iterator to the dict() function.
For example
keys = ['a', 'b', 'c']
values = [1, 2, 3]
my_dict = dict(zip(keys, values))
print(my_dict)
# Output: {'a': 1, 'b': 2, 'c': 3}
In this example, the zip() function creates an iterator that aggregates the elements from keys and values into tuples. The resulting iterator is then passed to the dict() function, which converts it into a dictionary. The resulting dictionary maps the elements from keys to the corresponding elements from values.
Note that the zip() function returns an iterator of tuples, so you can also use it to create a list of tuples, or to iterate over the tuples directly.
3.7 Check if a Dictionary is Empty
To check if a dictionary is empty, you can use a simple if statement.
For example
my_dict = {}
if not my_dict:
print("The dictionary is empty")
else:
print("The dictionary is not empty")
# Output
The dictionary is empty
This code initializes an empty dictionary, checks if it is empty using an if statement, and prints a message accordingly.
3.8 Sort a Dictionary by Value or Key
You can sort a dictionary by either its values or keys using the sorted() function. By default, the sorted() function sorts a dictionary by its keys. However, you can use the items() method to sort a dictionary by its values. Here are some examples:
my_dict = {'a': 1, 'c': 3, 'b': 2}
# Sort by key
sorted_dict = {k: my_dict[k] for k in sorted(my_dict)}
print(sorted_dict)
# Sort by value
sorted_dict = {k: v for k, v in sorted(my_dict.items(), key=lambda item: item[1])}
print(sorted_dict)
{'a': 1, 'b': 2, 'c': 3}
{'a': 1, 'b': 2, 'c': 3}
In the first example, we sort the dictionary by its keys using the sorted() function. We create a new dictionary with keys sorted in ascending order and their corresponding values.
In the second example, we sort the dictionary by its values using the sorted() function and the lambda function as a key. The lambda function specifies that the sorting should be based on the second element (value) of each item in the dictionary. We create a new dictionary with keys in the same order as the original dictionary and their corresponding values sorted in ascending order.
These are just a few examples of the various tips and tricks you can use with Python dictionaries. By using these techniques, you can write more efficient and elegant code and make the most of the powerful features offered by Python dictionaries.
4. Intermediate-Level Python Dictionary Tips, Tricks, and Shortcuts
Python dictionaries are a powerful data structure used to store and manipulate key-value pairs. They are versatile and easy to use, making them a popular choice for storing and organizing data. In this section, we will cover some intermediate-level tips, tricks, and shortcuts for using Python dictionaries effectively.
4.1 Creating a dictionary using a list of tuples
You can create a dictionary using a list of tuples. Each tuple in the list represents a key-value pair in the dictionary.
For example
my_list = [("apple", 1), ("banana", 2), ("orange", 3)]
my_dict = dict(my_list)
print(my_dict)
# Output
{'apple': 1, 'banana': 2, 'orange': 3}
4.2 Using defaultdict for default values
The defaultdict class from the collections module provides a convenient way to set default values for dictionary keys. If a key is not found in the dictionary, defaultdict will return the default value instead of raising a KeyError.
For example
from collections import defaultdict
my_dict = defaultdict(int)
my_dict["apple"] = 1
my_dict["banana"] = 2
print(my_dict["orange"])
# Output
0
In this example, we set the default value to 0 using the int() function. If we try to access the value of a key that does not exist in the dictionary, the default value of 0 will be returned instead of raising a KeyError.
4.3 Using Counter to count items in a dictionary
The Counter class from the collections module provides an easy way to count the frequency of items in a dictionary.
For example
from collections import Counter
my_list = ['apple','red','apple','red','red','pear']
my_counter = Counter(my_list)
print(my_counter)
# Output
Counter({'red': 3, 'apple': 2, 'pear': 1})
In this example, we create a Counter
object from the list my_list
. The Counter object counts the frequency of each item in the list and returns a new dictionary where the keys are the original dictionary keys and the values are the counts.
4.4 Using sorted() to sort a dictionary by value or key
You can use the sorted()
function to sort a dictionary by value or key.
For example
my_dict = {"apple": 1, "banana": 2, "orange": 3}
sorted_dict = sorted(my_dict.items(), key=lambda x: x[1]) # sort by value
print(sorted_dict)
# Output
[('apple', 1), ('banana', 2), ('orange', 3)]
In this example, we sort the dictionary by value using a lambda function as the key argument. The lambda function returns the value of each key-value pair, which is used for sorting. To sort by key, we can simply omit the lambda function.
4.5 Using max() and min() to find the maximum and minimum values in a dictionary
You can use the max()
and min()
functions to find the maximum and minimum values in a dictionary.
For example
my_dict = {"apple": 1, "banana": 2, "orange": 3}
max_value = max(my_dict.values())
min_value = min(my_dict.values())
print(max_value, min_value)
# Output
3 1
In this example, we find the maximum and minimum values in the dictionary using the max() and min() functions, respectively.
4.6 Merging dictionaries with the ** operator
Python provides an easy way to merge dictionaries using the ** operator
. This operator allows you to combine two or more dictionaries into a single dictionary. The ** operator
is also known as the "unpacking" operator because it unpacks the contents of a dictionary.
Here is an example of how to merge two dictionaries using the ** operator:
dict1 = {'a': 1, 'b': 2}
dict2 = {'c': 3, 'd': 4}
merged_dict = {**dict1, **dict2}
print(merged_dict)
# Output
{'a': 1, 'b': 2, 'c': 3, 'd': 4}
In this example, the ** operator is used to merge `dict1` and `dict2` into `merged_dict`.
4.7 Removing duplicates from a dictionary using dict.fromkeys()
Sometimes, you may have a dictionary with duplicate values and want to remove them. You can use the dict.fromkeys()
method to remove duplicates from a dictionary.
Here is an example of how to remove duplicates from a dictionary using the dict.fromkeys()
method:
d = {'a': 1, 'b': 2, 'c': 1}
deduped_dict = dict.fromkeys(d)
print(deduped_dict)
# Output
{'a': None, 'b': None, 'c': None}
In this example, dict.fromkeys()
is used to create a new dictionary deduped_dict with the keys of the original dictionary d
and the values set to None
.
4.8 Using copy() to create a copy of a dictionary
If you want to create a copy of a dictionary, you can use the copy()
method. This method returns a new dictionary with the same key-value pairs as the original dictionary.
Here is an example of how to use the copy()
method to create a copy of a dictionary:
original_dict = {'a': 1, 'b': 2}
copied_dict = original_dict.copy()
print(copied_dict)
# Output
{'a': 1, 'b': 2}
In this example, the copy()
method is used to create a copy of original_dict
and assign it to copied_dict
.
4.9 Using update() to add key-value pairs to a dictionary
The update()
method is used to add key-value pairs to a dictionary. You can pass a dictionary or an iterable of key-value pairs to the update()
method to add them to the dictionary.
Here is an example of how to use the update() method to add key-value pairs to a dictionary:
d = {'a': 1, 'b': 2}
d.update({'c': 3})
print(d)
# Output
{'a': 1, 'b': 2, 'c': 3}
In this example, the update()
method is used to add the key-value pair {'c': 3}
to the dictionary d
.
4.10 Using pop() to remove key-value pairs from a dictionary
The pop()
method removes and returns the value for a given key from a dictionary. If the key is not found in the dictionary, an optional default value can be provided.
dictionary.pop(key[, default])
- key: Key to be searched and deleted from the dictionary
- default: Value to be returned if the key is not found in the dictionary. If not specified and the key is not found, it raises a KeyError
Example
my_dict = {'a': 1, 'b': 2, 'c': 3}
value = my_dict.pop('b')
print(value) 2
print(my_dict)
# Output
{'a': 1, 'c': 3}
In the above example, the pop()
method removes the key 'b'
and returns its value 2. The updated dictionary contains only the remaining key-value pairs.
It's important to note that using pop() on an empty dictionary raises a KeyError
, so it's a good idea to check if the dictionary is empty before calling the method.
my_dict = {}
value = my_dict.pop('b', 'default_value')
print(value)
# Output
'default_value'
In this example, since the dictionary is empty, the pop() method returns the default value 'default_value'.
5. Tips to Write Better Python Code with Dictionaries
Python dictionaries are powerful and versatile data structures that can help you write efficient and readable code. Here are some tips to help you use dictionaries effectively and write better Python code:
5.1 Use List Comprehensions Instead of Loops
List comprehensions are a concise and efficient way to create lists and dictionaries in Python. They are faster and more readable than traditional for loops, and can help simplify your code.
For example, instead of writing:
squares = {}
for i in range(10):
squares[i] = i ** 2
You can use a list comprehension to achieve the same result with less code:
squares = {i: i ** 2 for i in range(10)}
5.2 Use Built-In Functions to Simplify Your Code
Python has many built-in functions that can help you write simpler and more readable code. For example, the sum() function can be used to calculate the sum of values in a dictionary:
my_dict = {'a': 1, 'b': 2, 'c': 3}
total = sum(my_dict.values())
5.3 Avoid Overusing Dictionaries
One common mistake when working with dictionaries is overusing them. While dictionaries can be powerful tools, they can also be overcomplicated and unnecessary for simple tasks.
For example, if you only need to store a small amount of data, it may be more efficient to use variables instead of dictionaries.
# Instead of using a dictionary to store variables, simply define them
name = "Joe"
age = 25
gender = "Male"
5.4 Use Descriptive Variable Names
Descriptive variable names can make your code much easier to understand and maintain. When working with dictionaries, it's important to use clear and descriptive keys to make the data more accessible.
For example
# Using descriptive keys to store data in a dictionary
person = {"name": "Joe", "age": 25, "gender": "Male"}
5.5 Don't Repeat Yourself (DRY) Principle
The Don't Repeat Yourself (DRY) principle is a programming concept that emphasizes code reuse and reducing redundancy. When working with dictionaries, it's important to follow this principle by avoiding duplicating code or repeating yourself.
# Duplicating code in a dictionary
person1 = {"name": "Joe", "age": 25, "gender": "Male"}
person2 = {"name": "Jane", "age": 30, "gender": "Female"}
# Using a dictionary to avoid code duplication
person_data = {
"Joe": {"age": 25, "gender": "Male"},
"Jane": {"age": 30, "gender": "Female"}
}
5.6 Use the Correct Data Type for Your Data
Using the correct data type for your data can make your code more efficient and reduce errors. For example, if you're working with numerical data, it's important to use integers or floats instead of strings.
# Using the correct data type for numerical data
numbers = {"one": 1, "two": 2, "three": 3}
5.7 Use a Consistent Style Guide
Using a consistent style guide can make your code more readable and maintainable. When working with dictionaries, it's important to follow a consistent style guide for key naming and formatting. For example, you could use camel case for key names and indent your code consistently.
# Using a consistent style guide for dictionary keys
person = {"firstName": "Joe", "lastName": "Vu", "age": 25}
5.8 Write Readable Code
Writing readable code is essential for collaboration and maintenance. When working with dictionaries, it's important to make your code as clear and concise as possible. This can be achieved by using comments, whitespace, and descriptive variable names.
# Adding comments and whitespace to improve code readability
person = {
# Personal Information
"firstName": "Joe",
"lastName": "Vu",
"age": 25,
# Contact Information
"email": "[email protected]",
"phone": "0987-0987-0987"
}
6. Conclusion
In conclusion, Python dictionaries are a crucial data structure that every programmer must master to become proficient in Python. In this article, we have covered various tips, tricks, and best practices for working with Python dictionaries, including iteration, merging, default values, sorting, and removing duplicates. We have also emphasized the importance of using descriptive variable names, avoiding overusing dictionaries, and following consistent style guides to write better Python code.
By mastering these tips and continuously learning and practicing, you can become more efficient and effective in your coding. Dictionaries are an essential part of many Python applications and can greatly improve the speed and functionality of your code. We hope that this article has provided you with valuable insights and resources to improve your Python programming skills.
References
- https://python.plainenglish.io/16-intermediate-level-python-dictionary-tips-tricks-and-shortcuts-1376859e1adc
- https://realpython.com/courses/python-dictionary-iteration/
- https://dev.to/byteslash/15-python-tips-and-tricks-every-beginner-should-know-3bm5
- https://www.inspiredpython.com/tip/python-dictionary-tricks-keys-values-and-items-are-dynamic
- https://richard-quinn.com/16-intermediate-level-python-dictionary-tips-tricks-and-shortcuts.html