Python Iterators: What you must know?
By JoeVu, at: Oct. 27, 2023, 4:56 p.m.
Estimated Reading Time: __READING_TIME__ minutes

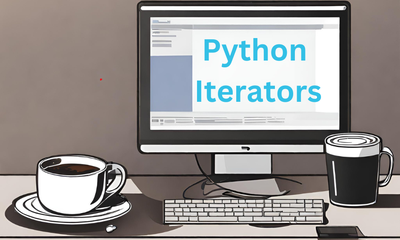
1. What is an Iterator?
In Python, an iterator is an object that represents a sequence of data. It allows you to traverse through a collection of items, such as a list, tuple, dictionary, or custom data structure, one element at a time. Iterators implement two essential methods: __iter__()
and __next__()
. The __iter__()
method initializes the iterator, and __next__()
retrieves the next item in the sequence. When there are no more items to return, __next__()
raises the StopIteration
exception.
2. Why are Iterators Important?
Iterators are fundamental to Python and play a crucial role in simplifying and optimizing data traversal and manipulation. They offer several key advantages:
- Efficiency: Iterators load and process data one element at a time, which is memory-efficient and suitable for large datasets.
- Lazy Evaluation: They support lazy evaluation, meaning elements are generated only when requested, which can save time and resources.
- Generalization: Iterators generalize the concept of data traversal, making it easier to work with diverse data structures in a uniform way.
- Clean Code: Using iterators often leads to cleaner and more readable code, reducing the complexity of loops and data processing.
One well-known built-in function that has changed from a list to an iterator is range
. In Python 2, range returns a list, while it returns an iterator in Python 3.
3. How to Use Iterators
To use iterators in Python, you can follow these steps:
- Create a class that defines the iterator, implementing the
__iter__()
and__next__()
methods. - In the
__iter__()
method, initialize any necessary variables and returnself
. - In the
__next__()
method, calculate and return the next item in the sequence. If there are no more items, raiseStopIteration
.
You can also use built-in iterators like iter()
and next()
with iterable objects like lists, dictionaries, or custom objects.
# Custom Iterator Example
class SquareNumbers:
def __init__(self, max_val):
self.max_val = max_val
self.current = 0
def __iter__(self):
return self
def __next__(self):
if self.current < self.max_val:
result = self.current * self.current
self.current += 1
return result
else:
raise StopIteration
my_iter = SquareNumbers(5)
for num in my_iter:
print(num) # 0, 1, 4, 9, 16
4. Issues with Iterators
While iterators offer efficiency benefits, they can introduce performance issues if not used wisely:
- Inefficient Data Structures: If your custom data structure used for iteration is not well-designed, accessing elements may be slow.
- Inefficient __next__() Implementation: Poorly optimized
__next__()
methods can lead to slow iteration. - Large Memory Consumption: When working with very large datasets, iterator state and memory usage may become a concern.
- One-Time Use: Iterators are generally one-time-use objects. Once an iterator reaches the end of the sequence, you cannot rewind it, and you need to create a new iterator.
- Concurrency: Using iterators in multithreaded or multiprocessing environments can lead to race conditions if not synchronized properly.
- Compatibility: Not all data structures can be iterated over with built-in iterators. Custom implementations may be required.
5. Libraries that Use Iterators Extensively for Performance
Several Python libraries make extensive use of iterators for performance optimization:
NumPy: NumPy uses iterators for array manipulation and data processing, providing efficient and vectorized operations.
Pandas: Pandas leverages iterators to process large datasets efficiently, and it provides DataFrame and Series iterators.
itertools: The itertools
module in Python's standard library provides a collection of fast, memory-efficient tools for working with iterators and iterables.
By understanding iterators and their benefits, you can write more efficient and Pythonic code, especially when dealing with large datasets or complex data manipulation tasks.
Another interesting topic is Generator - which is similar but not the same as Iterator