Python Performance Optimization: Techniques and Best Practices
By JoeVu, at: May 28, 2023, 3:56 p.m.
Estimated Reading Time: __READING_TIME__ minutes

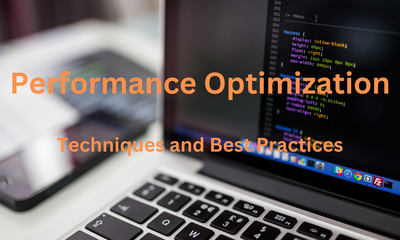
Introduction
Python is known for its simplicity and readability, but it can sometimes suffer from performance issues. This is mainly due to the inexperienced or wrong understanding of Python behaviors. In this article, we will explore common performance problems in Python and discuss various techniques and best practices to optimize your Python code. By understanding these problems and implementing the suggested solutions, you can significantly improve the performance of your Python applications.
1. Problem: Inefficient Looping
Lets consider the example below
numbers = [1, 2, 3, 4, 5]
result = 0
for num in numbers:
result += num
Solution: Use List Comprehension or Generator Expressions
numbers = [1, 2, 3, 4, 5]
result = sum(numbers)
Pros
- List comprehension or generator expressions can perform looping operations more efficiently.
- They eliminate the need for creating temporary lists, resulting in reduced memory consumption.
- The concise syntax enhances code readability.
Cons
- List comprehensions can become complex and reduce code clarity if used excessively.
- Generator expressions may not be suitable if the order of elements is crucial.
Few more examples are listed below
Example 2: Redundant calculation
numbers = [1, 2, 3, 4, 5]
total = 0
for num in numbers:
total += num * 2 # Redundant multiplication by 2
Solution: Perform the calculation outside the loop if it does not depend on the loop variable.
# Solution 1: Move calculation outside the loop
numbers = [1, 2, 3, 4, 5]
total = sum(numbers) * 2
# Solution 2: Use List Comprehensive
numbers = [1, 2, 3, 4, 5]
total = sum([number * 2 for number in numbers])
2. Problem: Excessive String Concatenation
Excessive string concatenation refers to the inefficient practice of repeatedly concatenating strings using the +
operator or +=
operator. This can lead to poor performance and unnecessary memory allocation, especially when dealing with large strings or within loops.
The problem with excessive string concatenation arises because strings in Python are immutable, meaning they cannot be modified in place. When concatenating strings using the +
operator or +=
operator, a new string object is created every time, leading to extra memory allocation and copying.
Lets consider the example below
result = ""
for i in range(1000):
result += str(i)
Solution: Use Join or String Formatting
result = ''.join(str(i) for i in range(1000))
Pros
- The
join
method or string formatting using placeholders(`%s` or `{}`)
are more efficient for concatenating strings. - They reduce the number of string copies, leading to improved performance.
Cons
- String formatting may be less readable if used excessively or in complex scenarios.
- Few more examples are listed below
Example 2: Excessive string concatenation in URL construction
base_url = "https://example.com/api/data?"
parameters = {'param1': 'value1', 'param2': 'value2', ...}
url = base_url
for key, value in parameters.items():
url += key + '=' + value + '&'
Solution 2: Using urllib.parse.urlencode()
from urllib.parse import urlencode
base_url = "https://example.com/api/data?"
parameters = {'param1': 'value1', 'param2': 'value2', ...}
url = base_url + urlencode(parameters)
Example 3: Excessive string concatenation in CSV generation
data = [['Name', 'Age', 'Country'], ['John', '25', 'USA'], ...]
csv_content = ""
for row in data:
csv_content += ','.join(row) + '\n'
Solution 3: Using the csv module
import csv
from io import StringIO
data = [['Name', 'Age', 'Country'], ['John', '25', 'USA'], ...]
csv_content = StringIO()
csv_writer = csv.writer(csv_content)
csv_writer.writerows(data)
csv_content.seek(0)
csv_string = csv_content.getvalue()
Example 4: Excessive string concatenation in SQL query construction
query = "SELECT * FROM users WHERE"
filters = {'age': 25, 'country': 'USA', ...}
for field, value in filters.items():
query += f" {field}='{value}' AND"
query = query.rstrip(' AND')
Solution 4: Using parameterized queries
import sqlite3
query = "SELECT * FROM users WHERE"
filters = {'age': 25, 'country': 'USA', ...}
placeholders = " AND ".join(f"{field} = ?" for field in filters)
values = tuple(filters.values())
full_query = f"{query} {placeholders}"
conn = sqlite3.connect('database.db')
cursor = conn.cursor()
result = cursor.execute(full_query, values).fetchall()
3. Problem: Inefficient File Reading
Inefficient file reading refers to suboptimal practices when reading files that can lead to poor performance and inefficient resource utilization. This can include issues like reading files line by line using a loop, performing excessive I/O operations, or reading the entire file into memory unnecessarily.
Lets consider the example below
lines = []
with open('data.txt', 'r') as file:
for line in file:
lines.append(line)
Solution: Use File Iteration
with open('data.txt', 'r') as file:
lines = list(file)
Pros
- Iterating over a file object directly avoids unnecessary memory consumption.
- It improves performance by reading the file incrementally.
Cons
- File iteration may not be suitable if you need random access to lines or perform complex operations on the file.
Few more examples are listed below
Example 2: Inefficient file reading with excessive I/O operations
file_path = 'data.txt'
with open(file_path, 'r') as file:
lines = file.readlines()
for line in lines:
# Perform multiple I/O operations for each line
# ...
Solution 2: Minimize I/O operations
file_path = 'data.txt'
with open(file_path, 'r') as file:
lines = file.readlines()
# Process the data outside the file context
for line in lines:
# Process each line
# ...
Example 3: Inefficient file reading by reading the entire file into memory unnecessarily
file_path = 'large_data.txt'
with open(file_path, 'r') as file:
file_content = file.read()
# Process the entire file content
Solution 3: Use file iteration or read in chunks
file_path = 'large_data.txt'
with open(file_path, 'r') as file:
for line in file:
# Process each line incrementally
# ...
# or
with open(file_path, 'r') as file:
chunk_size = 4096 # Adjust the chunk size as per your requirements
while True:
chunk = file.read(chunk_size)
if not chunk:
break
# Process each chunk
# ...
4. Problem: Costly Regular Expressions
Costly regular expressions in Python refer to the inefficient usage of regular expressions that can result in poor performance and excessive resource consumption. This can occur due to inefficient pattern matching, excessive backtracking, or unnecessary compilation of regular expressions. In this response, I will discuss the issues related to costly regular expressions, provide examples to illustrate the problem, and suggest solutions with code snippets.
Lets consider the example below
import re
data = ['apple', 'banana', 'cherry']
results = []
for item in data:
if re.match(r'a', item):
results.append(item)
Solution: Precompile Regular Expressions
import re
pattern = re.compile(r'a')
data = ['apple', 'banana', 'cherry']
results = [item for item in data if pattern.match(item)]
Pros
- Precompiling regular expressions improves performance by avoiding redundant compilation in each iteration.
- It provides a significant speed boost when using the same pattern multiple times.
Cons
- Precompiling regular expressions may add some initial overhead if the pattern is used infrequently or changes dynamically.
Few more examples are listed below
Example 2: Costly regular expression with unnecessary capturing groups
import re
text = "Hello, world!"
pattern = "(Hello), (world)!"
match = re.match(pattern, text)
Solution 2: Use non-capturing groups or remove capturing groups
pattern = r"Hello, (?:world)!"
match = re.match(pattern, text)
Example 3: Costly regular expression with redundant compilation
import re
text = "The quick brown fox jumps over the lazy dog"
pattern = r"fox"
for _ in range(1000):
match = re.match(pattern, text)
Solution 3: Compile the regular expression once and reuse it
import re
text = "The quick brown fox jumps over the lazy dog"
pattern = re.compile(r"fox")
for _ in range(1000):
match = pattern.match(text)
Conclusion
Optimizing Python performance is crucial for achieving faster and more efficient code execution. By addressing common problems like inefficient looping, excessive string concatenation, inefficient file reading, and costly regular expressions, you can significantly improve the performance of your Python applications. However, it's important to consider the pros and cons of each solution to ensure they align with your specific use case.
Remember, optimizing performance should always be balanced with code readability and maintainability.
Being master of Python Performance Optimization is a must for every senior Python developer.