Python Pytest Introduction
By JoeVu, at: April 1, 2023, 10:22 p.m.
Estimated Reading Time: __READING_TIME__ minutes

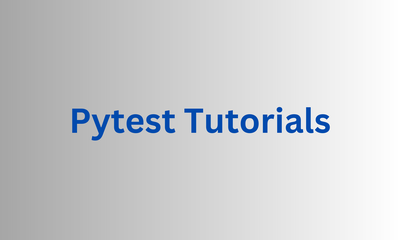
1. Introduction
Testing is an integral part of software development, and choosing the right testing framework is critical to the success of any software project. One of the most popular testing frameworks for Python is Pytest. Pytest is a mature and feature-rich testing framework that allows developers to write clear, maintainable, and scalable tests. In this article, we will provide a comprehensive introduction to Pytest and its features.
2. Installing Pytest
Before we can start writing tests with Pytest, we need to install it. Pytest requires Python 3.6 or later and can be installed using pip, Python's package manager. Here are the steps to install Pytest:
- Open a terminal or command prompt.
- Create a virtual environment for your project (optional but recommended).
- Run the command
pip install pytest
. - Once Pytest is installed, we can verify it by running the command
pytest --version
.
3. Writing Pytest Tests
Pytest allows us to write tests in a simple, easy-to-read syntax. Pytest tests are Python functions that start with the prefix "test_".
Here's an example of a basic Pytest test:
def test_addition():
assert 2 + 2 == 4
3.1 Pytest Fixtures
Pytest tests can also use fixtures, which are functions that provide test data and set up the testing environment.
Here's an example of a Pytest fixture:
import pytest
@pytest.fixture
def my_fixture():
return "Hello, world!"
def test_fixture(my_fixture):
assert my_fixture == "Hello, world!"
Pros:
- Easy and intuitive way to set up test data and the testing environment.
- Can be reused across multiple tests, reducing duplication of code.
- Can be composed and nested to build more complex test environments.
- Can be parameterized to generate different test data for different test cases.
Cons:
- Fixtures can add additional complexity to the test code.
- Fixtures can slow down test execution time, especially if they perform expensive setup operations.
3.2 Pytest Parameterized
Pytest also supports parameterized tests, where we can run the same test with different input values.
Here's an example:
import pytest
@pytest.mark.parametrize("input1, input2, expected_output", [
(1, 2, 3),
(4, 5, 9),
(-1, 1, 0),
])
def test_addition(input1, input2, expected_output):
assert input1 + input2 == expected_output
Pros:
- Allows us to test the same behavior with different input values, reducing the amount of code we need to write.
- Can be used to test edge cases and boundary conditions.
- Can help ensure that code is properly handling a range of input values.
- Can be used to generate test cases automatically.
Cons:
- Parameterized tests can add additional complexity to the test code.
- Parameterized tests can be more difficult to read and understand than regular tests.
3.3 Pytest assertions
Pytest provides a rich set of assertions that make it easy to test for expected behavior.
Here are some examples:
assert 2 + 2 == 4
assert [1, 2, 3] == [1, 2, 3]
assert "hello".startswith("h")
Pros:
- Allows us to group tests by category, making it easier to find and run specific tests.
- Can be used to apply configuration options to groups of tests.
- Can be used to skip tests that are known to be failing or not applicable to certain configurations.
Cons:
- Overuse of markers can make the test code harder to understand and maintain.
- Tags may be less useful for small projects with few tests.
3.4 Markers/Tags
Marking a test with a specific tag allows you to easily run only tests that are marked with that tag. This can be useful when you want to run only a specific subset of tests, for example, when you're debugging a specific feature.
To mark a test with a tag, you can use the @pytest.mark decorator followed by the name of the tag.
Here's an example:
import pytest
@pytest.mark.slow
def test_my_slow_test():
# test code here
pass
@pytest.mark.fast
def test_my_fast_test():
# test code here
pass
In the example above, we've marked two tests with the tags "slow" and "fast". We can now run only the slow tests using the following command:
pytest -m slow
This will only run the test_my_slow_test function.
3.5 Customizing Output
Pytest allows us to customize the output of test results to suit our needs. This can be useful when we want to display additional information, or when we want to use a custom format for our test results.
Here's an example of customizing the output to show the full error message for failed tests:
# conftest.py
def pytest_assertrepr_compare(op, left, right):
if isinstance(left, str) and isinstance(right, str) and op == "==":
return [
f"Comparing strings:\n{left}\nand\n{right}\n",
"Strings are different."
]
In the example above, we've defined a function that will be called when an assertion fails. The function takes the operator used in the assertion (op), the left and right values being compared (left and right), and returns a list of strings representing the error message.
Pros:
- Allows us to customize the format and style of test output, making it easier to read and understand.
- Can be used to generate custom reports or metrics based on test results.
Cons:
- Customizing output can add additional complexity to the test code.
- Customizing output may not be necessary for all projects or use cases.
4. Running Pytest Tests
Once we've written our Pytest tests, we can run them using the pytest command. By default, Pytest looks for files and directories that match the pattern test_*.py in the current directory and its subdirectories. Here are some examples of running Pytest tests:
# Run all tests in the current directory
pytest
# Run all tests in a specific file
pytest test_myfile.py
# Run a specific test
pytest test_myfile.py::test_addition
# Run tests in verbose mode
pytest -v
Pytest also provides many options for filtering tests, customizing output, and integrating with other tools.
5. Pytest Plugins
Pytest has a large ecosystem of plugins that provide additional functionality and integrations with other tools. Some popular Pytest plugins include:
- pytest-cov: generates code coverage reports
- pytest-html: generates HTML reports
- pytest-xdist: runs tests in parallel
- pytest-django: adds support for testing Django applications
- pytest-selenium: adds support for testing web applications using Selenium
To use a Pytest plugin, we need to install it using pip and then include it in our Pytest configuration file.
For example:
pip install pytest-cov
pytest --cov=my_package tests/
In the example above, we're using the pytest-cov plugin to generate a code coverage report for our my_package module. The --cov option tells Pytest to include coverage information in the test output, and the tests/ argument specifies the directory where our test files are located.
Pros:
- Pytest has a large ecosystem of plugins and integrations with other testing and development tools.
- Can be used to automate tasks such as test execution, code coverage analysis, and test reporting.
- Can be used to integrate with other tools in the development workflow, such as continuous integration systems.
Cons:
- Integrations with other tools may require additional setup and configuration.
- Some plugins may not be actively maintained or may not be compatible with the latest versions of Pytest.
6. Best Practices for Pytest
To write effective Pytest tests, we should follow some best practices that help us write clear, maintainable, and scalable tests.
Here are some recommended conventions for writing Pytest tests:
- Use descriptive test names that explain the expected behavior of the code being tested.
- Use fixtures to set up test data and the testing environment.
- Use parameterized tests to test the same behavior with different input values.
- Use assertions to check for expected behavior.
- Group related tests together in test classes or modules.
- Use pytest markers to group tests by category or to apply configuration options.
- Avoid using global state or mutable objects in tests.
- Mock external dependencies using Pytest-mock or other mocking libraries.
We should also follow some general tips for organizing our test code:
- Keep test code separate from production code.
- Organize test code into a separate directory structure.
- Use source control to track changes to test code.
- Write tests that cover all aspects of the code being tested.
- Write tests that check edge cases and error conditions.
- Update tests when code changes.
Finally, we should be aware of some common pitfalls when writing Pytest tests:
- Writing tests that are too complex or tightly coupled to implementation details.
- Using fixtures or mocking inappropriately.
- Neglecting to write tests for error conditions.
- Writing tests that are too slow or flaky.
- Failing to update tests when code changes.
7. Conclusion
Pytest is a powerful and flexible testing framework for Python that can help us write effective tests quickly and easily. By following the best practices and tips outlined in this article, we can write Pytest tests that are clear, maintainable, and scalable. Pytest's large ecosystem of plugins also provides additional functionality and integrations with other tools. We encourage readers to try Pytest in their own projects and see how it can improve their testing workflow.
8. References
- "Getting started with Pytest" by Real Python
- "Pytest: A Mature Python Testing Tool" by Testim Blog
- "An Introduction to Pytest" by Mouse vs Python
Hints/Tips/Tricks for Learning Pytest in a Few Weeks:
- Start with small, simple tests and gradually build up to more complex tests.
- Use the Pytest documentation and online resources to learn about Pytest's features and best practices.
- Practice writing tests for different types of code, including functions, classes, and modules.
- Experiment with Pytest's command-line options and plugins to see how they can improve your testing workflow.
- Work on a sample project or contribute to an open-source project to gain real-world experience with Pytest.
- Seek feedback from other developers and incorporate their suggestions into your testing process.
- Keep up-to-date with the latest developments in Pytest and testing best practices to stay ahead of the curve.
9. Exercises
- Write a test for a function that returns the sum of two integers.
- Write a test for a function that raises an exception when given an empty list as input.
- Write a test for a function that sorts a list of integers in ascending order.
- Write a test for a function that calculates the factorial of a positive integer.
- Write a test for a function that converts a temperature from Celsius to Fahrenheit.
- Write a fixture that sets up a database connection and closes it after each test.
- Write a test that checks if a function runs in a certain amount of time.
- Write a test that checks if a function raises a warning.
Solutions can be found here