Python Scrapers: A Guide to Web Scraping with Python
By JoeVu, at: March 10, 2023, 9:53 p.m.
Estimated Reading Time: __READING_TIME__ minutes

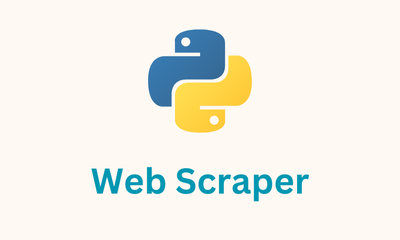
Web scraping is the process of extracting data from websites automatically. It's a useful skill for anyone who wants to gather large amounts of data quickly and efficiently. Python is a popular programming language for web scraping due to its versatility and a vast range of available libraries. In this article, we'll discuss different types of Python scrapers, along with their pros and cons.
1. Requests & Beautiful Soup Scraper
One of the simplest ways to scrape a website is by using the Requests and Beautiful Soup libraries in Python. Requests is a library that allows you to send HTTP requests in Python, while Beautiful Soup is a library for pulling data out of HTML and XML files. Here's an example of how to use Requests and Beautiful Soup to scrape a website:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
for link in soup.find_all('a'):
print(link.get('href'))
In this example, we simple use 2 libraries BeautifulSoup and Requests to download the website content and extract all hyperlinks in this website.
Pros
- Easy to use and quick to set up
- Handles basic HTML parsing well
- Ideal for smaller and simpler websites
Cons
- Requires knowledge of HTML and CSS
- May encounter issues with websites that use JavaScript or dynamic content (ex: Walmart.com, HomeDepot.com)
- Not scalable for larger websites or frequent scraping
You can find more detail in here
Sample code is in our github repo
2. Scrapy
Scrapy is an open-source and powerful Python framework for web scraping. It provides an integrated way to extract structured data from websites and supports various features such as XPath selectors, middleware, and item pipelines. Here's an example of how to use Scrapy to scrape a website:
ruby
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['https://example.com']
def parse(self, response):
for link in response.css('a::attr(href)').getall():
yield {
'link': link
}
We can simply run the spider above by scrapy crawl example
Pros
- Ideal for more complex websites and large-scale projects
- Provides robust scraping functionalities and tools
- Has built-in support for handling JavaScript and dynamic content
Cons
- Steeper learning curve than Requests and Beautiful Soup
- Requires understanding of XPath selectors and Scrapy architecture
- May require customization and configuration to handle more challenging scraping tasks.
You can find more details about this article: scrapers using scrapy
3. Selenium WebDriver Scraper
Selenium WebDriver is a browser automation tool used for web scraping, which allows you to control a browser programmatically. With the help of the WebDriver, you can interact with a website just like a user would. Selenium WebDriver is ideal for websites that have complex interactions and dynamic content. Here's an example of how to use Selenium WebDriver to scrape a website:
from selenium import webdriver
url = 'https://example.com'
browser = webdriver.Chrome()
browser.get(url)
for link in browser.find_elements_by_tag_name('a'):
print(link.get_attribute('href'))
browser.quit()
When we run this code snippet, an actual browser will be opened and navigate to ths site url https://example.com to collect all sub links.
Pros
- Allows you to scrape websites that are highly interactive and require user interactions.
- Provides access to more extensive sets of data and interactions.
- Handles JavaScript and dynamic content well.
Cons
- Can be slower than other scraping methods since it requires running a browser
- Requires more resources in terms of system memory and CPU usage.
- Can be prone to detection and blockage by anti-scraping measures.
More detail is explained here
4. PlayWright Scraper
Playwright is an open-source library that provides a high-level API for automating browsers. It can be used with Python through the Pyppeteer or Playwright Python libraries. Playwright is ideal for scraping modern web applications that use complex front-end frameworks, such as React or Angular. Here's an example of how to use Playwright Python to scrape a website:
from playwright.sync_api import Playwright, BrowserType
with Playwright() as playwright:
chromium = playwright.chromium
browser = chromium.launch(headless=True)
page = browser.new_page()
page.goto('https://example.com')
links = page.query_selector_all('a')
for link in links:
print(link.get_attribute('href'))
browser.close()
Similar to Selenium, PlayWright will open a new browser and navigate just like a human.
Pros
- Can handle complex front-end frameworks and JavaScript-heavy websites
- Provides access to multiple browser engines (Chromium, Firefox, and WebKit)
- Has built-in support for parallel execution and resource optimization.
Cons
- Requires knowledge of JavaScript and Node.js for more advanced tasks
- May require more system resources than other scraping methods
- Has a steeper learning curve than other Python scraping tools.
You can find more detail in here
5. Ethical concerns
Web scraping has become a popular technique for collecting data from websites. However, scraping websites without permission can raise ethical concerns. Here are some key considerations to keep in mind when scraping data from websites:
- Respect website terms of use: Most websites have a terms of use agreement that outlines acceptable uses of their website. Be sure to read and follow these terms before scraping any data.
- Do not cause harm: Scraping can put a strain on website resources, so it's essential to be mindful of the potential impact on the website's performance. Avoid scraping at high rates or with excessive frequency, as this can cause harm to the website.
- Do not scrape personal or sensitive information: Scraping personal information, such as login credentials, credit card information, or other sensitive data, is illegal and unethical. Be sure to only scrape publicly available data.
- Provide attribution: If you are using scraped data in research, reporting, or other public-facing materials, be sure to provide attribution to the source of the data.
- Obtain consent: In some cases, it may be appropriate to obtain consent from website owners before scraping data. This is especially true for websites that require login credentials or other forms of access.
In summary, ethical web scraping involves respecting website terms of use, avoiding harm, not scraping personal or sensitive information, providing attribution, and obtaining consent when necessary. By following these guidelines, you can ensure that your web scraping practices are ethical and legal.
The article discusses various Python libraries and frameworks for web scraping, including Requests & Beautiful Soup, Scrapy, Selenium WebDriver, and Playwright. It provides examples and highlights the pros and cons of each scraping method. Additionally, the article emphasizes the importance of ethical web scraping, including respecting website terms of use, avoiding harm, not scraping personal or sensitive information, providing attribution, and obtaining consent when necessary.