Testing Flask Applications: Best Practices
By khoanc, at: Oct. 12, 2023, 6:41 p.m.
Estimated Reading Time: __READING_TIME__ minutes

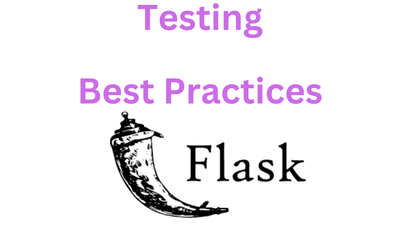
Introduction
Flask, a micro web framework for Python, has rapidly gained popularity among developers for its simplicity and flexibility. Its lightweight and unopinionated nature makes it an ideal choice for building web applications of all sizes. However, as Flask applications grow in complexity, ensuring their reliability becomes paramount. This is where testing comes into play.
Testing is an essential practice that helps identify and rectify issues before they reach the end-users. In the context of evey single web application, testing is crucial to ensure that your web services work as intended, handle requests properly, and remain robust as you make updates and changes.
This article is dedicated to exploring the best practices for testing Flask applications. We'll review various aspects of testing, from unit testing and integration testing to end-to-end testing, to help you build a robust and stable Flask application. By the end of this guide, you'll have a comprehensive understanding of how to create, organize, and execute tests effectively in your Flask projects.
The Importance of Testing
Before we dive into the specifics of testing Flask applications, let's briefly outline why testing is so important:
-
Bug Detection: Testing helps identify and rectify bugs and issues early in the development process. This reduces the likelihood of encountering critical problems in production, which can lead to user dissatisfaction and financial losses.
-
Code Quality: Writing tests forces developers to create well-structured and maintainable code. It promotes the use of best practices and design patterns, leading to cleaner and more manageable codebases.
-
Regression Prevention: As your Flask application evolves, new features and updates can inadvertently introduce regressions - issues that break existing functionality. Testing helps prevent these regressions and ensures that changes do not negatively impact the current codebase.
-
Documentation: Tests serve as a form of documentation for your code. They provide insights into how different components of your application should behave, making it easier for both new and experienced developers to understand the code.
-
Confidence in Deployments: By having a suite of tests that pass consistently, you can deploy your Flask application with confidence, knowing that it's less likely to break in production. This contributes to a smoother and more reliable deployment process.
With the importance of testing established, let's proceed to explore the various types of testing you can employ in your Flask projects.
Types of Testing
In the realm of Flask application testing, there are several different types of testing that serve specific purposes. Understanding these testing categories is crucial for developing a comprehensive testing strategy for your Flask projects. The three primary types of testing you'll encounter are:
1. Unit Testing
Definition and Purpose: Unit testing is the practice of testing individual components or functions of your Flask application in isolation. The goal is to ensure that each unit of code, such as a function or method, performs as expected.
Using Libraries like unittest
and pytest
: Python offers several testing libraries, with unittest
and pytest
being the most common choices. unittest
is part of the Python standard library, while pytest
is a popular third-party testing framework that provides a more concise and user-friendly syntax for writing tests.
Sample Code Examples:
# Using unittest for unit tests
import unittest
def add(a, b):
return a + b
class TestAddition(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add(3, 4), 7)
def test_add_negative_numbers(self):
self.assertEqual(add(-2, -5), -7)
Using Pytest
# Using pytest for unit tests
def test_add_positive_numbers():
assert add(3, 4) == 7
def test_add_negative_numbers():
assert add(-2, -5) == -7
2. Integration Testing
Definition and Purpose: Integration testing focuses on testing the interaction between different components or units of your Flask application. It ensures that these components work together seamlessly and produce the expected results when integrated.
Sample Code Examples:
# An example of Flask integration testing
from yourapp import create_app
def test_homepage_returns_200(client):
app = create_app(test_config)
with app.test_client() as client:
response = client.get('/')
assert response.status_code == 200
def test_login_functionality(client):
app = create_app(test_config)
with app.test_client() as client:
response = client.post('/login', data={'username': 'testuser', 'password': 'password123'})
assert b'Welcome, testuser' in response.data
3. End-to-End (E2E) Testing
Definition and Purpose: End-to-End testing, also known as E2E testing, focuses on testing the entire application flow. It simulates real user interactions with the application, ensuring that all components, from the frontend to the backend, work together harmoniously.
Sample Code Examples:
# An example of E2E testing with Selenium
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
def test_user_registration_and_login():
driver = webdriver.Chrome()
driver.get('http://yourapp.com')
# Perform actions like filling out a registration form and logging in
# Check if the expected user profile page is displayed
assert 'User Profile' in driver.page_source
driver.quit()
Each type of testing plays a crucial role in ensuring the reliability and stability of your Flask application. By utilizing a combination of these testing types, you can thoroughly assess the functionality of your application and catch issues at different levels of complexity.
Setting Up the Testing Environment
Before you can start writing tests for your Flask application, it's essential to set up a dedicated testing environment. A well-structured environment ensures that your tests run reliably and do not interfere with your development or production environments. Here are the key steps to set up your Flask testing environment:
1. Choosing a Testing Framework
Selecting the right testing framework is crucial for efficient testing. Flask applications are commonly tested using two main frameworks:
-
pytest: pytest is a popular third-party testing framework that offers a more straightforward and expressive syntax compared to the built-in
unittest
framework. It provides various plugins, extensive customization options, and strong community support. -
unittest: This is part of Python's standard library, making it readily available for testing. While it's more verbose than pytest, it offers robust testing capabilities and is well-suited for testing complex applications.
-
playwright: If your Flask application involves browser automation testing, especially for web applications with complex front-end interactions, consider using "playwright." Playwright provides a high-level, cross-browser API for performing automated browser tests.
-
selenium: Selenium is another strong contender for browser automation testing. It supports various programming languages and offers extensive browser compatibility.
2. Virtual Environments and Dependencies
Isolating your testing environment from your development environment is crucial to prevent conflicts between dependencies. To set up a virtual environment for your Flask testing, use a tool like virtualenv
. Here's how you can create a virtual environment:
# Create a virtual environment (Python 3.x)
python3 -m venv venv
# Activate the virtual environment
source venv/bin/activate
Once your virtual environment is activated, install the necessary dependencies:
pip install Flask pytest # Install Flask and pytest (or your chosen testing framework)
pip install -r requirements.txt # Install your application's dependencies
pip install playwright # install playwright
playwright install # install web browsers
pip install selenium # install selenium
3. Creating a Test Database
For Flask applications that interact with a database, it's essential to create a separate test database. This ensures that your tests do not affect your production data. You can do this by running database migrations with test-specific configurations. Popular databases like SQLite are commonly used for testing because they create in-memory databases that can be easily discarded after testing.
Everything can be achieved easily if you follow this instruction
4. Configuration and Environment Variables
Your Flask application likely uses configuration variables to determine settings for different environments (e.g., development, testing, production). Make sure to create a separate configuration for testing in your Flask app. This allows you to configure test-specific settings, such as using a test database or disabling certain features that are unnecessary for testing.
# Example of a testing configuration
class TestConfig:
TESTING = True
SQLALCHEMY_DATABASE_URI = 'sqlite:///test_database.db'
DEBUG = False
In your test setup, you can then load the appropriate configuration:
app.config.from_object('config.TestConfig')
By configuring your testing environment thoughtfully, you can ensure that your tests are executed in an isolated and controlled environment, minimizing interference with your development and production environments.
Best Practices in Flask Testing
Writing effective tests is not just about covering code but also ensuring that your tests provide meaningful feedback and are easy to maintain. Here are some best practices to consider when writing tests for your Flask application:
1. Isolation and Independence of Tests
-
Mock External Dependencies: Avoid external dependencies, such as databases, third-party APIs, or services, in your tests. Instead, use mocking libraries like
unittest.mock
orpytest-mock
to simulate the behavior of these dependencies. This keeps your tests fast, isolated, and independent of external factors. -
Using Fixtures (pytest): If you're using pytest, take advantage of fixtures to set up and tear down resources needed for your tests. Fixtures make your test code cleaner and more maintainable by encapsulating setup and teardown logic.
2. Writing Meaningful Test Cases
-
Test Naming Conventions: Follow a clear and consistent naming convention for your tests. This makes it easier to understand the purpose of each test case. For example, prefix test functions with "
test_
" to indicate their role. -
Testing Edge Cases: Ensure your tests cover various scenarios, including edge cases and boundary conditions. Consider situations where unexpected inputs or conditions could lead to errors.
3. Testing API Endpoints
-
Sending Requests and Validating Responses: When testing Flask API endpoints, use libraries like
requests
to send HTTP requests and validate responses. Ensure that the endpoints return the expected status codes, data, and headers. -
Authentication and Authorization Testing: Verify that your endpoints correctly handle authentication and authorization. Test different user roles, including anonymous users, regular users, and administrators, to ensure the application behaves as intended.
4. Testing Database Operations
-
CRUD Operations: For applications with database interactions, test Create, Read, Update, and Delete (CRUD) operations thoroughly. Confirm that data is stored, retrieved, and modified correctly in the database.
-
Rollback and Database Setup: Use database transactions or setup/teardown methods to ensure that database changes made during testing are rolled back. This maintains a clean database state for each test.
5. Handling Authentication and Sessions
-
Mocking User Sessions: When testing user-specific functionality, create mock user sessions to simulate the behavior of authenticated users. This allows you to verify that protected routes or features work as expected.
-
Testing with Various User Roles: Ensure that your tests cover different user roles and permissions. For example, test whether regular users can access their profiles and perform actions that should be restricted for others.
By following these best practices, you can create tests that are efficient, reliable, and maintainable. Whether you're testing the core functionality of your application or specific features, keeping your test suite organized and meaningful is key to successful Flask testing.
Continuous Integration and Deployment
1. Automating Tests in CI/CD Pipelines
Continuous Integration (CI) and Continuous Deployment (CD) are essential practices in modern software development. They involve automating the testing and deployment of your Flask applications to ensure that code changes are thoroughly tested before they are deployed to production.
-
CI Services: Platforms like Jenkins, Travis CI, and GitHub Actions enable you to automate your test suite. They can be configured to automatically run your tests whenever code changes are pushed to your repository. This helps catch issues early in the development process.
-
Pull Request Checks: Implement checks for pull requests. Before merging code, require that all tests pass. This ensures that code changes do not introduce regressions and that your testing suite remains effective.
2. Ensuring Consistent Environments for Testing
-
Docker Containers: Consider using Docker containers for your CI/CD pipeline. Docker ensures consistent environments across different stages of development, from local development to testing and production.
-
Environment Variables: When deploying to different environments, use environment variables to set configuration options. This allows you to configure your Flask application dynamically based on the environment it's running in.
3. Test Coverage and Code Quality
-
Measuring Test Coverage: Utilize tools like coverage.py to measure your test coverage. Aim for a high test coverage percentage to ensure that most of your code is exercised by tests.
-
Code Review and Peer Testing: Implement a code review process where peers review and test each other's code. This additional layer of testing can uncover issues that may be missed by automated tests.
-
Tools for Code Quality: Consider using code analysis tools such as flake8 and pylint to ensure code quality. These tools help identify coding standards violations and potential issues in your code.
Automating tests in your CI/CD pipeline and maintaining code quality through tools and peer review are integral to ensuring that your Flask application remains stable and reliable throughout its development lifecycle.
Handling Test Failures
No testing process is perfect, and occasionally, tests may fail. Handling test failures effectively is crucial for maintaining a robust testing workflow. Here are some practices to consider:
1. Debugging and Troubleshooting
-
Logging: Implement detailed logging in your application and tests. This helps identify the cause of test failures by providing insight into the test environment and the state of your application during testing.
-
Interactive Debugging: Leverage debugging tools, such as Python's
pdb
oripdb
, to interactively inspect code during test failures. This can be invaluable for identifying the source of an issue. -
Isolation: When a test fails, isolate the problem by running the failing test in isolation. This can help determine whether the failure is caused by the test itself or an issue in the application code.
2. Test Reports and Logs
-
Automated Reporting: Generate test reports that detail which tests have passed and which have failed. Most testing frameworks provide reporting features or integrate with reporting tools.
-
Continuous Monitoring: Continuously monitor test reports, and use tools like email notifications or messaging platforms to alert your team when a test fails.
3. Regression Testing
-
Regression Test Suites: Maintain a suite of regression tests that focus on the critical and frequently used features of your application. Running these tests regularly helps ensure that no previously fixed issues have resurfaced.
-
Integration Tests: Run integration tests on your application to catch issues that may occur when different components interact. Integration tests help ensure the overall functionality of your application remains intact.
-
Version Control: Use version control to track changes in your application code and tests. If a regression is detected, version control can help identify which code changes introduced the issue.
By adopting these practices for handling test failures, you can reduce the downtime caused by failing tests, expedite the debugging process, and maintain the integrity of your testing workflow.
Conclusion
In the world of web development, Flask has carved its niche by offering a lightweight and flexible framework for building web applications. However, the simplicity of Flask does not diminish the importance of testing. Testing remains a vital practice for ensuring the reliability and stability of your Flask applications.
Throughout this article, we've explored the best practices for testing Flask applications, covering a variety of essential topics:
-
Types of Testing: We discussed unit testing, integration testing, and end-to-end (E2E) testing, offering insight into when and how to use each type effectively.
-
Setting Up the Testing Environment: We delved into the importance of choosing the right testing framework, creating a dedicated testing environment, setting up a test database, and configuring environment variables for testing.
-
Best Practices: We emphasized the significance of isolation, naming conventions, testing edge cases, and various aspects of testing in Flask applications.
-
Continuous Integration and Deployment: We explained the value of automated testing in CI/CD pipelines, ensuring consistent environments, and maintaining code quality through test coverage and code analysis.
-
Handling Test Failures: We explored effective strategies for debugging and troubleshooting, generating test reports, and conducting regression testing.
As you embark on your journey to test Flask applications, remember that testing is an ongoing process. It's not merely a box to check; it's a commitment to delivering high-quality, reliable software. By following the best practices outlined in this article and continually refining your testing process, you'll build Flask applications that stand the test of time.
The journey to becoming a proficient Flask developer is marked by the knowledge and implementation of these best practices. By adhering to these principles, you can create Flask applications that are not only functional but also robust and dependable, earning the trust of both users and fellow developers.
Remember, in the world of Flask development, robust testing isn't just a best practice—it's a cornerstone for success.