The best practices with Pandas
By JoeVu, at: April 7, 2023, 11:02 p.m.
Estimated Reading Time: __READING_TIME__ minutes

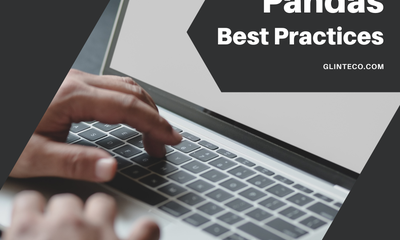
Pandas, the powerful Python library for data manipulation and analysis, has become an indispensable tool for both seasoned data scientists and budding all developers. However, mastering Pandas requires more than just knowing its functions; it involves understanding the nuances and avoiding common pitfalls that can hinder your data manipulation endeavors.
In this article, we'll delve into the world of Pandas to uncover some of the best practices by going through some common exercises. By familiarizing ourselves with these challenges and best practices, we can pave the way for more efficient, accurate, and confident data handling.
1. Using read_csv() and to_csv()
Problem: Not using the appropriate functions for reading and writing CSV files can lead to errors and inefficient code.
Solution: Use read_csv() to read CSV files and to_csv() to write DataFrames to CSV files:
df = pd.read_csv('data.csv')
df.to_csv('output.csv', index=False)
Explanation: Pandas provides dedicated functions for reading and writing CSV files, which handle various configurations and data types efficiently.
2. Method Chaining
Problem: Ignoring method chaining can result in less readable and more verbose code.
Solution: Embrace method chaining to chain operations together, improving code readability:
result = df.filter(['A', 'B']).dropna().groupby('A').mean()
Explanation: Method chaining allows you to perform a sequence of operations on a DataFrame or Series without the need for intermediate variables, making the code more concise.
3. Null Values Handling
Problem: Neglecting missing values can lead to incorrect analysis and calculations.
Solution: Handle missing values using methods like dropna(), fillna(),
and interpolate()
:
cleaned_df = df.dropna() # Remove rows with NaN values
filled_df = df.fillna(0) # Fill NaN values with 0
Explanation: Proper handling of missing values ensures that your analysis and calculations are accurate and unbiased.
4. Vectorized Operations
Problem: Using loops for element-wise calculations can result in slow and inefficient code.
Solution: Leverage vectorized operations for efficient element-wise calculations:
df['C'] = df['A'] * df['B'] # Multiply corresponding elements
Explanation: Pandas' vectorized operations perform calculations much faster than traditional loops, improving code performance.
5. GroupBy and Aggregation
Problem: Misusing the groupby()
function can lead to incorrect aggregations.
Solution: Use the groupby()
function along with aggregation functions like sum(), mean(),
and count()
:
grouped = df.groupby('Category').sum() # Sum values within groups
Explanation: Proper usage of groupby() and aggregation functions helps in analyzing and summarizing data accurately.
6. Indexing and Selection
Problem: Chained indexing can lead to ambiguity and unexpected behavior.
Solution: Use .loc[]
and .iloc[]
for label-based and integer-based indexing:
value = df.loc['B', 'A'] # Access element using labels
Explanation: Using proper indexing methods ensures unambiguous data selection and avoids indexing issues.
7. Data Type Management
Problem: Incorrect data types can lead to errors in calculations and analysis.
Solution: Convert columns to appropriate data types using methods like astype()
:
df['A'] = df['A'].astype(int) # Convert 'A' column to integer
Explanation: Ensuring correct data types is crucial to perform accurate calculations and operations on columns.
8. Memory Optimization
Problem: Large datasets can consume excessive memory if data types are not optimized.
Solution: Optimize memory usage by using appropriate data types and checking memory consumption:
optimized_df = df.copy()
optimized_df['A'] = optimized_df['A'].astype(np.int32)
memory_usage = optimized_df.memory_usage(deep=True).sum()
Explanation: Optimizing data types can significantly reduce memory usage, especially for large datasets.
9. Copying DataFrames
Problem: Modifying a copy of a DataFrame without using .copy() can unintentionally affect the original data.
Solution: Explicitly use .copy()
to create a copy of the DataFrame before modifying it:
subset = df[df['A'] > 1].copy()
subset['B'] = 10 # Modifying 'subset' does not affect 'df'
Explanation: Using .copy() ensures that modifications to a subset of the DataFrame do not alter the original DataFrame.
10. Documentation Reference
Problem: Not referring to the documentation can lead to misunderstanding and misuse of Pandas functions.
Solution: Consult the official Pandas documentation for function explanations and examples:
# Reference the documentation for a specific function
pd.read_csv?
Explanation: The documentation provides detailed explanations, parameter descriptions, and examples for each Pandas function.
11. Testing and Debugging
Problem: Failing to test code thoroughly on smaller datasets can lead to errors on larger datasets.
Solution: Test your code on smaller subsets before applying it to large datasets:
small_df = df.sample(frac=0.1) # Create a smaller subset for testing
# Test your code on 'small_df' before using 'df'
Explanation: Testing on smaller datasets helps identify and fix issues before processing larger amounts of data.
12. Working with DateTime Data
Problem: Incorrectly handling DateTime data can lead to incorrect time-based operations.
Solution: Convert columns to DateTime using pd.to_datetime() for proper DateTime manipulation:
df['Date'] = pd.to_datetime(df['Date'])
Explanation: Converting columns to DateTime data type enables easy and accurate time-based calculations.
13. Efficient Iteration
Problem: Using inefficient iteration methods can lead to slow code performance.
Solution: Use efficient iteration methods like .itertuples() or vectorized operations:
for row in df.itertuples():
print(row.Index, row.A, row.B)
Explanation: Efficient iteration methods improve code performance when iterating over DataFrames.
14. Avoiding apply() with Lambdas
Problem: Using apply()
with lambdas for simple operations can be less efficient than vectorized operations.
Solution: Use vectorized operations for simple element-wise calculations:
df['C'] = df['A'] + df['B'] # Add corresponding elements
Explanation: Vectorized operations are faster and more efficient for element-wise calculations than using apply()
with lambdas.
15. Combining DataFrames
Problem: Incorrect usage of functions like merge() and join() can lead to data misalignment.
Solution: Use functions like merge() with appropriate parameters to combine DataFrames:
merged_df = df1.merge(df2, on='key_column', how='inner')
Explanation: Proper usage of combining functions ensures accurate alignment of data based on common columns.
16. Working with Strings
Problem: Not using the .str accessor for string operations can lead to inefficient code.
Solution: Use the .str accessor for efficient string operations on DataFrame columns:
df['Name_Length'] = df['Name'].str.len()
Explanation: The .str accessor provides optimized string operations on DataFrame columns, improving code performance.
17. Plotting with Pandas
Problem: Neglecting to use Pandas' built-in plotting capabilities can lead to more complex visualization code.
Solution: Utilize Pandas' .plot()
function for quick and convenient data visualization:
df.plot(x='Date', y='Value', kind='line', title='Value Over Time')
Explanation: Pandas provides a simple way to create basic visualizations directly from DataFrame columns, eliminating the need for additional libraries for simple plots.
By adhering to these best practices and understanding their significance, you can enhance your proficiency in working with Pandas and ensure efficient, accurate, and readable data manipulation and analysis.