The Most Common Python Interviewing Questions - JUNIOR level
By JoeVu, at: Jan. 20, 2023, 3:54 p.m.
Estimated Reading Time: __READING_TIME__ minutes
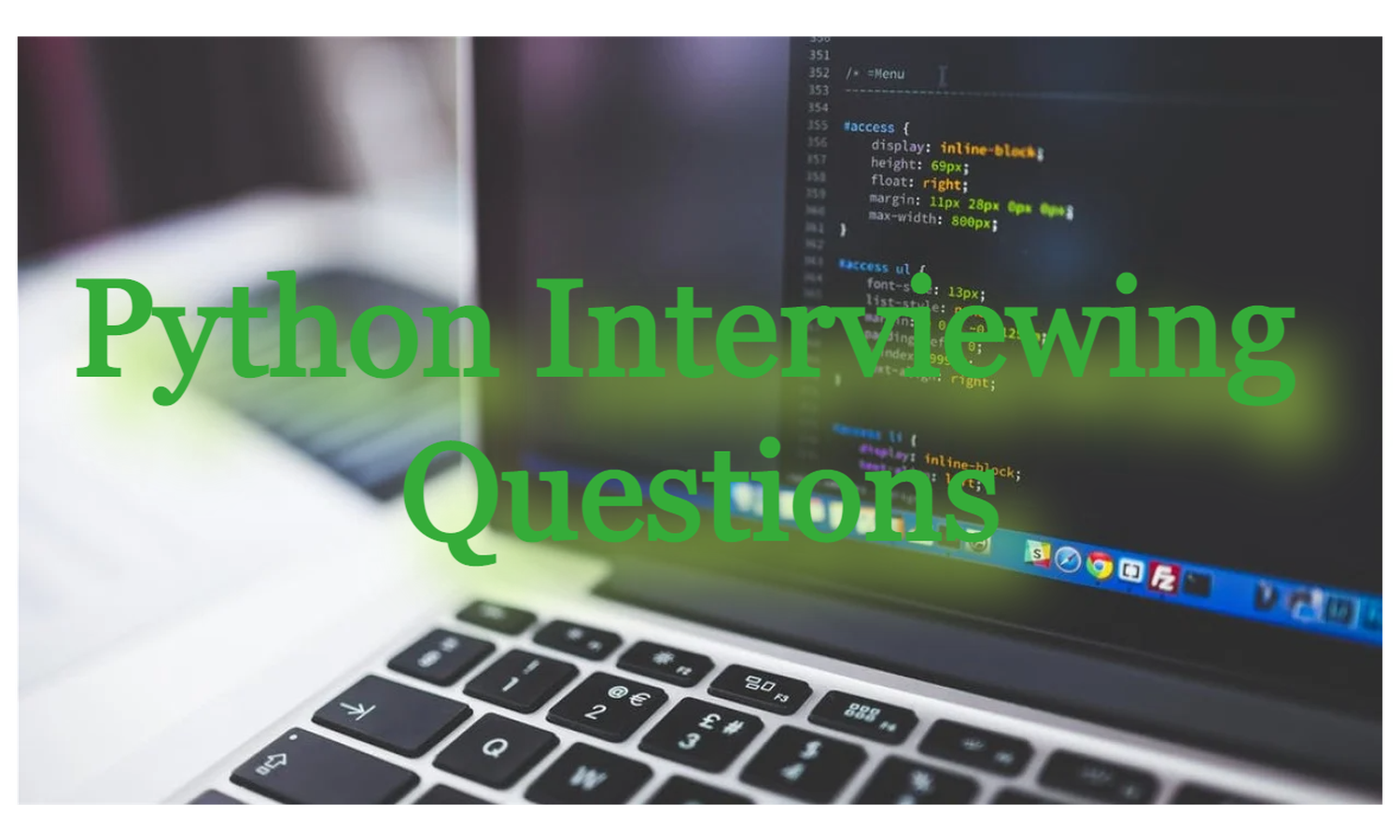
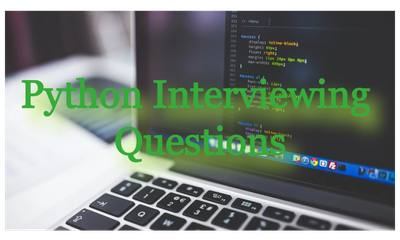
JUNIOR level
1. Name the differences between a list and a tuple in Python
- A list is mutable, meaning its contents can be changed, while a tuple is immutable and cannot be changed.
- A list uses square brackets, [ ], while a tuple uses parentheses, ( ).
- A list can contain any type of object, including other lists, while a tuple can only contain objects of the same type.
- A list can be extended or reduced at will, while a tuple has a fixed number of elements.
When to use tuple, and not to use list
Tuple should be used when the elements of the sequence are not going to be changed. This is useful when we work with a team and there is a variable being used in multiple places and by many developers. The author of the variable wants to keep its original values.
car_colors = (("black", 1), ("white", 2))
def pick_car_colors(color):
for car_color in car_colors:
if car_color[0] == color:
print(color)
car_color[1] += 1
In [26]: pick_car_colors("black")
black
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
Cell In[26], line 1
----> 1 pick_car_colors("black")
Cell In[25], line 7, in pick_car_colors(color)
5 if car_color[0] == color:
6 print(color)
----> 7 car_color[1] += 1
TypeError: 'tuple' object does not support item assignment
2. What are *args and *kwargs in function definition?
In Python, *args and *kwargs are two special symbols used to pass a variable number of arguments to a function.
The special syntax, *args and **kwargs in function definitions is used to pass a variable number of arguments to a function.
*args is used to send a non-keyworded variable length argument list to the function.
**kwargs is used to send a keyworded variable length argument list to the function.
def print_arguments(*args, **kwargs):
print(args)
print(kwargs)
In [2]: print_arguments(1, 2, 3, name="Joe", age=34)
(1, 2, 3)
{'name': 'Joe', 'age': 34}
However, don't overuse the *args and **kwargs keywords, as this conflicts with the "Clean Code" philosophy with Function/Method params limitation (>=3 params should be avoided)
3. What is the difference between a shallow copy and deep copy?
A shallow copy is a copy of an object that only copies the immediate values of the object, while a deep copy is a copy of an object that creates an entirely new object and its contents by copying all of the original object’s values.
In a deep copy, all the values of the original object are copied, including objects that are referenced inside the original object. In a shallow copy, only the values of the immediate object are copied, and any referenced objects are not copied.
original_list = [1, 2, 3]
shallow_copy = original_list
print(original_list)
print(shallow_copy)
original_list[0] = 100
[1, 2, 3]
[1, 2, 3]
print(original_list)
print(shallow_copy)
[100, 2, 3]
[100, 2, 3]
Another example
import copy
original_list = [1, 2, [3, 4]]
deep_copy = copy.deepcopy(original_list)
print(original_list)
print(deep_copy)
[1, 2, [3, 4]]
[1, 2, [3, 4]]
original_list[0] = 100
print(original_list)
print(deep_copy)
[100, 2, [3, 4]]
[1, 2, [3, 4]]
4. Specify the difference between local and global variables
Local variables are variables that are declared within a function and they can only be accessed within that function. Global variables are variables that are declared outside of any function and they can be accessed by any other function or script.
Examples of Local Variables:
- Variable declared in a function
- Variable declared in a loop
Examples of Global Variables:
- Variable declared outside of any function
- Variable declared in the main script
global_variable = "A1"
def get_shapes():
local_variable = "cubic"
global_variables = "B2"
print(local_variable)
print(global_variables)
get_shapes()
cubic
B2
global_variable
'A1'
5. Explain a “list comprehension” in Python.
List comprehension is a powerful way to create a new list from an existing list in Python.
- It is a concise way to create a list from an existing list by applying an expression or a filter to each element in the list.
- It is used to create a new list from existing lists, dictionaries, or other iterables.
- It is similar to the use of map() and filter() functions in functional programming.
- List comprehension is a shorthand way of writing a loop, and often takes less memory and time to execute.
For example, let's say you have a list of numbers, and you want to create a new list that contains each number multiplied by itself. You could do this with a list comprehension like this:
In [1]: [x * x for x in [1, 2, 3, 4, 5]]
Out[1]: [1, 4, 9, 16, 25]
6. Mention the key differences between “range()” and “xrange()” in Python 2
Range(): is a built-in function used to generate a sequence of numbers. It takes 3 parameters, start, stop and step. It returns a list object which contains the elements of the sequence.
Example:
range(1, 10, 2)
-> [1, 3, 5, 7, 9]
xrange(): is another built-in function used to generate a sequence of numbers. It is similar to range() but returns a generator object instead of a list. It is faster than range() and consumes less memory.
Example:
xrange(1, 10, 2)
-> xrange(1, 10, 2)
7. Explain “positive indexes” and “negative indexes” in Python
Positive indexes in Python are used to refer to items in a sequence that are located by their position from the beginning of the sequence, starting with 0.
Negative indexes in Python are used to refer to items in a sequence that are located by their position from the end of the sequence, starting with -1.
a = ['a', 'b', 'c', 'd', 'e', 'f']
a[0]
'a'
a[5]
'f'
a[-1]
'f'
a[-2]
'e'
a[-5]
'b'
a[-6]
'a'
8. What is a Python "iterator"?
A Python iterator is an object that can be iterated over, meaning it can produce a sequence of values. Iterators are implemented using the iter() and next() functions.
- __iter__(): The iter() method is called for the initialization of an iterator. This returns an iterator object
- __next__(): The next method returns the next value for the iterable. When we use a for loop to traverse any iterable object. This method raises a StopIteration to signal the end of the iteration.
Example 1: Iterating through a list
numbers = [1, 2, 3, 4]
for number in numbers:
print(number)
Output
1
2
3
4
Example 2: Iterating through a dictionary
a_dict = {'a': 1, 'b': 2, 'c': 3, 'd': 4}
for key, value in a_dict.items():
print(key, value)
Output
a 1
b 2
c 3
d 4
Example 3: Interating through a set
In [7]: a_set = set(['joe', 'snow', 'bill'])
...: for element in a_set:
...: print(element)
...:
Output
snow
bill
joe
9. What Does the "is" Operator Do?
The "is" operator is used to check if two variables point to the same object. It returns True if both variables point to the same object and False if they do not.
This is extremely useful when comparing two None object
Example 1
x = [1, 2, 3]
y = [1, 2, 3]
print(x is y)
Output
False
Example 2
x = [1,2,3]
y = x
print(x is y)
Output
True
Example 3
x = [1, 2, 3]
y = x
print(x is y)
Output
True
Example 4
if x is None:
print("x is None")
Output
x is None
10. What is the difference Between del() and remove() on Lists?
The del() function is used to delete an item at a specified index while the remove() function is used to remove an item by its value.
Example 1
list1 = [1, 2, 3, 2, 3]
del list1[2]
print(list1)
Output
[1, 2, 2, 3]
Example 2
list1 = [1, 2, 3, 2, 3]
list1.remove(2)
print(list1)
Output
[1, 3, 2, 3]
11. How do you utilize ternary operators in Python?
A ternary operator is a shorthand way of writing an if-else statement. It takes the following form:
condition_if_true if condition else condition_if_false
rating = 3
comment = "good" if rating >= 2.5 else "bad"
print(comment)
Output
good
12. Why do we use virtual environments?
A virtual environment is a tool used to create isolated Python environments that allow us to install packages and dependencies without affecting the global Python installation, and can also help prevent conflicts between different versions of packages and dependencies.
Some good virtual environment packages are:
- virtualenv
- pyenv
- anaconda
- venv
- conda
- pipenv
13. What is the yield keyword used for in Python?
In Python, yield is a keyword used to create generators. Generators are special functions that can be used to generate an iterable set of items. By using the "yield" keyword, a function returns a generator object which can be used in for-loops or be used to create lists, tuples, or sets.
Example 1
def generate_guess_names():
names = ['Johnson', 'Smith', 'Williams', 'Bill', 'Gate', 'Hilary', 'Tom', 'Hank']
for name in names:
yield name
for name in generate_guess_names():
print(name)
Output
Johnson
Smith
Williams
Bill
Gate
Hilary
Tom
Hank