The VueJS Ecosystem: CLI + Router + Vuex
By JoeVu, at: Jan. 10, 2024, 4:14 p.m.
Estimated Reading Time: __READING_TIME__ minutes

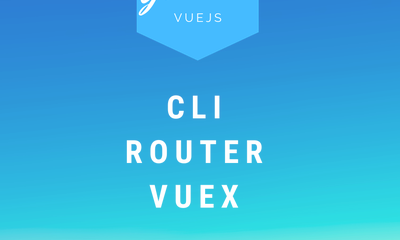
When we talk about the VueJS ecosystem - a powerful suite of tools designed to make web development both enjoyable and efficient, whether you're just starting your journey or you're a professional developer keen on mastering VueJS, this guide aims to provide you with a clear understanding of its core components: Vue CLI, Vue Router, and Vuex. We've structured this guide to ensure it's easy to follow, included code snippets for practical learning, and proposed exercises to reinforce your understanding.
Vue CLI: The Foundation of Vue Development
The Vue Command Line Interface (CLI) is your gateway to Vue development, offering a streamlined process for setting up, developing, and managing Vue projects.
Quick Start with Vue CLI
npm install -g @vue/cli
vue create my-vue-project
cd my-vue-project
npm run serve
This set of commands installs Vue CLI globally, creates a new Vue project named "my-vue-project
" and serves your new project at localhost:8080
.
Exercise: Create a new Vue project and explore the files generated by Vue CLI. Try to modify the App.vue
file to include a personalized message with some random QUOTES every 5 secs
Suggestion:
// Array of quotes
const quotes = [
"The best way to predict the future is to invent it. – Alan Kay",
"A journey of a thousand miles begins with a single step. – Lao Tzu",
"Do not be embarrassed by your failures, learn from them and start again. – Richard Branson",
"You must be the change you wish to see in the world. – Mahatma Gandhi",
"The only way to do great work is to love what you do. – Steve Jobs"
];
// Function to get a random quote
function getRandomQuote() {
const randomIndex = Math.floor(Math.random() * quotes.length);
return quotes[randomIndex];
}
Vue Router: Navigating Through Your Application
Vue Router manages navigation in Vue.js applications. It enables the development of single-page applications with ease.
Setting Up Vue Router
First, you need to add Vue Router to your project:
vue add router
This command automatically sets up routing in your project. Here's a simple example of defining routes in the router/index.js
file:
import Vue from 'vue'
import Router from 'vue-router'
import Home from '../views/Home.vue'
Vue.use(Router)
export default new Router({
routes: [
{
path: '/',
name: 'home',
component: Home
}
]
})
Home.vue:
Exercise: Add a new component called About.vue
in the views
folder. Then, update router/index.js
to include a route for /about
that loads the About
component.
Vuex: State Management Simplified
Vuex is a state management pattern and library for Vue.js applications. It provides a centralized store for all the components in an application.
Integrating Vuex
To add Vuex to your project:
vue add vuex
Here's a simple Vuex store definition in store/index.js
:
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
message: 'Hello Vue!'
},
mutations: {
updateMessage(state, newMessage) {
state.message = newMessage
}
}
})
Exercise: Create a mutation called updateMessage
that changes the message
state. In any component, use this mutation to update the message to something different when a button is clicked.
Conclusion
Congratulations on taking your first steps into the VueJS ecosystem! By exploring Vue CLI, Vue Router, and Vuex, you've laid the groundwork for building dynamic, efficient, and robust web applications. The exercises provided are just the beginning; try to expand upon them by creating a small project that incorporates all three tools.
Remember, the best way to learn is by doing. Don't hesitate to experiment with the code, break things, and then fix them. This process will deepen your understanding of VueJS and its ecosystem. Happy coding!