Unraveling the Complexities: Navigating Django and Celery Challenges
By JoeVu, at: Oct. 2, 2023, 11:19 a.m.

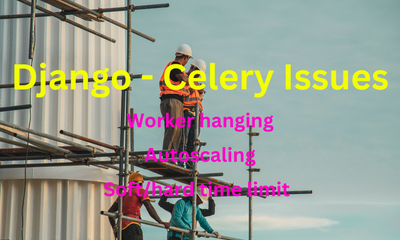
Unraveling the Complexities: Navigating Django and Celery Challenges
Django, the high-level Python web framework, and Celery, the distributed task queue system, they form a potent combination that empowers developers to build robust and scalable web applications. Django takes care of the web-related aspects, while Celery handles the asynchronous and background tasks efficiently. This synergy allows developers to create feature-rich web applications while offloading time-consuming tasks to background workers.
However, like any powerful tool, Django and Celery come with their own set of complexities and challenges. In this article, we will dive deep into some of the common hurdles that developers may encounter.
So, fasten your seatbelts as we journey through the following ten key challenges:
-
Django timezone and Celery timezone issue: Understanding and addressing the nuances of timezones in Django and Celery.
-
Pickle object exception: Tackling the notorious "pickle object exception" and keeping your tasks pickle-friendly.
-
Circular import: Navigating the maze of circular imports and preventing them from wreaking havoc in your codebase.
-
Unit test writing difficulties: Strategies for writing effective unit tests for Django and Celery tasks.
-
Broker connection lost: How to handle and recover from broker connection losses gracefully.
-
Hang issue: Identifying and resolving issues where Celery tasks seem to hang indefinitely.
-
One task queue instead of many task queues: When and why to use a single task queue for multiple tasks.
-
Task time limit: Managing task execution time limits to prevent performance bottlenecks.
-
Unique task execution: Ensuring that tasks are executed uniquely, preventing duplicates and concurrency issues.
-
Workers auto-scale: Techniques for automatically scaling Celery workers based on workload demands.
With each challenge, we'll look into the problem details, provide practical solutions, and equip you with the knowledge to tackle these issues head-on. Let's get started on our journey to conquer the complexities of Django and Celery integration.
Problem 1: Django Timezone and Celery Timezone Issue - issue link
Problem Description:
The problem typically arises when Django and Celery use different timezone settings, resulting in unexpected behavior or incorrect datetime calculations in your tasks. The default TIMEZONE
values in both services are UTC
, therefore if we don't setup them correctly, it would result in different timezones and might cause of inconsistent datetimes
Sample Code Snippet:
# in Django settings.py, we specify ETC timezone
TIME_ZONE = 'ETC'
# then there is a celery task with datetime calculation
@shared_task
def celery_task():
# Get the current time in Celery's timezone (e.g., 'local')
current_time = datetime.now() # This might not match the Django timezone
print(f"Current time in Celery: {current_time}")
Solution:
To resolve the Django timezone and Celery timezone issue, follow these steps:
# in Django settings.py, we specify ETC timezone
TIME_ZONE = 'ETC'
CELERY_TIMEZONE = TIME_ZONE
In the next section, we'll explore another common issue: the "Pickle object exception" in Celery tasks.
Problem 2: Pickle Object Exception - issue link
Problem Description:
Celery serializes (pickles) Python objects to pass them between the application and the workers. While this serialization process is convenient, it can lead to a common issue known as the "Pickle object exception." This exception occurs when Celery attempts to serialize an object that is not pickleable, causing the task to fail.
The PicklingError: Can't pickle <type>: attribute lookup __builtin__.instancemethod failed </type>
can be frustrating, especially when you encounter it with complex or custom Python objects that are not naturally serializable.
Sample Code Snippet:
# Sample Celery task
from celery import shared_task
@shared_task
def celery_task(product):
return product.calculate_profit()
Solution:
To resolve the "Pickle object exception" issue in Celery tasks, consider the following solutions:
-
Use Simple, Serializable Data Types: Stick to using simple data types (e.g., strings, integers, lists, dictionaries) for task arguments and return values. These are naturally pickleable and less likely to trigger exceptions.
data = {"key": "value", "number": 42}
-
Custom Serialization: If you need to pass non-pickleable objects, implement custom serialization and deserialization methods for those objects. You can achieve this by defining the
__reduce__
method in your class. This method should return a tuple of callable functions that can recreate the object.class Product:
def __reduce__(self):
return (self.__class__, ()) -
Celery Task Decorator Settings: Adjust Celery task settings to use alternative serialization methods, such as JSON or MessagePack, instead of the default pickle. This can be done by setting the
accept_content
andresult_serializer
options in your Celery configuration.# celery.py (Celery configuration)
from celery import Celery
app = Celery('myapp')
app.conf.update(accept_content=['json', 'msgpack'], result_serializer='json')
By applying these solutions, you can overcome the "Pickle object exception" and ensure the smooth execution of Celery tasks, even when dealing with complex or custom Python objects.
In the next section, we'll explore the challenge of dealing with circular imports in Django and Celery.
Problem 3: Circular Import - issue link
Problem Description:
Circular imports occur when two or more modules or components import each other directly or indirectly, creating a loop in the import chain. This can lead to unpredictable behavior, import errors, and make your code difficult to maintain.
In Django and Celery projects, circular imports often arise when tasks need to import models, views, or other tasks. Managing these imports correctly is crucial to avoid circular import issues.
Sample Code Snippet:
# Sample Celery task in tasks.py
from store.models import Product
from store.views import my_view
@shared_task
def celery_task():
# Perform some task using MyModel or my_view
pass
# Sample model in models.py
from store.tasks import celery_task
class Product(models.Model):
# Model fields and methods
pass
Solution:
To resolve circular import issues in Django and Celery projects, follow these best practices:
-
Import Where Needed: Import modules or components only where they are needed, rather than at the top of a file. Delaying imports until they are needed reduces the chances of circular imports.
# Sample Celery task in tasks.py
@shared_task
def celery_task():
from store.models import Product
from store.views import my_view
# Perform some task using MyModel or my_view -
Use Function-Based Imports: Import components within functions or methods when possible. This way, the import happens when the function is called, not when the module is loaded.
# Sample model in models.py
from myapp.tasks import celery_taskclass MyModel(models.Model):
def my_method(self):
celery_task.delay()
By adhering to these practices, you can effectively mitigate circular import challenges in your Django and Celery projects, resulting in cleaner, more maintainable code and fewer unexpected errors.
Problem 4: Unit Test Writing Difficulties - issue link
Problem Description:
Writing unit tests is an integral part of ensuring the reliability and stability of your Django and Celery applications. However, testing Celery tasks can sometimes be challenging, as they operate asynchronously and may have external dependencies like databases or message brokers.
Unit test writing difficulties can arise due to the need to handle task execution, assert expected outcomes, and manage test fixtures effectively.
Sample Code Snippet:
# Sample Celery task in tasks.py
from celery import shared_task
@shared_task
def celery_task(data):
# Task logic that interacts with external resources
pass
# Sample unit test in tests.py
from django.test import TestCase
from myapp.tasks import celery_task
class CeleryTaskTestCase(TestCase):
def test_celery_task(self):
data = {"key": "value"}
# How to properly test this Celery task?
# Task execution is asynchronous and external resources are involved
result = celery_task.apply_async(args=(data,))
self.assertTrue(result.successful())
Solution:
To overcome the difficulties of writing unit tests for Celery tasks in Django, consider the following strategies:
-
Override Celery settings to make tasks EAGER: In your settings.py, you can specify Celery settings to mark tasks executed eagerly:
CELERY_TASK_ALWAYS_EAGER = True
Now, in your unit test, you can call the task like a regular Python function:
result = celery_task.delay(data)
self.assertEqual(result, expected_result) -
Patch External Dependencies: Use the
unittest.mock
library to patch external dependencies such as databases, message brokers, or external APIs during testing. This allows you to control the behavior of these dependencies and isolate your tests.from unittest.mock import patch
@patch('myapp.tasks.some_external_function')
def test_celery_task(self, mock_external_function):
# Mock the external function's behavior
mock_external_function.return_value = 'mocked_result'
data = {"key": "value"}
result = celery_task(data)
# Assert the task's behavior based on the mocked external function
self.assertEqual(result, expected_result)
By applying these testing strategies and adapting your Celery tasks for easier testing, you can ensure that your Django and Celery applications remain robust and reliable, even as they grow in complexity. Testing will become more manageable, helping you catch and fix issues early in the development process.
Problem 5: Broker Connection Lost - issue link
Problem Description:
Django and Celery rely on message brokers like RabbitMQ or Redis to manage task queuing and distribution. However, broker connections can be prone to disruptions due to network issues, broker restarts, or other unexpected events. When the broker connection is lost, your Celery tasks may fail to execute, leading to delays and potential data loss.
Dealing with broker connection losses and ensuring the resilience of your Celery setup is crucial to maintaining the reliability of your application.
Sample Code Snippet:
[2023-10-02 11:02:24,502: ERROR/MainProcess] consumer: Cannot connect to redis://localhost:6379/0: Error 61 connecting to localhost:6379. Connection refused..
Trying again in 2.00 seconds... (1/100)
[2023-10-02 11:02:26,514: ERROR/MainProcess] consumer: Cannot connect to redis://localhost:6379/0: Error 61 connecting to localhost:6379. Connection refused..
Trying again in 4.00 seconds... (2/100)
Solution:
To address the issue of broker connection losses and ensure the robustness of your Django and Celery setup, follow these solutions:
-
Broker connection is not started yet: Make sure you start your broker service, ex: Redis
brew services start redis
-
Broker username and password are incorrect: Make sure you follow the correct tutorial/instruction from the official website
By implementing these solutions and best practices, you can minimize the impact of broker connection losses and ensure that your Celery tasks continue to execute reliably, even in the face of network disruptions or broker restarts.
In the next section, we'll address the challenge of task hanging or becoming unresponsive.
Problem 6: Hang Issue - issue link
Problem Description:
In a Celery-based application, tasks are typically expected to execute efficiently and return results promptly. However, in some cases, tasks may hang or become unresponsive, causing delays and potentially impacting the overall performance of your application. There are various reasons sinc PYthon memory management is bad.
Identifying the root cause of task hangs and preventing them is crucial to maintaining the reliability and responsiveness of your system.
Sample Code Snippet:
# Sample Celery task in tasks.py
from celery import shared_task
@shared_task
def celery_task(data):
# Task logic that may hang or become unresponsive
pass
# Sample usage in Django
from myapp.tasks import celery_task
data = {"key": "value"}
try:
result = celery_task.apply_async(args=(data,))
# The task may hang indefinitely, and this code may never proceed
except Exception as e:
print(f"Task failed: {str(e)}")
Solution:
To address the issue of tasks hanging or becoming unresponsive in Celery, follow these solutions:
-
Task Timeout: Implement a task timeout to limit the maximum execution time for your tasks. Celery allows you to set a time limit for task execution using the
soft_time_limit
andtime_limit
options in your task decorator.@shared_task(soft_time_limit=300, time_limit=360) # Soft limit of 5 minutes, hard limit of 6 minutes
def celery_task(data):
# Task logic that may hang or become unresponsive -
Graceful Termination: Within your task logic, periodically check if the task should terminate early. Implement graceful termination by checking for a termination signal or a timeout condition and exiting the task gracefully.
from celery.exceptions import SoftTimeLimitExceeded
@shared_task(soft_time_limit=300, time_limit=360)
def celery_task(data):
try:
# Task logic that may hang or become unresponsive
# Check for a timeout condition or termination signal
if should_terminate():
return "Task terminated gracefully"
except SoftTimeLimitExceeded:
# Handle soft time limit exceeded here
return "Task terminated due to time limit exceeded" -
Monitoring and Logging: Implement comprehensive monitoring and logging for your Celery tasks. Log critical information about task execution, including start times, progress, and completion. This can help diagnose issues when tasks hang. Flower is a great tool.
-
Task Profiling: Profile the execution of your tasks to identify bottlenecks or performance issues that may lead to hangs. Tools like Python's
cProfile
or specialized profiling libraries can help. -
Concurrency Control: Adjust the concurrency settings for your Celery workers to ensure they do not become overloaded. Overloaded workers are more likely to experience hangs. Experiment with worker pool settings and concurrency limits.
By implementing these solutions and best practices, you can mitigate the risk of tasks hanging or becoming unresponsive in your Celery-based application. This ensures that your application remains responsive and maintains high performance even under heavy workloads.
Problem 7: One Task Queue Instead of Many Task Queues - issue link
Problem Description:
In a complex Django and Celery application, you may have multiple types of tasks, each with different priorities, requirements, and execution characteristics. It's common to create separate task queues for different task types to manage them effectively.
However, managing numerous task queues can become challenging and may lead to increased complexity in your configuration and monitoring efforts.
Sample Code Snippet:
# Sample Celery configuration in celery.py
from celery import Celery
app = Celery('myapp')
app.conf.update(
task_queues={
'high_priority': {'exchange': 'high_priority'},
'default': {'exchange': 'default'},
'low_priority': {'exchange': 'low_priority'},
},
# Other Celery configuration settings...
)
Solution:
To simplify the management of task queues in your Django and Celery application and address the challenge of having multiple queues, consider the following solutions:
-
Default Queue for Most Tasks: Use a single default queue for most of your tasks. Reserve the use of additional queues for specific cases where it is necessary, such as high-priority or low-priority tasks.
# Sample Celery configuration with a default queue
from celery import Celery
app = Celery('myapp')
app.conf.update(
task_default_queue='default',
# Other Celery configuration settings...
) -
Priority Routing: Instead of using separate queues for different priorities, use task routing to assign priorities to tasks. You can define custom routing rules based on task attributes.
# Sample Celery configuration with priority routing
from celery import Celery
app = Celery('myapp')
app.conf.update(
task_routes={
'myapp.tasks.high_priority_task': {'queue': 'high_priority'},
'myapp.tasks.low_priority_task': {'queue': 'low_priority'},
# Default queue for all other tasks
},
# Other Celery configuration settings...
) -
Monitoring and Visibility: Implement monitoring and visibility tools like Celery Flower to gain insights into the status and performance of your task queues. These tools can help you identify and address bottlenecks or issues in your task processing.
-
Dynamic Queue Creation: For dynamic scenarios where you need to create task queues on-demand, consider using Celery's support for dynamic queue creation. You can create and configure queues programmatically based on your application's needs.
By implementing these solutions and focusing on a simplified task queue management strategy, you can reduce complexity, improve maintainability, and ensure efficient task processing in your Django and Celery application. This approach allows you to strike a balance between fine-grained task control and ease of management.
Problem 8: Task Time Limit - issue link
Problem Description:
In a Celery-based application, some tasks may take longer to complete than expected, potentially leading to performance issues and resource bottlenecks. It's essential to set time limits on tasks to prevent them from running indefinitely and affecting the overall responsiveness of your system.
However, setting the appropriate time limits for tasks can be challenging, as it requires balancing task complexity with execution time constraints.
Sample Code Snippet:
# Sample Celery task in tasks.py
from celery import shared_task
@shared_task
def long_running_task(data):
# Task logic that may take a long time to complete
pass
# Sample usage in Django
from myapp.tasks import long_running_task
data = {"key": "value"}
try:
result = long_running_task.apply_async(args=(data,))
print(result.get())
except Exception as e:
print(f"Task failed: {str(e)}")
Solution:
To address the challenge of setting appropriate time limits for tasks in your Django and Celery application, consider the following solutions:
-
Default Time Limits: Establish default time limits for most tasks based on your application's typical workload. Set these defaults in your Celery configuration.
# Sample Celery configuration with default time limit
from celery import Celery
app = Celery('myapp')
app.conf.update(
task_default_timeout=300, # Set a default time limit of 5 minutes (in seconds)
# Other Celery configuration settings...
) -
Custom Time Limits: For tasks with specific time constraints, set custom time limits in the task decorator. This allows you to fine-tune the time limit for individual tasks.
# Sample Celery task with a custom time limit
@shared_task(time_limit=60) # Set a custom time limit of 1 minute (in seconds)
def long_running_task(data):
# Task logic that may take a long time to complete -
Progressive Backoff: For tasks that frequently hit time limits, implement a progressive backoff strategy. Instead of failing immediately, allow tasks to retry with increasing time limits until they succeed or reach a maximum limit.
-
Task Splitting: If a task's logic is too complex and time-consuming, consider breaking it down into smaller subtasks. This can make it easier to manage time limits and improve parallelism.
-
Parallel Execution: Utilize Celery's concurrency features to execute tasks in parallel. By processing multiple tasks simultaneously, you can reduce the impact of time limits on task completion.
By implementing these solutions and strategies, you can effectively manage task time limits in your Django and Celery application, ensuring that tasks complete within acceptable timeframes while maintaining optimal system performance.
Problem 9: Unique Task Execution - issue link
Problem Description:
In many scenarios, you may want to ensure that a specific task is executed only once at a time, regardless of how it's triggered. Without proper handling, there's a risk of tasks running concurrently, leading to data inconsistencies, race conditions, or other unexpected issues.
Guaranteeing the uniqueness of task execution is essential for maintaining data integrity and ensuring that critical tasks are not duplicated.
Sample Code Snippet:
# Sample Celery task in tasks.py
from celery import shared_task
@shared_task
def unique_task(product_id):
from store.models import Product
# Task logic that must run as a single instance at any given time
product = Product.objects.get(id=product_id)
product.update_in_stock_quantity()
# Sample usage in Django
from myapp.tasks import unique_task
try:
for _ in range(10):
result = unique_task.apply_async(args=(10,))
print(result.get())
except Exception as e:
print(f"Task failed: {str(e)}")
Solution:
To address the challenge of ensuring unique task execution in your Django and Celery application, consider the following solutions:
-
Task Locking: Implement a task locking mechanism using a distributed lock manager like Redis or Memcached. Before executing the task, acquire a lock with a unique name. If the lock is already held, the task should not proceed.
# Sample Celery task with task locking
from celery import shared_task
from django.core.cache import cache
@shared_task
def unique_task(data):
task_lock = cache.lock('unique_task_lock', timeout=300) # Lock expires after 5 minutes
if task_lock.acquire(blocking=False):
try:
# Task logic that must run as a single instance at any given time
finally:
task_lock.release()
else:
# Another instance of the task is already running
pass -
Task Status Tracking: Maintain a task status in your database or a distributed data store. Before executing the task, check its status. If it's in progress or completed, skip the execution.
# Sample Celery task with task status tracking
from celery import shared_task
from myapp.models import TaskStatus
@shared_task
def unique_task(data):
task_status, created = TaskStatus.objects.get_or_create(name='unique_task')
if task_status.status == 'pending':
task_status.status = 'in_progress'
task_status.save()
try:
# Task logic that must run as a single instance at any given time
finally:
task_status.status = 'completed'
task_status.save()
else:
# Another instance of the task is already running
pass -
Task Deduplication: Use Celery's built-in task deduplication features. Celery provides the
task_id
attribute that allows you to deduplicate tasks based on their unique IDs. Check whether a task with the sametask_id
is already in progress before executing it.# Sample Celery task with task deduplication
from celery import shared_task
from celery.exceptions import Ignore
@shared_task
def unique_task(data):
task_id = data.get('task_id')
if task_id and cache.get(task_id):
# Task with the same task_id is already running
raise Ignore()
try:
# Task logic that must run as a single instance at any given time
finally:
cache.delete(task_id)
By implementing these solutions, you can ensure that specific tasks are executed as single instances, preventing concurrency issues and maintaining data consistency in your Django and Celery application.
Problem 10: Workers Auto Scale - issue link
Problem Description:
In a dynamic web application, the workload can vary significantly over time. At certain periods, the application may experience high traffic, leading to increased task processing demands. During low-traffic periods, maintaining a large number of active Celery workers can be inefficient and costly.
The challenge is to dynamically scale the number of Celery workers to match the current workload, ensuring that tasks are processed efficiently without overprovisioning resources during idle periods.
Sample Code Snippet:
# Sample Celery worker scaling using command-line arguments
$ celery -A myapp worker --concurrency=4 # Start with 4 worker processes
Solution:
To address the challenge of auto-scaling Celery workers in your Django and Celery application, consider the following solutions:
-
Use dynamic concurrency settings: Use dynamic concurrency settings for Celery workers that can be adjusted based on workload demands. Instead of manually specifying the number of worker processes, consider using a formula or algorithm to calculate the appropriate concurrency level.
# Sample Celery worker scaling with dynamic concurrency settings $ celery -A myapp worker --concurrency=$(python calculate_concurrency.py)
-
Load-Based Scaling: Implement load-based scaling by monitoring system resource utilization, task queue lengths, or other relevant metrics. Use this data to dynamically adjust the number of Celery workers. Tools like Prometheus and Grafana can assist in this process.
-
Celery Auto Scaling Plugins: Explore Celery auto-scaling plugins like
--autoscale
that provide mechanisms for automatic scaling based on criteria such as the length of the task queue.
# Auto scale worker processes from 2 to 10
$ celery -A myapp worker --concurrency=4 --autoscale=10,2 -
Scheduled Scaling: Implement scheduled scaling by scheduling Celery worker scaling actions at specific times or intervals. This approach allows you to pre-scale workers in anticipation of expected traffic spikes.
-
Cloud-Based Scaling: If your application is hosted on a cloud platform (e.g., AWS, Azure, Google Cloud), leverage auto-scaling features provided by the cloud provider to automatically adjust the number of Celery workers based on CPU usage, queue length, or other metrics.
-
Custom Scaling Logic: Develop custom scaling logic that considers application-specific workload patterns and requirements. Your logic can take into account historical data, traffic patterns, and user-defined rules for scaling.
-
Graceful Worker Shutdown: Ensure that workers are scaled up and down gracefully to avoid disrupting ongoing task processing. Allow workers to complete their current tasks before shutting them down during scale-down operations.
By implementing these solutions and strategies, you can achieve efficient and automatic scaling of Celery workers in your Django application. This ensures that your application can handle varying workloads effectively while optimizing resource utilization and cost.
Conclusion
Django and Celery offer powerful tools for building scalable and asynchronous web applications. However, as with any technology stack, you may encounter various challenges along the way. In this article, we've explored ten common issues that developers may face when working with Django and Celery, along with solutions to mitigate these challenges:
-
Django Timezone and Celery Timezone Issue: Addressing timezone inconsistencies between Django and Celery by configuring them correctly.
-
Pickle Object Exception: Handling exceptions related to non-pickleable objects in Celery tasks.
-
Circular Import: Managing circular imports that can lead to code complexity and errors.
-
Unit Test Writing Difficulties: Overcoming obstacles when writing unit tests for Celery tasks.
-
Broker Connection Lost: Ensuring resilience against broker connection disruptions.
-
Hang Issue: Preventing tasks from hanging or becoming unresponsive.
-
One Task Queue Instead of Many Task Queues: Simplifying task queue management.
-
Task Time Limit: Setting appropriate time limits for tasks.
-
Unique Task Execution: Guaranteeing that specific tasks are executed as single instances.
-
Workers Auto Scale: Dynamically scaling Celery workers based on workload demands.
By understanding and implementing these solutions, you can enhance the reliability, performance, and maintainability of your Django and Celery applications. These tools and best practices empower you to tackle common issues effectively and ensure that your application runs smoothly, even as it grows and evolves.
Remember that every application is unique, and you may encounter specific challenges that require tailored solutions. Continuously monitor and fine-tune your Django and Celery setup to meet the specific needs of your project, and you'll be well-prepared to build robust and scalable web applications.
Ongoing Monitoring and Maintenance
Building and deploying a Django and Celery application is just the beginning. Ongoing monitoring and maintenance are critical to ensuring the long-term success of your project:
-
Monitoring Tools: Implement comprehensive monitoring tools to keep an eye on the health and performance of your application. Tools like Celery Flower, Prometheus, Grafana, and Django Debug Toolbar can provide invaluable insights.
-
Error Handling and Logging: Implement robust error handling and logging mechanisms in your Celery tasks and Django application. This helps you quickly identify and address issues as they arise. Sentry is a great tool.
-
Task Versioning: Consider versioning your Celery tasks, especially if your application undergoes frequent updates. Task versioning ensures that tasks are compatible with the data structures they expect.
-
Backup and Recovery: Regularly back up critical data and configurations related to your Celery setup, including task results, broker configurations, and Celery settings. Establish recovery procedures in case of data loss or system failures.
Optimization and Performance
Optimizing your Django and Celery application is an ongoing process:
-
Database Optimization: Optimize database queries and indexes to reduce database load. Use tools like Django Debug Toolbar to analyze query performance.
-
Task Batching: Batch related tasks together to reduce the overhead of starting and stopping worker processes. This can improve task processing efficiency.
-
Concurrency Tuning: Experiment with Celery's concurrency settings to find the optimal balance between worker processes and system resources.
-
Caching: Implement caching for frequently accessed data to reduce the load on your database and improve response times.
-
Scaling Strategies: Continuously review your auto-scaling strategies and adjust them based on real-world performance data.
Documentation and Collaboration
Effective documentation and collaboration are key to maintaining a successful project:
-
Documentation: Maintain thorough documentation for your Django and Celery application, including task descriptions, configurations, and troubleshooting guides. Well-documented code and processes make it easier for your team to work efficiently.
-
Collaboration: Foster collaboration among your development, operations, and DevOps teams. Regular communication and collaboration can help identify and address issues more effectively.
-
Training: Ensure that your team is well-trained in using Django and Celery. Knowledge sharing and training sessions can help everyone stay up to date with best practices.
-
Community and Resources: Leverage the broader developer community and online resources, such as forums, blogs, and tutorials, to stay informed about the latest developments and solutions related to Django and Celery.
In conclusion, Django and Celery offer a powerful combination for building robust, scalable, and asynchronous web applications. While challenges may arise, a proactive approach to problem-solving, continuous monitoring, and ongoing optimization can help you navigate these hurdles successfully. By following best practices and staying engaged with the developer community, you'll be well-equipped to build and maintain high-quality Django and Celery projects.