Web Application E2E Testing with Python/Playwright
By khoanc, at: Oct. 16, 2023, 8:38 p.m.
Estimated Reading Time: __READING_TIME__ minutes

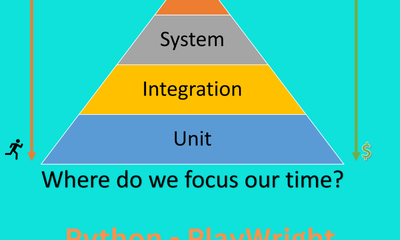
1. Introduction
What is End-to-End (E2E) Testing?
End-to-End (E2E) testing is a vital quality assurance process in web development. It involves testing an application's entire workflow, simulating real user interactions. The goal is to ensure that all components, from the user interface to the server, function seamlessly together.
E2E testing stands at the apex of the testing pyramid, covering the broadest scope. It validates that your web application works correctly under various conditions, from the front-end UI to the back-end database, addressing critical questions:
- Does the application perform as expected from the user's perspective?
- Can users perform essential tasks without issues?
- Are user interactions and data processing flawless from start to finish?
Importance of E2E Testing
E2E testing is indispensable for several reasons:
-
Detecting Critical Issues Early: E2E tests uncover issues early in the development cycle. Detecting and addressing these problems before they reach production can save significant time, effort, and resources.
-
Ensuring a Smooth User Experience: E2E tests ensure that your application provides a seamless and satisfactory experience for users. This includes testing the complete user journey, such as registration, login, shopping, or any other essential function.
-
Preventing Costly Bugs in Production: Identifying and rectifying issues in production can be extremely costly and can harm your reputation. E2E tests help minimize the chances of such issues by catching them in earlier stages.
Pros and Cons of E2E Testing
Pros:
- Comprehensive Validation: E2E tests cover the entire application, providing thorough validation.
- Real-World Scenarios: They simulate real user scenarios, helping ensure user satisfaction.
- Bug Prevention: Early detection of issues helps prevent bugs in production.
- Increased Confidence: Passing E2E tests gives confidence in the application's reliability.
Cons:
- Slower Execution: E2E tests are slower than unit tests or integration tests due to their extensive scope.
- Maintenance: Creating and maintaining E2E tests can be time-consuming.
- Setup Complexity: Configuring the E2E testing environment requires careful setup.
In this article, we'll explore the world of E2E testing with Python and Playwright, focusing on its importance, advantages, and challenges. We'll also dive into how to set up the testing environment and write effective E2E tests to ensure your web applications meet user expectations.
2. The Most Popular E2E Testing Python Tools
E2E testing in Python is made easier with a variety of testing libraries and frameworks. Each of these tools comes with its own unique features and capabilities, allowing you to choose the one that best suits your project requirements. Let's explore some of the most popular E2E testing tools available in the Python ecosystem:
Selenium
Features:
- Cross-browser compatibility: Selenium supports multiple web browsers.
- Extensive language support: It's not limited to Python; you can write tests in various languages.
- Large user community: A wide community of users provides ample resources and support.
- Parallel test execution: Selenium allows for the parallel execution of tests, speeding up the testing process.
from selenium import webdriver
# Example Selenium script to open a webpage and click a button
driver = webdriver.Chrome()
driver.get("https://example.com")
button = driver.find_element_by_id("myButton")
button.click()
Playwright
Features:
- Multi-browser support: Playwright works with all major web browsers (Chromium, Firefox, and WebKit).
- Automation capabilities: It offers automation for web pages, browser contexts, and browsers.
- Speed and reliability: Playwright is known for its speed and reliability in automating web interactions.
- Headless mode: You can run tests in headless mode to save resources and run tests silently.
from playwright.sync import sync_playwright
# Example Playwright script to open a webpage and click a button
with sync_playwright() as p:
browser = p.chromium.launch()
page = browser.new_page()
page.goto("https://example.com")
page.click("button")
Robot Framework
Features:
- Human-readable syntax: Robot Framework uses a simple, easy-to-understand syntax.
- Extensive libraries: It provides a rich set of libraries for various test automation tasks.
- Test documentation: Robot Framework generates detailed test documentation.
- Cross-platform support: It works on multiple operating systems.
# Example Robot Framework test to open a webpage and click a button
*** Settings ***
Library SeleniumLibrary
*** Test Cases ***
Open Example Webpage
Open Browser https://example.com chrome
Click Element id=myButton
Close Browser
Each of these tools has its strengths and may be better suited to different project needs. The choice of tool depends on your project's requirements, your team's familiarity with the tool, and other factors such as speed, ease of use, and maintenance.
3. Why Python/Playwright for E2E Testing
Convincing Reasons for Choosing Playwright
When it comes to choosing a tool for E2E testing, Playwright for Python offers several compelling advantages:
1. Cross-Browser Compatibility
Playwright supports all major web browsers, including Chromium, Firefox, and WebKit. This allows you to test your application across different browsers with the same codebase, ensuring maximum compatibility.
2. Modern Web Automation
Playwright is designed for modern web automation needs. It provides native support for interactions with web pages, browser contexts, and browsers, making it a powerful and versatile tool for E2E testing.
3. Speed and Reliability
Playwright is known for its speed and reliability. It executes tests quickly and accurately, which is essential for efficient and effective testing in a fast-paced development environment.
4. Headless Mode
Playwright can run tests in headless mode, which means that tests are executed without a graphical user interface. This not only conserves system resources but also allows for silent and efficient testing in automated pipelines.
Setting Up the E2E Testing Environment
To get started with E2E testing using Python and Playwright, you'll need to set up your testing environment. Here are the key steps:
1. Installing Python
If you haven't already, install Python on your system. Python is widely supported and can be installed from the official Python website or via package managers such as pip
or conda
.
2. Creating a Virtual Environment
It's a good practice to create a virtual environment for your E2E testing project. This isolates your project's dependencies from the system-wide Python installation and ensures a clean and consistent environment.
You can create a virtual environment using the following commands:
# Create a virtual environment
python -m venv myenv
# Activate the virtual environment
# On Windows
myenv\Scripts\activate
# On macOS and Linux
source myenv/bin/activate
3. Installing Playwright
Playwright can be easily installed within your virtual environment using pip
. The following commands install Playwright for Chromium, which is a popular choice for E2E testing:
# Install Playwright for Chromium
pip install playwright
playwright install # Install web browsers for testing
4. Configuring Web Browsers
You can configure Playwright to use different web browsers for your tests. For example, if you want to use Firefox or WebKit, you can install them with playwright install
and then configure your test scripts accordingly.
With the testing environment set up, you're ready to start writing your first E2E test using Python and Playwright.
4. Writing Your First E2E Test
Now that your testing environment is configured, it's time to create your first E2E test using Python and Playwright. In this section, we'll cover the basic structure of an E2E test script, how to interact with web pages, and how to perform assertions to validate your application's functionality.
Creating a Test Script
An E2E test script typically follows a structure that includes setup, execution, and cleanup phases. Here's a basic outline of a test script:
from playwright.sync import sync_playwright
def test_example():
with sync_playwright() as p:
browser = p.chromium.launch()
context = browser.new_context()
page = context.new_page()
# Test steps
page.goto("https://example.com")
# Perform actions (e.g., clicking buttons, filling forms)
# Assertions to validate the expected outcome
context.close()
Interacting with Web Pages
Interacting with web pages in your E2E tests is essential for simulating user actions. Playwright provides methods to perform various actions on web pages, such as:
goto(url)
: Navigate to a specific URL.click(selector)
: Click on elements identified by a CSS selector.type(selector, text)
: Type text into input fields.fill(selector, text)
: Fill text in form fields.check(selector)
: Check checkboxes.select_option(selector, value)
: Select options in dropdowns.- And many more...
Assertions and Test Validation
To validate the expected behavior of your web application, you need to include assertions in your test script. Assertions are checks that compare the actual state of the application with the expected state. In Python, you can use the assert
statement to perform assertions.
For example, you can check if a page's title matches your expectation:
# Assert the page title
assert page.title() == "Example" # the expected title
You can also assert other elements, values, or conditions on the page to ensure the correct functionality of your web application.
As you write more complex E2E tests, you'll use a combination of page interactions and assertions to thoroughly test your application.
5. Handling Difficulties in E2E Testing
E2E testing often involves addressing specific challenges related to testing real-world user scenarios. In this section, we'll explore common difficulties in E2E testing and how to handle them effectively.
Testing User Authentication
User authentication is a critical aspect of web applications, and it's essential to test it thoroughly. To address this challenge, your E2E tests should cover scenarios such as user registration, login, and password recovery. Here's how you can approach it:
-
Registration Testing: Write tests to simulate user registration. Ensure that users can sign up successfully and that their credentials are stored correctly in the database.
-
Login Testing: Create tests to verify that registered users can log in with their credentials. Check that the authentication process works as expected, including handling invalid login attempts.
-
Password Recovery Testing: Test the password recovery mechanism to ensure that users can reset their passwords securely.
Handling Web Forms
Web forms are a fundamental part of many web applications. To effectively test web forms, follow these guidelines:
-
Input Validation: Test input validation by providing both valid and invalid data to form fields. Check whether the application handles incorrect input gracefully and that it prevents malicious input.
-
Form Submission: Ensure that the form submission process works as expected. This includes checking that data is correctly processed and stored in the backend.
-
Error Handling: Test error handling scenarios, such as submission errors, network issues, or server failures. Verify that the application displays appropriate error messages and gracefully recovers from issues.
Testing Dynamic Content
Many web applications feature dynamic content that changes based on user interactions, real-time data updates, or external events. To address dynamic content in your E2E tests:
-
Real-Time Updates: Write tests to ensure that dynamic content updates in real-time. For example, if your application has a chat feature, verify that new messages are displayed without needing to refresh the page.
-
Data Synchronization: Test data synchronization between the frontend and the backend. Check that the displayed content accurately reflects the data stored on the server.
-
External Dependencies: If your application relies on external APIs or services, consider mocking or stubbing these services during testing to ensure stable and predictable tests.
Handling these difficulties in your E2E testing strategy is crucial for a comprehensive assessment of your web application's functionality. By addressing user authentication, web forms, and dynamic content, you can help ensure that your application performs flawlessly for real users.
6. Effective Test Data Management
Effective test data management is a critical component of E2E testing. It ensures that your tests are robust, repeatable, and reliable. In this section, we'll explore various aspects of test data management in the context of E2E testing.
Generating Test Data
Generating test data is a fundamental step in E2E testing. You need a variety of data to cover different test scenarios. Here's how you can approach test data generation:
-
Random Data Generation: Use libraries or functions to generate random data for form inputs, user credentials, and other test data requirements.
-
Test Data Factories: Create data factories or builders that allow you to generate structured test data. These factories can be reused across different tests.
-
Data Seeding: Seed your test environment with predefined data to ensure consistent and known starting points for your tests.
An well-known package is Faker
Handling Test Data Databases
Many web applications rely on databases for data storage. To effectively manage test data in databases:
-
Database Snapshot and Restore: Consider taking snapshots of your database in a known state before tests run. After the tests, restore the database to its original state. This ensures tests won't impact production data.
-
Database Migrations: Utilize database migration scripts to set up and tear down databases for testing. This allows you to create and destroy test databases with ease.
-
Transaction Rollback: Use transaction rollbacks to ensure that any changes made during a test are rolled back, leaving the database in its initial state.
Test Data Best Practices
To manage test data effectively and maintain test data integrity, consider the following best practices:
-
Isolation: Isolate test data from production data to prevent accidental data corruption.
-
Data Versioning: Version your test data to maintain compatibility with evolving database schemas.
-
Data Cleanup: Implement data cleanup routines to remove test data after tests have been executed, ensuring that your test environment remains clean.
-
Parameterization: Utilize parameterized test cases to test with different sets of test data, covering various scenarios.
-
Test Data as Code: Represent test data as code, making it more maintainable and allowing for version control.
Proper test data management ensures that your E2E tests are reliable and that they produce consistent results. It also enables you to test various scenarios and edge cases effectively, leading to a more robust testing strategy.
7. Advanced E2E Testing Techniques
To ensure a thorough evaluation of your web application's functionality and performance, advanced E2E testing techniques can be invaluable. In this section, we'll explore three advanced techniques: testing mobile responsiveness, integrating API testing within E2E tests, and assessing performance.
Testing Mobile Responsiveness
In today's digital landscape, mobile responsiveness is crucial. Users access websites and applications on a wide range of devices. Testing mobile responsiveness ensures a seamless user experience across various screen sizes. Here's how to approach this:
-
Emulate Mobile Devices: Use Playwright's built-in device emulation to simulate different mobile devices. Test your application's behavior and responsiveness on smartphones and tablets.
-
Viewport Testing: Verify that your application adapts to different viewport sizes. Check that content remains readable and interactive on small screens.
-
Orientation Testing: Test how your application responds to changes in device orientation (landscape to portrait and vice versa).
API Testing in E2E Tests
Modern web applications often rely on APIs for data exchange between the frontend and the backend. Including API testing within your E2E tests can uncover issues that may not be visible through UI testing alone. Here's how to integrate API testing:
-
Mocking APIs: Utilize tools and libraries to mock APIs, ensuring that you test against controlled responses without affecting production data.
-
Data Consistency: Confirm that data displayed in the UI is consistent with the data retrieved from the API. Ensure that API endpoints return the expected responses.
-
API Security: Include tests to assess API security, such as verifying that endpoints are properly secured and authentication mechanisms work as intended.
Performance Testing
Evaluating the performance of your web application is crucial to ensure it can handle real-world usage without slowdowns or errors. You can integrate performance testing into your E2E tests with the following techniques:
-
Load Testing: Use load testing tools to simulate a large number of concurrent users and assess how your application performs under heavy loads.
-
Response Time Testing: Measure response times for critical actions and ensure they meet acceptable thresholds.
-
Resource Utilization: Monitor resource utilization during E2E tests, such as CPU and memory usage, to identify any performance bottlenecks.
By incorporating these advanced techniques into your E2E testing strategy, you can gain a more comprehensive understanding of your web application's behavior, including its mobile responsiveness, API integrations, and performance under various conditions.
One famous package for Stress test is Locust
8. Best Practices for Web Application E2E Testing
Effective E2E testing relies on best practices to ensure that your testing process is organized, efficient, and reliable. In this section, we'll explore key best practices for E2E testing to help you achieve successful testing outcomes.
Test Script Organization
Organizing your test scripts is essential for maintainability and collaboration among team members. Here are some best practices for test script organization:
-
Modularization: Break down your E2E tests into modular components. Each test case should focus on a specific functionality or scenario.
-
Naming Conventions: Use clear and descriptive naming conventions for your test scripts, test cases, and test suites. This makes it easier to understand the purpose of each test.
-
Test Data Separation: Keep test data separate from test scripts. Store test data in external files or databases for easy management and updates.
-
Documentation: Include comments and documentation in your test scripts to explain the purpose of the test, the expected outcomes, and any preconditions.
Continuous Integration (CI) Integration
Integrating E2E testing into your CI pipeline ensures that tests are automatically executed whenever there are code changes. Here's how to effectively incorporate E2E testing into your CI process:
-
Automation: Automate the execution of E2E tests as part of your CI/CD process. Tools like Jenkins, Travis CI, and GitLab CI/CD can trigger test runs when code changes are pushed.
-
Parallel Execution: Consider parallel execution of tests to reduce test execution time. This is especially important for large test suites.
-
Environment Configuration: Ensure that your CI environment is configured to match your testing environment as closely as possible to avoid inconsistencies.
-
Notifications: Set up notifications and alerts to notify team members when E2E tests fail. Immediate feedback helps identify and address issues promptly.
Reporting and Monitoring
Effective reporting and monitoring provide insights into the results of your E2E tests and help identify areas for improvement. Consider the following practices:
-
Detailed Test Reports: Generate detailed test reports that include information on test execution, pass/fail status, and any errors encountered.
-
Screenshots and Logs: Include screenshots and logs in your reports for failed tests. These can help diagnose issues more easily.
-
Historical Data: Maintain a historical record of test results to identify trends and patterns in test performance and reliability.
-
Alerts and Notifications: Set up alerting and notification mechanisms to inform relevant team members when tests fail. This ensures that issues are promptly addressed.
By implementing these best practices, you can create a robust and efficient E2E testing process that improves the quality and reliability of your web applications.
9. Tips and Tricks for Efficient E2E Testing
Efficiency is crucial when conducting E2E testing. The following tips and tricks can help streamline your testing efforts, reduce test execution time, and improve the overall effectiveness of your E2E tests.
Reducing Test Execution Time
E2E tests can be time-consuming, so optimizing test execution time is essential. Here are some strategies to make your tests run faster:
-
Selective Test Execution: Prioritize critical tests for frequent execution and run less critical tests less frequently.
-
Parallel Execution: If your test framework supports it, run tests in parallel on multiple machines to reduce overall execution time.
-
Headless Mode: Execute tests in headless mode to avoid the graphical user interface, which can speed up test execution.
-
Sandboxing: Use sandboxes to isolate test environments and prevent interference between tests.
-
Data Seeding: Seed databases with test data to avoid redundant setup and teardown operations for each test.
Debugging E2E Tests
Debugging E2E tests can be challenging due to their complexity. Here are some debugging tips:
-
Logging: Implement extensive logging in your test scripts to record steps and actions. This can help identify issues during execution.
-
Screenshots: Capture screenshots at critical points in your tests, especially when an error occurs. Visual evidence can help diagnose problems.
-
Interactive Debugging: Some E2E testing frameworks support interactive debugging. This allows you to pause and inspect the test environment while it's running.
-
Parameterization: Use parameterized tests to isolate and diagnose issues with specific test data.
Test Data Management Strategies
Efficient test data management is crucial for maintaining a stable and reliable testing environment. Consider these strategies:
-
Database Cleanup: Implement routines to clean up test data after test execution to keep the testing environment tidy.
-
Data Versioning: Version your test data to ensure compatibility with changing database schemas.
-
Data Generators: Use data generators to create a variety of test data with minimal effort.
-
Data Mocking: When appropriate, mock external data sources, such as APIs or third-party services, to reduce dependencies and improve test reliability.
-
Randomization: Incorporate randomness in your test data to mimic real-world variability.
These tips and tricks can help you make your E2E testing process more efficient, leading to quicker test execution and more accurate identification of issues in your web application.
10. Code Snippet Examples
To help you get started with E2E testing using Python and Playwright, here are some code snippet examples that illustrate key concepts. These examples cover basic interactions with web pages and provide a foundation for building more complex E2E test scripts.
Example 1: Opening a Web Page and Clicking a Button
from playwright.sync import sync_playwright
with sync_playwright() as p:
browser = p.chromium.launch()
context = browser.new_context()
page = context.new_page()
# Navigate to a webpage
page.goto("https://example.com")
# Find an element by CSS selector and click it
page.click("button#myButton")
# Close the browser
context.close()
In this example, we launch a Chromium browser, navigate to a web page (https://example.com), and locate a button with the CSS selector "#myButton." We then click the button and close the browser context.
Example 2: Typing Text into a Form Field and Submitting
from playwright.sync import sync_playwright
with sync_playwright() as p:
browser = p.chromium.launch()
context = browser.new_context()
page = context.new_page()
# Navigate to a webpage with a form
page.goto("https://example.com/form-page")
# Find a form field by CSS selector and type text into it
page.type("input#username", "your_username")
page.type("input#password", "your_password")
# Submit the form
page.click("button[type='submit']")
# Close the browser
context.close()
This code navigates to a web page with a form, locates username and password input fields, types text into them, and then submits the form by clicking the submit button.
These examples provide a starting point for writing E2E tests using Python and Playwright. You can build upon them to create more comprehensive test scripts that cover various scenarios and edge cases.
11. Conclusion
In conclusion, End-to-End (E2E) testing is a crucial quality assurance process in web development. It ensures that your web application functions seamlessly from the user interface to the server, simulating real user interactions and validating the entire workflow. E2E testing is indispensable for detecting issues early, ensuring a smooth user experience, and preventing costly production bugs.
Python, in combination with the Playwright framework, provides a powerful toolset for conducting E2E testing. Playwright's cross-browser support, modern web automation capabilities, speed, and reliability make it an excellent choice for E2E testing.