Working with CSV Files: A Guide to Choosing the Right Library
By JoeVu, at: Oct. 5, 2023, 5:42 p.m.
Estimated Reading Time: __READING_TIME__ minutes

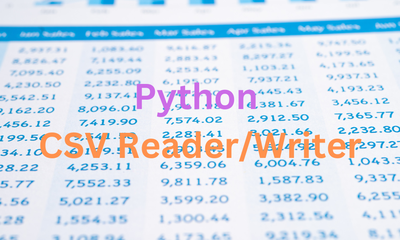
1. Introduction
CSV (Comma-Separated Values) files are a ubiquitous format for storing tabular data, and working with them programmatically is a common task for developers and data analysts. This article explores various Python libraries for parsing and manipulating CSV files, guiding you on selecting the right one for your specific needs.
A sample CSV file is
first_name,last_name,address,city,state,postal_code
John,Doe,120 jefferson st.,Riverside, NJ, 08075
Jack,McGinnis,220 hobo Av.,Phila, PA,09119
"John ""Da Man""",Repici,120 Jefferson St.,Riverside, NJ,08075
Stephen,Tyler,"7452 Terrace ""At the Plaza"" road",SomeTown,SD, 91234
,Blankman,,SomeTown, SD, 00298
"Joan ""the bone"", Anne",Jet,"9th, at Terrace plc",Desert City,CO,00123
2. Choose the Right Library
CSV Module (Built-in)
The CSV module is a built-in Python module that provides functionality for reading and writing CSV files. It is a lightweight option suitable for simple CSV tasks, offering basic functionalities without additional dependencies.
Pandas
Pandas, a powerful data manipulation library, is also proficient in handling CSV files. It provides a high-level interface for working with tabular data, making it an excellent choice for large datasets and complex data analysis tasks.
csvkit
csvkit is an external library that extends the functionality of the built-in CSV module. It offers additional features such as CSV file validation, SQL-like querying, and more. csvkit is a great choice when you need enhanced capabilities beyond the basic CSV functionalities.
Dask
Dask is a parallel computing library that integrates well with Pandas and is designed to handle larger-than-memory datasets. It can efficiently process and manipulate CSV files in parallel, making it suitable for big data scenarios.
3. Library Usage: Installation and Common Operations
CSV Module (Built-in)
Use Cases:
-
Read a CSV File:
import csv
with open('example.csv', 'r') as file:
reader = csv.reader(file)
data = list(reader) -
Write Data to CSV Files:
with open('new_data.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
Pandas
Installation:
pip install pandas
Use Cases:
-
Read a CSV File:
import pandas as pd
df = pd.read_csv('example.csv') -
Write Data to CSV Files:
df.to_csv('new_data.csv', index=False)
csvkit
Installation:
pip install csvkit
Use Cases:
-
Read a CSV File:
csvlook example.csv
-
Write Data to CSV Files:
csvformat -U 1 new_data.csv > formatted_data.csv
Dask
Installation:
pip install dask
Use Cases:
-
Read a CSV File:
import dask.dataframe as dd
df = dd.read_csv('example.csv') -
Write Data to CSV Files:
df.to_csv('new_data.csv', index=False, single_file=True)
4. How to Process a Big CSV File
Processing large CSV files efficiently is a common challenge. Here are examples using Pandas and Dask to handle big CSV files:
Pandas
Use Case: Read a Large CSV File in Chunks:
import pandas as pd
chunk_size = 100000 # Adjust the chunk size based on your system's memory
chunks = pd.read_csv('big_data.csv', chunksize=chunk_size)
for chunk in chunks:
# Process each chunk as needed
process_chunk(chunk)
Dask
Use Case: Parallel Processing for Big CSV Files:
import dask.dataframe as dd
df = dd.read_csv('big_data.csv')
# Perform computations in parallel
result = df.groupby('column_name').mean()
# Compute and get the result
result.compute()
5. Conclusion
Choosing the right library for working with CSV files depends on the complexity of your data and the specific tasks you need to perform. The built-in CSV module is suitable for simple operations, while Pandas and Dask offer advanced features for data analysis and handling large datasets. csvkit provides additional functionalities beyond the standard CSV module. Consider your project requirements to select the library that best fits your needs and efficiently manage CSV files in Python.