Working with Excel Files: A Guide to Choosing the Right Library
By hientd, at: Nov. 14, 2023, 11:33 p.m.
Estimated Reading Time: __READING_TIME__ minutes

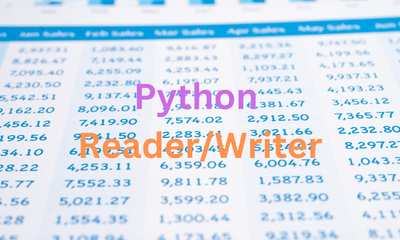
1. Introduction
Excel files are widely used for storing and organizing data, making them a common format for business and data analysis. Parsing, or reading and manipulating, Excel files programmatically is a crucial skill for many developers and data scientists. In this article, we will explore different Python libraries for parsing Excel files and guide you on choosing the right one for your needs.
2. Choose the Right Library
Openpyxl
Openpyxl is a powerful library for working with Excel files in Python. It supports both reading and writing Excel files and is compatible with Excel 2010 and later versions. Openpyxl is particularly useful for handling complex Excel files with various sheets and formatting.
Pandas
Pandas is a versatile data manipulation library that can also handle Excel files effortlessly. It provides a high-level interface for working with tabular data and seamlessly integrates with Excel. Pandas excels at data analysis tasks and is suitable for large datasets.
Xlsxwriter
Xlsxwriter is a Python module for creating Excel files, making it a good choice for scenarios where you need to generate new Excel files rather than parsing existing ones. It offers fine-grained control over formatting and is well-suited for creating reports and dashboards.
Xlrd
Xlrd is a lightweight library focused on reading data from Excel files. While it lacks the ability to write to Excel files, it is efficient in extracting data from existing workbooks. Xlrd is a good option for scenarios where you need a read-only solution with minimal dependencies.
3. Library Usage: Installation and Common Operations
Openpyxl
Installation:
pip install openpyxl
Use Cases:
-
Read an Excel File:
import openpyxl
wb = openpyxl.load_workbook('example.xlsx') sheet = wb.active -
Execute the Workbook:
# Perform operations on the workbook
wb.save('modified_example.xlsx') -
Read a Cell Value:
value = sheet['A1'].value
-
Write Data to Excel Files:
sheet['B1'] = 'New Data'
Pandas
Installation:
pip install pandas
Use Cases:
-
Read an Excel File:
import pandas as pd
df = pd.read_excel('example.xlsx') -
Read a Cell Value:
value = df.at[0, 'ColumnA']
-
Write Data to Excel Files:
df.to_excel('new_data.xlsx', index=False)
Xlsxwriter
Installation:
pip install xlsxwriter
Use Cases:
-
Read an Excel File: (Xlsxwriter is primarily for writing)
-
Execute the Workbook:
import xlsxwriter
workbook = xlsxwriter.Workbook('new_workbook.xlsx')
worksheet = workbook.add_worksheet() -
Read a Cell Value: (Xlsxwriter is primarily for writing)
-
Write Data to Excel Files:
worksheet.write('A1', 'Hello')
Xlrd
Installation:
pip install xlrd
Use Cases:
-
Read an Excel File:
import xlrd
workbook = xlrd.open_workbook('example.xls')
sheet = workbook.sheet_by_index(0) -
Execute the Workbook: (Xlrd is read-only)
-
Read a Cell Value:
value = sheet.cell_value(0, 0)
-
Write Data to Excel Files: (Xlrd is read-only)
4. How to Process a Big Excel File
Pandas
To handle large Excel files efficiently in Python, you can use the Pandas library along with the `chunksize` parameter. This allows you to read and process the Excel file in smaller chunks, preventing the entire file from being loaded into memory at once. Here's a simple example:
import pandas as pd
# Specify the chunk size based on your system's memory
chunk_size = 10000
# Create a Pandas ExcelFile object
excel_file = pd.ExcelFile('big_data.xlsx')
# Iterate through the chunks of the Excel file
for chunk in pd.read_excel(excel_file, chunksize=chunk_size):
# Process each chunk as needed
process_chunk(chunk)
Adjust the chunk_size
based on your system's memory constraints. This approach allows you to handle large Excel files without overwhelming your memory resources.
Openpyxl
To handle large Excel files efficiently using the Openpyxl library in Python, you can leverage the optimized read-only mode provided by the library. This mode allows you to access data without loading the entire workbook into memory. Here's a simple example:
from openpyxl import load_workbook
# Open the Excel file in read-only mode
workbook = load_workbook('big_data.xlsx', read_only=True)
# Access a specific sheet
sheet = workbook['Sheet1']
# Iterate through rows in the sheet
for row in sheet.iter_rows(min_row=1, max_row=sheet.max_row, values_only=True):
# Process each row as needed
process_row(row)
Using the read_only=True
parameter when loading the workbook ensures that only the necessary data is loaded into memory, making it more memory-efficient for handling large Excel files. Adjust the sheet name and processing logic based on your specific requirements.
5. Conclusion
Choosing the right library for parsing Excel files depends on your specific use case. Openpyxl and Pandas are robust choices for a wide range of tasks, while Xlsxwriter is suitable for creating new Excel files. If you need a lightweight read-only solution, Xlrd may be the right fit. Consider your project requirements and preferences to make an informed decision and streamline your Excel file parsing tasks in Python.