Working with JSON Files: A Guide to Choosing the Right Library
By khoanc, at: Nov. 17, 2023, 6:11 p.m.
Estimated Reading Time: __READING_TIME__ minutes

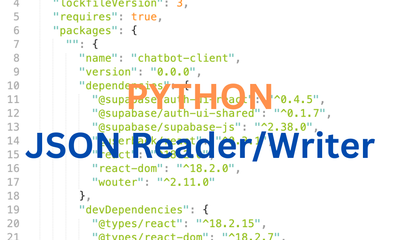
JSON (JavaScript Object Notation) files are a ubiquitous format for data interchange due to their simplicity and readability. Working with JSON files in Python is a common task for developers and data scientists. This guide explores various Python libraries for parsing and manipulating JSON files, assisting you in selecting the right one for your specific needs.
1. Introduction
JSON is a lightweight data interchange format that is easy for humans to read and write. Python provides several libraries to work with JSON data, each with its own strengths and use cases. Let's delve into the options available and understand how they can streamline your JSON file processing tasks.
2. Choose the Right Library
json (Built-in)
Python's built-in json
module provides functionality for encoding and decoding JSON data. It is a standard library module, making it readily available without the need for external installations. The json
module is suitable for basic JSON tasks and is a convenient option for projects with minimal dependencies.
simplejson
simplejson
is an external library that is compatible with the built-in json
module but offers additional features and performance improvements. It is a drop-in replacement for the json
module and is an excellent choice when advanced functionalities are required.
Pandas
Pandas, a popular data manipulation library, includes features for reading and writing JSON files. It is particularly powerful when working with JSON data in the context of larger datasets and complex data analysis tasks.
jq
jq
is a command-line JSON processor that allows for flexible and powerful JSON manipulation and extraction. While it is not a Python library, it is a valuable tool for handling JSON data directly from the command line.
ijson
ijson
is a Python library designed for parsing large JSON files incrementally. It's a powerful tool when dealing with big datasets, allowing you to process JSON data efficiently without loading the entire file into memory.
3. Library Usage: Installation and Common Operations
json (Built-in)
Use Cases:
-
Reading a JSON File:
import json
with open('example.json', 'r') as file:
data = json.load(file) -
Writing Data to a JSON File:
with open('new_data.json', 'w') as file:
json.dump(data, file)
simplejson
Installation:
pip install simplejson
Use Cases:
-
Reading a JSON File:
import simplejson as json
with open('example.json', 'r') as file:
data = json.load(file) -
Writing Data to a JSON File:
with open('new_data.json', 'w') as file:
json.dump(data, file)
Pandas
Installation:
pip install pandas
Use Cases:
-
Reading a JSON File:
import pandas as pd
df = pd.read_json('example.json') -
Writing Data to a JSON File:
df.to_json('new_data.json', orient='records')
jq
Installation:
# Installation depends on your operating system
# On Linux, you can use package managers like apt or yum
# On macOS, you can use Homebrew
# On Windows, you can download the binary from the jq website
Use Cases:
-
Selecting and Formatting Data (Command Line):
cat example.json | jq '.property | .nested_property'
-
Filtering and Transforming Data (Command Line):
cat example.json | jq '.[] | select(.age > 21) | {name, age}'
ijson
Installation:
pip install ijson
Use Case: Iterative Parsing for Large JSON Files:
import ijson
# Define a function to process each JSON object
def process_json_object(json_object):
# Implement your processing logic here
# Open the JSON file for iterative parsing
with open('big_data.json', 'r') as file:
# Create an ijson parser
parser = ijson.items(file, 'item') # 'item' is the JSON key to iterate over
# Iterate through the JSON objects
for json_object in parser:
process_json_object(json_object)
This approach using ijson.items()
allows you to iteratively parse through the JSON file, processing each JSON object as it is encountered. It efficiently handles large JSON files and ensures optimal memory usage during processing.
4. Conclusion
Choosing the right library for working with JSON files in Python depends on the complexity of your data and the specific tasks you need to perform. The built-in json
module is suitable for basic operations, while simplejson
provides additional features and performance improvements. Pandas
is an excellent choice for data analysis tasks involving JSON files, especially in the context of larger datasets. Tools like jq
offer powerful JSON manipulation directly from the command line, providing flexibility and convenience. When dealing with large JSON files, ijson
proves to be a valuable tool for efficient and memory-friendly processing. Consider your project requirements to select the library that best fits your needs and efficiently manage JSON files in Python.