Working with XML Files: A Guide to Choosing the Right Library
By JoeVu, at: Sept. 25, 2023, 6:06 p.m.

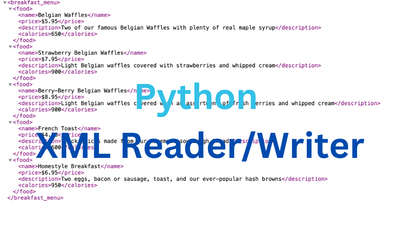
XML (eXtensible Markup Language) files are a common format for storing and exchanging structured data. As a developer or data enthusiast, efficiently parsing and manipulating XML files is a valuable skill. In this guide, we'll explore various Python libraries for working with XML files, helping you choose the right one for your specific needs.
1. Introduction
XML, with its human-readable structure, is widely used in diverse applications, including configuration files, data exchange between systems, and web services. To effectively work with XML files in Python, selecting the appropriate library is essential. Let's delve into the options available and understand their strengths and use cases.
2. Choose the Right Library
ElementTree (Built-in)
Python's standard library includes the xml.etree.ElementTree
module, providing a simple and efficient way to parse and create XML documents. It is suitable for basic XML processing tasks and is an excellent choice for projects with minimal external dependencies.
lxml
lxml is a third-party library that builds upon the ElementTree API, offering enhanced performance and additional features. It supports XPath, making it a powerful tool for complex XML processing tasks. If you need speed and versatility, lxml is a compelling choice.
minidom (Built-in)
The xml.dom.minidom
module is part of the standard library and provides a Document Object Model (DOM) interface for XML documents. While it's easy to use, minidom may not be the most memory-efficient option for large XML files.
3. Library Usage: Installation and Common Operations
ElementTree (Built-in)
Use Cases:
-
Parsing an XML File:
import xml.etree.ElementTree as ET
tree = ET.parse('example.xml')
root = tree.getroot() -
Accessing Elements:
for child in root:
print(child.tag, child.text) -
Modifying and Creating XML:
new_element = ET.Element('new_tag')
root.append(new_element)
tree.write('modified_example.xml')
lxml
Installation:
pip install lxml
Use Cases:
-
Parsing an XML File:
from lxml import etree
tree = etree.parse('example.xml')
root = tree.getroot() -
XPath Queries:
elements = tree.xpath('//element[@attribute="value"]')
-
Modifying and Creating XML:
new_element = etree.Element('new_tag')
root.append(new_element)
etree.ElementTree(root).write('modified_example.xml')
minidom (Built-in)
Use Cases:
-
Parsing an XML File:
from xml.dom import minidom
doc = minidom.parse('example.xml') -
Accessing Elements:
elements = doc.getElementsByTagName('element')
-
Modifying and Creating XML:
new_element = doc.createElement('new_tag')
doc.appendChild(new_element)
with open('modified_example.xml', 'w') as file:
doc.writexml(file)
4. How to Process a Big XML File
Processing large XML files efficiently in Python often involves techniques to avoid loading the entire file into memory. Here's a guide on handling big XML files using the ElementTree library:
ElementTree (Built-in)
Use Case: Iterative Parsing for Large XML Files:
import xml.etree.ElementTree as ET
# Define a function to process each element
def process_element(element):
# Implement your processing logic here
# Open the XML file for iterative parsing
with open('big_data.xml', 'rb') as file:
context = ET.iterparse(file, events=('start', 'end'))
# Turn off automatic cleanup to retain processed elements
context = iter(context)
_, root = next(context)
for event, element in context:
if event == 'end' and element.tag == 'your_target_element':
process_element(element)
# Clear the processed elements from memory
root.clear()
This approach allows you to iteratively parse through the XML file, processing each element as it is encountered. By periodically clearing the processed elements, you can manage memory efficiently.
5. Conclusion
Selecting the right XML processing library in Python depends on your project's complexity and performance requirements. ElementTree and its variants are suitable for basic tasks and are readily available in the standard library. For more advanced features and better performance, lxml is a robust choice. Consider your specific needs to choose the library that aligns with your XML file processing goals, ensuring efficiency and ease of use.