Pythonパッケージ BeautifulSoup のすべて
By JoeVu, at: 2023年8月22日21:52
Estimated Reading Time: __READING_TIME__ minutes
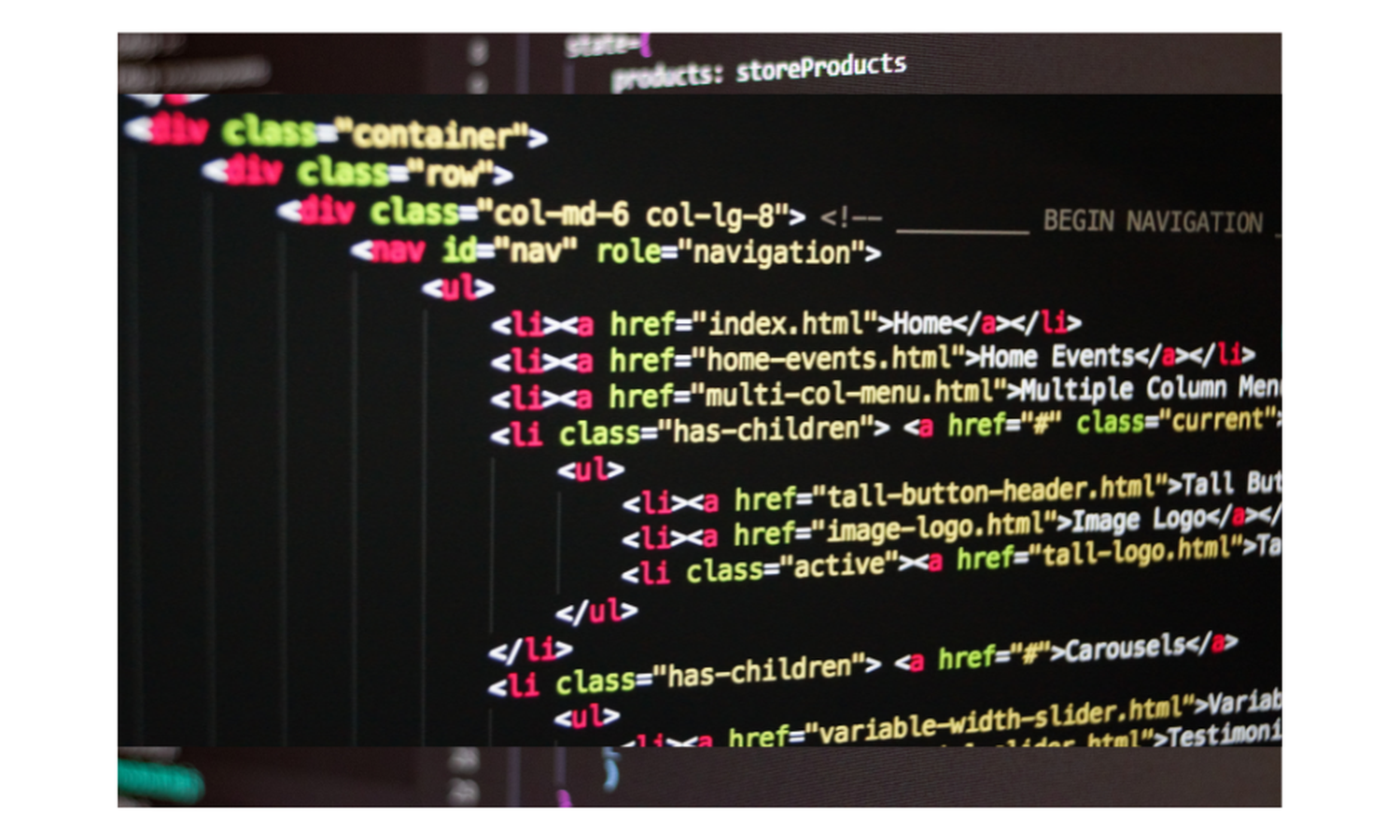
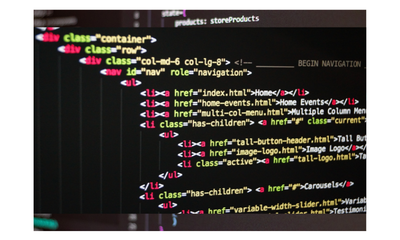
BeautifulSoup入門
PythonでWebスクレイピングやHTML文書の解析を行う場合、BeautifulSoupは最も強力で汎用性の高いツールの1つとして挙げられます。
この記事では、BeautifulSoupの長所と短所を詳しく調べ、その機能、利点、ベストプラクティス、そして厄介なエッジケースにも対処します。初心者でも経験豊富な開発者でも、BeautifulSoupを効果的に活用するための貴重な洞察を得ることができます。
BeautifulSoupおよび関連パッケージをインストールするには
pip install bs4 lxml
BeautifulSoupとは?
BeautifulSoupは、HTMLとXML文書の解析を専門とするPythonライブラリです。Webページから情報を抽出するプロセスを簡素化し、Webスクレイピング、データマイニング、コンテンツ抽出などのタスクに不可欠なツールとなります。ユーザーフレンドリーなAPIにより、開発者はHTML構造を容易にナビゲートして検索し、特定のデータの抽出、要素の操作、Webコンテンツの処理を伴うタスクの自動化を行うことができます。
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
soup.find('body')
主要機能と利点
シンプルなHTMLとXMLの解析
BeautifulSoupの主要な強みの1つは、複雑なHTMLやXML文書でも容易に解析できることです。不正な形式のコードや乱雑なコードも適切に処理するため、現実世界のWebページを扱う際に信頼できる選択肢となります。
xml_content = '''Book $35 Pen $24 Notebook $32 '''
soup = BeautifulSoup(content, 'xml')
print(soup.find('name').text)
パースツリーのナビゲーション
BeautifulSoupは、HTML文書の階層構造を反映したパースツリーを構築します。このツリーのような表現により、開発者は直感的なメソッドを使用して要素をナビゲートし、目的のコンテンツにアクセスして操作するための明確な方法を提供します。
soup.items.item
コンテンツの検索とフィルタリング
BeautifulSoupを使用すると、HTML文書内の特定の要素を簡単に検索できます。そのfind()
メソッドとfind_all()
メソッドにより、タグ名、属性、またはテキストコンテンツに基づいて正確なコンテンツを取得できます。より高度なクエリには、CSSセレクターを使用して簡単に要素をターゲットにすることができます。
soup.find_all('name')
基本的な使用方法と解析
HTMLコンテンツの解析は、BeautifulSoupを使用する基礎です。基本的な例を以下に示します。
import requests
from bs4 import BeautifulSoup
url = 'https://example.com/'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
paragraph = soup.p
print(paragraph.get_text()) # 出力: "This domain is for use in illustrative examples in documents. You may use this
domain in literature without prior coordination or asking for permission."
タグ名を使用したナビゲーション
first_heading = soup.h1
print(first_heading.get_text())
ツリーの上下移動
parent_element = paragraph.parent
next_sibling = paragraph.next_sibling
find()
とfind_all()
の使用
first_link = soup.find('a') all_paragraphs = soup.find_all('p')
高度な検索のためのCSSセレクター
important_elements = soup.select('.important')
属性に基づくフィルタリング
img_with_alt = soup.find_all('img', alt=True)
パースツリーの変更
new_tag = soup.new_tag('b')
new_tag.string = "Bold text"
first_paragraph.append(new_tag)
HTMLの再フォーマットと整形
prettified_html = soup.prettify()
不正なHTMLの処理
# 不完全なタグの処理
soup = BeautifulSoup('
不完全な壊れたHTML<
', 'html.parser')
print(soup.prettify())
ベストプラクティスとヒント
BeautifulSoupを使用する際には、次のベストプラクティスを考慮してください。
エラー処理のためのTry-Exceptの使用
try:
title = soup.title.text
except AttributeError:
title = "タイトルが見つかりません"
過剰な解析の回避
parsed_paragraphs = soup.find_all('p')
フィルタリングのためのリスト内包表記の使用
important_paragraphs = [p for p in parsed_paragraphs if 'important' in p.get('class', [])]
動的なWebコンテンツの処理
# 動的に生成されたコンテンツにはSeleniumを使用
from selenium import webdriver
browser = webdriver.Chrome()
browser.get('https://example.com')
page_source = browser.page_source
soup = BeautifulSoup(page_source, 'html.parser')
非同期リクエストの処理
# 非同期リクエストにはaiohttpを使用
import aiohttp
import asyncio
async def fetch_html(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.text()
loop = asyncio.get_event_loop()
html_content = loop.run_until_complete(fetch_html('https://example.com'))
soup = BeautifulSoup(html_content, 'html.parser')
JavaScriptでレンダリングされたページからのデータの解析
# JavaScriptのレンダリングにはSplashを使用
import requests
from bs4 import BeautifulSoup
url = 'https://quotes.toscrape.com/'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
現実世界の例
BeautifulSoupは、さまざまな現実世界のシナリオで応用されています。
Webサイトからのデータのスクレイピング
import requests
from bs4 import BeautifulSoup
url = 'https://quotes.toscrape.com/'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
データ収集タスクの自動化
# 複数のページからのデータ抽出を自動化
for page_num in range(1, 6):
url = f'https://quotes.toscrape.com/page/{page_num}/'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser') # データを処理する
結論
WebスクレイピングとHTML解析の分野では、BeautifulSoupは開発者にとって信頼できる仲間として登場します。直感的なAPI、堅牢な解析機能、複雑なシナリオへの対応により、不可欠なツールとなっています。ベストプラクティスに従い、その機能を理解し、現実世界の例から学ぶことで、Webコンテンツ抽出のニーズに合わせてBeautifulSoupの潜在能力を最大限に活用できます。
よくある質問
-
BeautifulSoupはXML文書の解析にも適していますか? はい、BeautifulSoupはHTMLとXMLの両方の文書の解析をサポートしており、さまざまな解析タスクに汎用性があります。
-
BeautifulSoupはJavaScriptで生成されたコンテンツを含むWebサイトを処理できますか? BeautifulSoupは主に静的コンテンツを解析しますが、SeleniumやSplashなどのツールと組み合わせることで、JavaScriptでレンダリングされたページを解析できます。
-
BeautifulSoupでCSSセレクターをタグ名よりも使用する利点は何ですか? CSSセレクターは、より柔軟で強力なクエリオプションを提供し、クラス、ID、属性などを基準にして要素をターゲットにすることができます。
-
入れ子になった要素から特定のデータを効率的に抽出するにはどうすればよいですか? BeautifulSoupのナビゲーションメソッド(
.find()
と.find_all()
など)と属性フィルタリングを組み合わせて使用することで、入れ子になった要素からデータを効果的に抽出できます。 -
BeautifulSoupはフォーマットの悪いHTMLを自動的に処理しますか? はい、BeautifulSoupの強みの1つは、フォーマットの悪いHTMLや乱雑なHTMLを適切に処理できることで、開発者は現実世界のWebページから情報を抽出できます。