Tất cả những gì bạn cần biết về gói BeautifulSoup của Python
By JoeVu, at: 21:52 Ngày 22 tháng 8 năm 2023
Thời gian đọc ước tính: __READING_TIME__ minutes
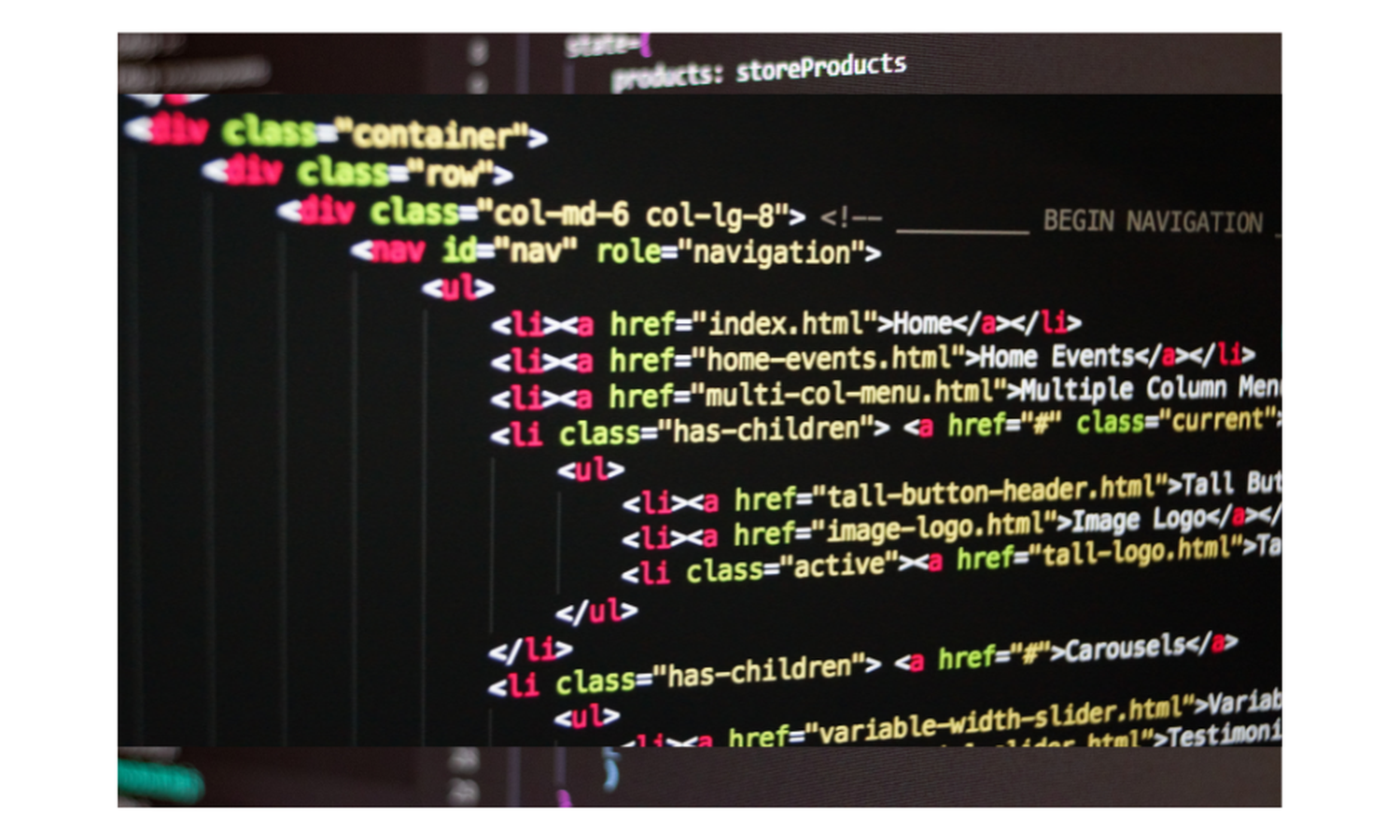
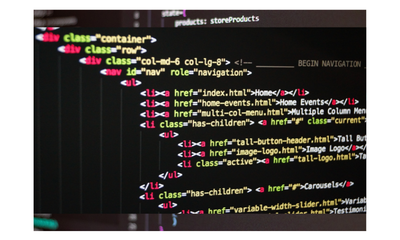
Giới thiệu về BeautifulSoup
Khi nói đến việc trích xuất dữ liệu web và phân tích cú pháp tài liệu HTML trong Python, BeautifulSoup được coi là một trong những công cụ mạnh mẽ và linh hoạt nhất.
Trong bài viết này, chúng ta sẽ đi sâu vào các ưu điểm và nhược điểm của BeautifulSoup, khám phá các tính năng, lợi ích, các thực tiễn tốt nhất, và thậm chí giải quyết một số trường hợp khó xử lý. Cho dù bạn là người mới bắt đầu hay là một nhà phát triển giàu kinh nghiệm, bạn sẽ tìm thấy những hiểu biết quý giá ở đây để giúp bạn sử dụng BeautifulSoup một cách hiệu quả.
Để cài đặt BeautifulSoup và các gói liên quan
pip install bs4 lxml
BeautifulSoup là gì?
BeautifulSoup là một thư viện Python chuyên về phân tích cú pháp tài liệu HTML và XML. Nó đơn giản hóa quá trình trích xuất thông tin từ các trang web, làm cho nó trở thành một công cụ vô giá cho các tác vụ như trích xuất dữ liệu web, khai thác dữ liệu và trích xuất nội dung. Với API thân thiện với người dùng, BeautifulSoup cho phép các nhà phát triển dễ dàng điều hướng và tìm kiếm cấu trúc HTML, giúp họ trích xuất dữ liệu cụ thể, thao tác các phần tử và tự động hóa các tác vụ liên quan đến xử lý nội dung web.
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
soup.find('body')
Các tính năng và lợi ích chính
Phân tích cú pháp HTML và XML đơn giản
Một trong những điểm mạnh chính của BeautifulSoup nằm ở khả năng phân tích cú pháp ngay cả các tài liệu HTML và XML phức tạp một cách dễ dàng. Nó xử lý mã được định dạng kém hoặc lộn xộn một cách khéo léo, làm cho nó trở thành một lựa chọn đáng tin cậy để xử lý các trang web thực tế.
xml_content = '''Book $35 Pen $24 Notebook $32 '''
soup = BeautifulSoup(content, 'xml')
print(soup.find('name').text)
Điều hướng cây phân tích cú pháp
BeautifulSoup xây dựng một cây phân tích cú pháp phản ánh cấu trúc phân cấp của tài liệu HTML. Mô tả dạng cây này cho phép các nhà phát triển điều hướng qua các phần tử bằng các phương pháp trực quan, cung cấp một cách rõ ràng để truy cập và thao tác nội dung mong muốn.
soup.items.item
Tìm kiếm và lọc nội dung
Tìm kiếm các phần tử cụ thể trong các tài liệu HTML rất dễ dàng với BeautifulSoup. Các phương thức find()
và find_all()
của nó cho phép truy xuất nội dung chính xác dựa trên tên thẻ, thuộc tính hoặc thậm chí nội dung văn bản. Để truy vấn nâng cao hơn, các bộ chọn CSS có thể được sử dụng để nhắm mục tiêu các phần tử một cách dễ dàng.
soup.find_all('name')
Sử dụng cơ bản và phân tích cú pháp
Phân tích cú pháp nội dung HTML là nền tảng của việc sử dụng BeautifulSoup. Đây là một ví dụ cơ bản:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com/'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
paragraph = soup.p
print(paragraph.get_text()) # Output: "This domain is for use in illustrative examples in documents. You may use this
domain in literature without prior coordination or asking for permission."
Điều hướng với tên thẻ
first_heading = soup.h1
print(first_heading.get_text())
Di chuyển lên và xuống cây
parent_element = paragraph.parent
next_sibling = paragraph.next_sibling
Sử dụng find()
và find_all()
first_link = soup.find('a') all_paragraphs = soup.find_all('p')
Bộ chọn CSS để tìm kiếm nâng cao
important_elements = soup.select('.important')
Lọc dựa trên thuộc tính
img_with_alt = soup.find_all('img', alt=True)
Sửa đổi cây phân tích cú pháp
new_tag = soup.new_tag('b')
new_tag.string = "Bold text"
first_paragraph.append(new_tag)
Định dạng lại và làm đẹp HTML
prettified_html = soup.prettify()
Xử lý HTML bị lỗi
# Xử lý các thẻ không đầy đủ
soup = BeautifulSoup('
HTML bị lỗi không đầy đủ<
', 'html.parser')
print(soup.prettify())
Thực tiễn tốt nhất và mẹo
Khi sử dụng BeautifulSoup, hãy xem xét các thực tiễn tốt nhất sau:
Sử dụng Try-Except để xử lý lỗi
try:
title = soup.title.text
except AttributeError:
title = "No title found"
Tránh phân tích cú pháp quá mức
parsed_paragraphs = soup.find_all('p')
Sử dụng List Comprehensions để lọc
important_paragraphs = [p for p in parsed_paragraphs if 'important' in p.get('class', [])]
Xử lý nội dung web động
# Sử dụng Selenium cho nội dung được tạo động
from selenium import webdriver
browser = webdriver.Chrome()
browser.get('https://example.com')
page_source = browser.page_source
soup = BeautifulSoup(page_source, 'html.parser')
Xử lý các yêu cầu không đồng bộ
# Sử dụng aiohttp cho các yêu cầu không đồng bộ
import aiohttp
import asyncio
async def fetch_html(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.text()
loop = asyncio.get_event_loop()
html_content = loop.run_until_complete(fetch_html('https://example.com'))
soup = BeautifulSoup(html_content, 'html.parser')
Phân tích cú pháp dữ liệu từ các trang được hiển thị bằng JavaScript
# Sử dụng Splash để hiển thị JavaScript
import requests
from bs4 import BeautifulSoup
url = 'https://quotes.toscrape.com/'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
Ví dụ thực tế
BeautifulSoup tìm thấy ứng dụng trong nhiều kịch bản thực tế:
Trích xuất dữ liệu từ các trang web
import requests
from bs4 import BeautifulSoup
url = 'https://quotes.toscrape.com/'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
Tự động hóa các tác vụ thu thập dữ liệu
# Tự động hóa việc trích xuất dữ liệu từ nhiều trang
for page_num in range(1, 6):
url = f'https://quotes.toscrape.com/page/{page_num}/'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser') # Xử lý dữ liệu
Kết luận
Trong lĩnh vực trích xuất dữ liệu web và phân tích cú pháp HTML, BeautifulSoup nổi lên như một người bạn đồng hành đáng tin cậy cho các nhà phát triển. API trực quan, khả năng phân tích cú pháp mạnh mẽ và hỗ trợ các kịch bản phức tạp làm cho nó trở thành một công cụ không thể thiếu. Bằng cách tuân theo các thực tiễn tốt nhất, hiểu các tính năng của nó và học hỏi từ các ví dụ thực tế, bạn sẽ khai thác được toàn bộ tiềm năng của BeautifulSoup cho nhu cầu trích xuất nội dung web của mình.
Câu hỏi thường gặp
-
BeautifulSoup có phù hợp để phân tích cú pháp tài liệu XML không? Có, BeautifulSoup hỗ trợ phân tích cú pháp cả tài liệu HTML và XML, làm cho nó linh hoạt cho nhiều tác vụ phân tích cú pháp khác nhau.
-
BeautifulSoup có thể xử lý các trang web có nội dung được tạo bằng JavaScript không? Mặc dù BeautifulSoup chủ yếu phân tích cú pháp nội dung tĩnh, nhưng nó có thể được kết hợp với các công cụ như Selenium hoặc Splash để phân tích cú pháp các trang được hiển thị bằng JavaScript.
-
Lợi thế của việc sử dụng bộ chọn CSS so với tên thẻ trong BeautifulSoup là gì? Bộ chọn CSS cung cấp các tùy chọn truy vấn linh hoạt và mạnh mẽ hơn, cho phép bạn nhắm mục tiêu các phần tử dựa trên lớp, ID, thuộc tính và hơn thế nữa.
-
Làm thế nào tôi có thể trích xuất dữ liệu cụ thể từ các phần tử lồng nhau một cách hiệu quả? Sử dụng các phương pháp điều hướng của BeautifulSoup, như
.find()
và.find_all()
, kết hợp với lọc thuộc tính, để trích xuất dữ liệu từ các phần tử lồng nhau một cách hiệu quả. -
BeautifulSoup có tự động xử lý HTML được định dạng kém không? Có, một trong những điểm mạnh của BeautifulSoup là khả năng xử lý HTML được định dạng kém hoặc lộn xộn một cách khéo léo, cho phép các nhà phát triển trích xuất thông tin từ các trang web thực tế.