Django Migration - A MUST known feature
By hientd, at: 09:31 Ngày 07 tháng 8 năm 2023
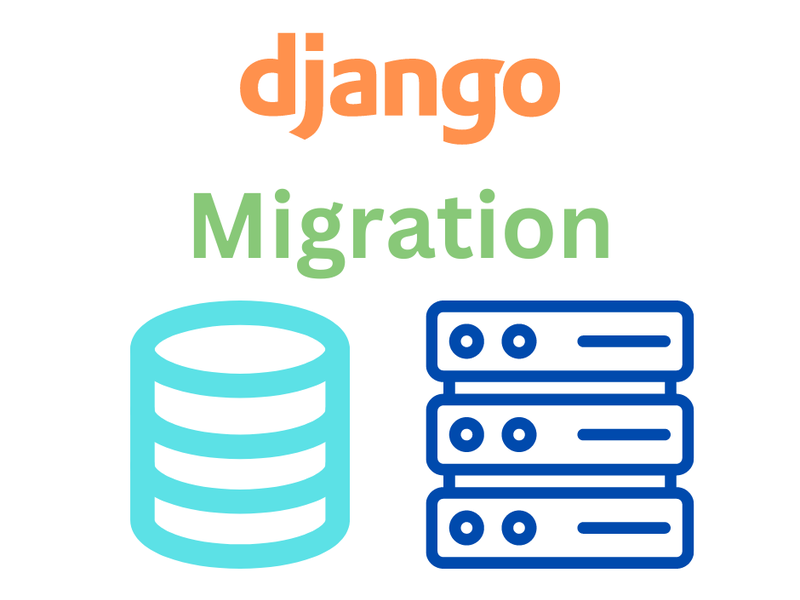
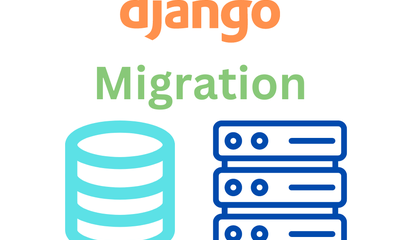
Welcome to the world of Django migrations, where database schema evolution becomes an automated breeze. Whether you're just starting out or aiming for mastery, this article will guide you through the various phases of Django migrations, from the straightforward to the complex. Let's dive in and uncover the tips, tricks, and best practices that will help you harness the full potential of Django migrations.
Another useful topic that we cover is here
Understanding Django Migrations
In the realm of web development, maintaining the consistency of your database schema is crucial. Django migrations are a set of tools that allow you to achieve this seamlessly - which is a built-in feature since Django 1.7. Previously, the Django-South is the main package to use for migrations.
These migrations enable you to make changes to your models – the Python classes that define your database structure – and automatically apply these changes to the database, ensuring its schema evolves alongside your codebase. This is especially significant as your application grows and evolves over time.
The migration process is essentially Django's answer to the question: "How can we keep our database schema in sync with our code changes?" This automation saves developers countless hours that would otherwise be spent manually altering the database schema.
The Easy Phase: Getting Started with Migrations
Starting on your migration journey is as easy as creating a new Django project and app. Once you've defined your models within an app, Django helps you generate the initial migration – a snapshot of your current models. Running the python manage.py makemigrations
command compiles this snapshot into a migration script. Subsequently, the python manage.py migrate
command applies these migrations to your database.
For instance, imagine creating a simple blog app. You define a Post
model with fields like title
, content
, and publish_date
. With a few simple commands, Django captures these changes and incorporates them into your database schema.
# models.py
from django.db import models
class Post(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
publish_date = models.DateTimeField()
def __str__(self):
return self.title
Navigating Through Common Scenarios
As you journey further into the world of migrations, you'll encounter various scenarios that demand schema modifications. Adding new fields, altering existing field properties, deleting obsolete fields – all of these are part of the game.
Let's say you decide to enhance your Post
model by introducing a category
field. This addition requires creating a new migration to reflect the change in your database schema. Similarly, if you wish to rename a field or modify its properties, migrations handle this seamlessly.
The original Post is
# models.py
from django.db import models
class Post(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
publish_date = models.DateTimeField()
name = models.CharField(max_length=200) # a duplicated field with title
def __str__(self):
return self.title
For example: Adding a new field or Removing a field
# models.py
from django.db import models
class Post(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
publish_date = models.DateTimeField()
category = models.CharField(max_length=100) # Adding the category field and removing the name field
def __str__(self):
return self.title
In order to generate migration file, we need to run
python manage.py makemigrations
python manage.py migrate
Dealing with Data Migrations
Migrations don't stop at just schema modifications. They also cover data migrations, ensuring that your data remains intact even as your schema evolves. When you need to manipulate data alongside a schema change, data migrations come to the rescue.
Consider a scenario where you're adding a new field, author
, to your Post
model. This field needs to be populated with data, and a data migration script allows you to achieve this while applying the schema change.
For example, if the string "Conan Doyle" exists in the Post content, then the author is "Connan Doyle". The migration script to update the author value is
# Generated by Django A.B on YYYY-MM-DD HH:MM
from django.db import migrations
def update_author(apps, schema_editor):
Post = apps.get_model("blogapp", "Post")
known_authors = ["Conan Doyle", "Stephen King", "William Shakespeare"]
for post in Post.objects.all():
if known_author in post.content:
post.author = known_author
break
post.save(update_fields=["author"])
class Migration(migrations.Migration):
dependencies = [
("blogapp", "0004_add_author_field"),
]
operations = [
# omit reverse_code=... if you don't want the migration to be reversible.
migrations.RunPython(update_author, reverse_code=migrations.RunPython.noop),
]
Intermediate Challenges and Solutions
As you progress, you'll face more intricate challenges. Handling model relationships, renaming models or apps, and dealing with circular dependencies might seem daunting, but Django migrations have you covered.
Imagine you already has a model Post with author
field as a CharField
. Now we would like to create a new model Author
to keep track of all authors and use ForeignKey
in the Post
model.
What we have to do is:
1. Change the field name from author
to author_name
then run python manage.py makemigrations then python manage.py migrate
2. Add new model Author
, and author
ForeignKey
to model Post
(null=True, blank=True
)
from django.db import models
class Author(models.Model):
name = models.CharField(max_length=100)
class Post(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
publish_date = models.DateTimeField()
category = models.CharField(max_length=100)
author = models.ForeignKey('Author', on_delete=models.CASCADE, related_name="posts", null=True, blank=True)
def __str__(self):
return self.title
3. Add new migration to populate data correctly
# In your data migration file (blogapp/migrations/xxxx_data_migration.py)
from django.db import migrations
def populate_authors(apps, schema_editor):
Post = apps.get_model('blogapp', 'Post')
Author = apps.get_model('blogapp', 'Author')
for post in Post.objects.all():
author, _ = Author.objects.get_or_create(name=post.author_name)
book.author = author
book.save()
class Migration(migrations.Migration):
dependencies = [
('blogapp', '0002_rename_author_field'),
]
operations = [
migrations.RunPython(populate_authors),
]
4. Remove null=True, blank=True
in the field author (model Post). Remove field author_name
class Post(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
publish_date = models.DateTimeField()
category = models.CharField(max_length=100)
author = models.ForeignKey('Author', on_delete=models.CASCADE, related_name="posts")
def __str__(self):
return self.title
Advanced Techniques and Best Practices
To truly master migrations, advanced techniques come into play.
Version control becomes invaluable, allowing you to track changes to your migration history effectively.
Squashing migrations, which involves combining multiple migrations into one, helps maintain a cleaner and more manageable history.
Optimizing database queries within migrations is also a skill worth honing. Ensuring your queries are efficient can prevent bottlenecks during migration execution.
Fake migration is a great tool to manage resolve inconsistency between Django application source code and the database change. As many teams are working on the same database, some might alter the database schema and we need to make sure that the Django application following.
Migration Testing and Rollback
Testing your migrations is crucial to prevent issues down the line. Django allows you to simulate migrations on a test database, ensuring everything works as expected before applying changes to your production environment.
In case a migration fails or leads to unexpected consequences, having a rollback strategy is essential. Django's migration framework provides tools to revert migrations and restore your database to a stable state.
python manage.py migrate blogapp 0001
python manage.py migrate blogapp
python manage.py migrate blogapp 0003
Mastering Migrations: Performance and Optimization
Reaching the mastery level involves not only understanding the basics but also optimizing the entire process. Profiling migration performance helps identify areas for improvement. Splitting large migrations into smaller ones minimizes the risk of errors and improves maintainability.
Leveraging database transactions effectively can significantly speed up migration execution, especially for large datasets.
One well-known issue with the Django migraiton is the slowness of adding index in big tables. As Django add index without CONCURRENTLY option. To resolve this, we should manually create CONCURRENTLY INDEX, then migrate with fake
option
python manage.py migrate blogapp 0004 --fake
Conclusion
From the straightforward initiation of migrations to mastering optimization techniques, this journey is a transformative one. Django migrations are not just a technical tool; they're a vital aspect of maintaining a robust and evolving codebase.
So, embrace the challenges, experiment with the solutions, and let Django migrations be your companion in the ever-evolving landscape of web development.
Useful Packages for Database
FAQs
- Question 1: Can migrations lead to data loss?
- A: No, migrations are designed to preserve data integrity during schema changes.
- Question 2: How can I handle circular dependencies between models in migrations?
- A: By using the
apps.get_model
function and careful ordering of migrations.
- A: By using the
- Question 3: Is squashing migrations always necessary?
- A: Squashing can improve the clarity of your migration history, but it's not mandatory.
- Question 4: Are migrations reversible?
- A: Yes, most migrations can be reversed using the
migrate
command.
- A: Yes, most migrations can be reversed using the
Remember that mastery takes time, practice, and a willingness to learn from challenges. Happy migrating!