Exploring FastAPI: All you need to know
By JoeVu, at: 10:55 Ngày 25 tháng 4 năm 2024
Thời gian đọc ước tính: __READING_TIME__ minutes
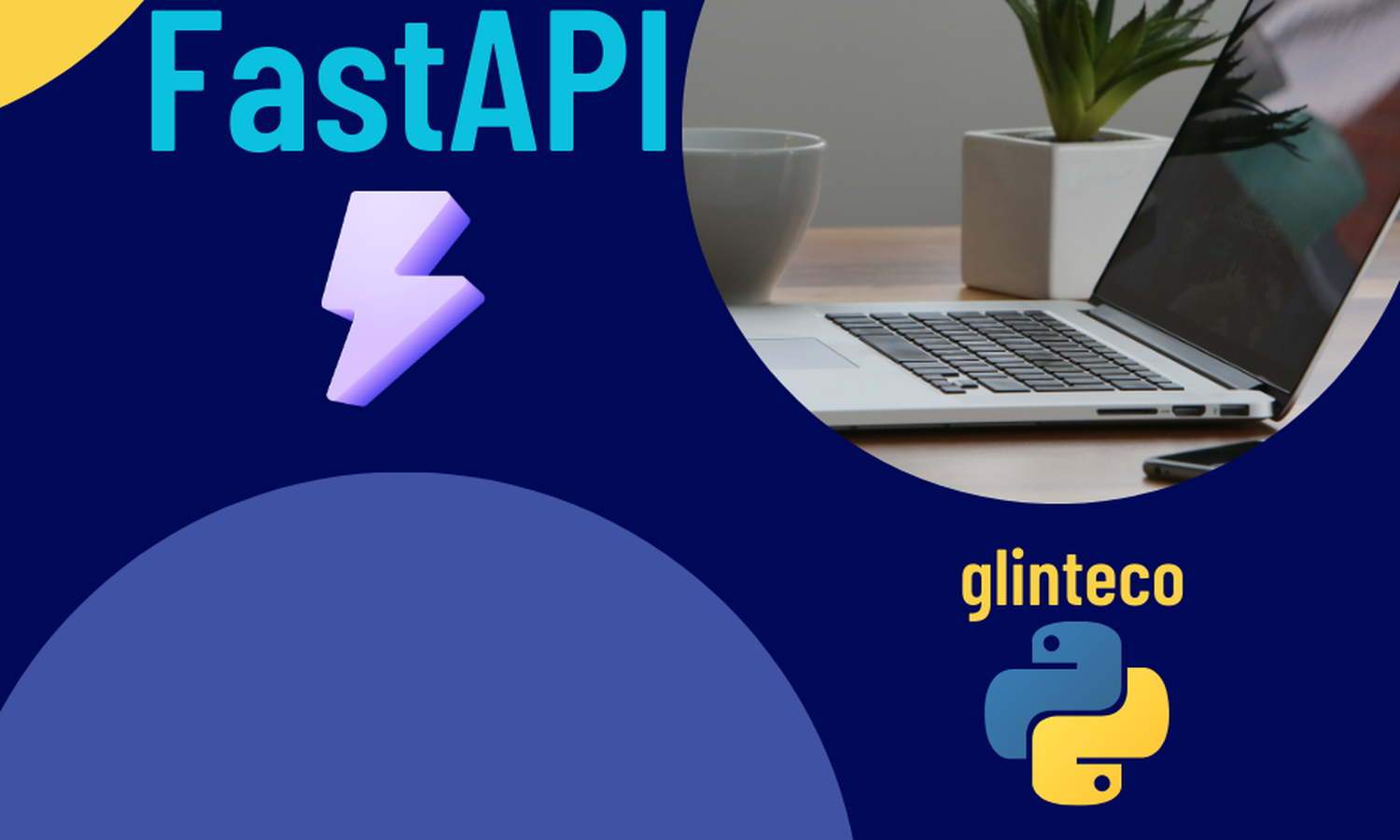
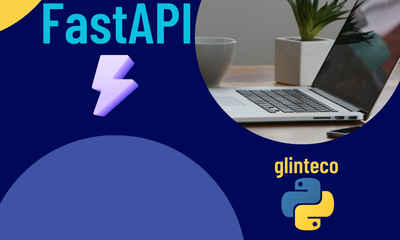
Exploring FastAPI: All you need to know
Today, FastAPI is a fast growing framework that has garnered attention for its speed, ease of use, and robust features. This article demonstrates the key aspects of FastAPI, offering insights and answering prevalent questions to help both novice and experienced developers navigate their choices in web frameworks.
Is FastAPI Worth Learning?
FastAPI is not only worth learning; it's a valuable addition to a developer's toolkit, especially for those focusing on building APIs or web applications with Python. It combines the best of modern Python features with performance that rivals NodeJS and Go, thanks to its asynchronous support and type checking.
Connecting SQLAlchemy with FastAPI
SQLAlchemy is a powerful ORM (Object Relational Mapper) for Python, and it integrates seamlessly with FastAPI, allowing developers to manage databases elegantly. Here's a simple example to connect SQLAlchemy with FastAPI:
from fastapi import FastAPI
from sqlalchemy import create_engine, MetaData
engine = create_engine("sqlite:///./test.db")
meta = MetaData()
conn = engine.connect()
app = FastAPI()
@app.on_event("startup")
async def startup():
# Create tables
meta.create_all(engine)
@app.get("/")
async def read_root():
return {"Hello": "World"}
This snippet establishes a connection to an SQLite database, demonstrating FastAPI's ability to easily integrate with SQLAlchemy for database operations.
Which ORM to Use with FastAPI?
While SQLAlchemy is a popular choice due to its comprehensive feature set and maturity, FastAPI is ORM agnostic. This means developers are free to choose any ORM they prefer.
Tortoise ORM is another excellent choice for asynchronous ORM operations, fitting well with FastAPI's async capabilities.
To me, Pydantic is the most widely used data validation library for Python.
Is FastAPI Slower Than Flask?
FastAPI is actually faster than Flask in most scenarios, primarily due to its asynchronous request handling. Flask, a synchronous framework, can be made asynchronous with additional tools, but FastAPI is designed with async capabilities from the ground up, providing better performance out-of-the-box.
Can FastAPI Replace Flask?
FastAPI can replace Flask for applications where asynchronous handling and speed are critical. However, Flask's simplicity and extensive ecosystem make it a better choice for smaller projects or those with specific dependencies on Flask extensions.
Should I Learn FastAPI or Flask?
The choice between FastAPI and Flask hinges on your project requirements and learning objectives. If you're starting and looking for simplicity, Flask might be the way to go. For building high-performance, modern web APIs, learning FastAPI would be more beneficial.
Is FastAPI Better Than Django?
FastAPI and Django serve different purposes. Django is a full-stack web framework with a vast ecosystem, suitable for complex web applications requiring an ORM, authentication, and templating out-of-the-box. FastAPI, while excellent for APIs and microservices, doesn't offer Django's breadth for full-stack development. Your choice should depend on the project's requirements.
Why is FastAPI So Popular?
FastAPI's popularity stems from its performance, ease of use, and robust features like automatic Swagger documentation, dependency injection, and Pydantic model support for data validation. Its design encourages writing clean, maintainable code, making development faster and more efficient.
What Companies Use FastAPI?
Several forward-thinking companies have adopted FastAPI, recognizing its performance and productivity benefits. Notable examples include Microsoft, Uber, and Netflix, which use FastAPI for various microservices and applications within their infrastructure.
Is FastAPI a REST API?
FastAPI is a web framework for building APIs, and while it can be used to create RESTful services, it's not limited to REST architecture. It supports GraphQL, WebSockets, and other protocols, making it a versatile choice for modern web applications.
How Does FastAPI Facilitate Asynchronous Programming?
FastAPI is built on Starlette and Pydantic, which are designed for asynchronous programming. This means it can handle many requests concurrently, significantly improving performance for I/O-bound applications. Here's an example of an asynchronous route in FastAPI:
from fastapi import FastAPI
import httpx
app = FastAPI()
@app.get("/async")
async def fetch_data():
async with httpx.AsyncClient() as client:
result = await client.get("https://example.com")
return result.json()
This example demonstrates how FastAPI makes it straightforward to perform asynchronous HTTP requests, thereby leveraging Python's async
and await
keywords for non-blocking operations.
Integrating WebSocket in FastAPI
WebSockets allow for bidirectional communication between the client and server. FastAPI simplifies the integration of WebSockets into your application:
from fastapi import FastAPI, WebSocket
app = FastAPI()
@app.websocket("/ws")
async def websocket_endpoint(websocket: WebSocket):
await websocket.accept()
while True:
data = await websocket.receive_text()
await websocket.send_text(f"Message text was: {data}")
This code snippet showcases how to set up a basic WebSocket connection in FastAPI, enabling real-time data exchange.
FastAPI with Docker: Best Practices
Containerizing FastAPI applications with Docker enhances portability and scalability. Here's a minimal Dockerfile for FastAPI:
# Use an official Python runtime as a parent image
FROM python:3.8-slim
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install --no-cache-dir -r requirements.txt
# Make port 80 available to the world outside this container
EXPOSE 80
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["uvicorn", "main:app", "--host", "0.0.0.0", "--port", "80"]
This Dockerfile outlines a basic setup for running a FastAPI application, showcasing the ease of deploying FastAPI apps in containerized environments.
FastAPI for Microservices: Why and How?
FastAPI's design caters well to microservices architecture, thanks to its scalability, performance, and support for asynchronous programming. Microservices built with FastAPI can easily communicate with each other using HTTP requests or message brokers, while the framework's scalability ensures that each service can handle a high volume of requests.
How to Implement Authentication in FastAPI?
FastAPI provides several tools to implement authentication, including OAuth2 with Password (and hashing), JWT tokens, and more. Here's a brief snippet on setting up OAuth2 password flow:
from fastapi import Depends, FastAPI
from fastapi.security import OAuth2PasswordBearer
app = FastAPI()
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token")
@app.post("/token")
async def token():
return {"token": "your_token"}
@app.get("/users/me")
async def read_users_me(token: str = Depends(oauth2_scheme)):
return {"token": token}
This example shows how to define an OAuth2 scheme for token generation and verification, illustrating FastAPI's capabilities for secure authentication mechanisms.
FastAPI vs. Other Asynchronous Frameworks
While FastAPI shines for its speed and features, it's worth comparing it to other asynchronous frameworks like Sanic or Tornado. FastAPI stands out for its automatic OpenAPI documentation, dependency injection system, and type checking, offering a more comprehensive package for API development.
Transitioning from Flask or Django to FastAPI
Developers familiar with Flask or Django can transition to FastAPI by leveraging their understanding of Python web development fundamentals. The main adjustment involves adopting FastAPI's asynchronous features and type annotations, which can lead to performance improvements and more robust code.
The Future of FastAPI
As FastAPI continues to evolve, it's expected to gain more features, integrations, and community-driven enhancements. Its emphasis on speed, scalability, and developer experience positions FastAPI as a leading framework for modern web applications and APIs.
Conclusion
All in all, FastAPI represents a significant advancement in web development frameworks, offering speed, flexibility, and an array of features that cater to modern application development needs. Whether integrating with SQLAlchemy, choosing the right ORM, or comparing it with Flask and Django, FastAPI holds its ground as a compelling choice for Python developers. As the web development landscape continues to evolve, FastAPI's popularity among developers and companies alike is a testament to its value in the ecosystem.