Dọn dẹp và tối ưu hóa ứng dụng Flask
By khoanc, at: 11:05 Ngày 15 tháng 8 năm 2023
Thời gian đọc ước tính: __READING_TIME__ minutes

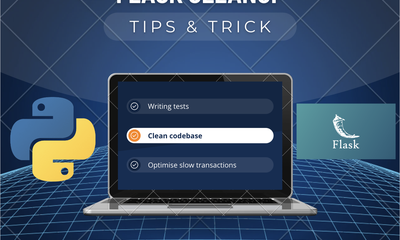
Chào mừng đến với thế giới dọn dẹp và tối ưu hóa ứng dụng Flask!
Trong hướng dẫn này, chúng ta sẽ khám phá các thực tiễn cần thiết để giữ cho các ứng dụng Flask của bạn hoạt động trơn tru, hiệu quả và không có sự lộn xộn không cần thiết. Một ứng dụng Flask được tối ưu hóa tốt không chỉ mang lại trải nghiệm người dùng tuyệt vời mà còn đảm bảo rằng codebase của bạn vẫn có thể duy trì và mở rộng. Vì vậy, hãy cùng tìm hiểu và khám phá các chiến lược tốt nhất để dọn dẹp và tối ưu hóa các ứng dụng Flask của bạn.
1. Hiểu về việc dọn dẹp ứng dụng Flask
Khi ứng dụng Flask của bạn xử lý các yêu cầu, nó tạo ra nhiều đối tượng và tài nguyên trong bộ nhớ. Theo thời gian, nếu các tài nguyên này không được quản lý và dọn dẹp đúng cách, chúng có thể dẫn đến rò rỉ bộ nhớ, hiệu suất chậm chạp và thậm chí là sự cố ứng dụng. Việc dọn dẹp đúng cách trở nên rất quan trọng để duy trì sức khỏe và tuổi thọ của ứng dụng của bạn.
Các gói hữu ích:
2. Các khu vực thường xuyên cần dọn dẹp
Các ứng dụng Flask liên quan đến một số khu vực cần dọn dẹp đúng cách:
2.1 Kết nối cơ sở dữ liệu
Khi tương tác với cơ sở dữ liệu, điều cần thiết là phải đóng các kết nối cơ sở dữ liệu khi chúng không còn cần thiết nữa. Nếu không làm như vậy có thể dẫn đến một nhóm các kết nối không được sử dụng, cuối cùng dẫn đến việc cạn kiệt tài nguyên.
Có hai gói phổ biến để quản lý cơ sở dữ liệu Flask: Flask-SQLAlchemy và Flask-Migrate. Cả hai đều hoạt động tốt và hỗ trợ quản lý kết nối cơ sở dữ liệu.
2.2 Xử lý tệp
Mở các tệp mà không đóng đúng cách có thể dẫn đến rò rỉ mô tả tệp. Hãy chắc chắn đóng các tệp bằng trình quản lý ngữ cảnh để ngăn chặn các rò rỉ này. Điều này có thể được sửa dễ dàng bằng câu lệnh with
trong Python.
# điều này không tốt my_file = open("hello.txt", "r") print(my_file.read())
# điều này tốt hơn nhiềuwith open("hello.txt", "w") as my_file: my_file.write("Hello world \n") my_file.write("I hope you're doing well today \n") my_file.write("This is a text file \n") my_file.write("Have a nice time \n")
2.3 Gọi API của bên thứ ba
Nếu ứng dụng Flask của bạn tương tác với các API bên ngoài, hãy đảm bảo rằng các kết nối đã được đóng và các tài nguyên đã được phát hành sau mỗi cuộc gọi API để tránh tiêu tốn tài nguyên không cần thiết.
Có một câu hỏi rò rỉ bộ nhớ phổ biến trong StackOverflow nữa.
2.4 Giao dịch chậm
Có nhiều lý do tại sao ứng dụng Flask ngày càng tệ hơn theo thời gian:
- Nhanh chóng và phá vỡ sau đó
- Tài nguyên nhân lực có hạn
- Ngân sách eo hẹp
- Hạn chót khắt khe
Điều này dẫn đến mã nguồn kém/lộn xộn và chất lượng hiệu suất thấp.
3. Quản lý bộ nhớ và thu gom rác
Python, ngôn ngữ đằng sau Flask, sử dụng cơ chế thu gom rác để tự động giải phóng bộ nhớ bị chiếm dụng bởi các đối tượng không còn được tham chiếu. Việc hiểu cách thức hoạt động này sẽ giúp bạn tối ưu hóa việc sử dụng bộ nhớ trong ứng dụng Flask của mình.
Kiểm tra điều này để biết thêm thông tin, tóm lại, Pythoon không phải là chuyên gia trong việc quản lý bộ nhớ, tuy nhiên, nó hỗ trợ mọi thứ đằng sau hậu trường để chúng ta không cần phải lo lắng.
4. Các thực tiễn tốt nhất để dọn dẹp ứng dụng Flask
Làm theo các thực tiễn tốt nhất này để giữ cho ứng dụng Flask của bạn gọn gàng và được tối ưu hóa:
4.1 Đóng kết nối cơ sở dữ liệu bằng Trình quản lý ngữ cảnh
@app.teardown_appcontext
def close_db(error):
if hasattr(g, 'db'):
g.db.close()
điều này có thể được thực hiện dễ dàng với gói Flask-SQLAlchemy
4.2 Phát hành tay cầm tệp
with open('file.txt', 'r') as file:
content = file.read() # Tệp sẽ tự động đóng khi khối lệnh kết thúc.
4.3 Sử dụng mô-đun atexit
import atexit
def cleanup():
# Thực hiện các tác vụ dọn dẹp ở đây
atexit.register(cleanup)
4.4 Triển khai trình trang trí dọn dẹp tùy chỉnh
def cleanup_resources(f):
def wrapper(*args, **kwargs):
result = f(*args, **kwargs) # Thực hiện các tác vụ dọn dẹp ở đây return result
return wrapper
4.5 Cấu trúc lại môi trường và thiết lập cấu hình
Khi ứng dụng phát triển, sẽ có nhiều biến môi trường và cấu hình dẫn đến thiết lập và điều khiển ứng dụng lộn xộn. Nhờ gói Python-dotenv, cấu hình môi trường ứng dụng được quản lý dễ dàng và sạch sẽ.
5. Tối ưu hóa trình xử lý tuyến đường
Trình xử lý tuyến đường là trung tâm của ứng dụng Flask của bạn. Để đảm bảo hiệu suất tối ưu, hãy xem xét các mẹo sau:
5.1 Giảm thiểu các phép tính không cần thiết
Tránh các phép tính dư thừa trong trình xử lý tuyến đường. Lưu trữ kết quả của các phép tính tốn kém nếu có thể.
@app.route("/") @cache.cached(timeout=50) def index(): return json.dumps({"a big dictionary": "value"})
5.2 Tận dụng bộ nhớ đệm cho dữ liệu được truy cập thường xuyên
Sử dụng các thư viện bộ nhớ đệm như Flask-Caching để lưu trữ và truy xuất dữ liệu được truy cập thường xuyên, giảm tải cho ứng dụng của bạn.
@cache.cached(timeout=50, key_prefix='all_comments') def get_all_comments(): comments = do_serious_dbio() return [x.author for x in comments] cached_comments = get_all_comments()
6. Hiệu quả kết xuất mẫu
Việc kết xuất mẫu hiệu quả đóng vai trò quan trọng trong việc tối ưu hóa ứng dụng:
6.1 Sử dụng kế thừa mẫu
Tận dụng kế thừa mẫu để giữ cho codebase của bạn DRY (Don't Repeat Yourself) và dễ dàng duy trì.
Mẫu con sẽ là
{% extends "base.html" %} {% block title %}Index{% endblock %} {% block head %} {{ super() }} {% endblock %} {% block content %}
6.2 Bộ nhớ đệm các mẫu đã được kết xuất
Bộ nhớ đệm các mẫu đã được kết xuất để giảm chi phí của việc kết xuất cùng một nội dung nhiều lần. Kiểm tra điều này để biết thêm chi tiết
7. Xử lý tệp tĩnh
Trong hầu hết các trường hợp, các tệp tĩnh được cấu hình nằm trong thư mục/thư mục tĩnh. Điều này đang bị quá tải và tiêu thụ một lượng lớn dữ liệu truyền trong ứng dụng hiện tại, điều này có thể được tránh/giảm thiểu bằng cách sử dụng gói Flask-S3
Việc xử lý tệp tĩnh hiệu quả khác góp phần vào trải nghiệm người dùng nhanh hơn:
7.1 Triển khai bộ nhớ đệm trình duyệt
Đặt các tiêu đề bộ nhớ đệm thích hợp để cho phép trình duyệt bộ nhớ đệm các tài sản tĩnh như CSS, JavaScript và hình ảnh.
7.2 Sử dụng Mạng phân phối nội dung (CDN)
Dỡ tải việc phân phối các tệp tĩnh sang CDN để thời gian tải nhanh hơn, đặc biệt là đối với người dùng toàn cầu. Điều này có thể được cấu hình dễ dàng bằng cách sử dụng dịch vụ Cloudflare
8. Cấu hình Gunicorn
Tối ưu hóa Gunicorn, một máy chủ HTTP phổ biến để chạy các ứng dụng Python, để có hiệu suất tốt hơn:
8.1 Thiết lập các tiến trình và luồng Worker
Điều chỉnh số lượng tiến trình và luồng worker dựa trên tài nguyên của máy chủ và tải dự kiến.
8.2 Quản lý thời gian chờ Worker
Cấu hình thời gian chờ worker để ngăn chặn các tiến trình bị kẹt và gây ra tắc nghẽn.
NUM_WORKERS=3
TIMEOUT=120
exec gunicorn ${DJANGO_WSGI_MODULE}:application \
--name $NAME \
--workers $NUM_WORKERS \
--timeout $TIMEOUT \
--log-level=debug \
--bind=127.0.0.1:9000 \
--pid=$PIDFILE
9. Trường hợp đặc biệt và các kịch bản không mong muốn
Xử lý các trường hợp đặc biệt đảm bảo ứng dụng của bạn vẫn mạnh mẽ:
9.1 Xử lý tải tệp lớn
Triển khai tải tệp chia nhỏ và xử lý tệp đúng cách để ngăn chặn sự cố bộ nhớ trong quá trình tải tệp lớn.
9.2 Ngăn ngừa rò rỉ bộ nhớ
Thường xuyên kiểm tra và phân tích mã của bạn để tìm các rò rỉ bộ nhớ tiềm ẩn, đặc biệt là trong các tiến trình chạy lâu.
10. Giám sát và lập hồ sơ
Giám sát và lập hồ sơ ứng dụng Flask của bạn để xác định các điểm nghẽn và các khu vực cần cải thiện:
10.1 Thanh công cụ gỡ lỗi Flask
Sử dụng các công cụ như Thanh công cụ gỡ lỗi Flask để có được thông tin chi tiết về các chu kỳ yêu cầu-phản hồi, truy vấn cơ sở dữ liệu, v.v.
10.2 Lập hồ sơ với cProfile
Sử dụng mô-đun cProfile
tích hợp sẵn của Python để xác định các điểm nghẽn hiệu suất trong mã của bạn.
11. Kết luận
Tối ưu hóa và dọn dẹp ứng dụng Flask của bạn có vẻ như là công việc thêm, nhưng đó là một khoản đầu tư mang lại lợi ích về hiệu suất, trải nghiệm người dùng và khả năng bảo trì. Bằng cách làm theo các thực tiễn tốt nhất được nêu trong hướng dẫn này, bạn có thể đảm bảo rằng Flask của bạn
12. Câu hỏi thường gặp
- Câu 1: Tại sao việc dọn dẹp ứng dụng Flask lại quan trọng? Đáp 1: Việc dọn dẹp đúng cách đảm bảo rằng ứng dụng Flask của bạn vẫn hiệu quả, không bị rò rỉ bộ nhớ và mang lại hiệu suất tối ưu. Việc bỏ qua việc dọn dẹp có thể dẫn đến sự chậm chạp và thậm chí là sự cố ứng dụng.
- Câu 2: Làm thế nào tôi có thể đóng các kết nối cơ sở dữ liệu trong Flask? Đáp 2: Bạn có thể sử dụng trình trang trí
@app.teardown_appcontext
để đóng các kết nối cơ sở dữ liệu tự động. Dưới đây là một ví dụ:
@app.teardown_appcontext
def close_db(error):
if hasattr(g, 'db'):
g.db.close()
- Câu 3: Ý nghĩa của việc kế thừa mẫu trong việc kết xuất hiệu quả là gì? Đáp 3: Kế thừa mẫu cho phép bạn tạo một mẫu cơ sở với các phần tử chung, giảm thiểu sự trùng lặp mã. Điều này dẫn đến mã sạch hơn và dễ bảo trì hơn.
- Câu 4: Làm thế nào tôi có thể tối ưu hóa Gunicorn cho ứng dụng Flask của mình? Đáp 4: Điều chỉnh số lượng tiến trình và luồng worker dựa trên tài nguyên của máy chủ và tải dự kiến. Ngoài ra, hãy quản lý thời gian chờ worker để ngăn chặn tắc nghẽn.
- Câu 5: Tại sao tôi nên xử lý các tệp tĩnh một cách hiệu quả? Đáp 5: Xử lý các tệp tĩnh đúng cách cải thiện thời gian tải trang. Triển khai bộ nhớ đệm trình duyệt và sử dụng CDN có thể cải thiện đáng kể trải nghiệm người dùng.
- Câu 6: Một số trường hợp đặc biệt cần xem xét trong việc tối ưu hóa ứng dụng Flask là gì? Đáp 6: Xử lý tải tệp lớn và ngăn ngừa rò rỉ bộ nhớ là những trường hợp đặc biệt quan trọng. Việc triển khai tải tệp chia nhỏ và thường xuyên kiểm tra rò rỉ được khuyến nghị.
- Câu 7: Làm thế nào tôi có thể giám sát và lập hồ sơ ứng dụng Flask của mình? Đáp 7: Các công cụ như Thanh công cụ gỡ lỗi Flask cung cấp thông tin chi tiết về các chu kỳ yêu cầu-phản hồi và truy vấn cơ sở dữ liệu. Sử dụng mô-đun
cProfile
tích hợp sẵn của Python để xác định các điểm nghẽn hiệu suất.
- Câu 8: Việc dọn dẹp có cần thiết cho các cuộc gọi API của bên thứ ba không? Đáp 8: Có, điều quan trọng là phải đóng các kết nối đúng cách và phát hành tài nguyên sau các cuộc gọi API của bên thứ ba. Nếu không làm như vậy có thể dẫn đến lãng phí tài nguyên.
- Câu 9: Tôi có thể tự động hóa các tác vụ dọn dẹp bằng mô-đun
atexit
không? Đáp 9: Có, bạn có thể sử dụng mô-đunatexit
để đăng ký các hàm sẽ được thực thi khi chương trình kết thúc. Điều này hữu ích để thực hiện các tác vụ dọn dẹp trước khi ứng dụng kết thúc.
- Câu 10: Lợi ích của việc sử dụng bộ nhớ đệm cho dữ liệu được truy cập thường xuyên là gì? Đáp 10: Bộ nhớ đệm làm giảm nhu cầu tính toán hoặc truy xuất dữ liệu nhiều lần, cải thiện thời gian phản hồi và giảm tải cho ứng dụng của bạn.