By hientd, at: 17:22 Ngày 01 tháng 12 năm 2022
Thời gian đọc ước tính: __READING_TIME__ minutes
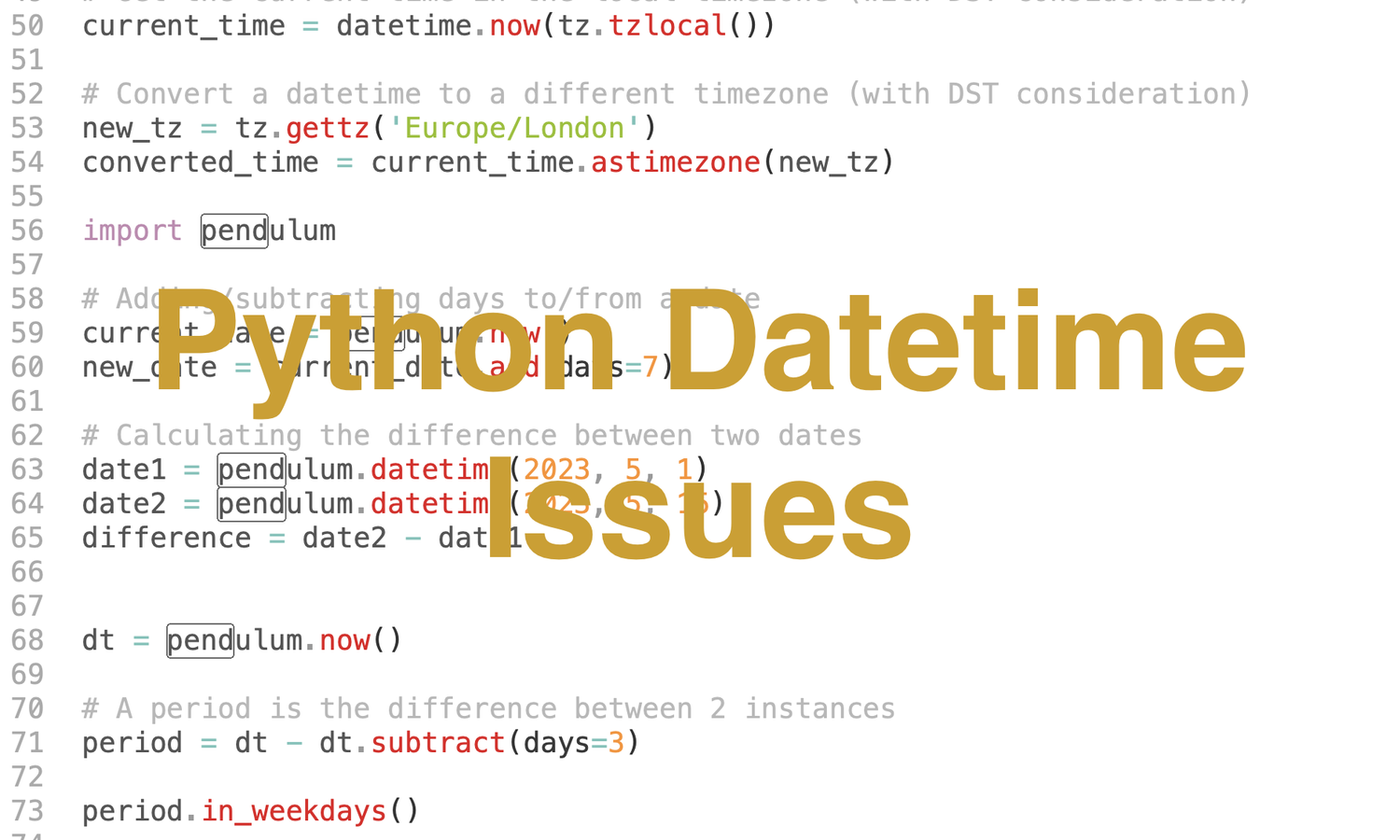
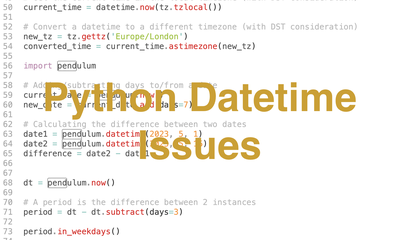
By hientd, at: 17:22 Ngày 01 tháng 12 năm 2022
Thời gian đọc ước tính: __READING_TIME__ minutes
Theo dõi bản tin của chúng tôi và không bao giờ bỏ lỡ những tin tức mới nhất.