How to Build a Web Scraper with Python and Selenium
By hientd, at: 11:54 Ngày 16 tháng 3 năm 2023
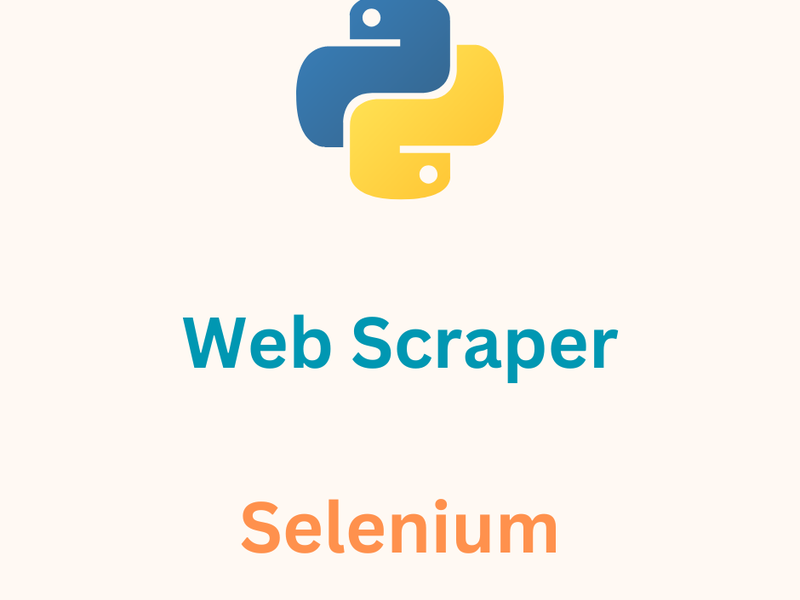
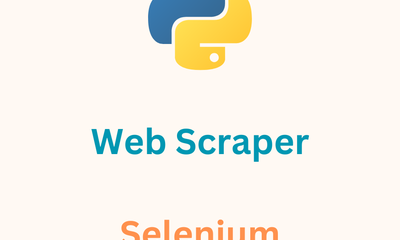
Web scraping is a technique that allows us to extract data from websites automatically. Python is a great programming language for web scraping, and it has many libraries that make the process easy. In this article, we will explore how to build a web scraper with Python and Selenium. Selenium is a powerful tool that can automate web browsers, making it perfect for web scraping. We will discuss how to install Selenium and configure it to work with Python. We will also cover the basics of web scraping with Selenium and demonstrate how to extract data from websites.
1. Setting up Selenium with Python
Before we can begin web scraping with Selenium, we need to set up our environment. The first step is to install Selenium. We can install Selenium using pip, which is a package manager for Python. Here is the command to install Selenium:
pip install selenium
Once we have installed Selenium, we also need to download a web driver that corresponds to the browser we want to automate. Selenium supports various browsers, including Chrome, Firefox, and Safari. For this example, we will use Chrome. Here is the link to download the ChromeDriver: https://chromedriver.chromium.org/downloads
Make sure to download the version that corresponds to the version of Chrome you have installed. Once we have downloaded the ChromeDriver, we need to add it to our system path. Here is an example of how to do this on Windows:
import os
from selenium import webdriver
os.environ["PATH"] += os.pathsep + "path/to/chromedriver"
driver = webdriver.Chrome()
2. Basic Web Scraping with Selenium
Now that we have set up our environment, we can begin web scraping with Selenium. The first step is to open a webpage using the get method. Here is an example:
driver.get("https://www.example.com")
Once we have opened a webpage, we can begin extracting data from it. One of the simplest ways to extract data is by using the find_element_by method. This method allows us to locate an element on the webpage using a variety of attributes, such as its ID, class, or name. Once we have located the element, we can extract its text using the text attribute. Here is an example:
element = driver.find_element_by_id("example_id")
text = element.text
print(text)
3. Basic Selenium features
Selenium is a powerful tool for automating web browsers and can be used for web scraping. Here are some basic functions and features of Selenium that can be used for web scraping:
- Opening a Web Page: Selenium can be used to open a web page in a browser. This can be done using the webdriver.get(url) function, where url is the URL of the web page.
- Finding Elements: Selenium can be used to find elements on a web page using CSS selectors, XPATH expressions, or by tag name. This can be done using the find_element_by_* functions, where * is the selector or tag name.
- Interacting with Elements: Selenium can be used to interact with elements on a web page, such as clicking buttons, entering text into forms, or scrolling. This can be done using the element.click(), element.send_keys(), or driver.execute_script() functions, respectively.
- Waiting: Selenium can be used to wait for elements to appear on a web page before interacting with them. This can be done using the WebDriverWait(driver, timeout).until() function, where driver is the Selenium webdriver instance and timeout is the maximum time to wait.
- Extracting Data: Selenium can be used to extract data from elements on a web page, such as text, attributes, or images. This can be done using the element.text, element.get_attribute(), or element.screenshot() functions, respectively.
Let's see an example of how to use these functions in practice. Suppose we want to scrape the title and description of a product from an e-commerce website.
Here's how we can do it using Selenium:
Examples
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# Set up Selenium webdriver
driver = webdriver.Chrome()
url = 'https://www.example.com/product/123'
driver.get(url)
# Wait for the title to load
title_element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.TAG_NAME, 'h1'))
)
# Extract the title and description
title = title_element.text
description_element = driver.find_element_by_css_selector('.description')
description = description_element.text
# Print the results
print(title)
print(description)
# Close the webdriver
driver.quit()
In this example, we first set up the Selenium webdriver and open the product page. We then use WebDriverWait to wait for the title to load, and then extract it using the text attribute. We also find the description element using a CSS selector, and extract its text. Finally, we print the results and close the webdriver.
Using these basic functions, you can scrape a wide range of web pages and extract valuable data for your projects.
4. Advanced Web Scraping with Selenium
Selenium is a powerful tool for web scraping, and with its advanced features, you can scrape even the most complex web pages. Here are some advanced features of Selenium that you can use for web scraping:
- Handling Frames and Windows: Selenium can be used to switch between frames and windows on a web page. This can be done using the switch_to.frame() and switch_to.window() functions, respectively.
- Handling Pop-ups and Alerts: Selenium can be used to handle pop-ups and alerts on a web page using the switch_to.alert() function.
- Executing JavaScript: Selenium can be used to execute JavaScript code on a web page using the execute_script() function. This can be useful for interacting with elements that are not directly accessible through the DOM.
- Capturing Screenshots and Videos: Selenium can be used to capture screenshots and videos of web pages using the screenshot() and get_screenshot_as_video() functions, respectively.
- Handling Cookies and Sessions: Selenium can be used to handle cookies and sessions on a web page using the add_cookie() and delete_all_cookies() functions, respectively.
Best Practices: When using Selenium for web scraping, it is important to follow best practices to avoid detection and to ensure the stability of your scraper. Some best practices include using a headless browser, using a user agent, and limiting the rate of requests.
Performance wise: Selenium can be slow when scraping large or complex web pages, especially when using a non-headless browser. To improve performance, you can use a headless browser, limit the number of requests, and optimize your code.
Tips and Tricks: Here are some tips and tricks for using Selenium for web scraping:
- Use the developer tools in your browser to inspect elements and find their selectors.
- Use the ActionChains class to simulate mouse and keyboard actions, such as scrolling or hovering over elements.
- Use the find_elements_* functions to find multiple elements on a web page, and iterate over them to extract data.
- Use the expected_conditions module to wait for specific events to occur on a web page, such as an element becoming visible or clickable.
Let's see an example of how to use some of these advanced features in practice. Suppose we want to scrape a website that requires authentication.
Here's how we can do it using Selenium:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# Set up Selenium webdriver
options = webdriver.ChromeOptions()
options.add_argument('--headless')
driver = webdriver.Chrome(options=options)
url = 'https://www.example.com/login'
driver.get(url)
# Log in
username_element = driver.find_element_by_name('username')
password_element = driver.find_element_by_name('password')
submit_element = driver.find_element_by_css_selector('.submit')
username_element.send_keys('my_username')
password_element.send_keys('my_password')
submit_element.click()
# Wait for the dashboard to load
dashboard_element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.CSS_SELECTOR, '.dashboard'))
)
# Scrape the data
data_elements = driver.find_elements_by_css_selector('.data')
data = []
for element in data_elements:
name_element = element.find_element_by_css_selector('.name')
value_element = element.find_element_by_css_selector('.value')
name = name_element.text
value = value_element.text
data.append((name, value))
# Print the results
print(data)
# Close the webdriver
driver.quit()
We first set up the Selenium webdriver with a headless Chrome browser, and navigate to the login page. We then locate the username, password, and submit elements on the page using their selectors, and enter our login credentials.
Next, we use the WebDriverWait function to wait for the dashboard element to load, and then scrape the data elements on the page using their selectors. We use the find_elements_by_* function to find multiple elements and iterate over them to extract the data we need.
Finally, we print the results and close the webdriver. Note that we use the quit() function to ensure that the webdriver is properly closed and resources are freed.
In terms of best practices, we used a headless browser to avoid detection and limit the rate of requests using the WebDriverWait function. We also used CSS selectors to locate elements, which can be more stable than using XPath selectors.
In terms of performance, we used a headless browser to speed up the scraping process, and optimized our code by using the find_elements_by_* function instead of repeatedly calling the find_element_by_* function.
5. Comparison with Other Tools
Feature | Selenium | PlayWright | Scrapy | requests and BeautifulSoup | Puppeteer | Cypress |
---|---|---|---|---|---|---|
Browser Support | Cross-platform, supports all major browsers | Chromium-based browsers | N/A | N/A | Chromium-based browsers | N/A |
JavaScript Support | Yes | Yes | No | No | Yes | Yes |
Parallel Requests | Yes | Yes | Yes | No | Yes | Yes |
Headless Mode | Yes | Yes | Yes | No | Yes | Yes |
Community Support | Large and active community | Growing community | Active community | Large community | Growing community | Growing community |
Learning Curve | Steep | Moderate | Moderate | Easy | Moderate | Easy |
Language Support | Multiple languages including Python, Java, and C# | Multiple languages including Python and JavaScript | Python | Python | JavaScript | JavaScript |
Ease of Installation | Requires installation of Selenium, webdriver, and browser driver | Requires installation of PlayWright and Chromium browser | Requires installation of Scrapy | Requires installation of requests and BeautifulSoup | Requires installation of Puppeteer and Chromium browser | Requires installation of Cypress |
Documentation | Extensive and well-documented | Growing documentation | Extensive and well-documented | Well-documented | Growing documentation | Growing documentation |
Community Support | Large and active community | Growing community | Active community | Large community | Growing community | Growing community |
Scalability | Can handle large and complex scraping tasks | Can handle large and complex scraping tasks | Can handle large and complex scraping tasks | Limited scalability for large and complex tasks | Can handle large and complex scraping tasks | Limited scalability for large and complex tasks |
Flexibility | Highly flexible and customizable | Highly flexible and customizable | Highly flexible and customizable | Limited flexibility for complex tasks | Highly flexible and customizable | Limited flexibility for complex tasks |
Integration with other tools | Integrates well with other Python-based tools and libraries | Integrates well with other JavaScript-based tools and libraries | Integrates well with other Python-based tools and libraries | Integrates well with other Python-based tools and libraries | Integrates well with other JavaScript-based tools and libraries | Integrates well with other JavaScript-based tools and libraries |
Speed | Can be slower due to use of a full browser | Can be faster due to use of a browser automation API | Can be faster than Selenium for simple tasks | Can be faster than Selenium for simple tasks | Can be faster due to use of a browser automation API | Can be slower due to use of a full browser |
6. Conclusion
In conclusion, building a web scraper with Python and Selenium is a useful skill for anyone who needs to extract data from websites. In this article, we've covered the basics of web scraping with Selenium, including setting up the Selenium environment, selecting elements on a webpage, and interacting with those elements. We also explored more advanced topics such as handling dynamic content, using headless mode, and improving scraping performance.
Additionally, we compared Selenium with other popular web scraping tools like PlayWright, Scrapy, Requests and BeautifulSoup, Puppeteer, and Cypress, discussing their respective features, strengths, and weaknesses. Choosing the right tool for your specific needs will depend on a variety of factors, including the complexity of the scraping task, the desired speed, and the language or environment you are comfortable working with.
With the knowledge gained from this article, readers should be able to build their own web scraper with Python and Selenium, and have a better understanding of the various tools available for web scraping. However, it's important to keep in mind the ethical considerations around web scraping and to always obtain data in a legal and ethical manner.