Thủ thuật Hoàn chỉnh về Chuỗi Hóa Dữ liệu trong Python
By khoanc, at: 14:01 Ngày 10 tháng 12 năm 2023
Thời gian đọc ước tính: __READING_TIME__ minutes

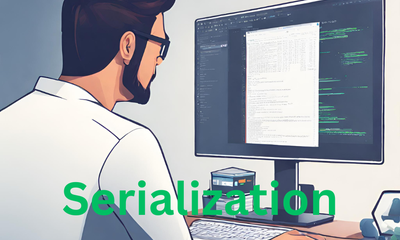
Trong bối cảnh xử lý dữ liệu khổng lồ, nơi petabyte và exabyte là chuẩn mực, việc xử lý, lưu trữ và truyền dữ liệu hiệu quả trở nên tối quan trọng. Một khía cạnh then chốt trong việc quản lý dữ liệu là tuần tự hóa (serialization), một quá trình giúp chuyển đổi cấu trúc dữ liệu trong bộ nhớ thành định dạng phù hợp để lưu trữ hoặc truyền tải. Hướng dẫn toàn diện này khám phá các khía cạnh khác nhau của tuần tự hóa Python, bao gồm cả định dạng dựa trên văn bản và nhị phân
1. Hiểu về Tuần tự hóa
Tuần tự hóa là chìa khóa để lưu, gửi và nhận dữ liệu trong khi vẫn giữ nguyên cấu trúc ban đầu. Python, giống như nhiều ngôn ngữ khác, cung cấp hỗ trợ tích hợp cho tuần tự hóa, một công cụ quan trọng trong lập trình hiện đại. Hai mục tiêu chính của tuần tự hóa là tính bền vững của dữ liệu và truyền dữ liệu hiệu quả
2. Định dạng Tuần tự hóa dựa trên Văn bản
2.1: Tuần tự hóa JSON
JSON (JavaScript Object Notation) là tiêu chuẩn trao đổi dữ liệu. Thư viện json
tích hợp sẵn của Python đơn giản hóa việc tuần tự hóa JSON.
Ví dụ:
import json
data = {'name': 'Joe', 'age': 30, 'city': 'Hanoi'}
# Tuần tự hóa
json_data = json.dumps(data) # '{"name": "Joe", "age": 30, "city": "Hanoi"}'
# Giải tuần tự hóa
loaded_data = json.loads(json_data) # {'name': 'Joe', 'age': 30, 'city': 'Hanoi'}
Kiểm thử đơn vị:
def test_json_serialization():
assert json.loads(json.dumps(data)) == data
Ưu điểm và Nhược điểm:
- Ưu điểm: Dễ đọc, được hỗ trợ rộng rãi, dễ sử dụng.
- Nhược điểm: Có thể không tiết kiệm dung lượng nhất cho các tập dữ liệu lớn.
2.2: Tuần tự hóa YAML
YAML (YAML Ain’t Markup Language) mở rộng khả năng đọc của JSON và giới thiệu các tính năng như tham chiếu.
Ví dụ:
import yaml
data = {'name': 'Joe', 'age': 30, 'city': 'Hanoi'}
# Tuần tự hóa
yaml_data = yaml.dump(data) # 'age: 30\ncity: Hanoi\nname: Joe\n'
# Giải tuần tự hóa
loaded_data = yaml.load(yaml_data, Loader=yaml.FullLoader) # {'age': 30, 'city': 'Hanoi', 'name': 'Joe'}
Kiểm thử đơn vị:
def test_yaml_serialization():
assert yaml.load(yaml.dump(data), Loader=yaml.FullLoader) == data
Ưu điểm và Nhược điểm:
- Ưu điểm: Dễ đọc, hỗ trợ tham chiếu và chú thích.
- Nhược điểm: Phức tạp hơn, tiềm ẩn vấn đề bảo mật.
3. Định dạng Tuần tự hóa Nhị phân
3.1: Tuần tự hóa Pickle
Pickle là định dạng tuần tự hóa riêng của Python, hỗ trợ nhiều loại đối tượng.
Ví dụ:
import pickle
book = {'price': 89, 'title': 'python django book', 'published_date': 2022}
# Tuần tự hóa
serialized_grades = pickle.dumps(grades) # b'\x80\x04\x95#\x00\x00\x00\x00\x00\x00\x00}\x94(\x8c\x05Alice\x94KY\x8c\x03Bob\x94KH\x8c\x07Charles\x94KWu.'
# Giải tuần tự hóa
received_grades = pickle.loads(serialized_grades) # {'Alice': 89, 'Bob': 72, 'Charles': 87}
Kiểm thử đơn vị:
def test_pickle_serialization():
assert pickle.loads(pickle.dumps(grades)) == grades
Ưu điểm và Nhược điểm:
- Ưu điểm: Hỗ trợ tích hợp, xử lý các đối tượng phức tạp.
- Nhược điểm: Vấn đề bảo mật, chỉ dành riêng cho Python.
3.2: Tuần tự hóa mảng NumPy
Mảng NumPy cung cấp tuần tự hóa hiệu quả cho các tập dữ liệu đa chiều lớn.
Ví dụ:
import numpy as np
data_array = np.array([[1, 2, 3, 4], [5, 6, 7, 8]])
# Tuần tự hóa
byte_output = data_array.tobytes() # b'\x01\x00\x00\x00\x00\x00\x00\x00\x02\x00\x00\x00\x00\x00\x00\x00\x03\x00\x00\x00\x00\x00\x00\x00\x04\x00\x00\x00\x00\x00\x00\x00\x05\x00\x00\x00\x00\x00\x00\x00\x06\x00\x00\x00\x00\x00\x00\x00\x07\x00\x00\x00\x00\x00\x00\x00\x08\x00\x00\x00\x00\x00\x00\x00'
# Giải tuần tự hóa
array_format = np.frombuffer(byte_output) # array([4.9e-324, 9.9e-324, 1.5e-323, 2.0e-323, 2.5e-323, 3.0e-323,
3.5e-323, 4.0e-323])
Kiểm thử đơn vị:
def test_numpy_serialization():
assert np.array_equal(np.frombuffer(data_array.tobytes()), data_array)
Ưu điểm và Nhược điểm:
- Ưu điểm: Hiệu quả đối với dữ liệu số, hỗ trợ các tập dữ liệu lớn.
- Nhược điểm: Định dạng nhị phân, có thể không dễ đọc.
4. Thực tiễn tốt nhất và những điểm cần lưu ý
Vấn đề bảo mật
- Khuyến nghị: Cẩn thận khi giải tuần tự hóa dữ liệu từ nguồn không đáng tin cậy.
- Ví dụ: Sử dụng các phương pháp bảo mật như
ast.literal_eval
hoặc các thư viện của bên thứ ba.
Khả năng tương thích phiên bản
- Khuyến nghị: Lưu ý về phiên bản Python và thay đổi thư viện.
- Ví dụ: Cân nhắc việc quản lý phiên bản hoặc sử dụng các định dạng chuẩn để lưu trữ lâu dài.
Truyền thông giữa các ngôn ngữ
- Khuyến nghị: Chọn định dạng tuần tự hóa tương thích với nhiều ngôn ngữ.
- Ví dụ: JSON, MessagePack, hoặc Protobuf để giao tiếp giữa các ngôn ngữ.
5. Vấn đề thường gặp
5.1 Vấn đề bảo mật
Giải tuần tự hóa dữ liệu từ các nguồn không đáng tin cậy có thể gây ra rủi ro bảo mật. Dữ liệu tuần tự hóa được tạo ra một cách độc hại có thể dẫn đến việc thực thi mã tùy ý, tiềm ẩn nguy cơ bị khai thác lỗ hổng bảo mật.
Hãy tưởng tượng ứng dụng nhận được dòng lệnh của người dùng, và nó được tuần tự hóa. Ứng dụng trích xuất và thực thi lệnh đó.
import pickle
import subprocess
# Vấn đề: Dữ liệu tuần tự hóa độc hại
malicious_data = b'\x80\x04\x95\x05\x00\x00\x00\x00\x00\x00\x00}\x94.'
# Giải tuần tự hóa có vấn đề
try:
commands = pickle.loads(malicious_data)
subprocess.run(commands) # chúng ta mong đợi rằng commands là "ls -al /home/user/"
# điều gì sẽ xảy ra nếu lệnh là "rm -rf /home/user/"
except Exception as e:
print(f"Vấn đề bảo mật: {e}")
5.2 Kích thước tệp và băng thông
Các định dạng tuần tự hóa dựa trên văn bản, chẳng hạn như JSON, có thể tạo ra kích thước tệp lớn hơn so với các định dạng nhị phân nhỏ gọn hơn, ảnh hưởng đến yêu cầu về dung lượng lưu trữ và băng thông.
import json
import pickle
import numpy as np
import time
# Dữ liệu mẫu để tuần tự hóa
data = {'key': 'value'}
numpy_array = np.random.random((1000, 1000))
# Vấn đề: Ảnh hưởng đến kích thước tệp và băng thông với các tập dữ liệu lớn
large_data = {'key': 'value'} * 10**10
# Tuần tự hóa JSON - Kích thước tệp lớn hơn
json_start_time = time.time()
json_data = json.dumps(large_data)
print(f"Thời gian tuần tự hóa JSON: {time.time() - json_start_time} giây")
print(f"Kích thước đã tuần tự hóa JSON: {len(json_data)} byte")
# Tuần tự hóa Pickle - Kích thước tệp nhỏ hơn
pickle_start_time = time.time()
pickle_data = pickle.dumps(large_data)
print(f"Thời gian tuần tự hóa Pickle: {time.time() - pickle_start_time} giây")
print(f"Kích thước đã tuần tự hóa Pickle: {len(pickle_data)} byte")
# Tuần tự hóa Numpy - Định dạng nhị phân nhỏ gọn
numpy_start_time = time.time()
numpy_data = numpy_array.tobytes()
print(f"Thời gian tuần tự hóa Numpy: {time.time() - numpy_start_time} giây")
print(f"Kích thước đã tuần tự hóa Numpy: {len(numpy_data)} byte")
5.2 Hiệu suất và hiệu quả
Các quá trình tuần tự hóa và giải tuần tự hóa, đặc biệt là với các tập dữ liệu lớn, có thể tốn nhiều tài nguyên tính toán và có thể ảnh hưởng đến hiệu suất.
import pickle
import time
# Vấn đề: Ảnh hưởng đến hiệu suất với các tập dữ liệu lớn
large_data = {'key': 'value'} * 10**6
# Tuần tự hóa chậm
start_time = time.time()
serialized_data = pickle.dumps(large_data)
print(f"Thời gian tuần tự hóa: {time.time() - start_time} giây")
6. Kết luận
Thủ thuật tuần tự hóa dữ liệu trong Python giúp các nhà phát triển quản lý dữ liệu hiệu quả trong nhiều trường hợp. Mỗi phương pháp tuần tự hóa đều có ưu điểm và nhược điểm riêng, và việc lựa chọn phương pháp phù hợp phụ thuộc vào trường hợp sử dụng cụ thể. Bằng cách hiểu rõ các sắc thái và thực tiễn tốt nhất của tuần tự hóa, các nhà phát triển có thể tối ưu hóa việc lưu trữ, truyền và truy xuất dữ liệu trong các ứng dụng Python của họ.