Tackling Infinite Loops in Django Model Save
By manhnv, at: 09:10 Ngày 07 tháng 4 năm 2024
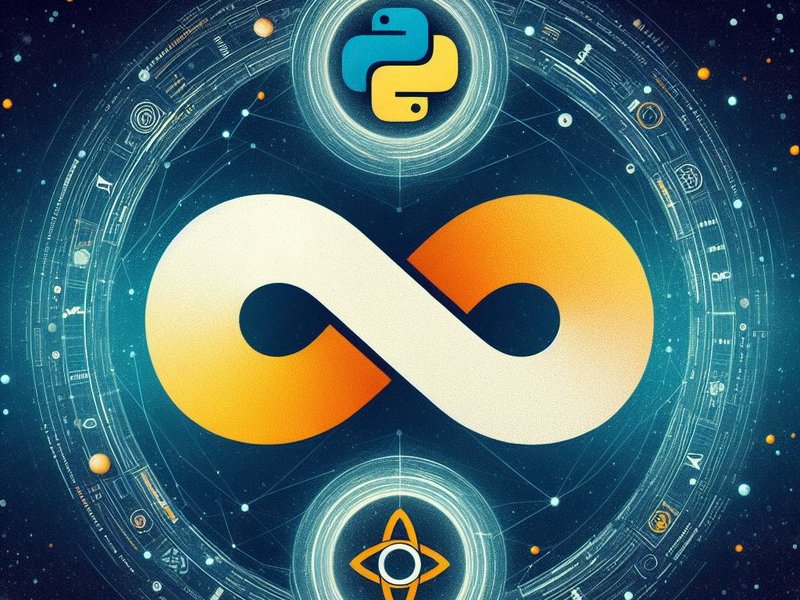

Tackling Infinite Loops in Django Model Save
Welcome to our latest blog post! Today, we’re researching the framework Django, a high-level Python web framework that encourages rapid development and clean, pragmatic design. Specifically, we’re going to explore a common issue that many Django developers encounter - infinite loops during model save operations.
Infinite loops can be a tricky problem, causing your application to freeze or consume excessive system resources. They occur when a sequence of instructions in a program loops endlessly, often due to a condition that never becomes false or is never met. In the context of Django, these infinite loops can sometimes occur during model save operations, leading to unexpected behavior and potential system issues.
In this blog post, we’ll define what an infinite loop is, discuss how to identify them, and delve into why they might occur when saving Django models. More importantly, we’ll provide you with solutions on how to prevent these infinite loops, ensuring your Django applications run smoothly and efficiently.
So, whether you’re a seasoned Django developer or just starting your journey, this blog post is for you. Let’s get started!
Understanding Infinite Loops
Definition of infinite loop:
An infinite loop is a sequence of instructions in a computer program which loops endlessly, either due to the loop having no terminating condition, having one that can never be met, or one that causes the loop to start over. To better visualize the infinite loop, let's look at the following example:
while True:
print("hello")
If you run the above command, because the while command's conditional expression is always True, the text hello will be printed to the screen forever:
hello
hello
hello
hello
hello
hello
hello
hello
hello
hello
.....
.....
Cause of infinite loop:
Infinite loops often occur due to several common programming errors, including:
- Wrong Stop Condition: When a loop's stop condition is set incorrectly or is not updated properly, the loop may never end.
- Missing Break or Return Command: In some cases, missing a
break
orreturn
command to exit the loop when the condition is met can lead to an infinite loop.
- Logical Errors in Code: Logical errors in code can cause infinite loops by incorrectly or incorrectly changing condition variables.
Infinite Loops When Saving Django Model
The save Method in Django Model
In Django, each model has a save
method. This method is used to save the current instance of the model into the database. When you call save
, Django performs an SQL INSERT
or UPDATE
command behind the scenes, depending on whether it’s a new instance or an existing one.
Overriding the save
Method
Sometimes, you might want to customize the save behavior of a model. This can be done by overriding the save
method. When you override save
, you can add extra logic before or after saving the model.
For example, you might want to automatically fill in a field, or update some cached value. Here’s an example:
class Blog(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
updated_at = models.DateTimeField(auto_now=True)
def save(self, *args, **kwargs):
self.title = self.title.upper()
super().save(*args, **kwargs) # Call the "super" save() method
In this example, every time a blog post is saved, its title is converted to uppercase.
Infinite Loops When Saving Django Model
However, you need to be careful when overriding the save
method. One common mistake is to cause an infinite loop by calling save
inside the overridden save
method. For example:
class Blog(models.Model):
title = models.CharField(max_length=200)
content = models.TextField(null=True, blank=True)
def save(self, *args, **kwargs):
self.title = self.title.upper()
super().save(*args, **kwargs)
if self.content:
self.title = self.content[:10]
self.save(update_fields=["title"]) # this will cause the save method to be called infinite in case the content has value
In this case, calling save
will call the save method of the parent class, then check if the content of the instance is existed, if yes, then the save method will be called again, and again, and again, cause an infinite loop.
Solutions to Prevent Infinite Loops in Django Model Save
Limit Overriding the save
Method
While overriding the save
method can be powerful, it also opens the door to potential pitfalls. Consider whether you truly need to override it. If possible, explore other hooks (such as signals) before resorting to direct save
method modifications.
Leverage Signals (Pre-Save and Post-Save)
- Purpose: The
pre_save
signal allows you to execute custom logic just before saving a model instance.
- Usage:
- Create a signal handler function (decorated with
@receiver(pre_save, sender=YourModel)
). - Perform any necessary checks or modifications.
- Avoid calling
save
within this signal handler to prevent recursion.
- Create a signal handler function (decorated with
- Example:
from django.db.models.signals import pre_save
from django.dispatch import receiver
@receiver(pre_save, sender=Blog)
def capitalize_title(sender, instance, **kwargs):
instance.title = instance.title.upper()
- Purpose: The
post_save
signal triggers after a model instance is saved.
- Usage:
- Similar to
pre_save
, create a signal handler function. - Use it for actions that should occur after the model instance has been successfully saved.
- Again, avoid calling
save
within this signal handler.
- Similar to
- Example:
from django.db.models.signals import post_save
from django.dispatch import receiver
@receiver(post_save, sender=Blog)
def notify_admin(sender, instance, **kwargs):
# Send an email or perform other post-save actions
pass
Rigorous Logic Review
If you must override the save
method, meticulously review your custom logic. Double-check for any unintentional recursive calls to save
. Debugging techniques like print statements or logging can help identify problematic cases.
class Blog(models.Model):
title = models.CharField(max_length=200)
content = models.TextField(null=True, blank=True)
def save(self, *args, **kwargs):
self.title = self.title.upper()
print("Calling super.save()...".upper())
super().save(*args, **kwargs)
print("Checking if content existed".upper())
if self.content:
print("Content is existed, edit the title and save".upper())
self.title = self.content[:10]
self.save(update_fields=["title"]) # this will cause the save method to be called infinite in case the content has value
print("Content is not existed".upper())
In the above code, I placed print
statements before executing each statement to understand how the code will run. If self.content
has a value, then my code will loop forever. The print
statements will alert me to this, allowing me to fix my code before deploying it to production.
Trick: If you pay attention, you will see that I use
.upper()
for all
Unit Testing
Thoroughly test your overridden save
methods. Cover various scenarios, including edge cases. Ensure that your custom logic behaves as expected without causing infinite loops.
from django.test import TestCase
from .models import Blog
# Create your tests here.
class BlogTestCase(TestCase):
def test_save_without_content(self):
blog = Blog.objects.create(title="Test Blog")
self.assertEqual(blog.content, None)
self.assertEqual(Blog.objects.all().count(), 1)
def test_save_with_content(self):
with self.assertRaises(RecursionError):
blog = Blog.objects.create(title="title", content="This is a test blog")
Also with the above code, I wrote 2 test cases for them. If the second test case runs OK
, it means my code is repeating infinitely, and needs to be fixed immediately, to avoid affecting production.
Conclusion
Infinite loops during model save operations can be a developer’s nightmare. They can freeze your application, hog system resources, and lead to unexpected behavior. However, armed with the right knowledge and strategies, you can prevent these loops and maintain a stable Django application.
Here’s a quick recap of our key takeaways:
- Think Twice Before Overriding
save
: While overriding thesave
method provides flexibility, it also introduces risks. Consider other hooks like signals before diving into direct modifications.
- Signals Are Your Allies:
- Use the
pre_save
signal for logic just before saving. - Leverage the
post_save
signal for actions after successful saves. - Avoid recursive calls to
save
within signal handlers.
- Use the
- Review Logic and Test Thoroughly:
- If you must override
save
, meticulously review your custom logic. - Write comprehensive unit tests to cover various scenarios.
- If you must override
Remember, preventing infinite loops isn’t just about code; it’s about thoughtful design and rigorous testing. So go forth, write efficient models, and keep those loops in check!
Happy coding!